TutorialsPoint CPP is an online platform that provides comprehensive and beginner-friendly resources for learning C++ programming through concise examples and clear explanations.
Here's a simple code snippet to demonstrate a basic "Hello, World!" program in C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is Tutorialspoint?
Overview of the Platform
Tutorialspoint is an extensive online learning platform that offers tutorials on various programming languages, including C++. It provides a structured approach to learning, with topics arranged logically from basic to advanced levels. Each tutorial consists of explanations, examples, and code snippets that help reinforce learning through practice.
Why Choose Tutorialspoint for C++ Learning?
Choosing Tutorialspoint for learning C++ is advantageous due to its user-friendly interface. The layout is intuitive, making it easy to navigate between different tutorials and subjects. Additionally, it offers extensive resources which are crucial for beginners and seasoned programmers alike. The platform includes quizzes, coding exercises, and community forums, fostering a collaborative environment where learners can engage and seek help.
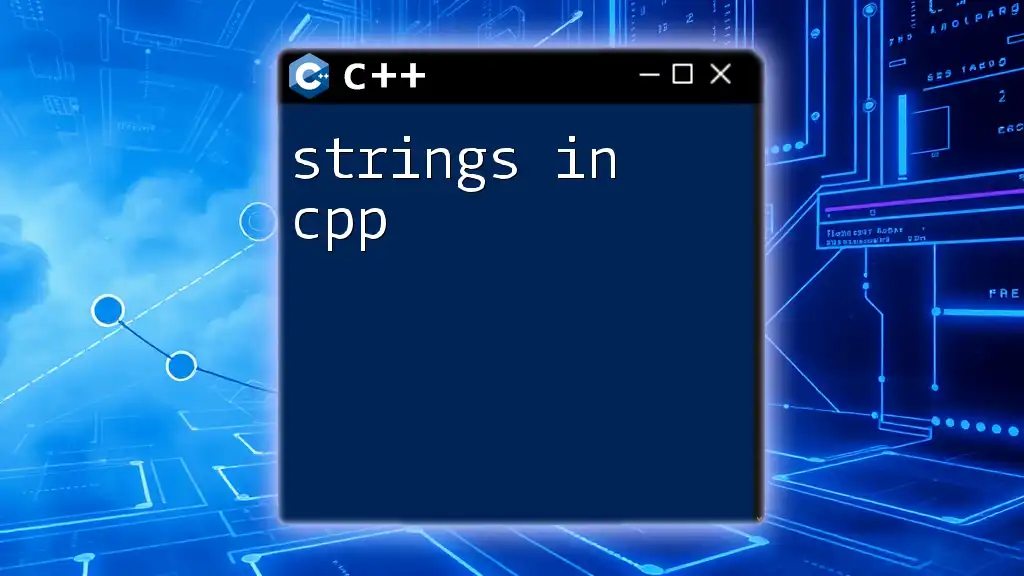
Getting Started with C++ on Tutorialspoint
Creating an Account
To access additional features like saving progress and participating in forums, creating an account on Tutorialspoint is recommended. The process is simple and requires only basic information such as your email and a password. Once registered, you can benefit from personalized learning experiences and track your progress over time.
Navigation of the C++ Tutorial Section
The C++ tutorial section on Tutorialspoint is well-organized, comprising various key components. These include:
- Basics of C++: syntax, data types, operators
- Control structures: decision-making and loops
- Functions and scope: modular programming
- Object-Oriented Programming: classes and objects
- Advanced topics: templates and STL (Standard Template Library)
As you navigate through these sections, each tutorial contains practical examples, making it easier to grasp complex concepts.
