In C++, strings are used to handle sequences of characters, and you can utilize the standard library `std::string` class to perform various operations on these character sequences.
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl; // Output: Hello, World!
return 0;
}
What Are Strings in C++?
In C++, strings are used to represent sequences of characters. They are fundamental data types used to handle textual data effectively. Strings in C++ can be divided into two categories: C-style strings and C++ strings.
C-style strings, or null-terminated strings, are essentially arrays of characters that end with a special null character (`'\0'`). However, they can be cumbersome to work with, leading to potential errors such as buffer overflows. On the other hand, C++ strings are part of the Standard Template Library (STL) and are designed to manage memory automatically and provide a rich set of functions for string manipulation.
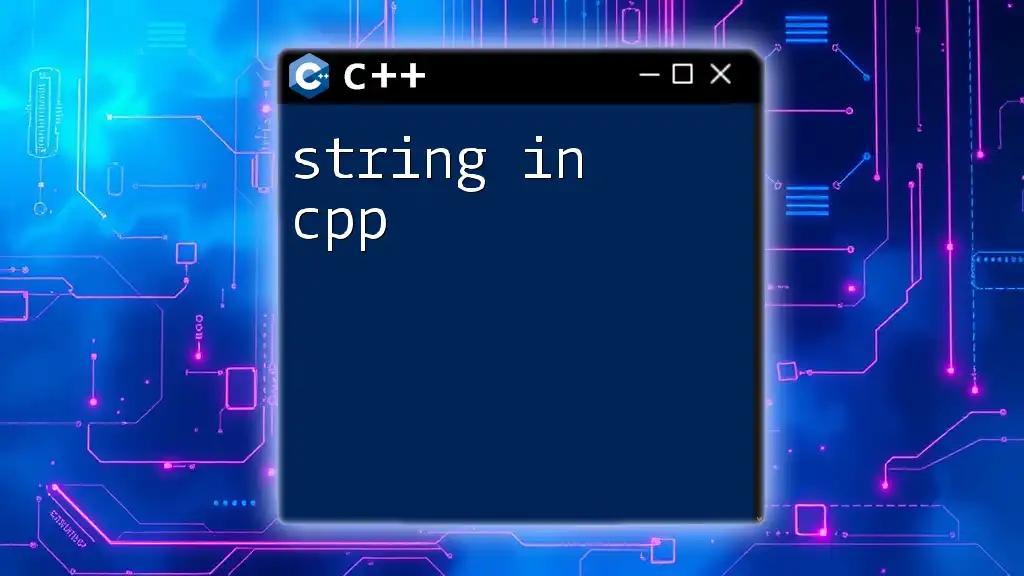
Define String in C++
When it comes to defining a string in C++, the `std::string` class is used. This gives programmers a flexible and powerful way to work with strings. Below is a simple example of how to create a string using `std::string`:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, C++ Strings!";
std::cout << myString << std::endl;
return 0;
}
In this code snippet, a string called `myString` is initialized with a character literal. This demonstrates just how easy it is to work with strings in C++.
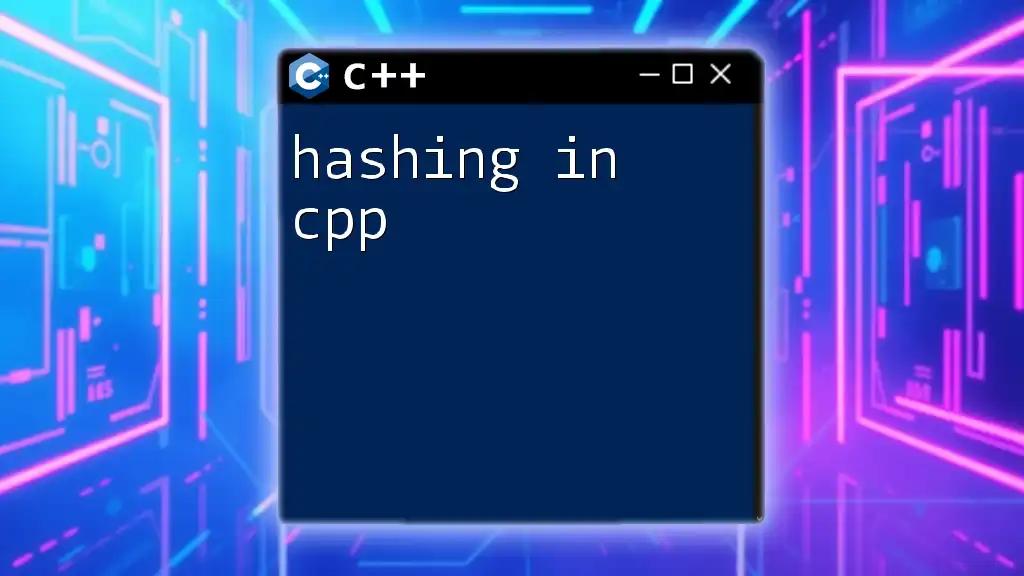
String Initialization and Assignment
Different Ways to Initialize a String
-
Using String Literals: You can create a string directly from a quoted text.
