A 3D vector in C++ is a mathematical representation of a point or direction in three-dimensional space, commonly implemented using structures or classes to store x, y, and z coordinates.
Here’s a simple code snippet demonstrating a basic 3D vector implementation in C++:
#include <iostream>
struct Vector3D {
float x, y, z;
Vector3D operator+(const Vector3D& v) {
return { x + v.x, y + v.y, z + v.z };
}
};
int main() {
Vector3D a {1.0f, 2.0f, 3.0f};
Vector3D b {4.0f, 5.0f, 6.0f};
Vector3D result = a + b;
std::cout << "Result: (" << result.x << ", " << result.y << ", " << result.z << ")\n";
return 0;
}
What is a 3D Vector?
Definition of a 3D Vector
A 3D vector is a mathematical construct that represents a quantity defined in three-dimensional space. It encompasses both direction and magnitude, making it a fundamental component in many mathematical, graphical, and physical applications. In simple terms, a vector can be thought of as an arrow pointing from one point to another in three-dimensional space.
Components of a 3D Vector
A 3D vector is composed of three fundamental components: x, y, and z. These components correspond to the coordinates of a point in 3D space relative to a defined origin.
For example, consider the vector:
Vector A = (3, 4, 5)
- x = 3
- y = 4
- z = 5
This vector points to the location (3, 4, 5) in a three-dimensional coordinate system.
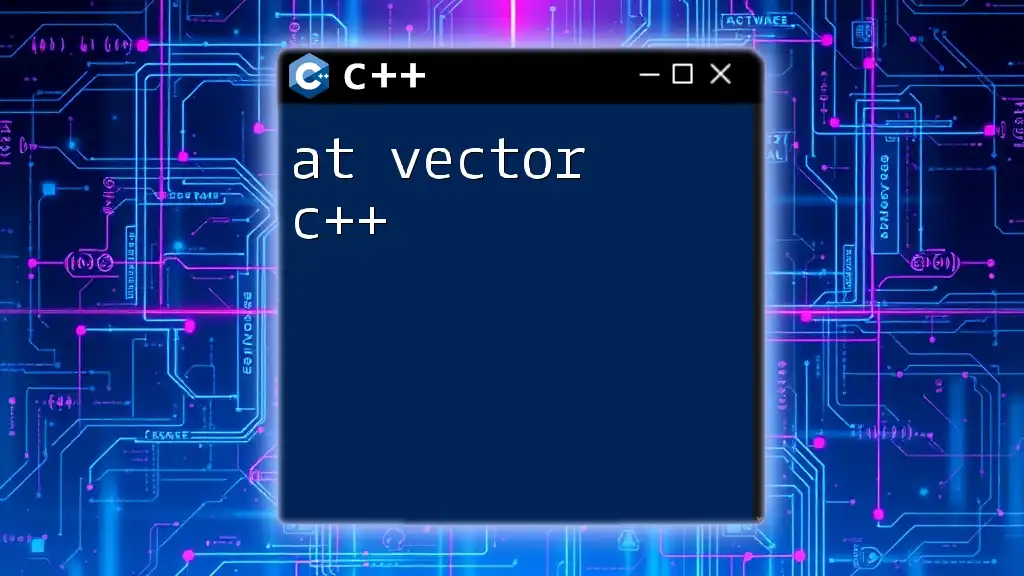
Declaring and Initializing 3D Vectors in C++
Using Standard Library Types
In C++, you can represent a 3D vector using standard library types like `std::array` or `std::vector`.
Using `std::array`, you can declare a 3D vector as follows:
std::array<float, 3> vectorA = {3.0f, 4.0f, 5.0f};
This approach utilizes fixed-size arrays, making it straightforward but limiting if you need dynamic resizing.
Creating a Custom 3D Vector Class
Class Definition
You can create a custom class to encapsulate the properties and behavior of a 3D vector more effectively. A typical definition might look like this:
class Vector3 {
public:
float x, y, z;
Vector3(float x, float y, float z) : x(x), y(y), z(z) {}
};
This class has three public member variables (`x`, `y`, and `z`) initialized through a constructor. This encapsulation allows for easier management and manipulation of vector instances.
