"Building a simple C++ program typically involves writing a main function that serves as the entry point, where you can include headers, execute commands, and display outputs."
Here’s a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Command Structure
C++ syntax is foundational to effectively programming in this powerful language. At its core, a C++ program consists of a sequence of instructions that are executed to achieve a specific task. Each instruction follows rules laid out by the C++ syntax, which needs to be grasped to write effective code.
Common Components of C++ Programs
A C++ program typically consists of several key components:
-
Header Files: These files contain declarations that introduce functions, classes, and variables from standard libraries.
-
main() Function: This is the entry point of any C++ program. When you execute a C++ application, the operating system looks for the `main()` function to start running the code.
-
Standard Input/Output (I/O): C++ uses streams for input and output operations. The `iostream` library is commonly used to handle console input and output.
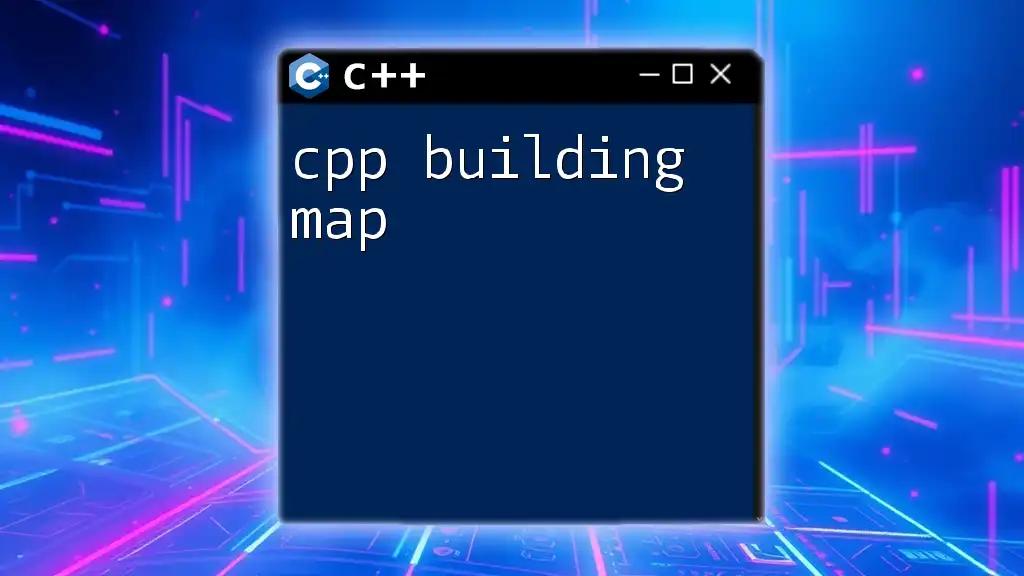
Setting Up Your Development Environment
Before you can start building your C++ program, it’s important to set up a conducive development environment.
Choosing the Right Compiler
Choosing an appropriate C++ compiler is crucial. Some of the most popular options include:
- GCC (GNU Compiler Collection): Widely used on Linux systems.
- MSVC (Microsoft Visual C++): A part of the Visual Studio suite, best for Windows.
- Clang: Known for its fast compilation and excellent diagnostics.
To install a compiler, visit the official site of the compiler you choose and follow the instructions specific to your operating system.
Setting Up an IDE
An Integrated Development Environment (IDE) simplifies the coding process with features like code highlighting, auto-completion, and debugging tools. Here are a few popular IDEs:
- Visual Studio: Excellent for Windows with a robust feature set.
- Code::Blocks: Lightweight and customizable, it’s available on multiple platforms.
- CodeLite: A free, open-source IDE that supports various compilers.
To set up an IDE, download and install the software of your choice, then configure it to use the compiler you installed.
