"CPP Building 24" refers to the efficient compilation and execution of a C++ program that demonstrates the use of single-file projects incorporating basic C++ features.
#include <iostream>
int main() {
std::cout << "Hello, CPP Building 24!" << std::endl;
return 0;
}
Understanding C++ Build Systems
What is a Build System?
A build system is a crucial part of software development. It automates the process of compiling source code, linking dependencies, and generating executable programs or libraries. By using a build system, developers can save time and reduce errors that might occur when managing builds manually.
A well-structured build system streamlines the development process, ensuring that changes in the codebase are efficiently compiled, and all dependencies are properly managed. The importance of using a build system in large projects cannot be overstated. It helps convene order in complexity, allowing for smooth collaboration among teams.
Key Features of a Build System
A robust build system comprises several key features, including:
- Dependency management: Automatically identifies and builds only the parts of the project that have changed.
- File organization: Keeps the source code, headers, and build files systematically organized.
- Build automation: Allows users to compile their software with simple commands, reducing human error.
The most common tools in the C++ world include Make, CMake, and Ninja. Each has its own strengths and weaknesses, but they all contribute to efficient management of the build process.
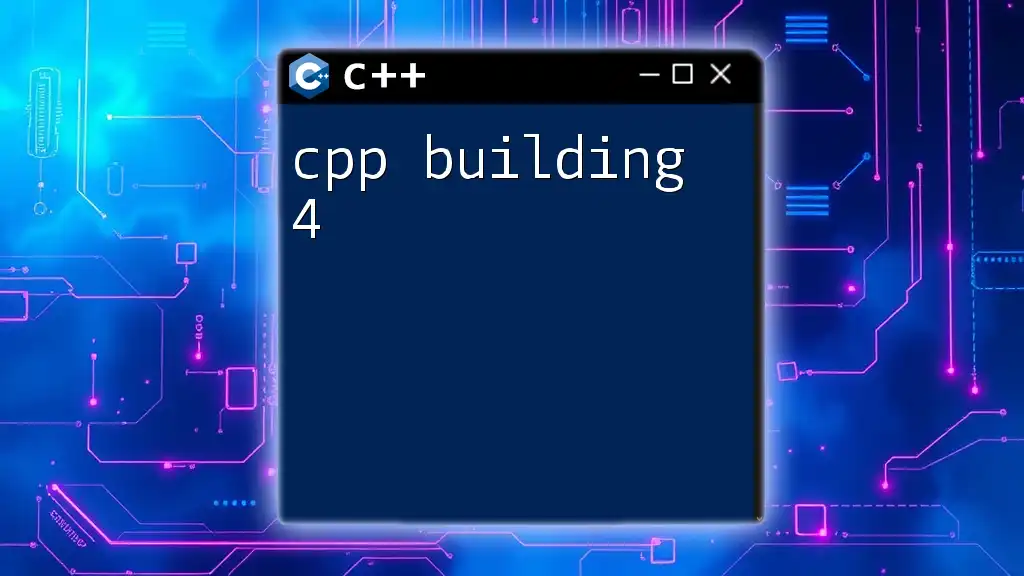
C++ Building 24 Explained
What Does "CPP Building 24" Refer To?
"CPP Building 24" refers to a specific framework or methodology in C++ programming designed to enhance build efficiency and streamline workflows in modern software development. This concept highlights best practices and tools that aid in the organization and automation of building C++ projects.
It serves as a guide for developers to adopt optimal structures in their code and build processes, resulting in improved code quality and reduced build times. Understanding and implementing "CPP Building 24" can significantly ease the burden that comes with managing large and complex C++ applications.
Key Components of C++ Building 24
Source Code Organization
Organizing source code properly is vital for any C++ project. A clean folder structure not only improves readability but also plays a critical role in maintaining the project's integrity. Here is an example of a typical project layout:
/MyProject
├── src
│ └── main.cpp
├── include
│ └── myheader.h
├── build
└── CMakeLists.txt
In this structure:
- The src directory holds the main source code files.