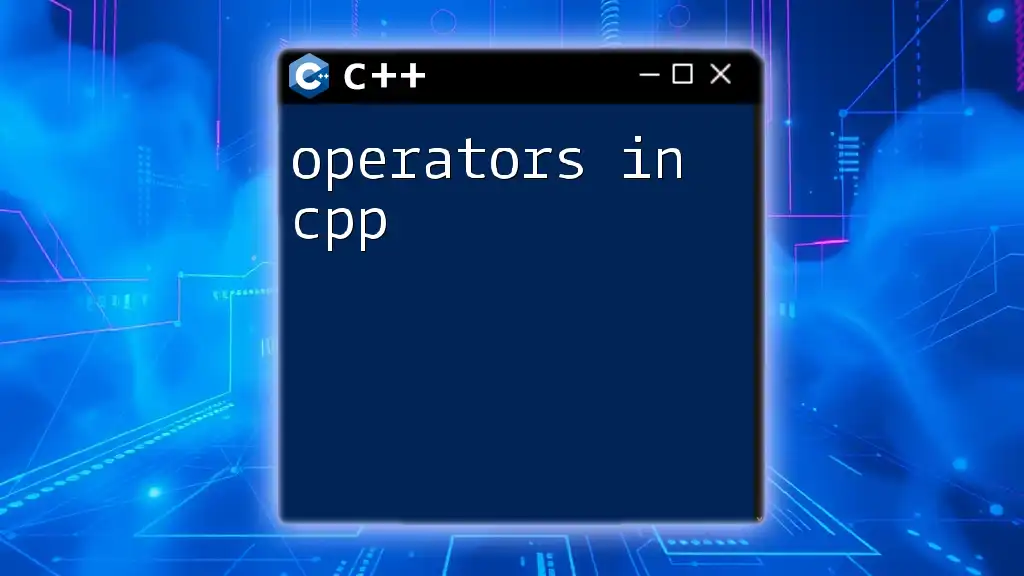
Understanding C++ Commands
What are C++ Commands?
C++ commands are the building blocks of programs written in C++. They dictate the behavior of the program, allowing you to perform operations, make decisions, and manage data. Learning C++ commands is essential to becoming proficient in programming.
Basic C++ Commands on Tutorialspoint
The foundational commands in C++ play a pivotal role in interacting with users. One of the primary components is iostream, which facilitates input and output operations.
For instance, the commands `cout` (for output) and `cin` (for input) are essential for basic programming tasks. Here’s a simple example demonstrating their usage:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Outputs Hello, World!
return 0;
}
In this code:
- `#include <iostream>` imports the input-output stream library.
- `using namespace std;` allows the use of standard functions without needing to prefix them with `std::`.
- The `cout` command displays text to the user.
This basic structure serves as the starting point for many C++ programs.
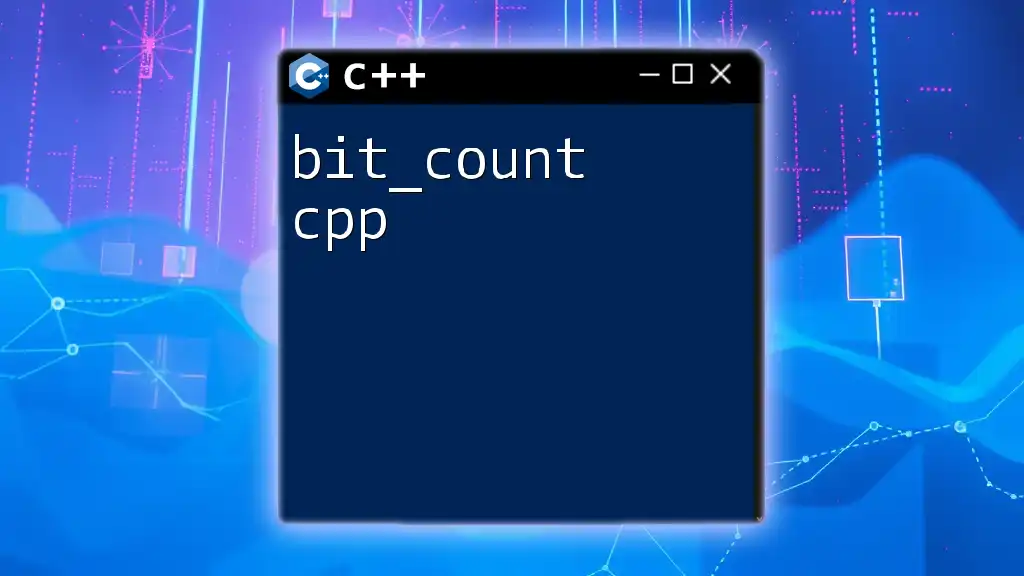
Intermediate C++ Commands
Control Structures
Control structures are vital for directing the flow of the program based on conditions. The two most common types include:
- Conditional Statements: These allow your program to make decisions. An example is the `if` statement:
int x = 10, y = 20;
if (x > y) {
cout << "X is greater than Y";
} else {
cout << "Y is greater than or equal to X"; // This will execute
}
- Loops: Repeating a block of code is made possible through loops (e.g., `for` and `while` loops). Here's how a `for` loop works:
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i << endl; // Outputs Iteration 0 to 4
}
These control structures are crucial for developing dynamic and responsive programs.