-
Using Other Strings: One string can be initialized using another string, allowing for easy manipulation and assignment.
Here's an example of string initialization and assignment:
std::string str1 = "Good Morning";
std::string str2(str1); // Copy initialization
In this example, `str1` is initialized using a string literal, while `str2` is a copied version of `str1`.
Retrieving String Properties
One of the strengths of using C++ strings is the ability to easily inspect their properties. You can use the `.length()` or `.size()` methods to get the number of characters in the string. Here’s how it looks:
std::cout << "Length of str1: " << str1.length() << std::endl;
Both methods return the same value, giving you a convenient way to check string sizes.

Basic Operations on C++ Strings
Concatenating Strings
Combining strings is a common operation. In C++, you can concatenate strings using the `+` operator, which makes your code clean and straightforward:
std::string greeting = "Hello, ";
std::string name = "Alice";
std::string fullGreeting = greeting + name;
Here, `fullGreeting` will hold the value "Hello, Alice" as a result of the concatenation.
Accessing Characters in a String
You can access individual characters in a string using the `[]` operator or the `.at()` method. The following code demonstrates how to access characters:
char firstChar = myString[0]; // Access first character
Utilizing the `.at()` method has the added benefit of performing bounds checking, which can help avoid accessing invalid memory.
Modifying Strings
Strings in C++ can be easily modified by changing individual characters:
myString[0] = 'h'; // Changes 'H' to 'h'
This line of code alters the original string, showcasing C++'s power and simplicity in managing string data.

Advanced String Functions
Finding Substrings
Searching for a substring within a string can be achieved using the `.find()` method. It returns the position of the first occurrence of the substring or `std::string::npos` if it is not found. Here's an example:
std::size_t found = myString.find("C++");
if (found != std::string::npos) {
std::cout << "Found 'C++' at: " << found << std::endl;
}
This code snippet checks for the substring "C++" and prints its location within the string.
String Comparison
Comparing strings is made easy with the built-in comparison operators such as `==`, `!=`, `<`, and others. Below is a simple comparison example:
if (str1 == str2) {
std::cout << "Strings are equal!" << std::endl;
}
This code checks if two strings are identical and prints a message accordingly.
String Substring and Erasing
The `.substr()` method allows you to extract parts of a string, while the `.erase()` method deletes characters. Here’s how they work:
std::string sub = myString.substr(0, 5); // "Hello"
myString.erase(0, 5); // Removes "Hello"
The first line extracts the first five characters, while the second line erases those characters from the original string.

Working with C-style Strings
What Is a C-style String?
C-style strings are simply arrays of characters that use the null character (`'\0'`) to indicate the end. They require manual memory management, which can lead to errors if not handled properly. For example, the following declaration shows a C-style string:
char cStr[100] = "Hello, C-style Strings!";
Converting Between C++ Strings and C-style Strings
When you need to interact with functions that expect C-style strings, you can convert a C++ string using the `.c_str()` method. For instance:
const char* cStr = myString.c_str();
This allows you to pass `myString` to C-style functions seamlessly.