Constructor Overloading
You can also implement constructor overloading for flexibility. For example, you might want to create a zero vector or a vector from an array:
class Vector3 {
public:
float x, y, z;
Vector3() : x(0), y(0), z(0) {} // Default constructor
Vector3(float x, float y, float z) : x(x), y(y), z(z) {}
Vector3(const std::array<float, 3>& vec) : x(vec[0]), y(vec[1]), z(vec[2]) {}
};
This allows users to create vectors in various ways without compromising readability.
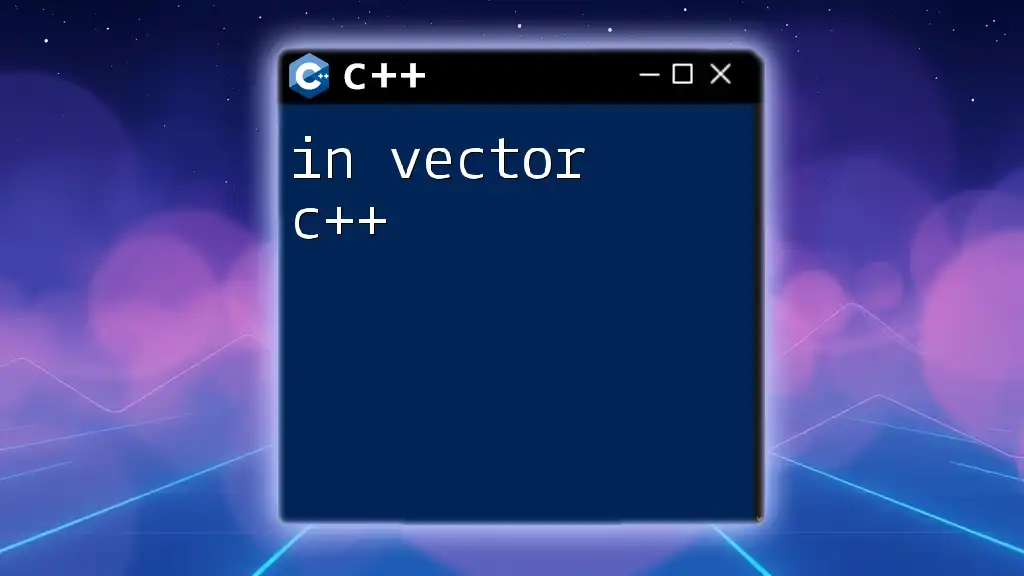
Basic Operations with 3D Vectors
Vector Addition
Vector addition is performed by adding the corresponding components of two vectors.
You can overload the `+` operator to facilitate this:
Vector3 operator+(const Vector3& v) {
return Vector3(x + v.x, y + v.y, z + v.z);
}
For instance, if you add `Vector A = (1, 2, 3)` and `Vector B = (4, 5, 6)`, the resulting vector will be `(5, 7, 9)`.
Vector Subtraction
Similarly, vector subtraction involves subtracting the components of one vector from another:
Vector3 operator-(const Vector3& v) {
return Vector3(x - v.x, y - v.y, z - v.z);
}
If we take `Vector A = (3, 5, 7)` and subtract `Vector B = (1, 2, 3)`, the result will yield `(2, 3, 4)`.
Scalar Multiplication
To scale a vector by a scalar value, you can overload the `*` operator:
Vector3 operator*(float scalar) {
return Vector3(x * scalar, y * scalar, z * scalar);
}
This means multiplying `Vector A = (2, 3, 4)` by `2` would provide a resultant vector of `(4, 6, 8)`.
