File handling in C++ involves performing various operations on files, such as opening, reading, writing, and closing them using file streams. Here's a simple example of writing to and reading from a text file:
#include <fstream>
#include <iostream>
int main() {
// Writing to a file
std::ofstream outFile("example.txt");
outFile << "Hello, C++ File Handling!" << std::endl;
outFile.close();
// Reading from a file
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
What is File Handling?
File handling refers to the processes of creating, reading, updating, and deleting files within a program. In the context of programming, C++ offers robust capabilities for file handling, enabling developers to manage data efficiently. With file handling in C++, you can easily store user preferences, log application data, or handle any other data that you want to persist beyond a program's execution.
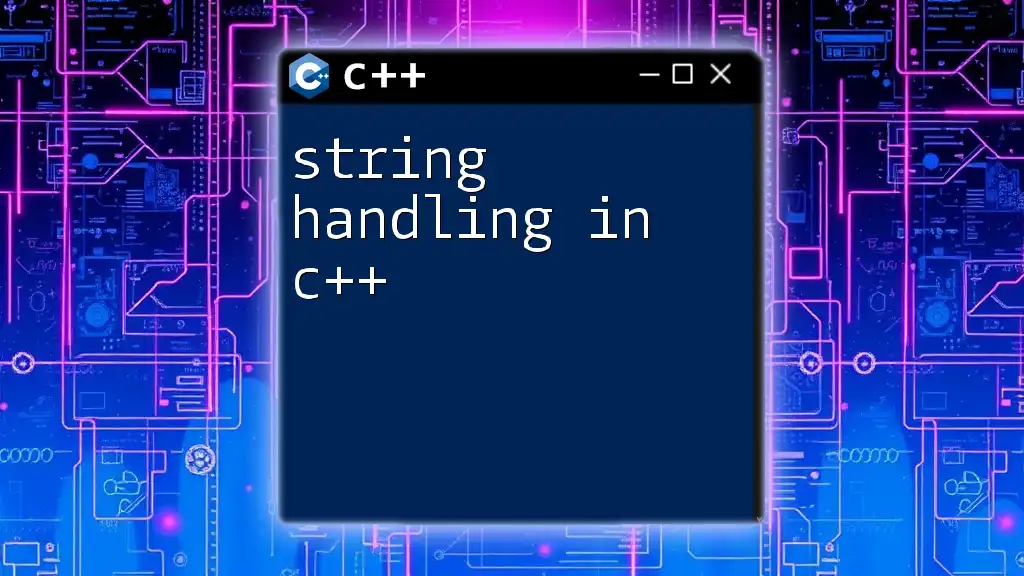
Why Use File Handling in C++?
Using file handling in C++ comes with a myriad of benefits. It allows for:
- Data Persistence: Unlike data stored in memory, files enable you to retain information even after a program terminates.
- Configuration Files: Applications can store user settings in files, enhancing user experience and customization.
- Logging: You can track the behavior of your application by writing logs for various events to a file.
Compared to using databases, file handling is often simpler for smaller applications and tasks, making it a convenient choice for quick data management.
