In C++, you can obtain the size of a vector (i.e., the number of elements it contains) using the `size()` member function. Here's a code snippet demonstrating this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::cout << "Size of vector: " << myVector.size() << std::endl; // Output: Size of vector: 5
return 0;
}
What is a Vector in C++?
In C++, a vector is a fundamental component of the Standard Template Library (STL). Unlike fixed-size arrays, vectors are dynamic arrays that can change in size during runtime, making them versatile and efficient for handling collections of data. Understanding the size of a vector in C++ is crucial for developers as it directly impacts memory management and performance.
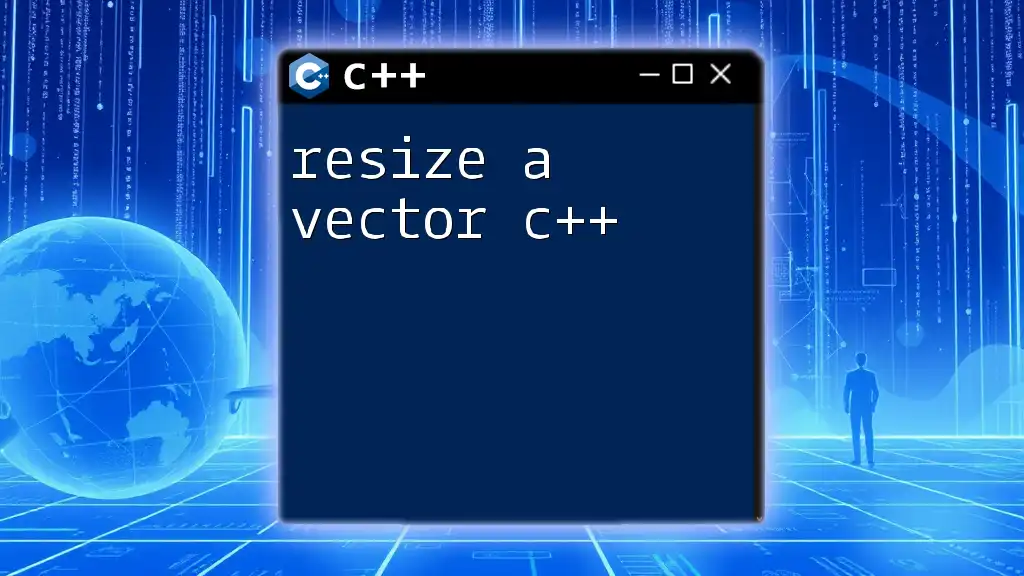
Understanding Vector Size
What Does 'Size' Mean for a Vector?
In the context of a vector, size refers to the number of elements it currently holds. This is different from capacity, which is the total amount of space allocated for the vector, regardless of how many items it contains. Knowing the difference between size and capacity is vital for efficient code and memory usage in C++.
Getting the Size of a Vector
Using the `size()` Method
To determine the number of elements in a vector, the `size()` method should be used. This method returns the current size of the vector, which is particularly useful for iterating through its elements or when you need to validate the number of items present.
Here’s a simple example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::cout << "Size of the vector: " << myVector.size() << std::endl;
return 0;
}
In this example, the output will be `Size of the vector: 5`, which indicates that the vector contains five elements.
Using the `max_size()` Method
The `max_size()` method is another way to gather information about the vector. This method returns the maximum number of elements that the vector can theoretically hold, which can be very useful when planning for large data sets.
Consider this snippet:
std::cout << "Maximum size of the vector: " << myVector.max_size() << std::endl;
While the actual maximum size may depend on system limitations, `max_size()` gives you a good idea concerning the theoretical bounds of the vector.
Capacity vs. Size: What’s the Difference?
Understanding Vector Capacity
Capacity refers to the amount of storage allocated for a vector, which may exceed its current size. When a vector reaches its capacity and a new element is added, it automatically increases its capacity, usually by a factor of two. Such behavior optimizes performance but can lead to increased memory usage.
Using the `capacity()` Method
To access the current capacity of a vector, the `capacity()` method is utilized. This method provides insights into how much space has been allocated for future additions to the vector without reallocating memory.
Example:
std::cout << "Capacity of the vector: " << myVector.capacity() << std::endl;
By knowing both the size and capacity, developers can make informed decisions about the efficiency of their data structures and avoid unnecessary reallocations.
Resizing a Vector
Using the `resize()` Method
Vectors allow developers to change their size dynamically at runtime using the `resize()` method. This is particularly useful when manipulating data sets where the number of elements may change.
For instance:
myVector.resize(10);
std::cout << "New size after resizing: " << myVector.size() << std::endl;
If the vector previously had a size of 5, resizing it to 10 will change its size accordingly while filling the new elements with default values (e.g., 0 for numeric types).
Impact of Resizing on Capacity
When resizing a vector, you might also want to consider how this affects capacity. If you increase the size beyond the vector’s current capacity, the vector will typically allocate more memory, which can involve copying existing elements to the new memory location. Understanding these dynamics can help you optimize your code.