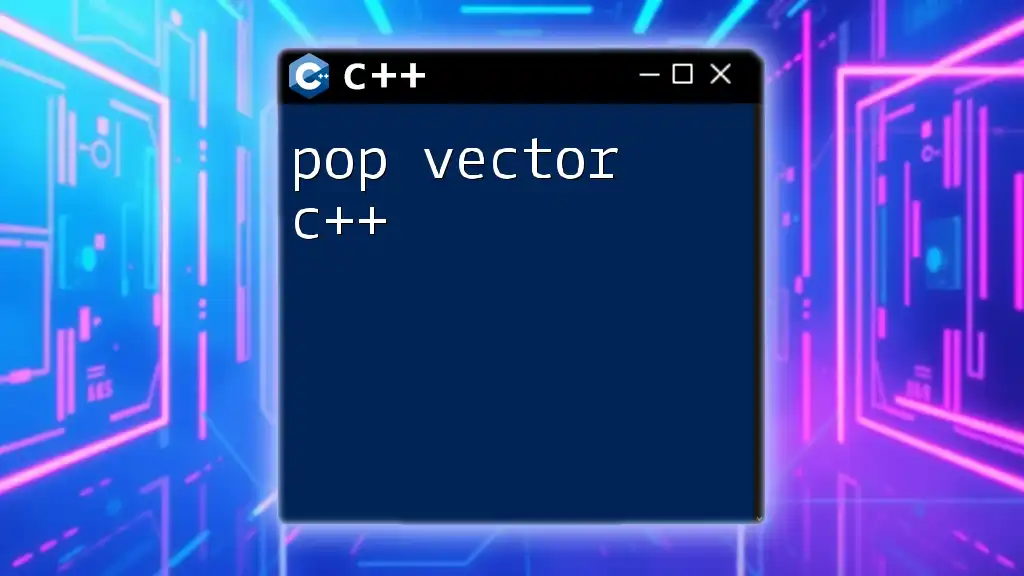
Advanced Operations
Dot Product
The dot product is a scalar value representing the magnitude of one vector in the direction of another and is calculated using the following formula:
float dot(const Vector3& v) {
return (x * v.x + y * v.y + z * v.z);
}
The dot product is useful in many calculations, such as determining the angle between two vectors or projecting one vector onto another.
Cross Product
The cross product results in a new vector that is orthogonal (perpendicular) to the two original vectors. This operation can be implemented as follows:
Vector3 cross(const Vector3& v) {
return Vector3(
y * v.z - z * v.y,
z * v.x - x * v.z,
x * v.y - y * v.x
);
}
For vectors `A = (1, 0, 0)` and `B = (0, 1, 0)`, the cross product produces `(0, 0, 1)`, demonstrating how it defines a new direction.
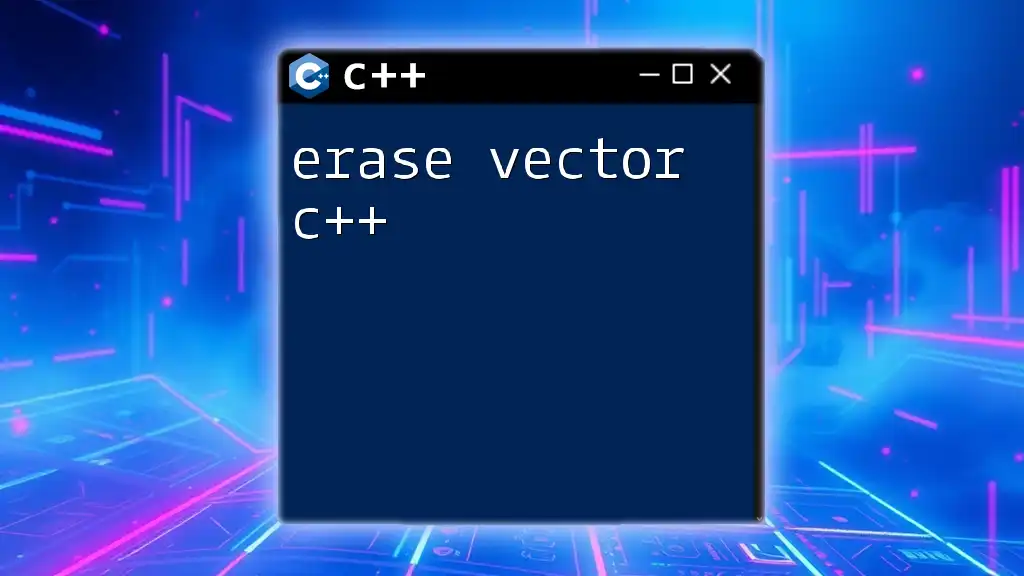
Normalization of 3D Vectors
Explanation of Normalization
Normalization is the process of transforming a vector into a unit vector, which has a magnitude of 1 but retains its direction. This is crucial in various applications, especially in 3D graphics where a unit direction vector is often needed.
