"Thinking in C++ means understanding and leveraging the core principles of C++ programming, such as object-oriented design and memory management, to write efficient and maintainable code."
Here’s a simple code snippet demonstrating the creation of a class in C++:
#include <iostream>
class Rectangle {
private:
int width, height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
int area() {
return width * height;
}
};
int main() {
Rectangle rect(10, 5);
std::cout << "Area: " << rect.area() << std::endl;
return 0;
}
What Does "Thinking in C++" Mean?
Thinking in C++ entails shifting your perspective from merely using C++ commands to deeply understanding the language's fundamental concepts and their implications. It involves adopting a mindset that aligns with C++’s features and paradigms, thereby elevating your coding skills beyond basic syntax.
Importance of Mindset in Mastering C++
The journey to mastering C++ begins with a creative and analytical mindset. This mindset allows developers to tackle complex problems effectively, make optimal design choices, and create maintainable code. As you grow in this journey, you will notice how a deep understanding of concepts helps you devise solutions that are not only functional but also efficient.
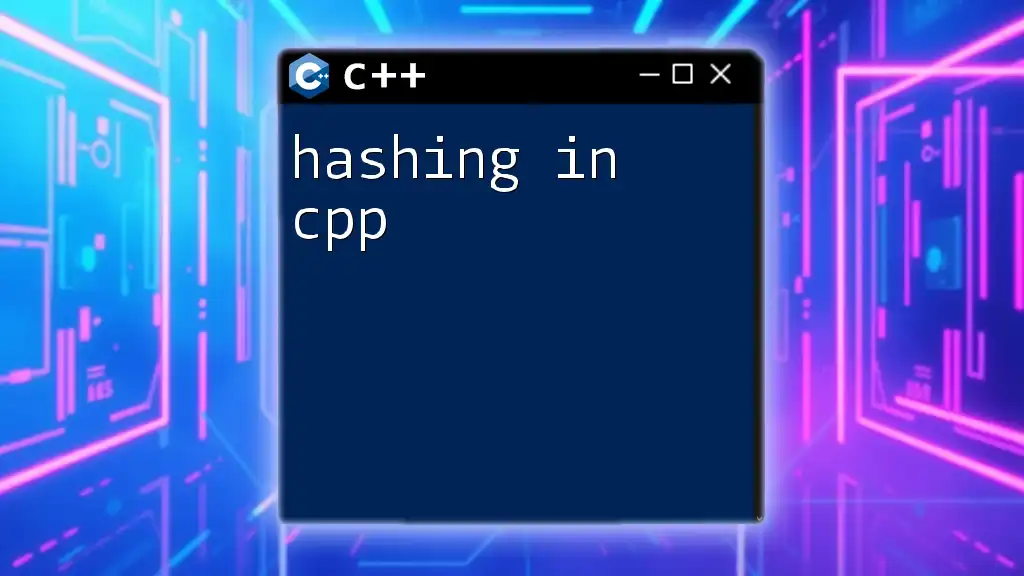
Benefits of Developing a C++ Mindset
-
Enhanced Problem-Solving Skills: A well-formed understanding of C++ translates into better analytical skills that enable you to dissect problems and forge strong solutions.
-
Improved Code Quality and Efficiency: With a thorough grasp of the language, you can write cleaner, more efficient code that adheres to best practices, making it easier for others—and yourself—to read and debug.
-
Simplified Debugging and Maintenance: Knowledge of C++ internals allows you to pinpoint issues faster and implement robust solutions, simplifying the overall debugging process.