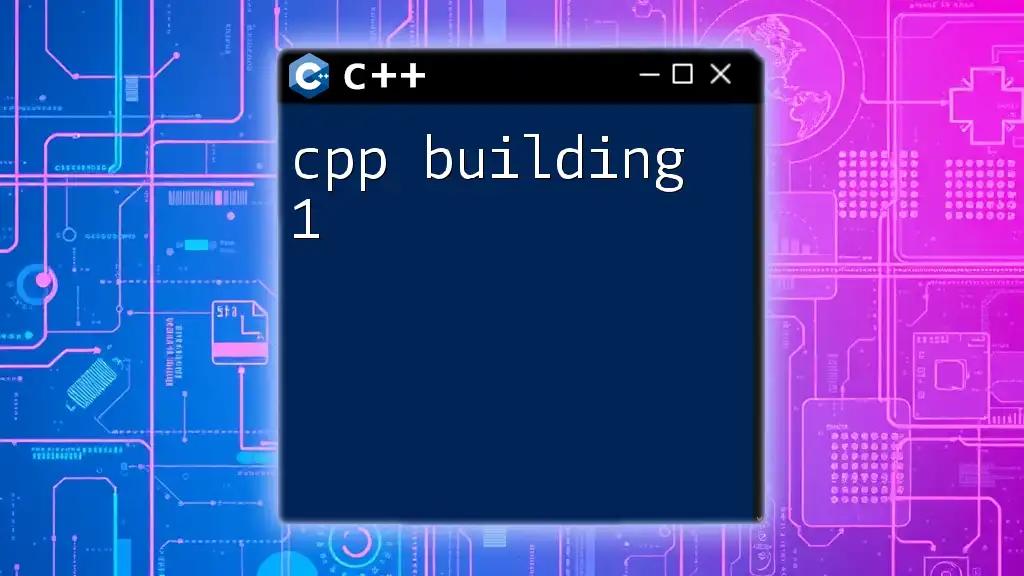
Writing Your First C++ Program
Now that you have your environment set up, it's time to write your first C++ program.
Creating a New Project
In your chosen IDE, locate the option to create a new project. When prompted, choose the appropriate C++ project template. Create a new source file within your project.
Hello World Example
The quintessential first program in any language is the "Hello, World!" program. This program outputs a simple greeting to the console.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Code Explanation:
- `#include <iostream>`: This line includes the input-output stream library, which is necessary for using `std::cout`.
- `int main()`: This declares the `main()` function, which returns an integer value upon completion.
- `std::cout << "Hello, World!" << std::endl;`: This line prints "Hello, World!" to the console followed by a newline.
- `return 0;`: This signifies that the program finished successfully.
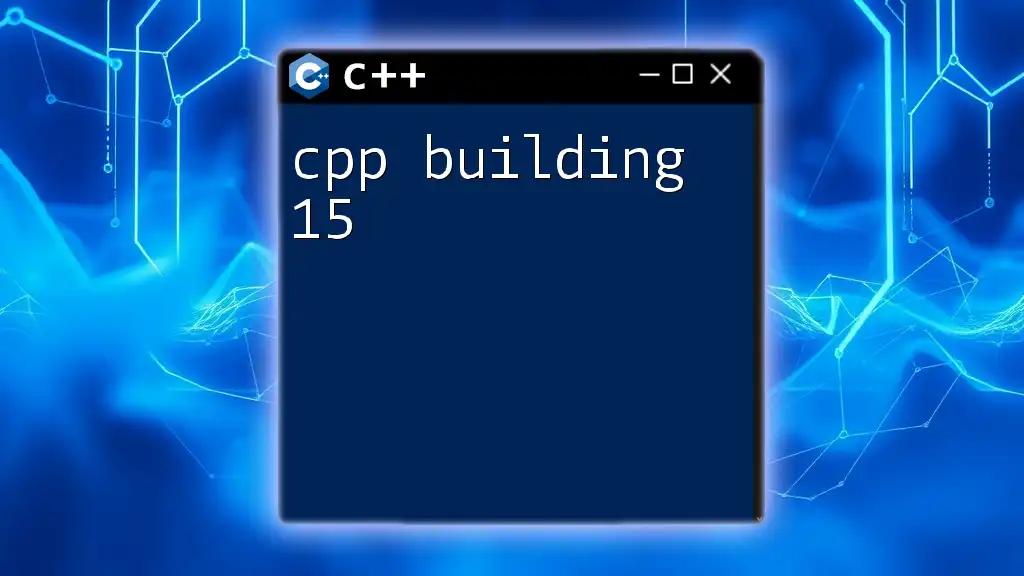
Compiling and Running Your C++ Program
After writing your program, the next step is to compile and run it.