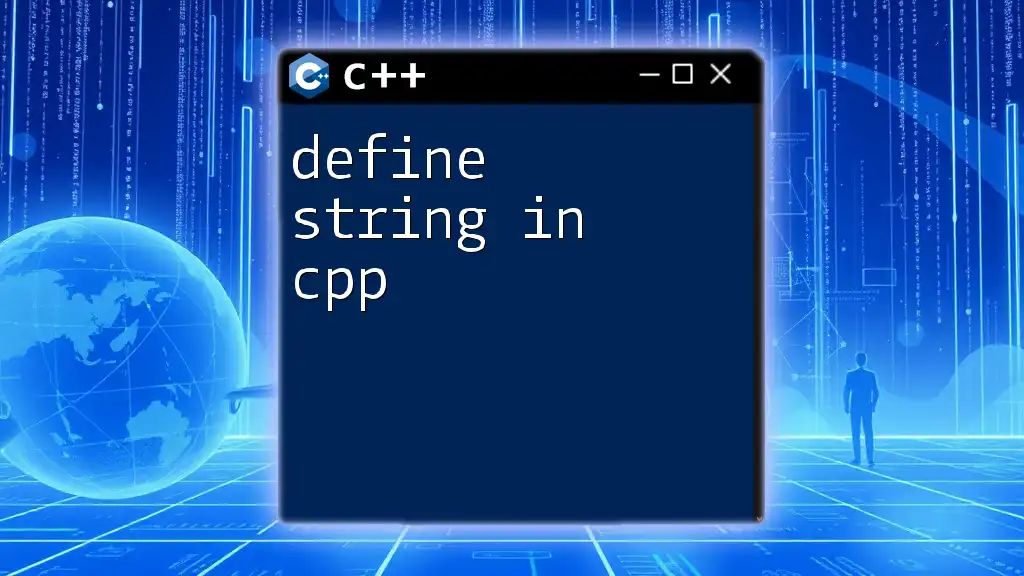
Understanding File Streams in C++
What are File Streams?
In C++, a stream is an abstraction that allows you to read and write data to various sources, including files. Streams facilitate a unified approach to data input and output, allowing you to work seamlessly regardless of the underlying data source.
Standard File Stream Classes
C++ provides several classes for file handling:
- ifstream: This class is designed for reading data from files.
- ofstream: This class is used for writing data to files.
- fstream: It allows both reading from and writing to files.
To work with file handling in C++, you need to include the fstream library:
#include <fstream>
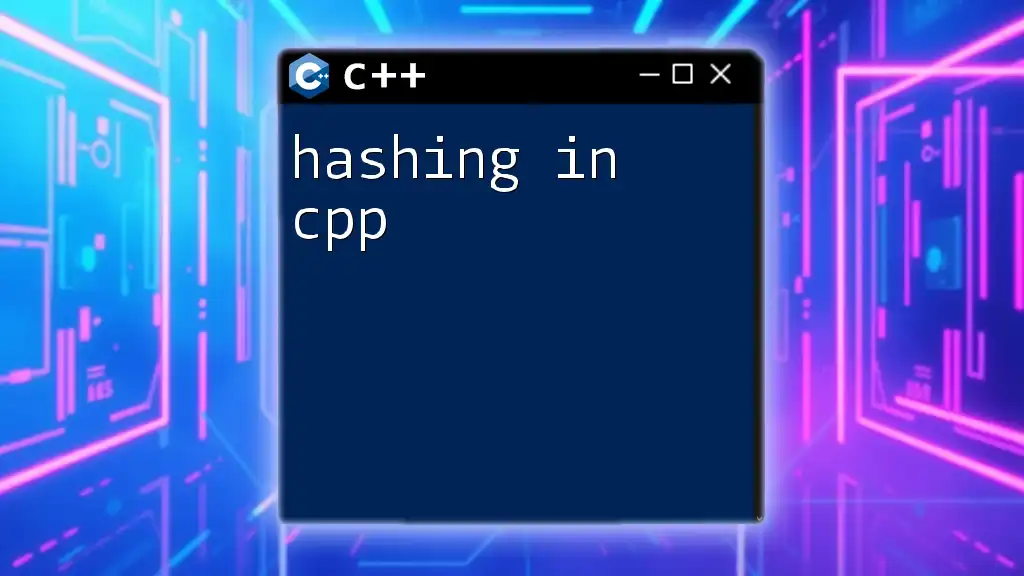
Opening and Closing Files
How to Open a File
Opening a file involves creating an instance of one of the file stream classes. The syntax is straightforward:
ifstream inputFile("example.txt"); // Opening a file for reading
In this example, if the file does not exist, the stream will fail to open, and you will need to manage this error.