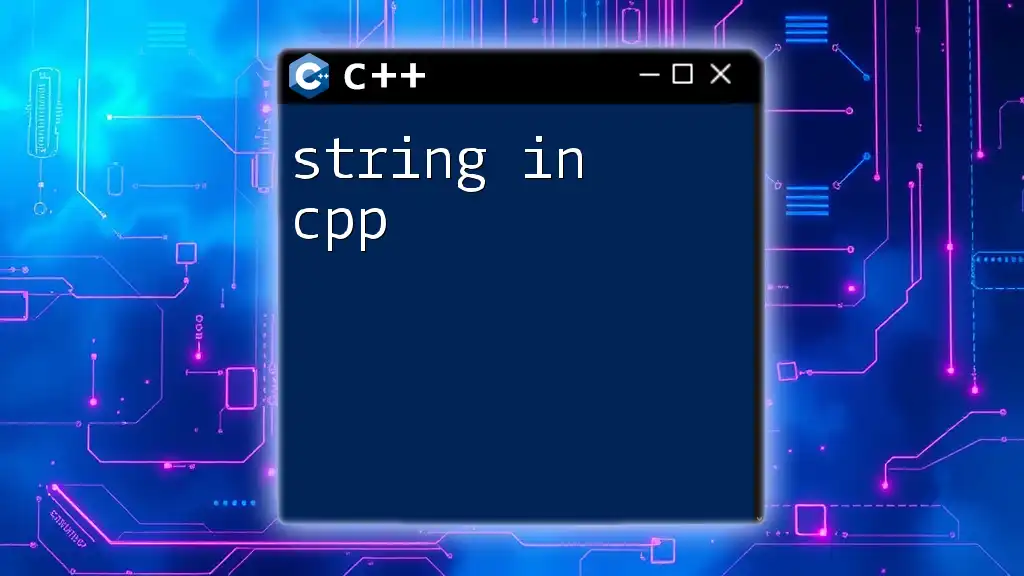
Core Concepts of C++ Thinking
Object-Oriented Programming (OOP)
To truly master thinking in C++, understanding the principles of Object-Oriented Programming (OOP) is crucial. OOP allows you to model complex systems in a more manageable way by encapsulating data and functionality.
Understanding OOP Principles
-
Encapsulation: Protecting data by bundling it with methods that operate on that data. This helps in safeguarding the integrity of the data.
-
Inheritance: Facilitating code reuse through base and derived classes. By establishing a base class, you can extend its functionality without altering its original structure.
-
Polymorphism: Enhancing code flexibility. With polymorphism, two or more classes can offer a method with the same name while performing different operations based on the object that invokes it.
Code Example: Simple OOP Implementation
class Animal {
public:
virtual void sound() {
std::cout << "Some sound" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark!" << std::endl;
}
};
In this example, the `Animal` class serves as a base class with a virtual function `sound()`. The `Dog` class inherits from `Animal` and overrides the `sound()` method to provide a specific implementation.
Memory Management
Understanding memory management is another cornerstone of thinking in C++. Unlike languages that handle memory automatically, C++ allows—and requires—you to manage memory explicitly.
Importance of Memory Management in C++
Managing memory effectively in C++ is crucial for both performance and stability. If not handled properly, issues like memory leaks and segmentation faults can arise.
Code Example: Dynamic Memory Allocation
int* arr = new int[10]; // Allocate memory
// Use the array
delete[] arr; // Deallocate memory
In this snippet, memory for an array of integers is allocated on the heap using `new` and later released with `delete[]`. This awareness of memory usage exemplifies thinking in C++.
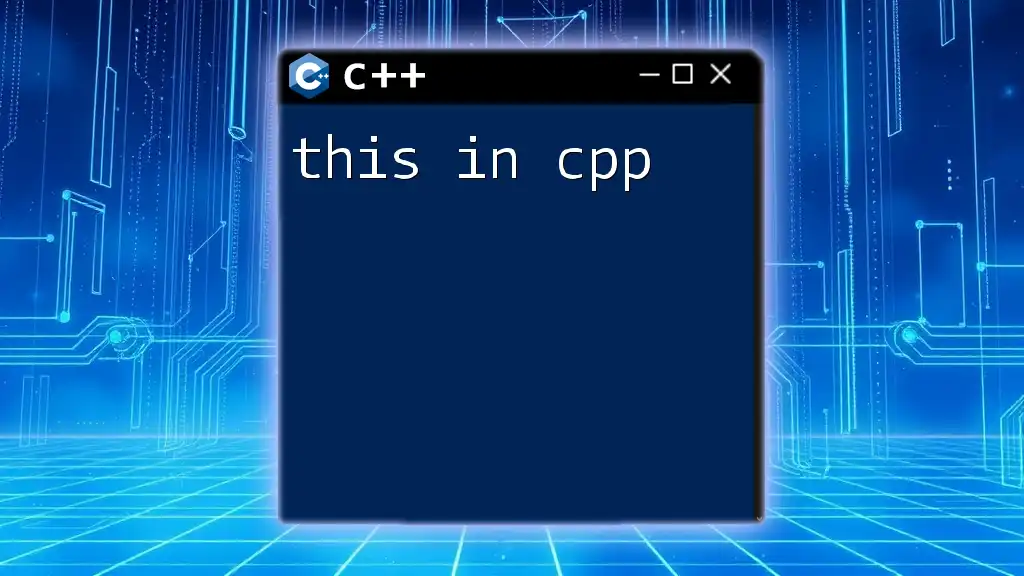
Essential C++ Features to Master
Templates and Generic Programming
Templates are a powerful feature of C++ that allows you to write generic, type-safe code. They enable you to create functions and classes that can operate with any data type.