Functions in C++
Functions are essential for modular programming, allowing code reuse and better organization. To define a function, you specify the return type, name, and parameters. For instance:
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
cout << add(5, 3); // Outputs 8
By structuring code into functions, you enhance clarity and simplify maintenance.
Arrays and Pointers
Arrays store multiple values of the same type, making them efficient for managing collections of data. Here’s how you can declare and use an array:
int arr[5] = {1, 2, 3, 4, 5};
cout << arr[0]; // Outputs 1
Pointers, on the other hand, hold memory addresses of other variables, allowing for dynamic memory management and more efficient array handling. For example:
int var = 20;
int* ptr = &var; // ptr now holds the address of var
cout << *ptr; // Outputs 20
This concept is foundational for advanced programming techniques.
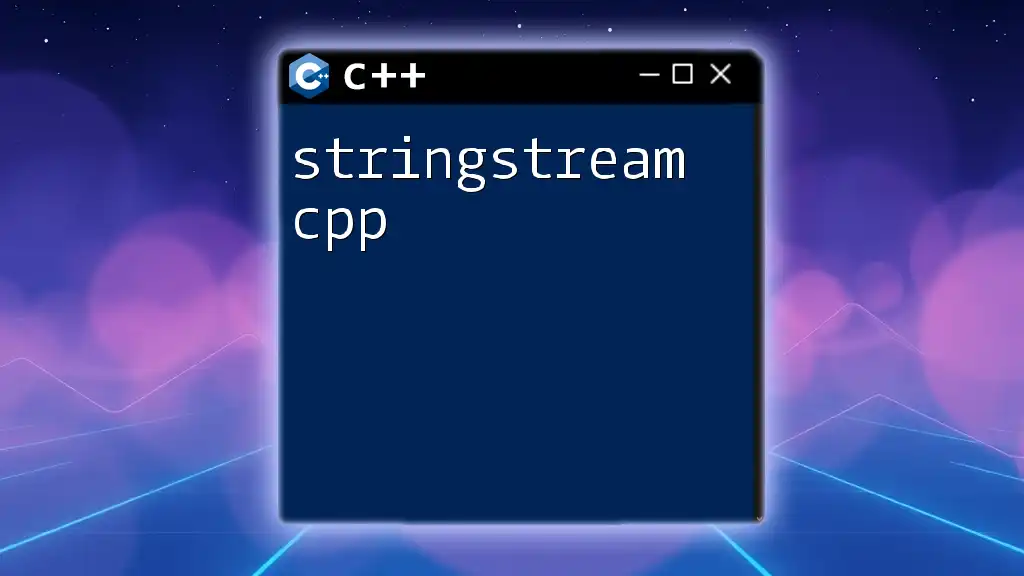
Advanced C++ Commands
Object-Oriented Programming (OOP) Concepts
C++ embraces Object-Oriented Programming principles, which facilitate better code management and reusability. The key principles are:
- Encapsulation: Bundling data and methods that operate on that data within one unit (class).
- Inheritance: Creating a new class derived from an existing class to promote code reuse.
- Polymorphism: Allowing methods to do different things based on the object that it is acting upon.