How to Check File Status
Before proceeding with file operations, it’s prudent to check if the file opened successfully. You can use methods like `.is_open()` or `.fail()` to determine the status of your file.
if (inputFile.is_open()) {
// File is open and ready to be used
} else {
// Handle file opening error
}
Closing a File
Closing a file is an essential practice to release system resources. Always ensure you close files after finishing operations to prevent memory leaks:
inputFile.close(); // Closing a file after operations
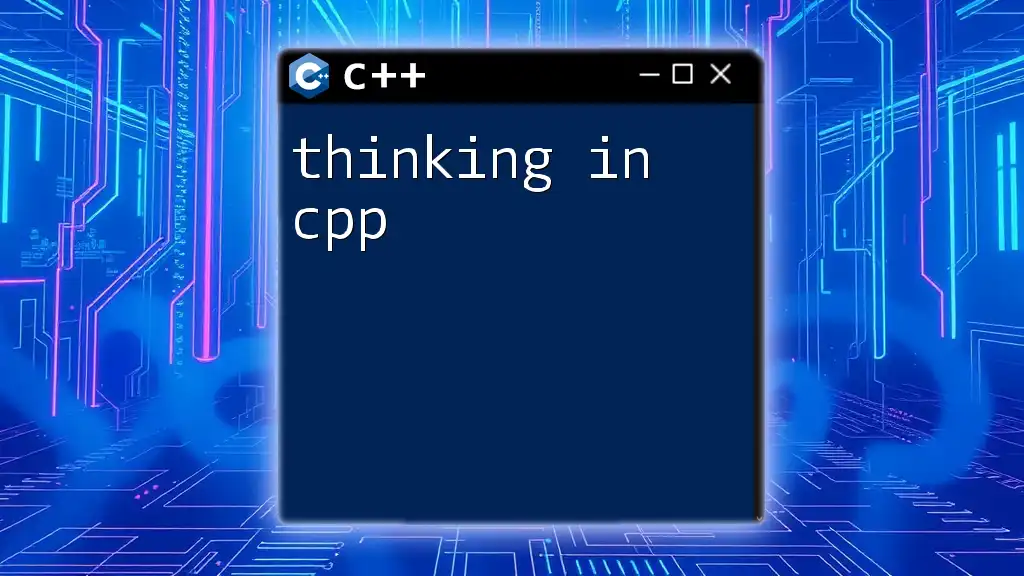
Reading from Files
Basic File Reading Techniques
C++ provides the extraction operator (`>>`) for reading data from files. This operator allows you to read formatted data easily:
int number;
inputFile >> number; // Reading an integer from the file
Reading Line by Line
Sometimes, you may want to read an entire line of text. The `getline()` function is perfect for this purpose. It reads a line from a stream until a newline character is encountered:
string line;
while (getline(inputFile, line)) {
// Process each line as needed
}
Error Handling During Reading
When dealing with file operations, errors may occur, such as file not found or incorrect format. It’s crucial to handle these errors gracefully. Always check the stream state after read operations to ensure everything went smoothly.