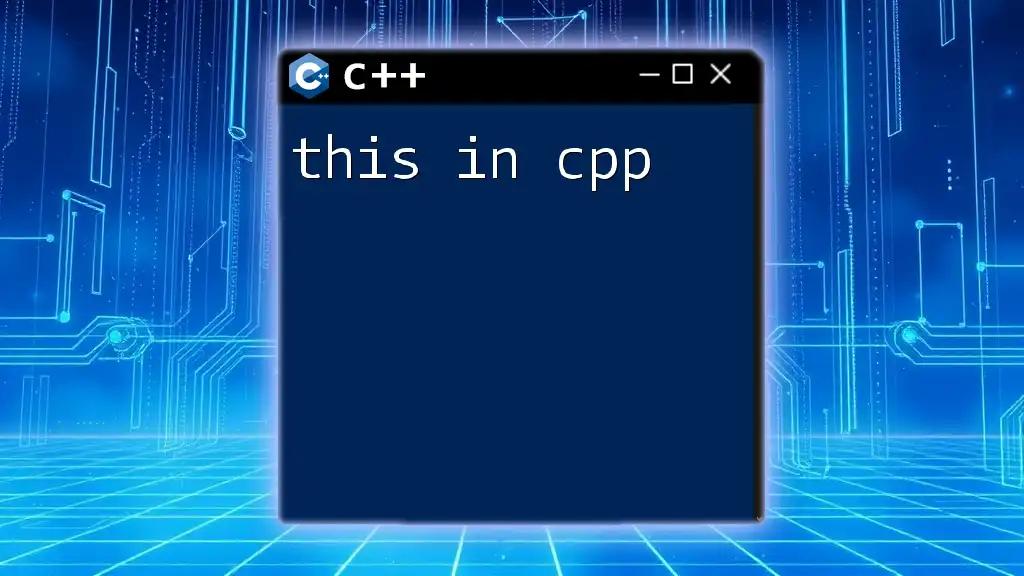
Common Pitfalls and Best Practices
Avoiding Buffer Overflow
C-style strings can lead to buffer overflow issues if you're not careful with memory allocation. It's essential to always ensure that your arrays are large enough to hold the intended data.
Using Standard Libraries
Making use of the `<string>` library in C++ is vital for safety and convenience. The rich set of functions provided by `std::string` allows you to manipulate strings without worrying about memory management.
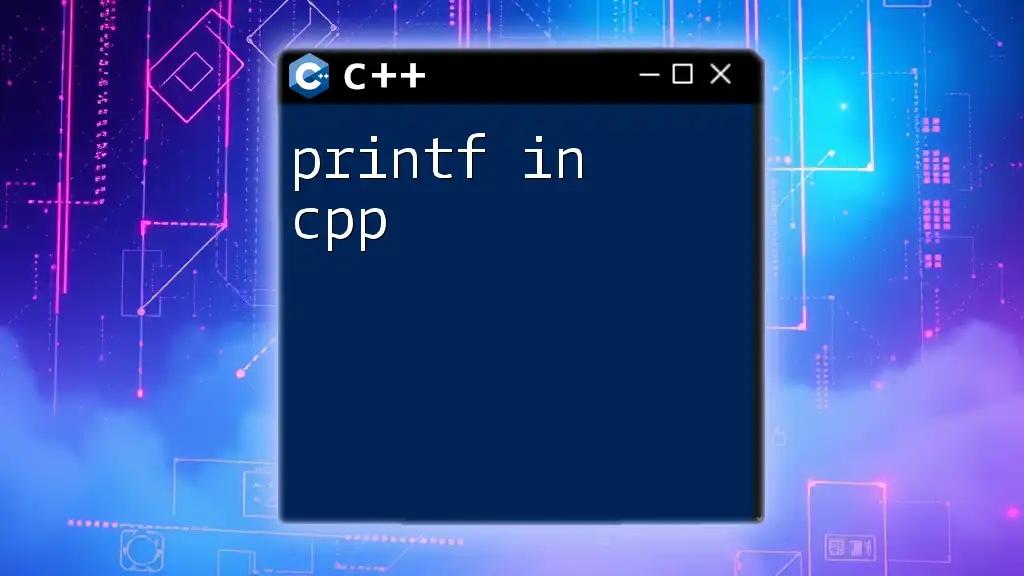
The Importance of Mastering Strings in C++
Understanding how to work with strings in C++ is not just a checkbox in your learning journey; it is central to effective programming. Strings are everywhere, from user interfaces to file handling and networking. Mastering strings in C++ will empower you to build more robust and efficient applications.

Additional Resources
To deepen your knowledge about strings in C++, consider exploring additional books, online tutorials, and code repositories. Engaging in coding challenges that focus on string manipulation will significantly enhance your programming skills and confidence.
This guide aims to provide you with foundational knowledge about strings in C++, equipping you with the tools needed for both basic and advanced string operations. As you practice, you'll find that working with strings becomes second nature, helping you write cleaner and more efficient code.