- The include directory contains header files, promoting code reuse.
- The build directory is where the compilation happens.
- The CMakeLists.txt file is crucial for defining the build process.
CMakeLists.txt Explained
CMake is one of the most popular build tools used in C++ programming. It simplifies the compilation process by generating makefiles or project files for various IDEs. Understanding the structure of `CMakeLists.txt` is essential in "CPP Building 24". This is how a simple `CMakeLists.txt` looks:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
add_executable(MyExecutable src/main.cpp)
include_directories(include)
In this example:
- The minimum required CMake version is specified.
- The project name is defined.
- An executable target is created from the main source file, and directories for headers are included.
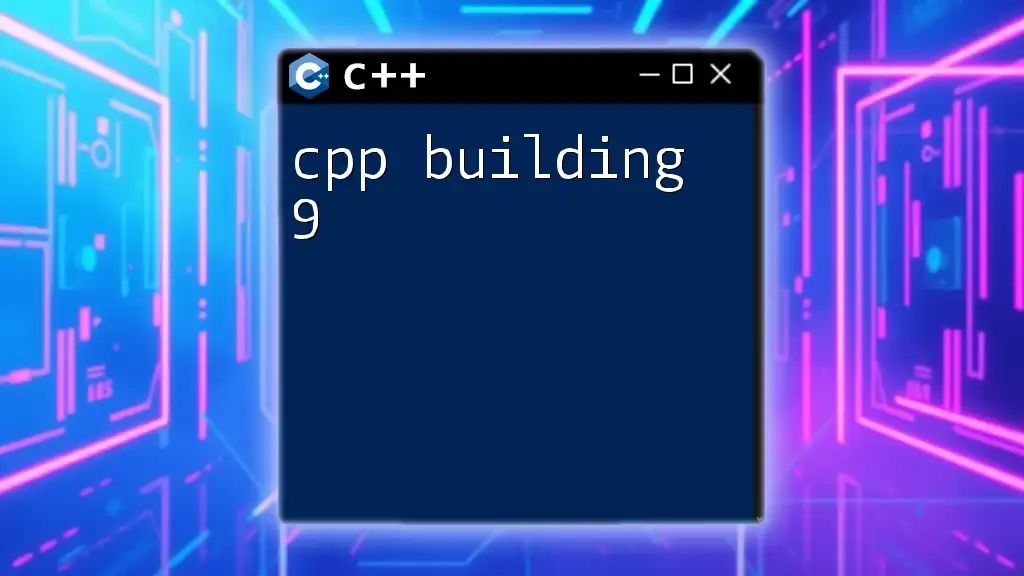
Building C++ Projects Using C++ Building 24
Setting Up Your Environment
Before embarking on building a C++ project, it is crucial to set up your environment. Key prerequisites include:
- C++ Compiler: Ensure you have a compiler installed, such as GCC or Clang.
- CMake: Download and install CMake to facilitate the build process.
- IDE or Editor: Use a suitable IDE (like Visual Studio, CLion) or any code editor (like VS Code) that suits your preferences.
Step-by-Step Guide to Building with C++ Building 24
Creating a New Project
To start a new C++ project, you need to create a proper directory structure. Here’s how you can set it up using command line on a Unix-like system:
mkdir MyCPPProject
cd MyCPPProject
mkdir src include
Writing Your First C++ Program
Now, let’s create a simple "Hello, World!" program in the `src` directory. Open `main.cpp` and add the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code introduces you to basic C++ syntax and forms a good starting point for more complex programs.
Compiling and Running Your Program
The next step is to compile and run your program using CMake. Here’s how to do it:
-
Create a build directory:
mkdir build cd build
-
Generate build files:
cmake ..
-
Compile the program:
make
-
Run the executable:
./MyExecutable
You should see "Hello, World!" printed in your terminal, showcasing that your program has been successfully built and executed.
Error Handling and Debugging Tips
Errors during the building process are common. Here are some strategies for addressing them:
- Reading Error Messages: CMake and the compiler provide informative error messages. Always read them carefully to understand what went wrong.
