In C++, a string is a sequence of characters managed by the `std::string` class, which facilitates manipulation and storage of textual data.
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
What is a String in C++?
Definition
A string in C++ is defined as a sequence of characters used to represent text. Strings can be manipulated, compared, and altered with ease, thanks to C++'s standard library, which offers robust classes and functions for working with strings.
When discussing strings in C++, it's essential to recognize the difference between C-style strings and C++ strings.
C-style Strings
C-style strings are arrays of `char` that are terminated by a null character (`\0`). Although they provide a low-level way of handling strings, they come with certain limitations, such as manual memory management and the necessity to manage the null terminator manually. Here's a basic example:
char myString[] = "Hello, World!";
In this example, `myString` is an array that stores the characters of the phrase along with a null terminator at the end. It is crucial to remember this terminator when manipulating C-style strings to prevent memory errors and undefined behaviors.
C++ Standard Library String
In contrast, C++ provides `std::string`, a part of the standard library, designed to handle string operations more conveniently. Using `std::string` simplifies many aspects of working with strings. For instance:
#include <string>
std::string myString = "Hello, World!";
The C++ `std::string` class automatically manages the underlying memory, allowing you to focus on string manipulation instead of memory management issues.
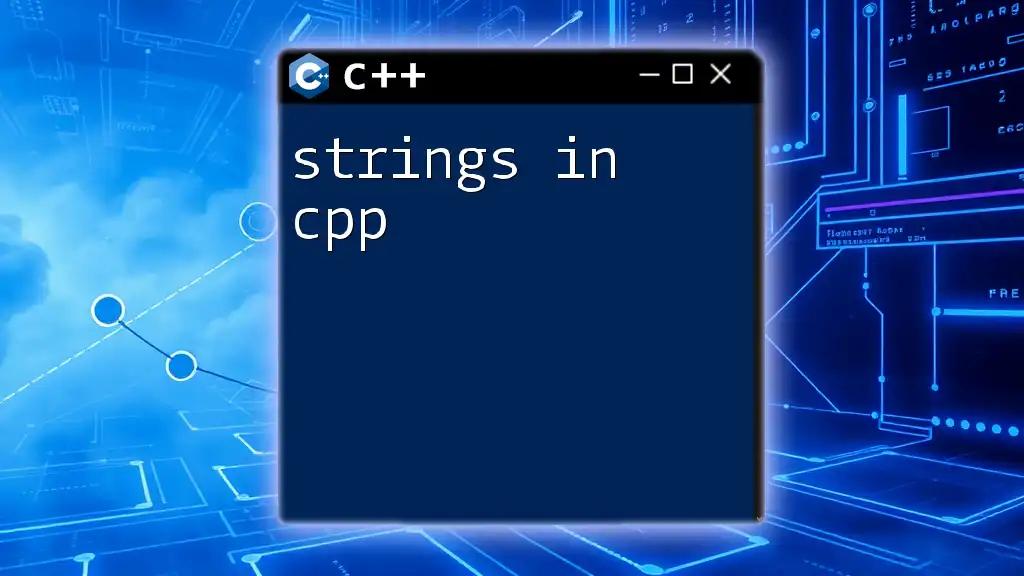
Creating Strings
Declaring Strings
You can declare and initialize strings in several ways. Here are some common methods you can use:
std::string str1 = "Hello";
std::string str2("World");
std::string str3{ 'C', 'P', 'P' };
Each of these methods is valid, with the first one being the most common.
String Length
To determine the length of a string in C++, you can utilize the `.length()` or `.size()` methods. Both methods yield the same result, returning the number of characters in the string:
std::string str = "Hello";
size_t length = str.length(); // or str.size();
This is particularly useful for validating input or performing operations that depend on string size.
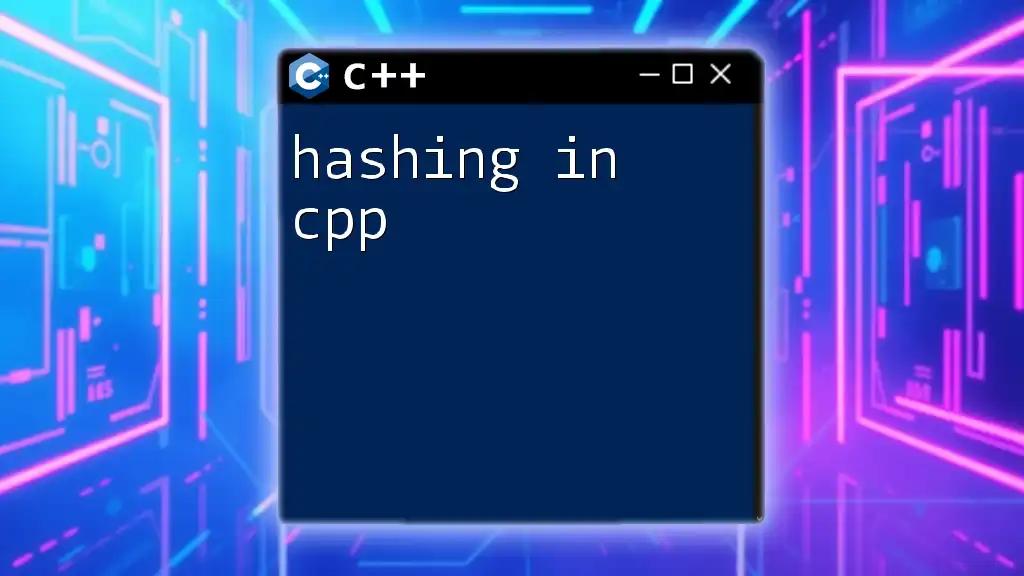
Basic String Operations
Concatenation
Combining or joining strings is known as concatenation, and in C++, you can achieve this easily using the `+` operator. Here's an example:
std::string hello = "Hello, ";
std::string world = "World!";
std::string greeting = hello + world; // "Hello, World!"
This flexibility allows for the seamless creation of more complex strings by simply adding smaller strings together.
Accessing Characters
To access individual characters in a string, C++ provides the index operator (`[]`) and the `.at()` method. Both methods can be used as shown below:
char firstChar = str[0]; // 'H'
char secondChar = str.at(1); // 'e'
While the index operator is faster, the `.at()` method provides additional bounds checking, making it a safer option.
Modifying Strings
C++ strings come with several member functions that allow you to modify them. Common operations include:
- .append(): This method adds content to the end of the string.
- .replace(): Utilizes a range to replace part of the string with another string.
- .insert(): Inserts characters into the string at a specified position.
For example:
myString.append(" Welcome!"); // "Hello, World! Welcome!"
myString.replace(0, 5, "Hi"); // "Hi, World! Welcome!"
myString.insert(3, " my"); // "Hi my, World! Welcome!"
These methods make it easy to modify and manipulate strings dynamically.