What are Templates?
Templates allow for code reuse without compromising type safety. They enable flexibility, making your code adaptable to a variety of types while maintaining performance.
Code Example: Template Function
template<typename T>
T add(T a, T b) {
return a + b;
}
This template function `add` illustrates how one function can operate on various data types, returning the generic type `T`. By using templates, you reduce redundancy while ensuring type safety.
Standard Template Library (STL)
The Standard Template Library (STL) is an essential part of C++ that provides robust and flexible data structures and algorithms.
Understanding STL
The components of STL include:
- Containers: Such as `vector` and `map`, which store collections of data.
- Algorithms: Predefined methods for manipulating data structures.
- Iterators: Objects that allow traversal of container elements.
Using Vectors and Maps
Vectors are dynamic arrays that allow resizing, while maps are associative arrays that store key-value pairs, making data retrieval efficient.
Code Example: Using STL Map
#include <map>
#include <string>
#include <iostream>
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 28;
// Accessing and displaying values
for (const auto& pair : ageMap) {
std::cout << pair.first << " is " << pair.second << " years old." << std::endl;
}
In this example, a `map` is utilized to associate names with ages, demonstrating how STL simplifies tasks related to data handling.
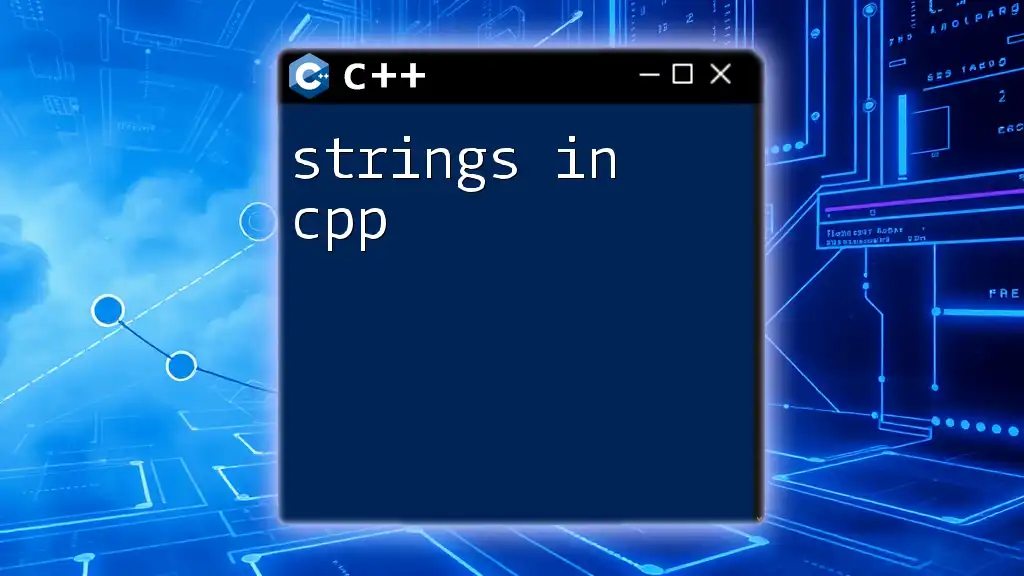
Advanced Concepts in C++
Multi-threading and Concurrency
As modern software increasingly demands responsiveness, understanding multi-threading and concurrency is essential.
Why is Multi-threading Important?
Multi-threading optimizes the utilization of system resources, allowing tasks to run concurrently. This is especially beneficial in applications needing high performance, such as games and real-time systems.
Code Example: Basic Thread Creation
#include <iostream>
#include <thread>
void threadFunc() {
std::cout << "Thread Running!" << std::endl;
}
int main() {
std::thread t(threadFunc);
t.join(); // Wait for the thread to finish
}
Here, a new thread is spawned to run `threadFunc`, showcasing how parallelism can be integrated into your applications.
Design Patterns
Familiarity with design patterns is vital for writing maintainable and scalable code. Designing reusable object-oriented solutions helps streamline development and enhances communication among developers regarding systemic problems.
Overview of Key Design Patterns
Patterns like Singleton, Factory, and Observer provide reusable solutions to common software design issues. They allow developers to use proven methods, improving code reliability and clarity.