- Check Dependency Paths: Ensure that all your include paths and library links are correctly specified in your CMakeLists.txt.
- Debugging with gdb: The GNU Debugger (gdb) is a powerful tool for debugging C++ programs. Here’s how to use it:
gdb ./MyExecutable
Executing this command allows you to debug the program line by line, helping identify any runtime errors.
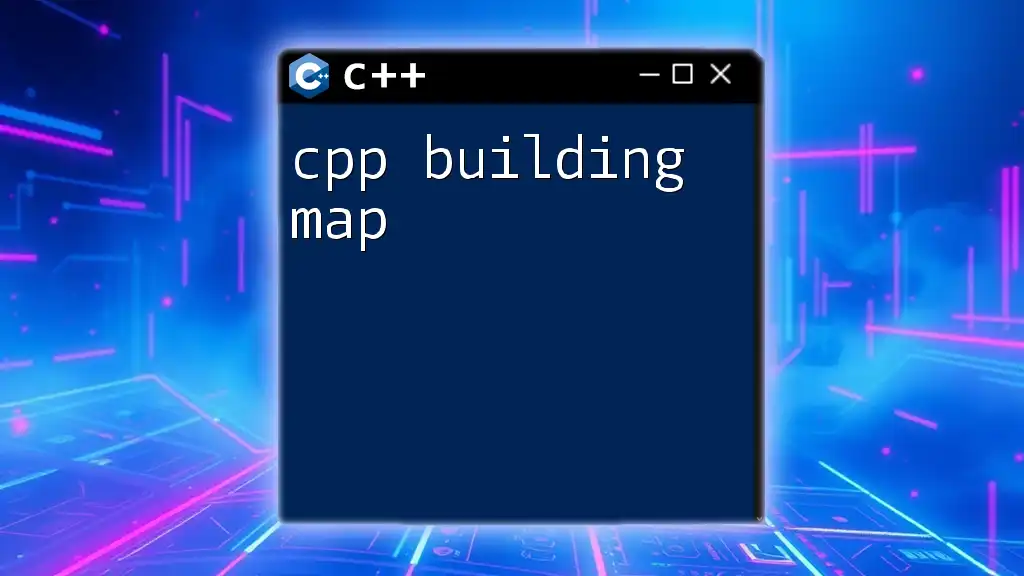
Optimizing Your Build Process
Techniques for Faster Builds
Efficiency is key in modern development. Here are techniques to enhance the speed of your builds:
- Precompiled Headers: By compiling header files once and reusing them, you can significantly reduce compile times.
- Avoid Unnecessary Recompilation: CMake is intelligent enough to track file dependencies. Ensure you structure your `CMakeLists.txt` in a way that only modified files are recompiled.
- Parallel Builds: Utilize multicore processors by running builds in parallel:
make -j$(nproc)
This uses all available cores to compile faster.
Enhancing Code Quality
Quality is as essential as speed. Implementing robust testing frameworks can catch bugs before they reach production. For instance, Google Test integrates seamlessly into C++ projects. Here’s a simple test example:
#include <gtest/gtest.h>
TEST(SampleTest, SimpleAssertion) {
EXPECT_EQ(1, 1);
}
Incorporating testing ensures your application remains stable and maintainable, following the principles of "CPP Building 24".