Advanced String Operations
Substrings
C++ strings allow you to extract a portion of the string through the `.substr()` method. This function takes two parameters: the starting index and the length of the substring.
std::string sub = str.substr(0, 5); // "Hello"
This property is quite useful for splitting strings or processing specific parts of larger strings.
Finding and Replacing
You can search for characters or sub-strings within a string using the `.find()` method, which returns the index of the first occurrence. Here’s how you can find and replace elements within a string:
size_t pos = str.find("World");
if (pos != std::string::npos) {
str.replace(pos, 5, "C++");
}
This code checks if "World" is found and replaces it with "C++". The `std::string::npos` value indicates that the target was not found, thus providing a straightforward way to handle string modifications.
Comparing Strings
C++ allows easy comparison of strings using simple operators such as `==`, `!=`, `<`, and more. For instance:
if (str1 == str2) {
// Strings are equal
}
Understanding string comparisons is crucial for tasks like validating user inputs and ensuring logic within code structure.

String Formatting
Using `std::stringstream`
Sometimes, formatted string output is required. `std::stringstream` in C++ allows you to create formatted strings easily. This is handy when you need to combine different data types into a single string.
#include <sstream>
std::stringstream ss;
ss << "The value of x is: " << x;
std::string str = ss.str();
Using `stringstream` enhances flexibility when assembling strings that consist of various data types.

String Iterators
Using Iterators
C++ provides iterators for strings, enabling you to loop through each character efficiently. You can use both forward and reverse iterators. Here is an example of iterating through a string:
for (auto it = myString.begin(); it != myString.end(); ++it) {
std::cout << *it; // prints each character
}
Iterators offer a convenient and powerful way to navigate through complex data structures, including strings.

