The size of an `int` in C++ is typically 4 bytes on most platforms, but it can vary based on the system architecture; here’s how you can check it using `sizeof`:
#include <iostream>
int main() {
std::cout << "Size of int: " << sizeof(int) << " bytes" << std::endl;
return 0;
}
Understanding the Fundamentals of C++
What is C++?
C++ is a powerful programming language that combines high-level functionality with low-level memory manipulation. Naturally, it is widely used in various domains like system software, game development, and application software. Its flexibility and performance-oriented design make it an essential language for many programmers.
Why Data Types Matter?
Data types in programming define the nature of data that can be stored and manipulated. They are crucial because:
- Memory management: Different data types occupy different amounts of memory. Knowing how much memory various types consume can help optimize performance and resource usage.
- Type safety: Using the correct data type reduces the risk of bugs in the code, which could arise from unintended data operations.
Among these data types, `int` is one of the most fundamental types, representing integer values used extensively in calculations, counters, and more.
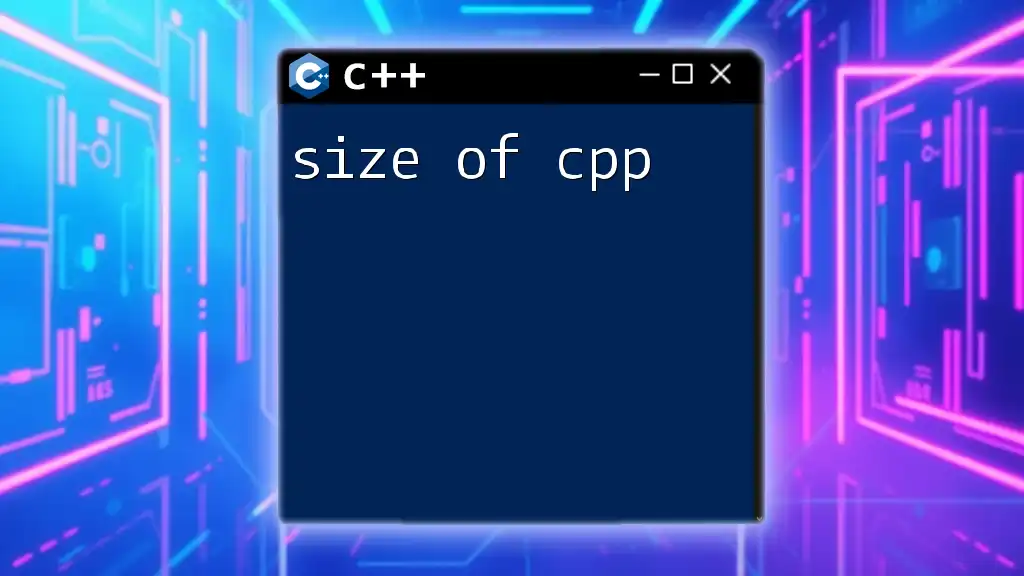
The Size of Int in C++
What is the Size of Int in C++?
The "size of int" in C++ refers to the amount of memory allocated for integer variables. Understanding this size is important for efficient memory utilization and preventing overflow errors. Knowing the size helps programmers make informed choices when handling numerical data.
Variability of Int Size
One of the unique aspects of the size of int in C++ is its variability. The C++ Standard specifies that an `int` must have a minimum size of 16 bits (2 bytes), but its actual size depends on the underlying hardware and compiler.
- 32-bit systems typically allocate 4 bytes (or 32 bits) for an `int`.
- 64-bit systems also commonly use 4 bytes, but it's not a strict rule.
This variability of size raises critical considerations when writing portable code.
Typical Size of Int in C++
The typical expectation for the size of `int` in C++ is 4 bytes on most modern platforms, though this can vary based on system architecture and the compiler used.
Using the `sizeof` operator, developers can dynamically check the size of an `int` in their environment. The following code snippet demonstrates this:
#include <iostream>
int main() {
std::cout << "Size of int: " << sizeof(int) << " bytes" << std::endl;
return 0;
}
Running this code will yield the size of `int` based on your system, allowing you to confirm the expectations programmatically.
Importance of Int Size in Applications
Knowing the size of `int` carries significant weight in various applications. For instance, in environments with limited memory resources, such as embedded systems, using larger data types than necessary can lead to inefficiencies.