Example: Singleton Pattern
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private constructor
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
// Initialize the static member variable
Singleton* Singleton::instance = nullptr;
In this example, the Singleton pattern ensures that only one instance of `Singleton` exists throughout the application, controlling the access to a shared resource.
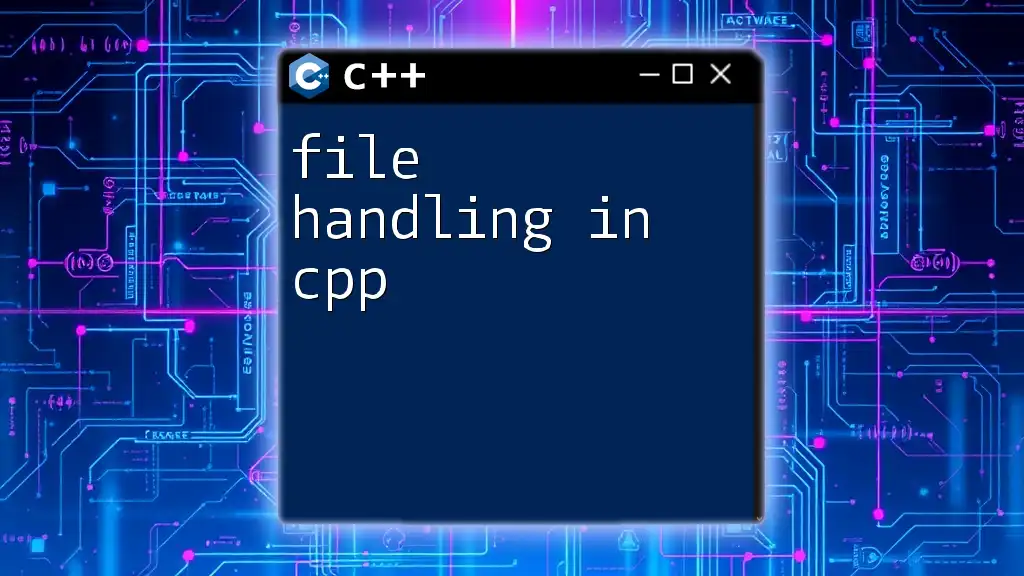
Developing a C++ Mindset
Continuous Learning and Practice
Thinking in C++ requires ongoing learning. Writing code consistently, participating in coding challenges, and tackling real-world problems help solidify your understanding of language features.
Community and Collaboration
Engaging with the C++ community can accelerate your learning curve. Whether through online forums, local meetups, or collaboration on open-source projects, interacting with peers enhances your skills and fosters creativity.
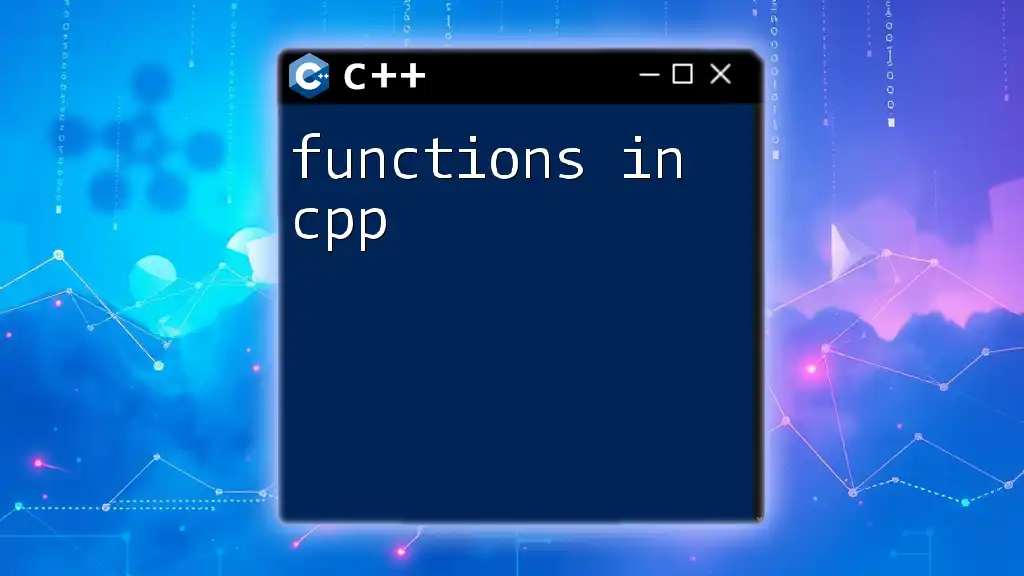
Recap of Key Points
To genuinely adopt thinking in C++, focus on understanding OOP principles, memory management, templates, and STL while diving into advanced topics like multi-threading and design patterns. Adopting this mindset will empower you to leverage C++’s full potential.
