In "cpp building 162," we explore the efficient use of C++ commands to streamline coding processes, exemplified by a simple program that calculates the sum of two numbers.
#include <iostream>
int main() {
int a, b;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
std::cout << "Sum: " << (a + b) << std::endl;
return 0;
}
Understanding C++ Building Concepts
What is CPP?
CPP, short for C Preprocessor, is a powerful tool in the ecosystem of C and C++ programming languages. It plays an essential role in the initial stages of C++ program compilation by transforming code before it is actually compiled. By handling tasks such as file inclusion, macro substitution, and conditional compilation, C++ streamlines the development process, ensuring that programmers can manage larger and more complex codebases effectively.
Components of C++ Building
In a C++ building environment, several key components work together to create an executable program:
- Source Code Files: These are the .cpp files containing your actual C++ code, alongside header files (.h) that define your structures and functions.
- Makefiles: These files automate the build process by specifying how to compile and link the program.
- Build Tools: Tools such as g++, make, and IDEs which facilitate the compilation of the code and make the workflow more efficient.
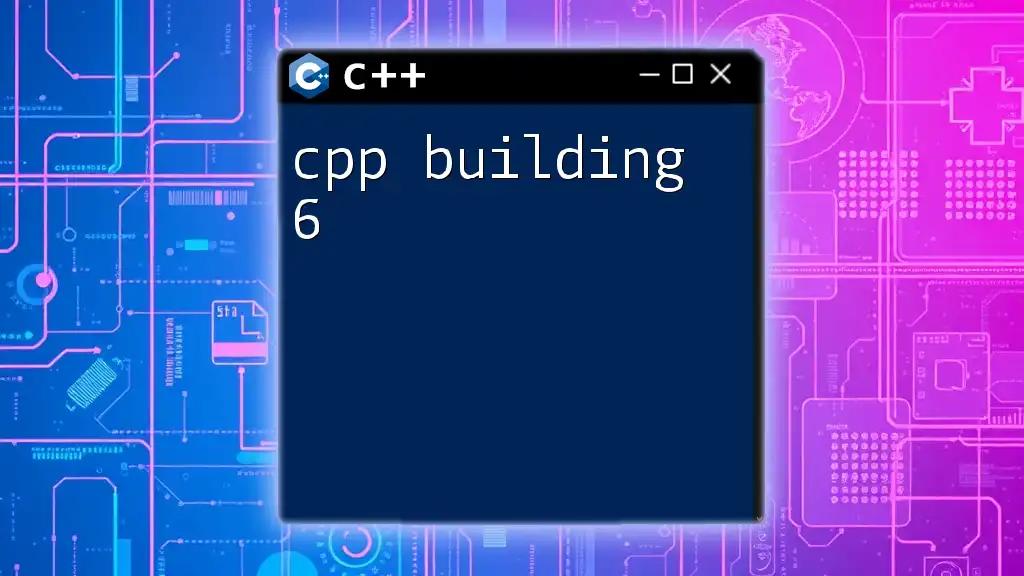
Getting Started with C++ Building 162
Setting Up Your Environment
To begin with C++ building, you need to set up your development environment. Here’s how:
-
Install an Integrated Development Environment (IDE): Popular choices include Visual Studio, Code::Blocks, and CLion. These IDEs come equipped with built-in tools for code editing, debugging, and compiling.
-
Compiler Installation: You can choose from several compilers such as GCC or Clang. Make sure to install the one that is compatible with your system and IDE.
-
Configuration Tips: After installation, configure your IDE to use the installed compiler. This may involve setting the path in environment variables or adjusting the IDE settings.
Creating Your First C++ Project
Initializing Your Project
Begin your first C++ project by establishing a clean directory structure. This means creating folders for your source files, headers, build outputs, and other resources. A recommended layout might look like this:
/MyCPPProject
/src
main.cpp
other.cpp
/include
other.h
/build
Sample Project: Hello, World!
Let's write a simple Hello World program to get our feet wet. Here’s the C++ code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet, we include the iostream library to enable input and output operations. The `main()` function serves as the entry point of the program, and `std::cout` is used to print "Hello, World!" to the console.