Code Example for Normalizing a Vector
To normalize a vector, you can implement the following method:
Vector3 normalize() {
float length = sqrt(x * x + y * y + z * z);
return Vector3(x / length, y / length, z / length);
}
This function divides each component by the vector's length, resulting in a vector of length 1. For example, normalizing `(3, 4, 5)` produces approximately `(0.424, 0.566, 0.707)`.
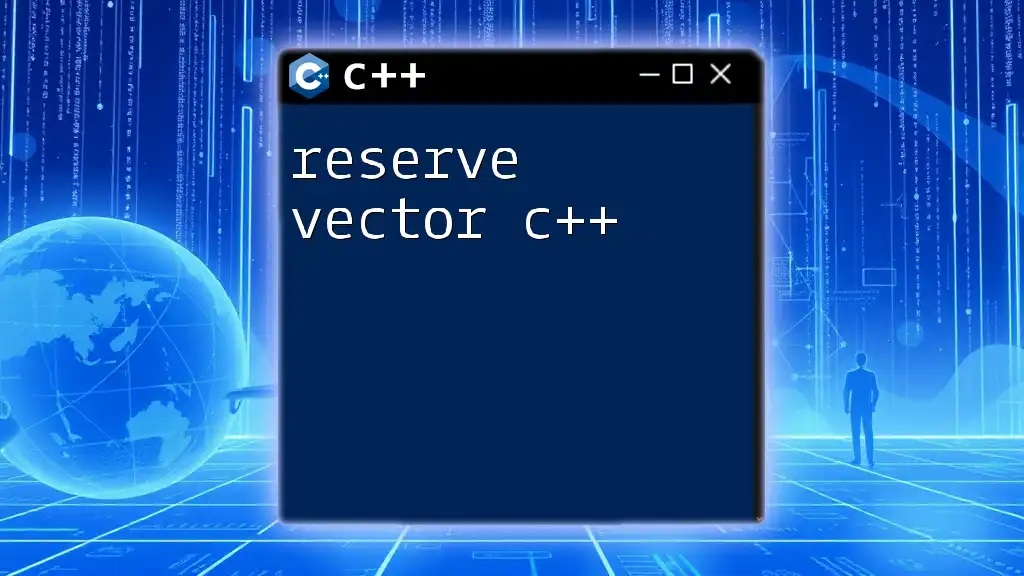
Applications of 3D Vectors
Computer Graphics
In computer graphics, 3D vectors form the backbone of geometric representation, allowing for the creation of images and animations. They are pivotal in determining positions, lighting, and various transformations such as translations and rotations.
Physics Simulations
In physics simulations, vectors describe force, velocity, and acceleration, providing a foundation for simulating real-world physics. The equations governing motion utilize vector mathematics to predict behaviors accurately.
Game Development
In game development, vectors are essential for character movement, camera control, and environmental interaction. Both 2D and 3D environments rely on vectors to implement realistic movements and interactions between characters and objects.