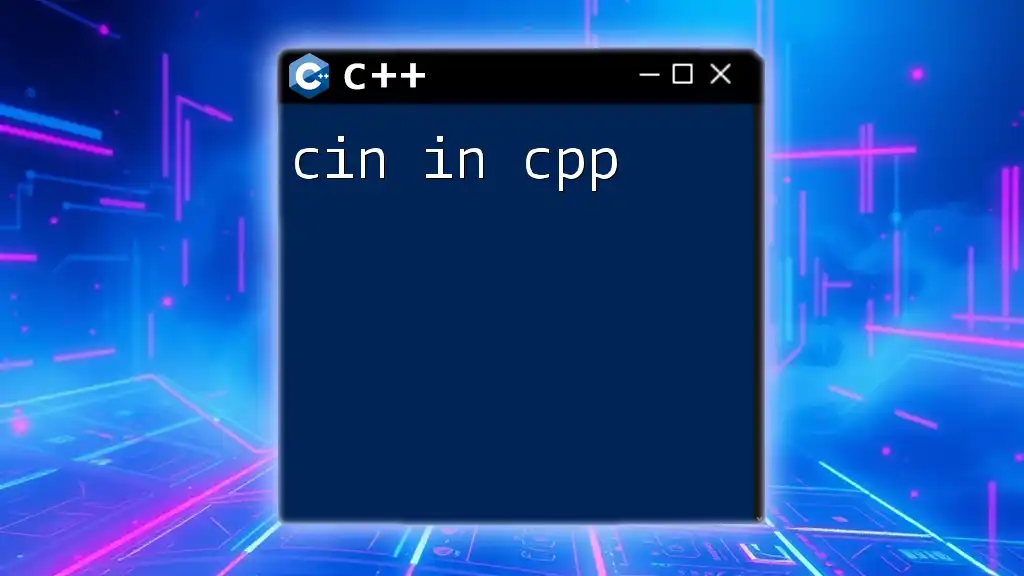
Call to Action
Embark on your journey towards thinking in C++ by embracing the principles discussed. Continue exploring advanced concepts, practice regularly, and engage with the C++ community to grow your skills and confidence as a programmer.
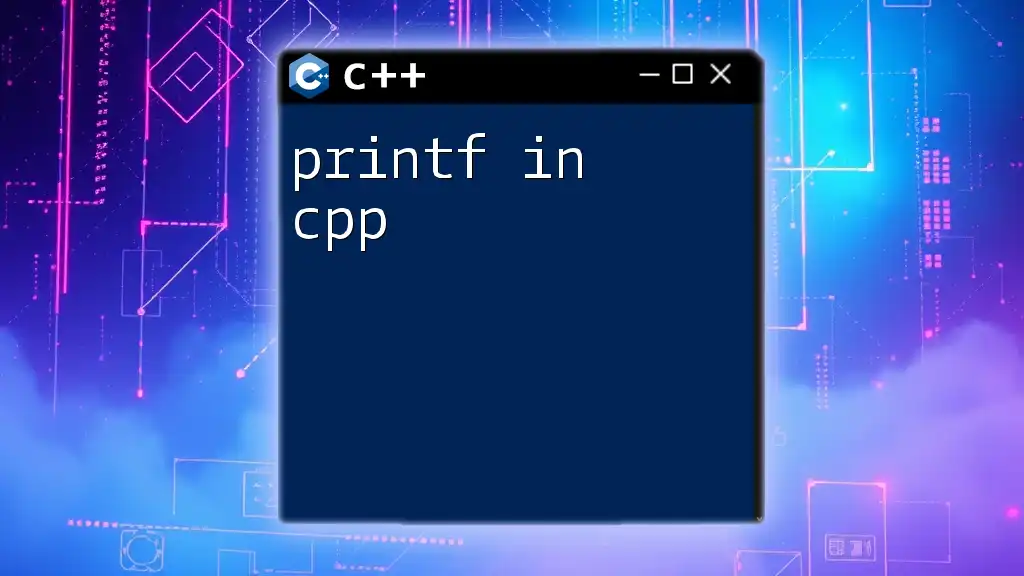
Additional Resources
For further reading and resources, consider diving into recommended books such as “Thinking in C++” by Bruce Eckel and exploring online platforms dedicated to mastering C++. The journey of thinking in C++ is rewarding—embrace it!