Compilation Process
Compiling is the process of converting your C++ code into machine code that the computer can understand. This transformation occurs in stages, with various checks along the way. Understanding compilation errors can save you considerable debugging time.
Running the Program
Once compiled successfully, running your program is straightforward. Whether you’re using a terminal or your IDE, typically, you will execute a command (in the case of the command line) or press a "Run" button (in the IDE).
If you’re using the command line, here’s how it would look:
g++ -o hello hello.cpp
./hello
The first command compiles the code, while the second command runs the compiled program.
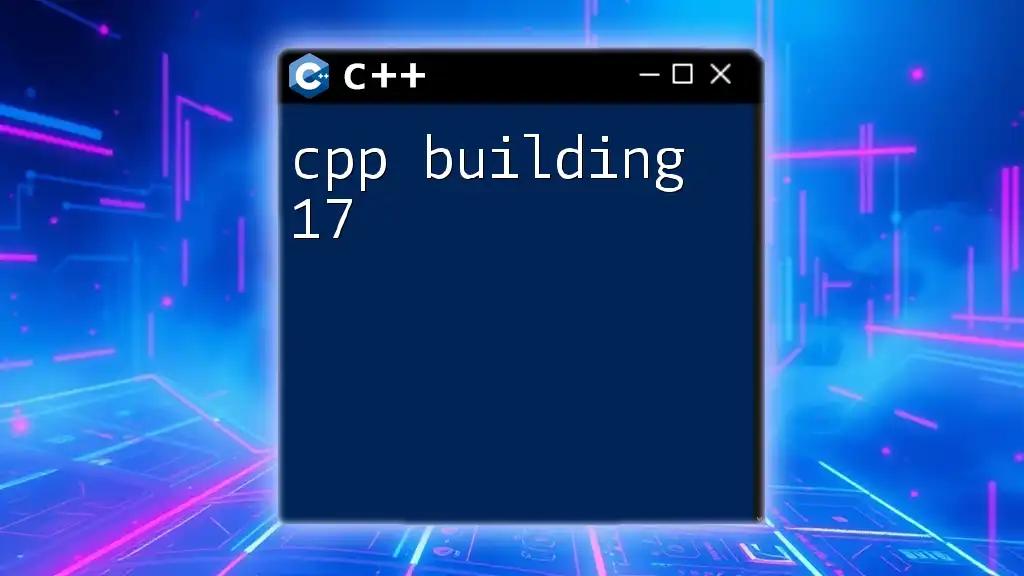
Leveraging C++ Command Line for Effective Development
Having a strong grasp of command-line tools can significantly improve your efficiency in C++ development.
Using Command Line Tools
Understanding basic command-line instructions will allow you to compile and execute your C++ files quickly. Commands like `g++` or `clang++` are essential for compiling your C++ programs without relying solely on an IDE.
Example Commands for Compiling and Running a C++ File
g++ -o hello hello.cpp
./hello
These commands will compile and run the "Hello World!" program you created earlier. Mastering such commands will enable you to work more flexibly and avoid the overhead of graphical interfaces when unnecessary.