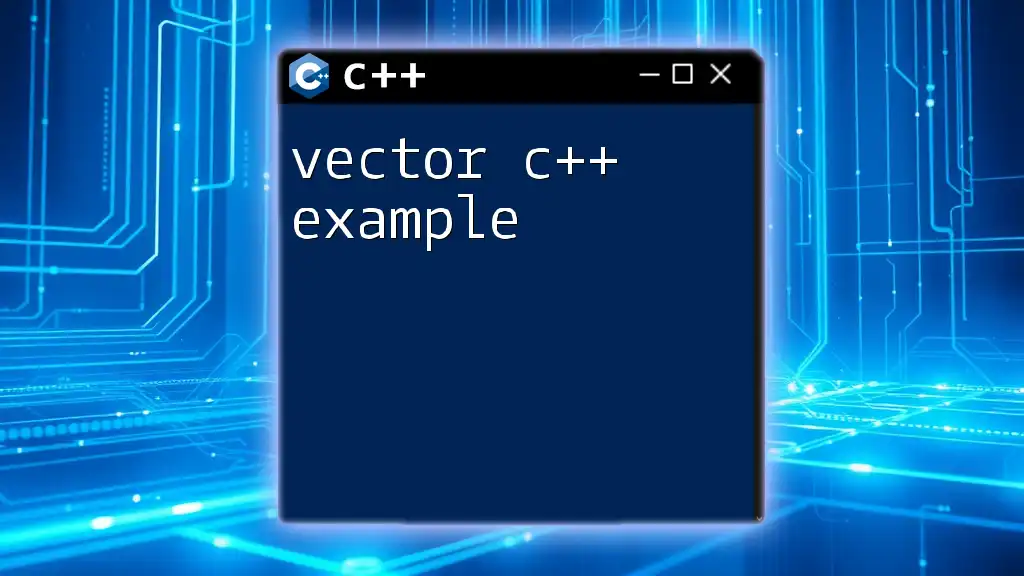
Conclusion
Understanding 3D vectors in C++ is vital for anyone interested in fields that involve mathematical computations, computer graphics, or game development. Whether through standard library types or custom classes, mastering vector operations not only deepens your programming skills but also expands your capabilities in creating sophisticated simulations and applications.
As you continue to learn, consider exploring advanced topics such as vector transformations, matrix representations, and more complex vector algebra to further enhance your programming toolbox.
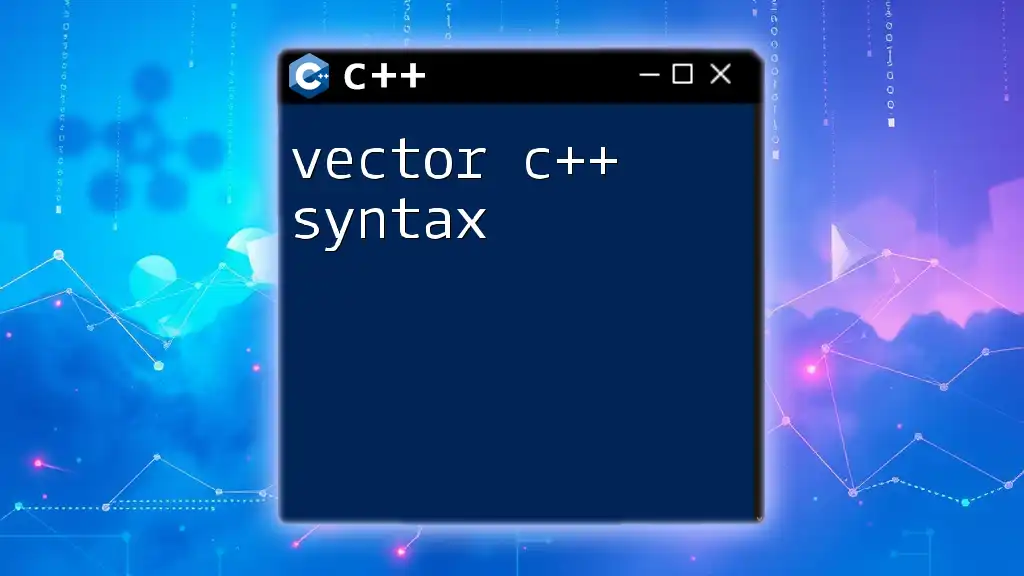
Further Reading
To reinforce your learning experience, explore recommended books, articles, and online courses focusing on elementary to advanced vector mathematics and C++ programming. Engaging with sample projects that utilize 3D vectors will also help solidify your understanding as you apply concepts in practical scenarios.