Moreover, it plays a crucial role in algorithms. For example, if an algorithm relies on counting iterations or storing values within a specific range, knowing that an `int` has a limited size can prevent overflow issues. A poorly chosen data type could lead to unexpected results, program crashes, or memory inefficiencies.
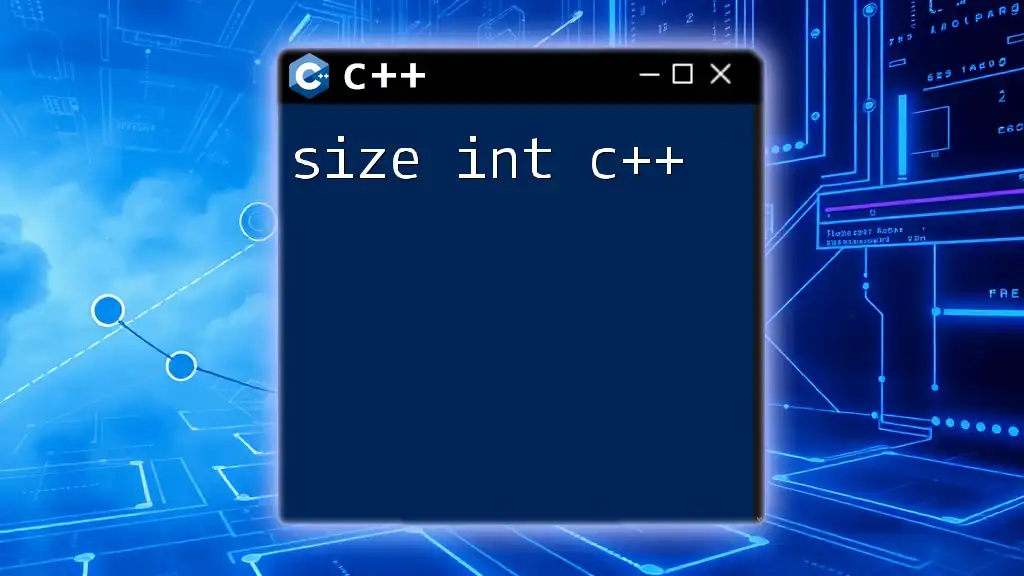
Factors Affecting Size of Int
Compiler and Environment Influence
Different compilers (like GCC, MSVC, or Clang) may implement the C++ standard in slightly varying ways, leading to differences in size.
- Compiler flags and settings can influence the definition of `int`. For instance, using specific flags during compilation may enforce different data sizes to optimize for speed or memory.
Being aware of these differences is critical for writing portable code across different systems and maintaining consistency in your application.
Data-Type Modifiers
C++ offers several data type modifiers that can alter the size of integer types. These include `short`, `long`, and `unsigned`, among others. Here’s a brief overview:
- short: Typically 2 bytes (16 bits)
- long: Usually 4 bytes on 32-bit systems and 8 bytes on 64-bit systems
- unsigned int: Can have the same size as `int` but represents only non-negative values
The following code snippet showcases various integer types and their sizes:
#include <iostream>
int main() {
std::cout << "Size of short: " << sizeof(short) << " bytes" << std::endl;
std::cout << "Size of long: " << sizeof(long) << " bytes" << std::endl;
std::cout << "Size of unsigned int: " << sizeof(unsigned int) << " bytes" << std::endl;
return 0;
}
This snippet helps illustrate how different modifiers can affect the size and behavior of integer variables.