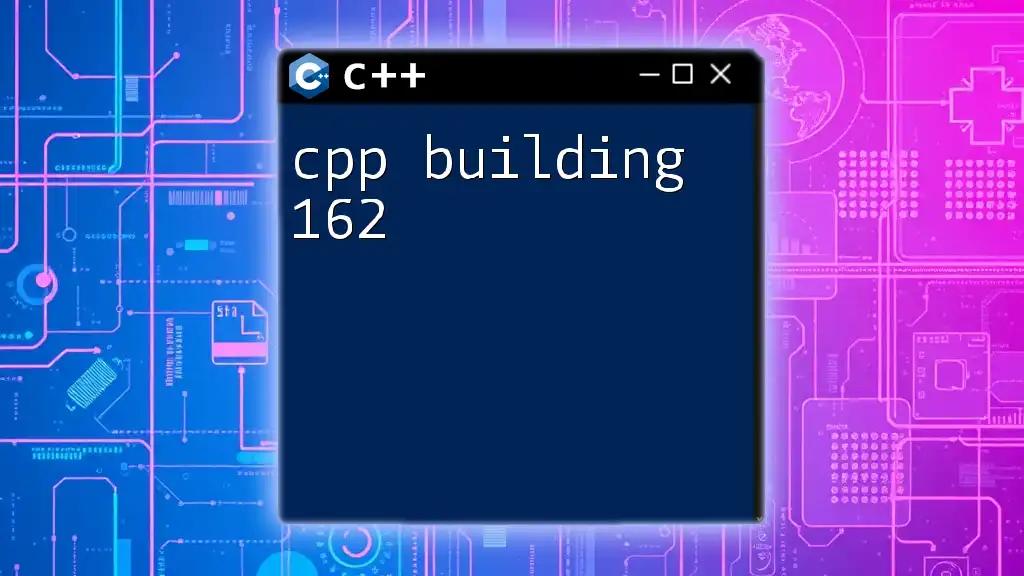
Basic C++ Commands and Concepts
To understand building 1 cpp, it's vital to familiarize yourself with basic C++ commands and concepts.
Variables and Data Types
Variables are the cornerstone of programming. C++ supports various data types, which dictate what kind of data a variable can hold. Common types include:
- int: for integers
- double: for floating-point numbers
- char: for single characters
Example of variable declarations:
int age = 30;
double height = 5.9;
char initial = 'J';
Control Structures
Control structures such as loops and conditional statements dictate the flow of your program.
If Statements & Loops:
if (age >= 18) {
std::cout << "You are an adult." << std::endl;
} else {
std::cout << "You are a minor." << std::endl;
}
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Functions
Functions encapsulate specific tasks you may want to perform repeatedly, promoting reusability and organization in your code.
Defining and Calling Functions:
int add(int a, int b) {
return a + b;
}
This simple `add` function takes two integers as parameters and returns their sum.
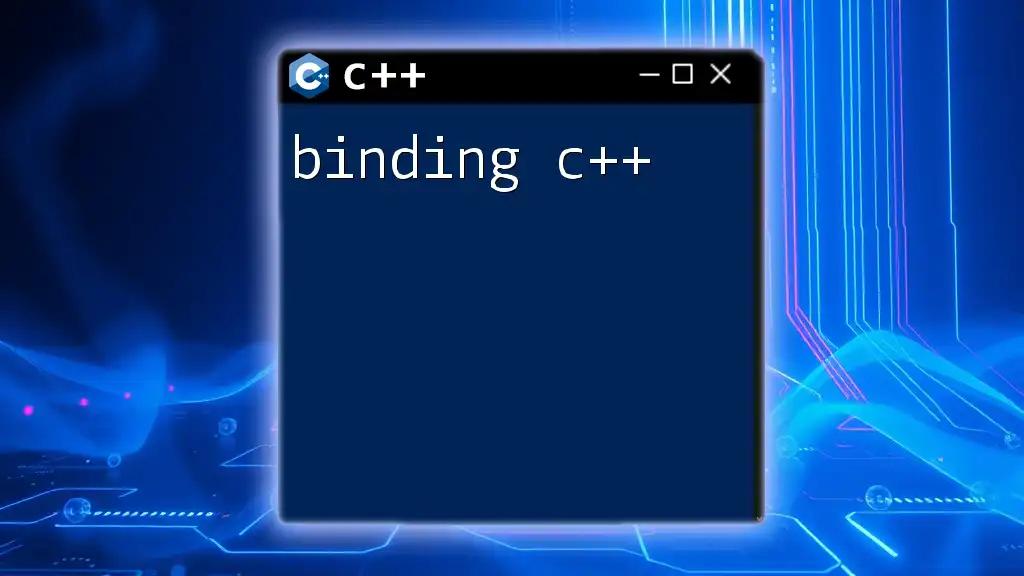
Debugging Your C++ Program
Debugging is a crucial skill in programming. Knowing how to efficiently identify and correct errors will save you time and help you understand your own code better.