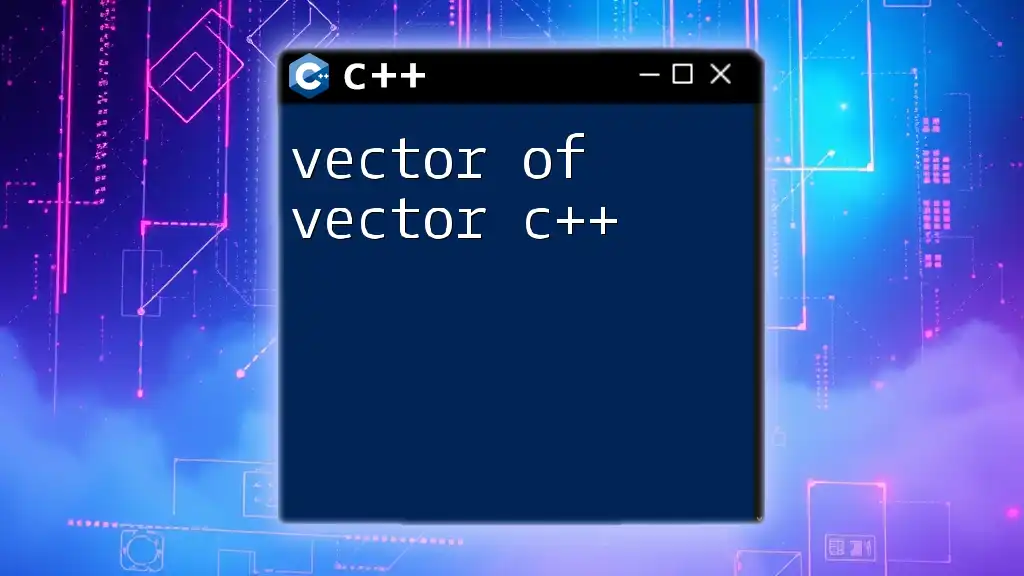
Performance Considerations
Efficiency of Getting Size
Obtaining the size of a vector using the `size()` method operates in constant time, O(1), meaning it’s very efficient. However, it is important to note that estimating its capacity may also have a significant role in performance, particularly for large data sets.
Memory Management in Vectors
C++ manages memory for vectors dynamically, but this adds a layer of complexity. When vectors resize, it might lead to fragmentation or inefficient memory usage. Therefore, if you know the expected number of elements, consider using `reserve()` beforehand to allocate enough capacity in advance. This minimizes the number of memory allocations required during runtime.
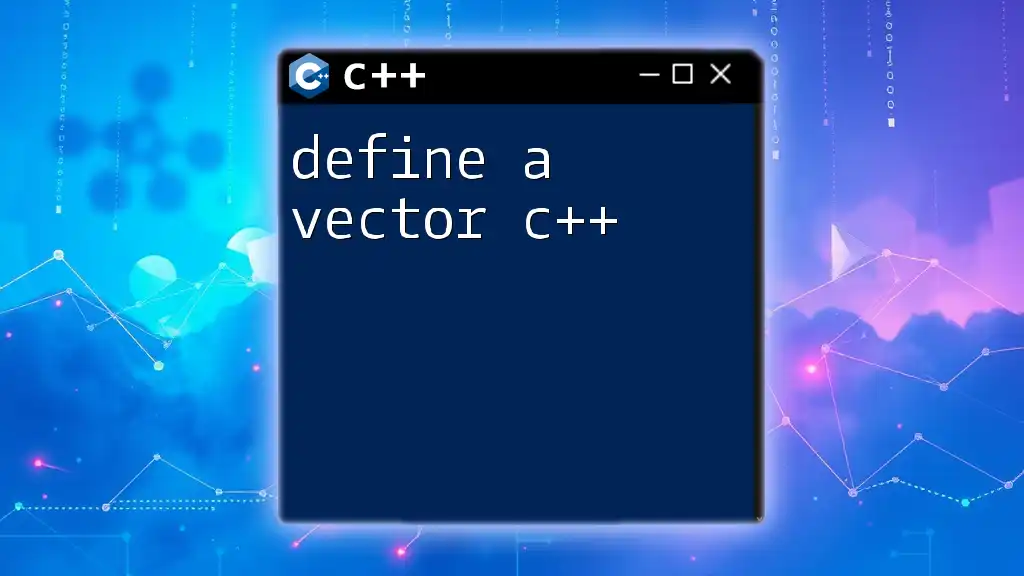
Common Use Cases
When to Use Vectors over Arrays
Vectors provide numerous benefits over traditional arrays. Their ability to resize and manage memory dynamically makes them ideal for situations where the size of the data collection is unknown at compile time. Use cases in scenarios such as dynamic data input or real-time data processing highlight the superiority of vectors.
