In C++, the size of a `float` is typically 4 bytes, though it can vary depending on the system and compiler, and you can determine it using the `sizeof` operator as shown in the code snippet below:
#include <iostream>
int main() {
std::cout << "Size of float: " << sizeof(float) << " bytes" << std::endl;
return 0;
}
Understanding Floating-Point Numbers
What is a Float?
In C++, a float is a data type used to represent numbers with fractional parts. The float data type stores single-precision floating-point numbers, which means it can represent a wide range of values, albeit with limited precision compared to other floating-point types like double and long double. Floats are commonly used in scientific calculations where decimal precision is not critical but a large range of numbers is required.
Characteristics of Floating-Point Representation
Floating-point representation is characterized by precision and range. The precision indicates how many significant digits a float can accurately store, while the range refers to the smallest and largest values it can represent.
-
Precision: A float typically provides about 7 decimal digits of precision, which means that if your calculations require more precision, you may need to use a double (which offers about 15 decimal digits).
-
Range: The range determines how large or small a float can be. A float can typically represent values from approximately 1.5 × 10^−45 to 3.4 × 10^38. Understanding this range is crucial for choosing the appropriate data type in your applications.

The Size of Float in C++
Standard Size of a Float
To determine the size of a float in C++, the `sizeof` operator is used. This operator returns the number of bytes allocated for the specified data type.
In most modern computing environments, a float is typically 4 bytes. To dynamically determine the size of a float, you can use the following code:
#include <iostream>
int main() {
std::cout << "Size of float: " << sizeof(float) << " bytes" << std::endl;
return 0;
}
This code snippet will output: `Size of float: 4 bytes`, confirming the common size of a float across most platforms.
Factors Affecting Size
Although a float is generally 4 bytes, several factors can affect its size:
-
Compiler and Architecture: Different compilers and hardware architectures might impose specific size requirements. For instance, some embedded systems may use custom configurations that alter default sizes.
-
Comparison of Sizes Between Types: It's essential to understand how the float compares to other data types. Typically, a double occupies 8 bytes, and a long double can occupy more than 8 bytes, depending on the compiler and architecture in use.
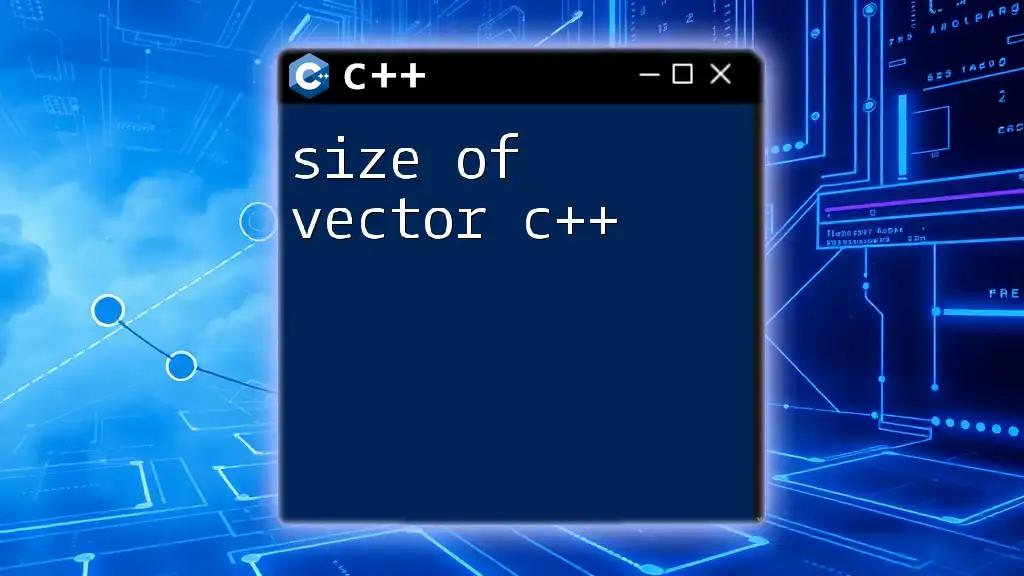
Different Data Types in Relation to Float Size
Comparisons: Float vs Double vs Long Double
To appreciate the impact of float size, it’s essential to compare it with double and long double types.
- Float: 4 bytes, ~7 decimal digits of precision
- Double: 8 bytes, ~15 decimal digits of precision
- Long Double: Sizes can vary, but often 10, 12, or even 16 bytes, providing at least 15 or more digits of precision
By evaluating the size and precision required for your application, you can choose the most suitable data type. Consider the example code below, which prints the sizes of various floating-point types:
#include <iostream>
int main() {
std::cout << "Size of float: " << sizeof(float) << " bytes" << std::endl;
std::cout << "Size of double: " << sizeof(double) << " bytes" << std::endl;
std::cout << "Size of long double: " << sizeof(long double) << " bytes" << std::endl;
return 0;
}
Why Choosing the Right Type Matters
Selecting the appropriate type is crucial for performance and precision, especially in applications that handle vast amounts of data or require mathematical calculations that depend on significant accuracy. Misunderstanding the size of float compared to other types can lead to unexpected behavior and inaccuracies in your program.