Common Debugging Techniques
Familiarize yourself with debugging tools available in your IDE, such as breakpoints, watch variables, and step execution. Using these tools will help you isolate issues more quickly than relying on print statements alone.
Using Print Statements for Debugging
While IDEs provide advanced debugging capabilities, simple print statements often suffice for smaller issues. Insert `std::cout` statements to track variable states throughout your program.
int main() {
int result = add(5, 3);
std::cout << "Result of adding 5 and 3: " << result << std::endl;
return 0;
}
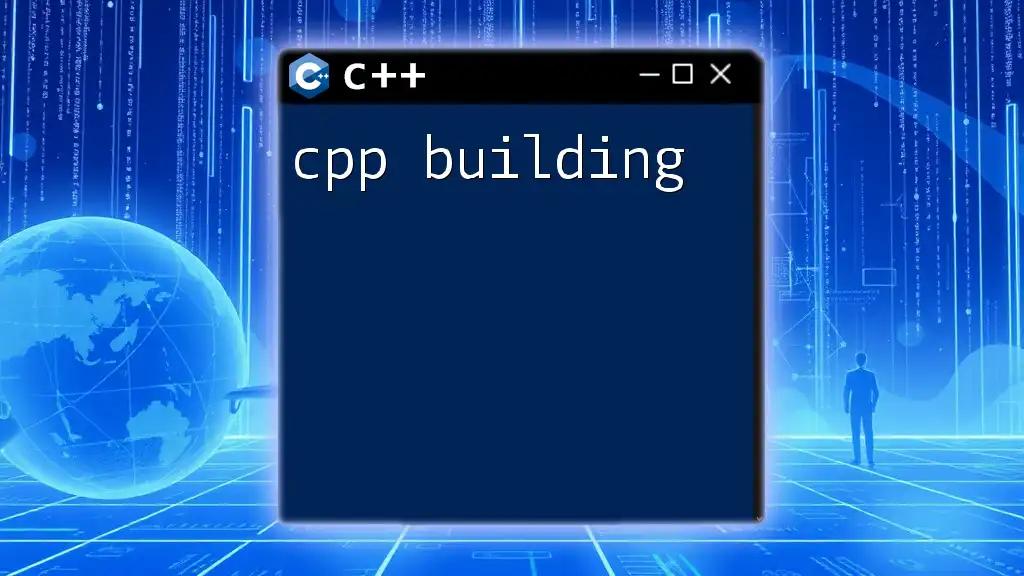
Conclusion
Learning to effectively use C++ commands is an essential step in your programming journey. The processes of compiling, debugging, and executing your programs serve as the backbone of your development experience.
By grasping basic commands, understanding program structure, and setting up the right environment, you position yourself for continued success in mastering C++.