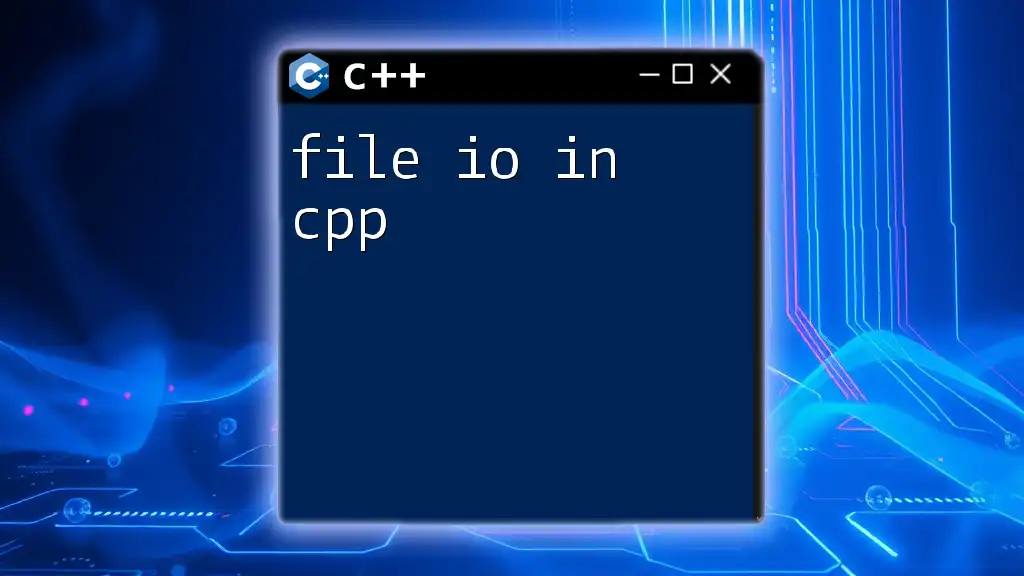
Writing to Files
Basic File Writing Techniques
Writing data to files can be similarly straightforward. Using the insertion operator (`<<`), you can write various types of data easily:
ofstream outputFile("output.txt");
outputFile << "Hello, World!"; // Writing a string to a file
Appending vs. Overwriting
When opening a file, you can specify its mode. If you want to add data to an existing file without erasing current content, use the append mode (`ios::app`):
ofstream outputFile("output.txt", ios::app); // Appending to a file
Conversely, the default mode overwrites existing data, so be cautious when creating a new `ofstream` instance without specifying `ios::app`.
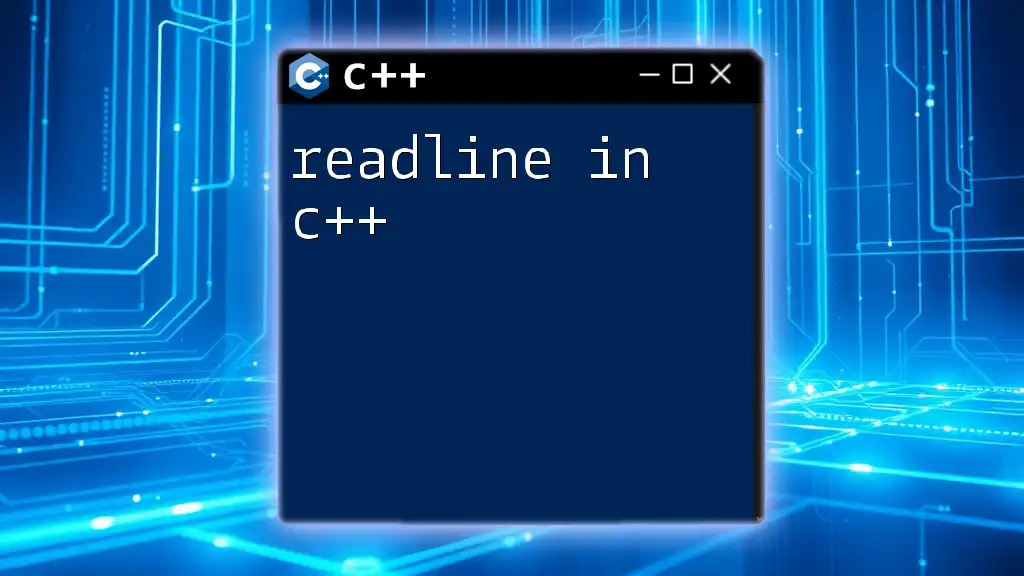
File Handling Modes in C++
Different file handling modes dictate how you can interact with a file. Here's a summary:
- `ios::in`: Open a file for reading.
- `ios::out`: Open a file for writing.
- `ios::app`: Append to a file.
- `ios::binary`: Open the file in binary mode.
Understanding these modes is crucial for effective file handling in C++.