String Conversions
Conversion between std::string and C-style strings
It’s sometimes necessary to convert between `std::string` and C-style strings. You can do so with the `.c_str()` method to retrieve a C-style string from an `std::string`, and by using constructors to convert back:
const char* cStr = myString.c_str();
std::string newStr(cStr);
Being proficient in these conversions is essential, especially when interfacing with APIs or libraries that require C-style strings.
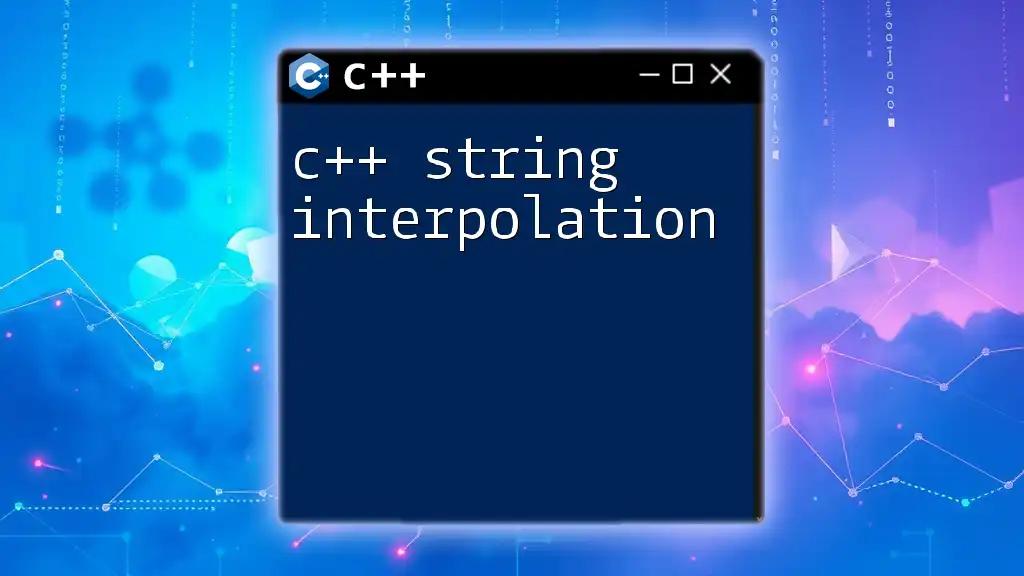
Common String Problems
Handling Empty Strings
When working with strings in C++, checking for empty strings is vital. You can utilize the `.empty()` method to verify whether a string has any characters:
if (myString.empty()) {
std::cout << "String is empty!";
}
This check can help prevent errors during string manipulation or processing.
String Splitting
C++ does not have built-in functionality to split strings by delimiters. However, you can create a custom function to achieve this. Below is a simple example showing how to split a string using a specified delimiter:
std::vector<std::string> split(const std::string &s, char delimiter) {
std::stringstream ss(s);
std::string item;
std::vector<std::string> elements;
while (std::getline(ss, item, delimiter)) {
elements.push_back(item);
}
return elements;
}
Such a function is crucial for data parsing and text manipulation tasks.

Conclusion
Understanding how to effectively use strings in C++ is essential for any programmer. From basic operations like concatenation to more advanced tasks such as formatting and string manipulation, `std::string` simplifies the handling of text in C++. Embrace these techniques and practices to enhance your programming skills and streamline your coding workflow.
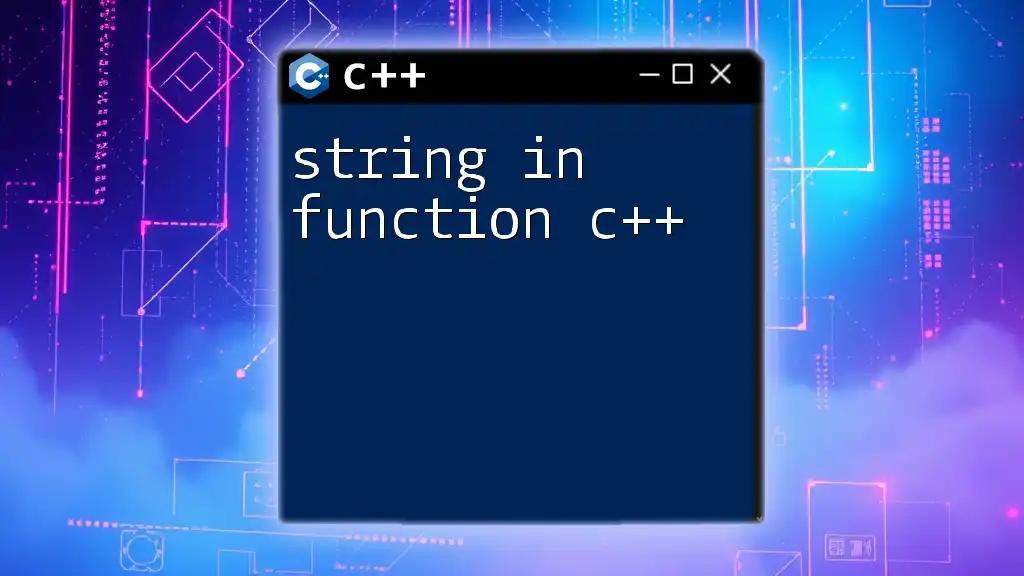
Additional Resources
To deepen your understanding of strings in C++, consider reviewing the official C++ documentation, recommended books on C++, or participating in online coding communities. Engaging with these resources will help you improve your skills and adapt to more complex programming challenges.