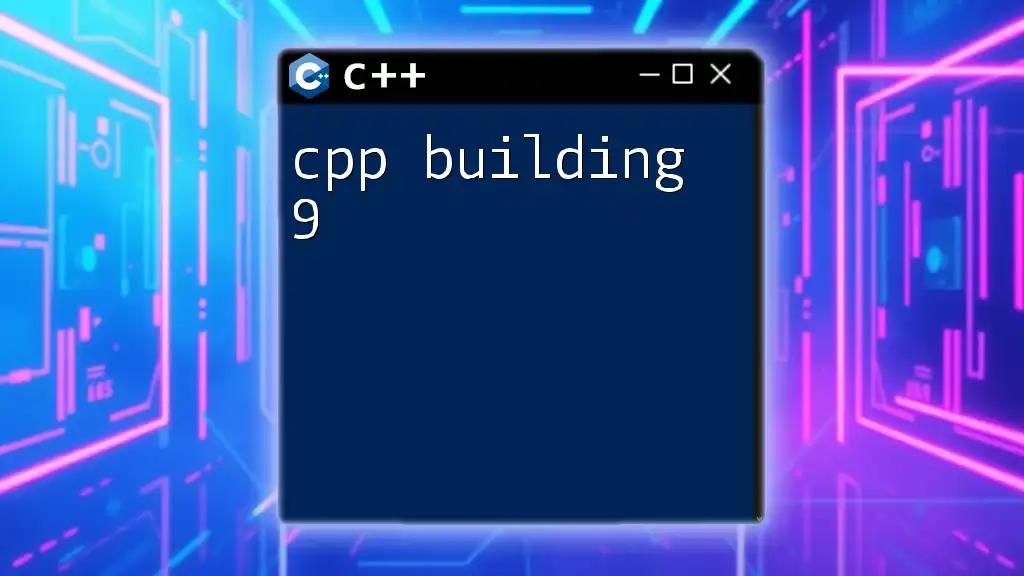
Additional Resources
To further enhance your knowledge, consider exploring the following resources:
- C++ Documentation: Official C++ documentation is a great place to deepen your understanding of language features.
- Community Forums: Engage with communities such as Stack Overflow and Reddit to learn from experienced developers.
- Online Coding Platforms: Websites like LeetCode and HackerRank offer challenges that can bolster your C++ skills.
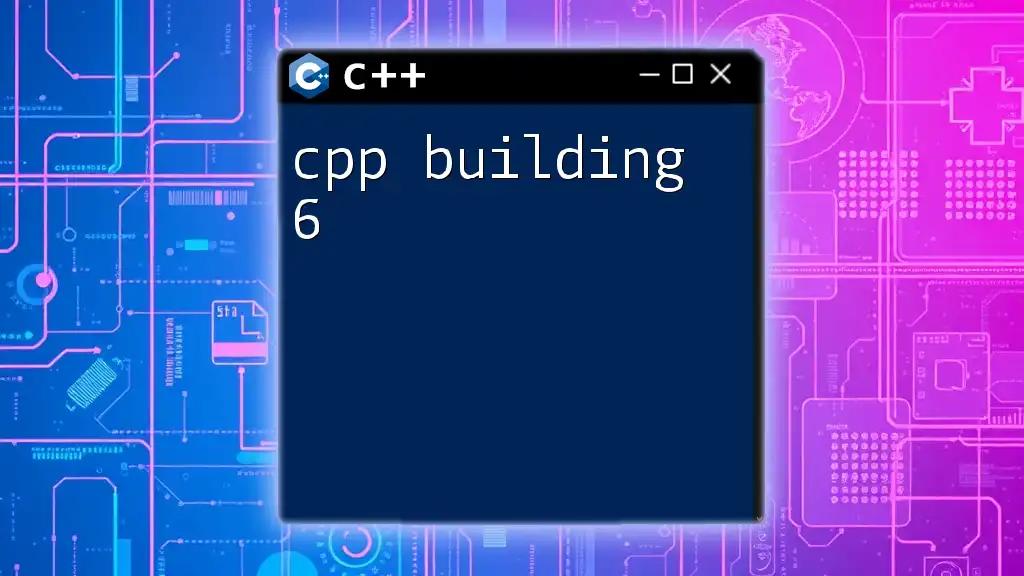
Call to Action
Feel free to share your experiences or queries regarding C++ in the comments below! If you’re interested in learning more about mastering C++ commands in a quick, concise manner, don’t hesitate to check out our company's services. We’re here to help you on your programming journey!