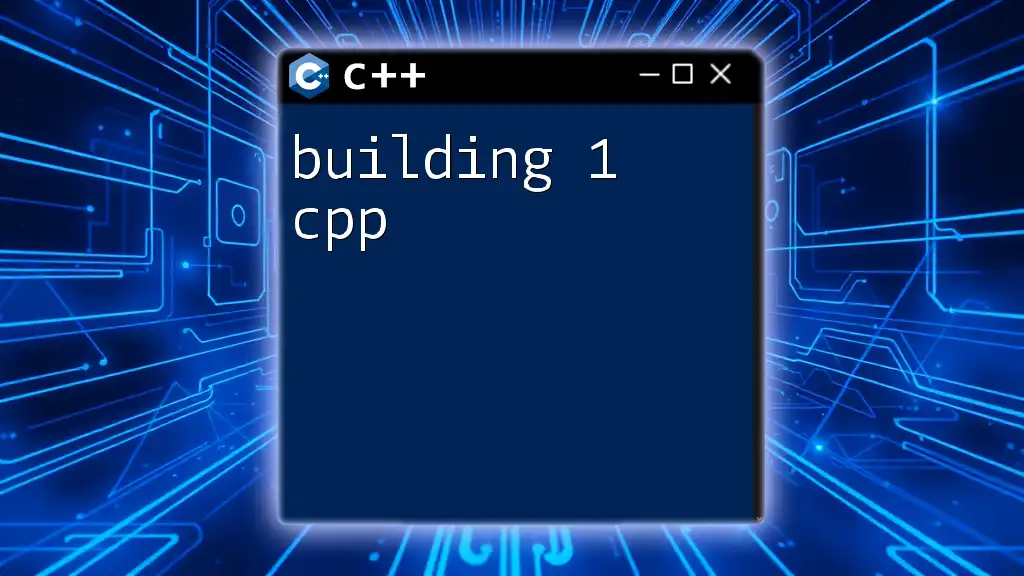
Binary File Handling in C++
What is Binary File Handling?
Binary file handling refers to the reading from and writing to files in a binary format, as opposed to text format. This is useful for storing complex data types like images, audio files, or structured data formats due to its compactness and efficiency.
Reading and Writing Binary Files
To write and read binary data, you’ll use `.write()` and `.read()` functions. Here’s an example of writing an integer to a binary file:
ofstream binaryFile("data.bin", ios::binary);
int value = 42;
binaryFile.write(reinterpret_cast<char*>(&value), sizeof(value)); // Writing an integer
Example of Struct with Binary File Operations
You can also manage complex data structures, such as structs. Here’s an example of defining a struct and writing it to a binary file:
struct Person {
char name[50];
int age;
};
Person person = {"Alice", 30};
binaryFile.write(reinterpret_cast<char*>(&person), sizeof(person)); // Writing struct data
By using binary files, you can significantly reduce file size and increase read/write speeds, but be vigilant about compatibility issues when sharing binary files between different systems.
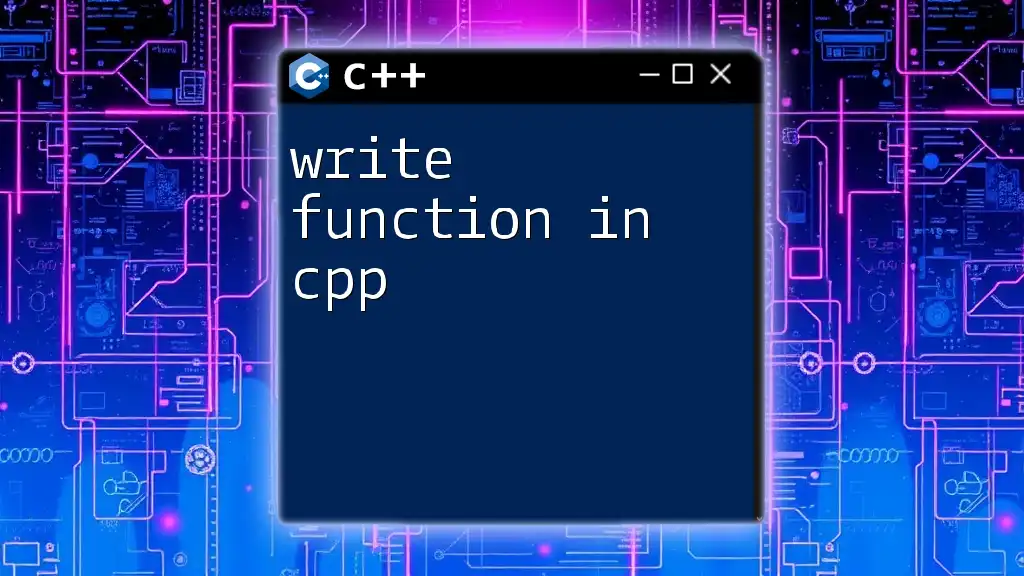
Common Mistakes in File Handling
When implementing file handling in C++, several mistakes can arise:
- Forgetting to Close Files: Failing to close a file can lead to data loss or memory issues.