Real-world Examples in Code
Consider creating a program that reads user input, where the total number of inputs isn’t predetermined:
#include <iostream>
#include <vector>
int main() {
std::vector<int> userInputs;
int input;
std::cout << "Enter numbers (0 to stop): ";
while (std::cin >> input && input != 0) {
userInputs.push_back(input);
}
std::cout << "You entered " << userInputs.size() << " numbers." << std::endl;
return 0;
}
In this example, using a vector allows you to collect user input without worrying about setting a maximum array size.
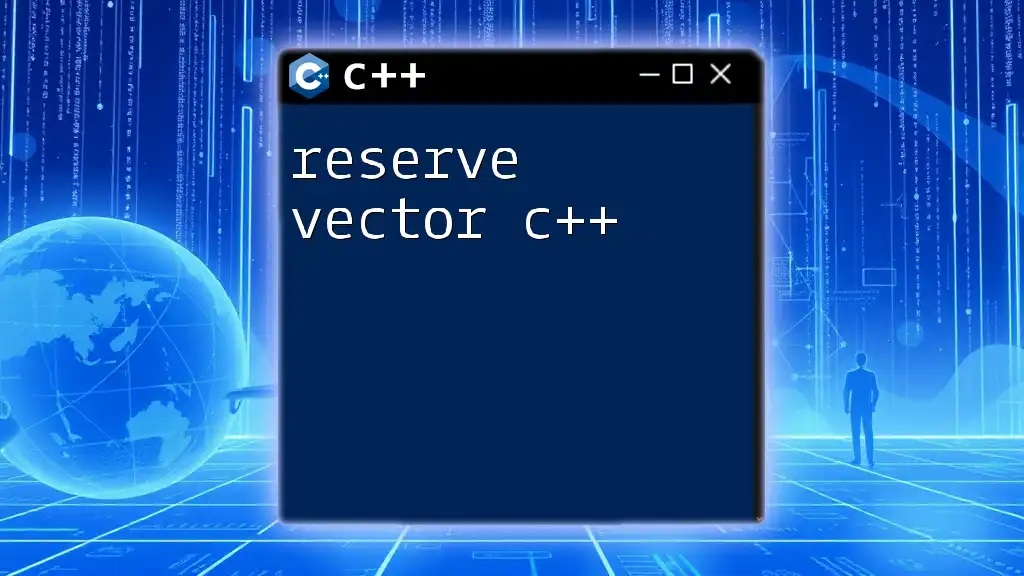
Conclusion
Understanding the size of a vector in C++ is crucial for effective programming. Knowing how to efficiently retrieve and manipulate the size helps in creating high-performing applications. By grasping the difference between size and capacity, resizing techniques, and best practices for memory management, developers can harness the full potential of vectors.
Remember to explore further into vector operations and their implications on performance to master this powerful data structure in C++. Whether you're working on small applications or substantial software projects, the knowledge of vector sizes will undeniably enhance your coding efficiency.
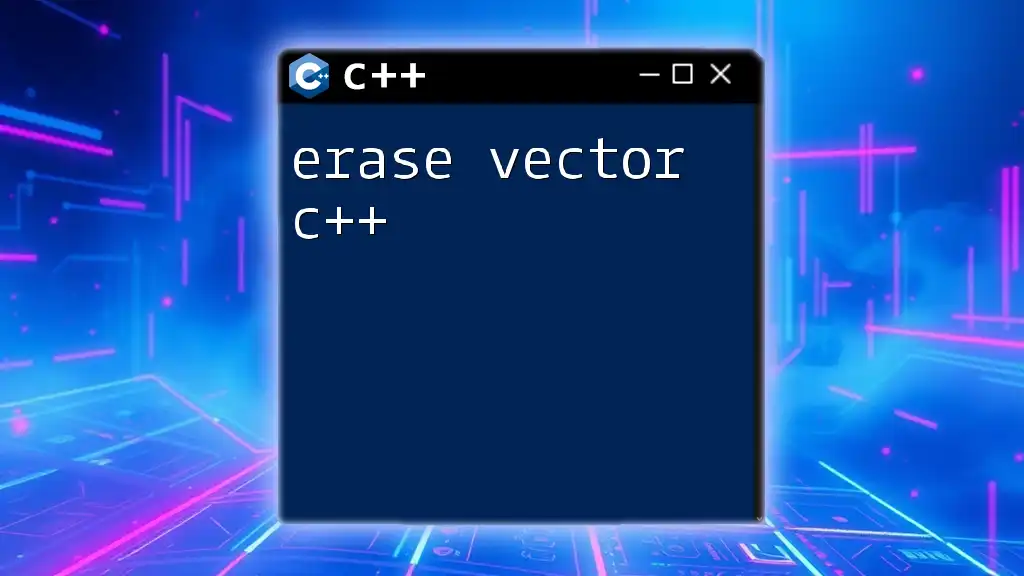
Additional Resources
To deepen your understanding, consider exploring more resources on C++ vectors and the STL. Engaging with online communities and forums can also provide valuable insights and support as you continue your coding journey. Feel free to share your thoughts or questions, as community feedback is always welcome!