Here’s an illustrative example of OOP in C++:
class Animal {
public:
virtual void sound() {
cout << "Some sound";
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark"; // Overrides the base class method
}
};
This encapsulation of behavior enhances the program's structure and functionality.
Templates and STL (Standard Template Library)
Templates in C++ allow programmers to create generic functions and classes. This promotes reusability without compromising type safety. Here’s a simple example with a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
cout << add<int>(2, 3); // Outputs 5
The Standard Template Library (STL) is an invaluable resource, providing a collection of template classes that implement data structures and algorithms. For instance, you can efficiently manage collections with vectors:
#include <vector>
vector<int> vec;
vec.push_back(10);
cout << vec[0]; // Outputs 10
STL significantly enhances productivity by reducing the amount of code developers have to write.
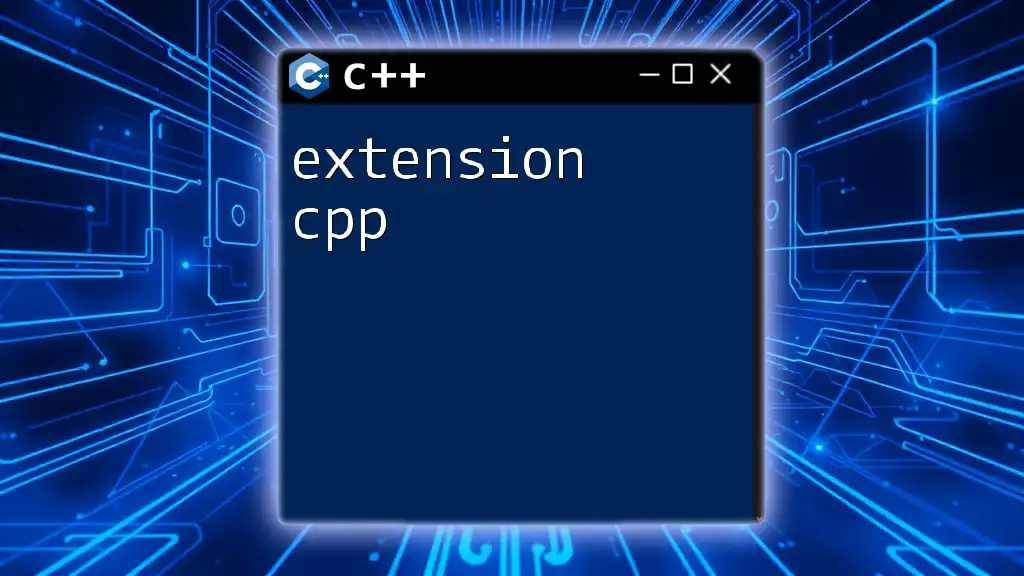
Utilizing Tutorialspoint for Practice
Online Compiler Feature
The online compiler feature on Tutorialspoint is an excellent tool for immediate hands-on practice. You can write, compile, and run C++ code directly in your browser, which is particularly useful for experimenting with new concepts and commands without needing a local setup.
Recommended Exercises and Quizzes
To solidify your understanding, Tutorialspoint offers a range of exercises and quizzes. These resources are crucial for reinforcing the concepts learned and assessing your grasp of C++ commands. Regular practice through these exercises will increase your confidence and proficiency.