- Errors in File Path: Always ensure that the specified file path is correct to avoid file not found errors.
- Incorrect File Modes: Using the wrong mode when opening a file can lead to unexpected behavior.
Be attentive to these common pitfalls to enhance your file handling practices.
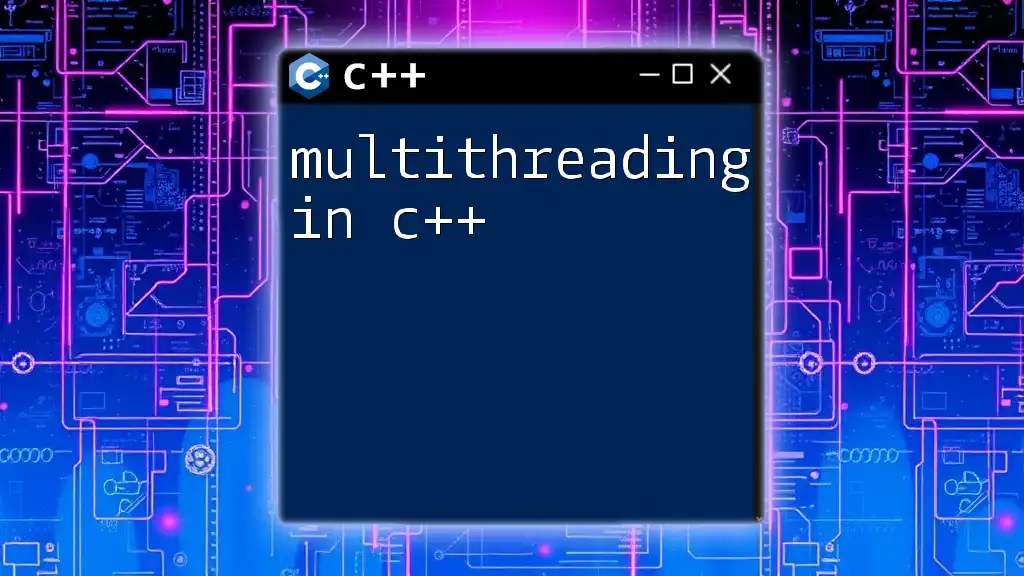
Best Practices for File Handling in C++
To ensure efficient and error-free file handling, consider the following best practices:
- Consistent Error Checking: Make it a habit to check for errors at every stage of file operations. This vigilance helps you catch issues sooner rather than later.
- Resource Management: Employ RAII (Resource Acquisition Is Initialization) principles to manage file resource allocation and deallocation automagically.
- Using Smart Pointers: Enhance resource management by employing smart pointers where appropriate for automatic cleanup.