Ensuring Portability with Float Sizes
Importance of Standardized Sizes
C++ standards, such as C++98 and C++11, guarantee certain properties concerning data types, promoting consistent behavior across compliant platforms. Recognizing the expectations set by these standards helps developers write more reliable and maintainable code.
Using Fixed-Size Types
For increased portability, consider using fixed-size types as defined in the `<cstdint>` header. Although standard float types offer flexibility, fixed-size types can ensure consistent behavior across different environments. Utilize the fixed-size types when available for enhanced compatibility, as shown in the following code snippet:
#include <iostream>
#include <cstdint>
int main() {
std::cout << "Size of float: " << sizeof(float) << " bytes (using cstdint)" << std::endl;
return 0;
}

Practical Considerations When Using Float
Precision and Accuracy
The precision limits of a float can lead to unexpected results, especially in calculations that depend on accuracy. For instance, a float may not accurately represent certain decimal values. To test and visualize this behavior, consider the example below, which evaluates the precision of floating-point representation:
#include <iostream>
#include <iomanip>
int main() {
float number = 1.0f / 3.0f;
std::cout << std::fixed << std::setprecision(10) << number << std::endl; // Testing precision
return 0;
}
Common Mistakes with Float
Developers often make critical mistakes when using float types, especially in financial applications where precision is paramount. One common pitfall is assuming the equality of two floating-point numbers. Below is an example that illustrates this problem:
#include <iostream>
int main() {
float a = 0.1f + 0.2f; // Expecting this to equal 0.3
float b = 0.3f; // Theoretically equal
if (a == b) {
std::cout << "They are equal" << std::endl;
} else {
std::cout << "They are NOT equal due to precision issues" << std::endl;
}
return 0;
}
Due to the imprecise nature of floating-point arithmetic, the output will likely reveal that `a` and `b` are not equal, leading to unexpected results.
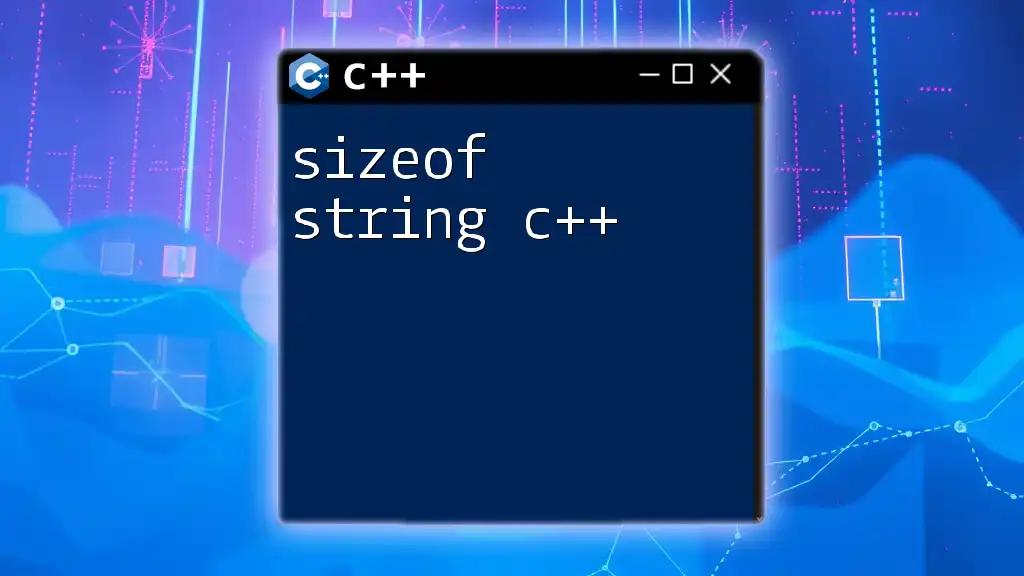
Conclusion
A solid understanding of the size of float in C++ is essential for developers aiming to write efficient and accurate applications. By recognizing the implications of using floats, as well as the nuances of float sizes and related types, programmers can make informed decisions that enhance the reliability and effectiveness of their code. Experimentation and familiarity with these concepts will empower developers to manipulate floating-point arithmetic with confidence in their respective pursuits.

Additional Resources
For further exploration of floating-point types and their implications, refer to the official C++ documentation and consider engaging with community discussions on platforms like Stack Overflow or C++ development forums for shared insights and best practices.