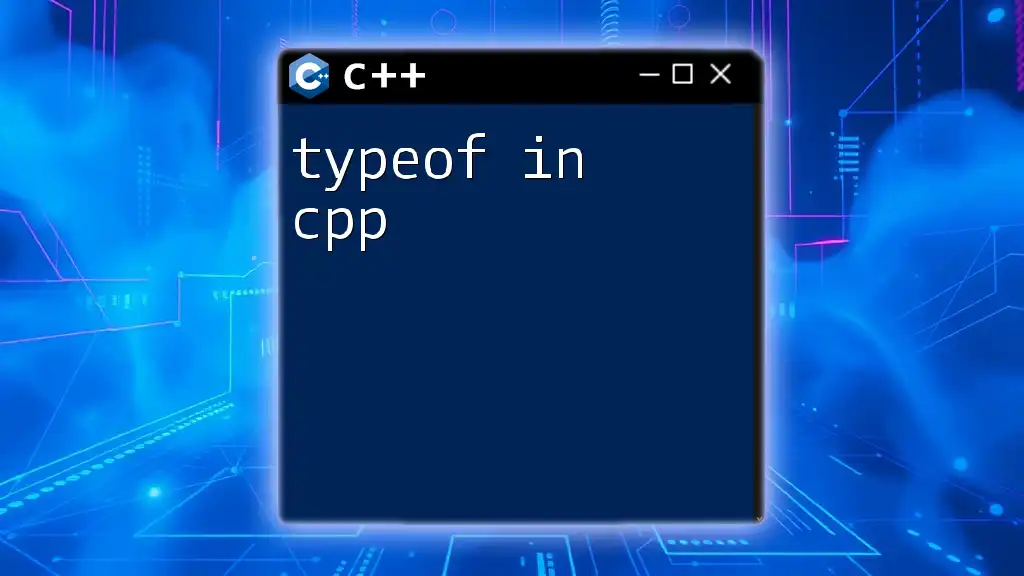
Best Practices with Int Size
Choosing the Right Data Type
When writing C++ code, it is essential to choose the right data type based on the application. Here are some guidelines:
- Use int for general-purpose integer needs.
- Use short for memory-constrained applications and when values are always within the range of 16 bits.
- Use long for larger values and when specific requirements dictate its usage.
- Use unsigned variants when you know the values will not be negative.
Choosing the most appropriate data type can lead to better memory efficiency and program performance.
Checking Int Size in Code
To ensure your code remains portable and predictable across different environments, always check the size of your `int` using the `sizeof` operator. This practice can help you avoid discrepancies in expected and actual behavior. Below is an example of a function that checks the size of `int`:
#include <iostream>
void checkIntSize() {
std::cout << "The size of int is: " << sizeof(int) << " bytes" << std::endl;
}
int main() {
checkIntSize();
return 0;
}
As demonstrated, this function provides insight into your specific implementation, reinforcing the importance of understanding the implications of the size of `int cpp`.
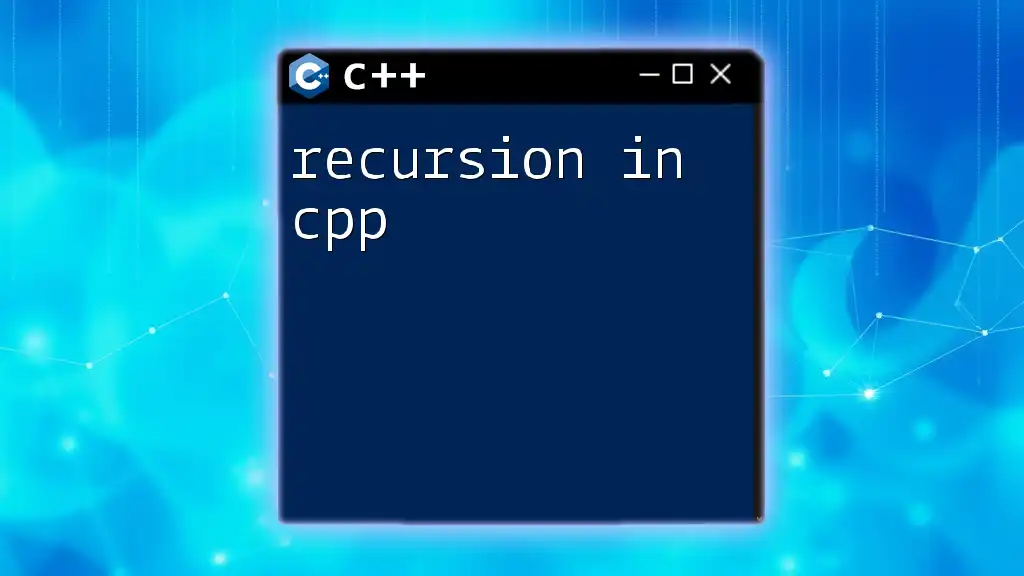
Conclusion
Recap of Key Points
In summary, understanding the size of int in C++ is vital for effective programming and memory management. The variability in size across systems means that you must be cautious when selecting data types. Remember that the choice between various integer types can significantly impact your application's performance and stability.
Call to Action
As you continue to work with C++, take the time to experiment with different data types and their sizes. By doing so, you’ll not only enhance your understanding of how to efficiently manage memory but also solidify your foundation in good programming practices. Consider subscribing for more quick and concise C++ programming tips to further sharpen your skills!