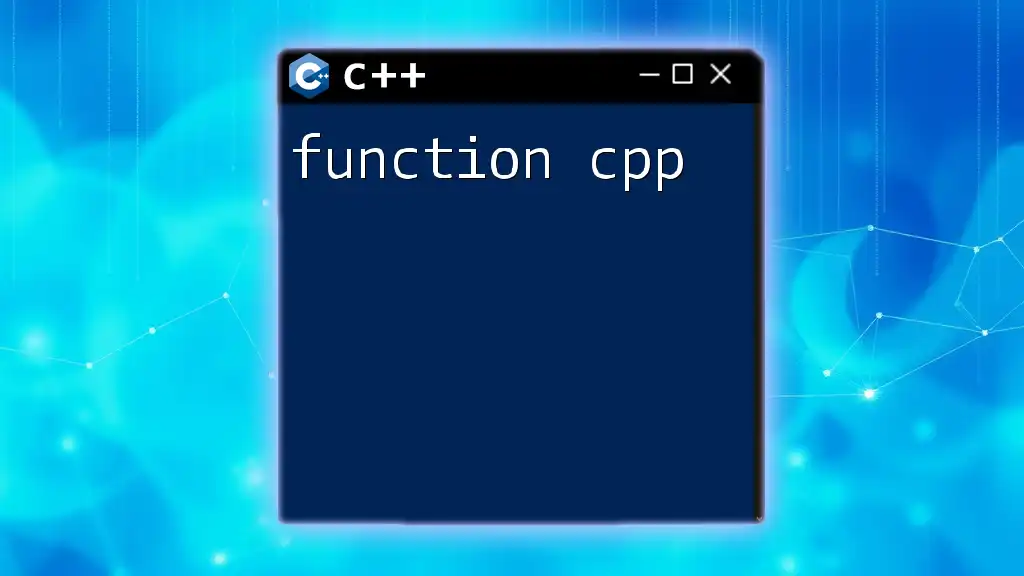
Community and Support
Engaging with the Tutorialspoint Community
Interacting with the Tutorialspoint community can provide additional support. The forums are a good place to ask questions, share insights, and learn from experienced developers. Engaging with others can also expose you to new ways of thinking about C++ programming.
Finding Additional Resources
While Tutorialspoint serves as a fantastic starting point, exploring other platforms can enrich your learning experience further. Consider supplementing your studies with recommended textbooks, online courses, and coding challenges from sites like LeetCode or HackerRank.
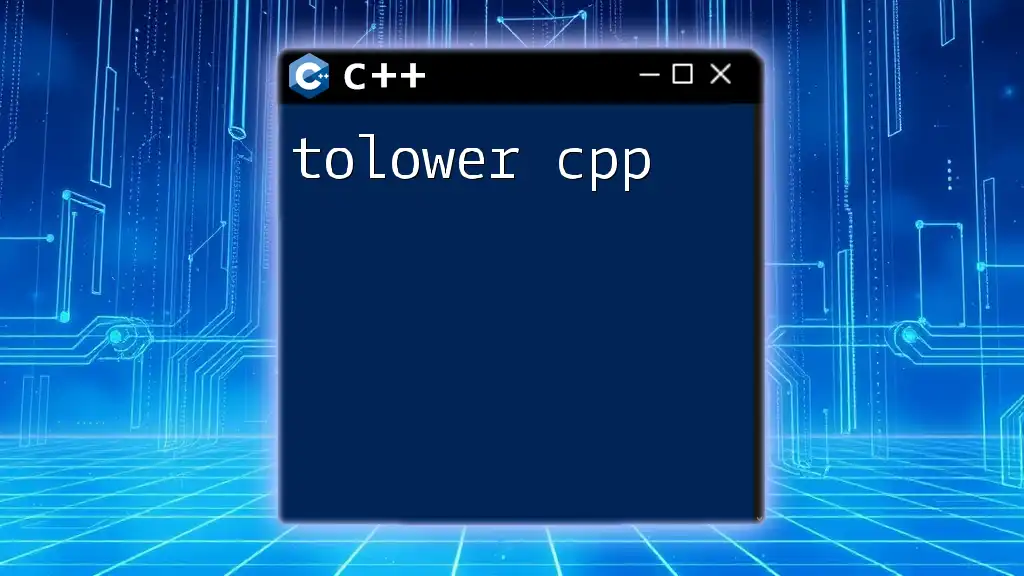
Conclusion
Utilizing Tutorialspoint for learning C++ commands not only streamlines the process but also equips you with practical skills essential for programming. By engaging with the tutorials, practicing regularly, and leveraging community support, you can master C++ quickly and effectively. Start your journey today and unlock the vast potential of this powerful programming language!