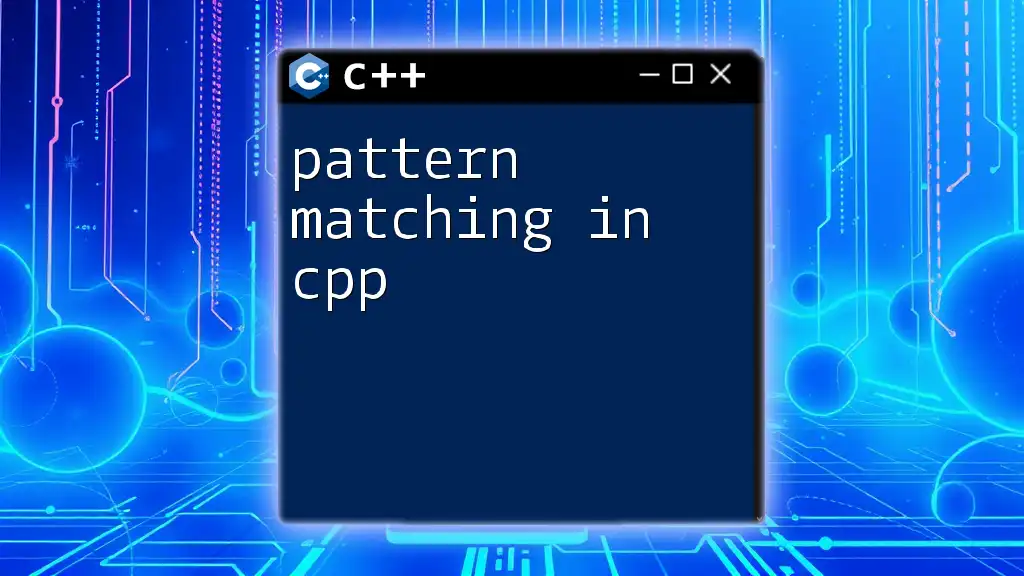
Conclusion
Mastering file handling in C++ is vital for anyone looking to implement data persistence solutions effectively. By understanding streams, managing file operations carefully, and adhering to best practices, you can robustly handle data in various forms. Whether you are developing a simple application or a complex system, skillful file handling will pave the way toward successful data management.
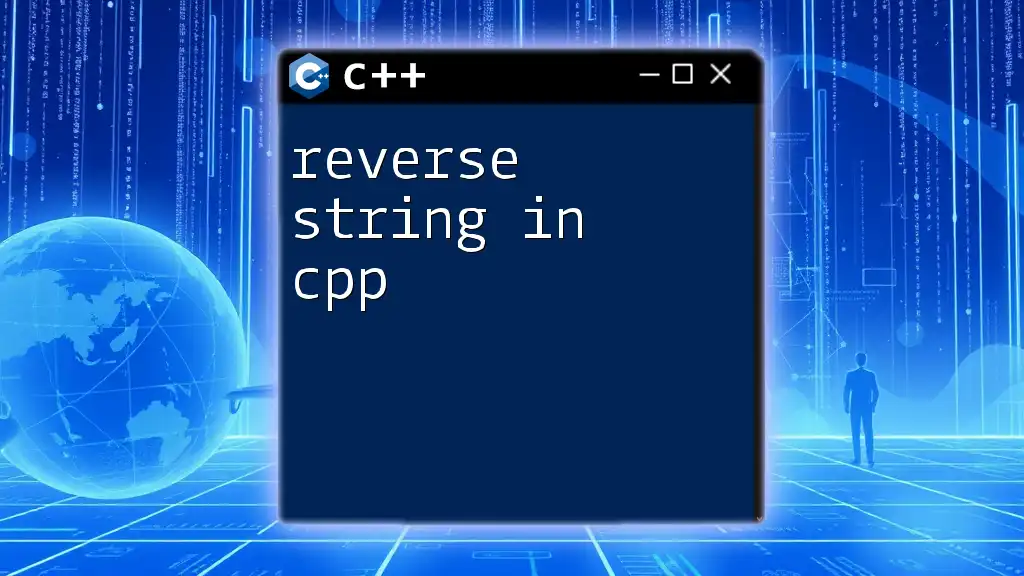
Call to Action
Join our community today for more concise and practical C++ tutorials. Perfect your skills in file handling and beyond as you take your programming journey to the next level!