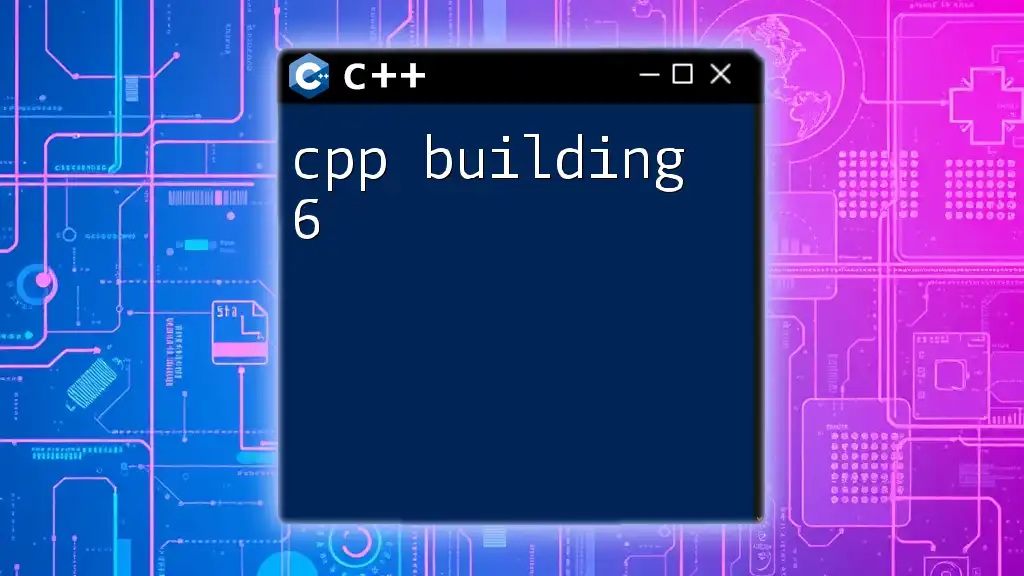
Conclusion
In conclusion, CPP Building 24 provides a framework for optimizing C++ builds, simplifying complex processes, and enhancing productivity. By adhering to the best practices outlined in this guide, developers can build their projects efficiently and maintain quality throughout the development lifecycle. Embrace these practices and see marked improvements in your C++ development experience!
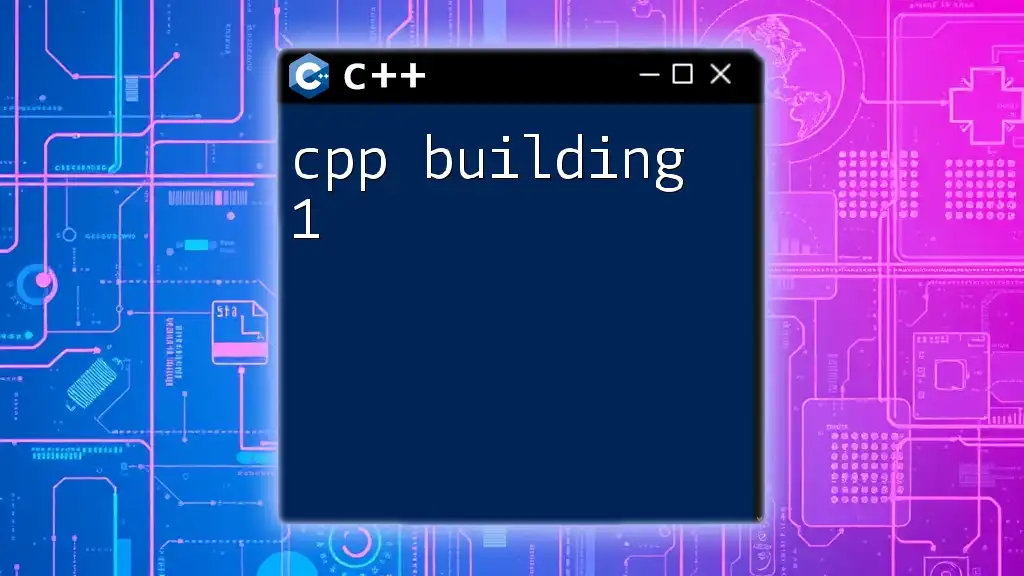
Additional Resources
For those looking to deepen their understanding, consider exploring the following resources:
- C++ Official Documentation: A comprehensive source for C++ language specifications.
- CMake Documentation: Details on building CMakeLists.
- Online Courses: Platforms like Udemy, Coursera, or edX offer in-depth courses on C++ programming. By continuously learning and applying these principles, you can ensure a successful journey in C++ development.