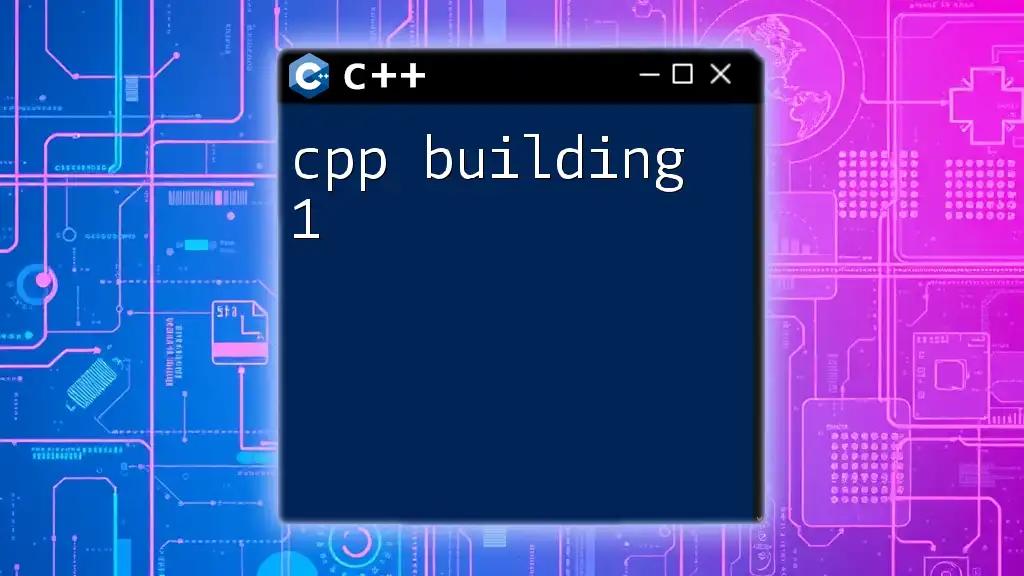
Building Your C++ Project
Understanding the Build Process
The process of building a C++ project involves three critical stages:
- Preprocessing: This is where C++ directives are processed. It handles file inclusion and macro expansions.
- Compilation: The preprocessed code is converted into object code. This includes syntax checking and converting the high-level code into machine-readable format.
- Linking: Finally, the object code is linked with libraries and other object files to produce the final executable.
Using Makefiles for Build Automation
What is a Makefile?
A Makefile is an essential script that facilitates the automation of your build process. It specifically outlines how to compile and link the program, making development much more efficient, especially for larger projects.
Creating a Basic Makefile
Here’s a simple example of a Makefile for your project:
CC=g++
CFLAGS=-I.
all: my_program
my_program: main.o other.o
$(CC) -o my_program main.o other.o
main.o: main.cpp
$(CC) -c main.cpp
other.o: other.cpp
$(CC) -c other.cpp
clean:
rm -f *.o my_program
In this Makefile:
- `CC` sets the compiler variable.
- `CFLAGS` specifies compiler flags.
- Each target specifies how to compile or link components of the project. Using the command `make`, you can automate the build process with ease.
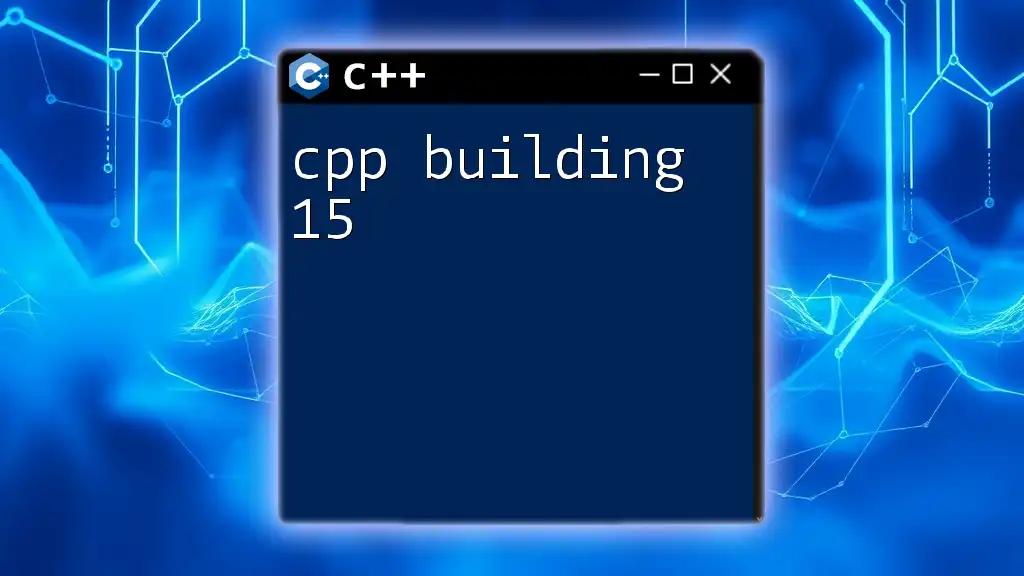
Advanced C++ Building Techniques
Debugging and Optimizing Builds
Common Errors in C++ Building
As you build projects, you will encounter various errors. Common errors include syntax errors, missing files, and linking errors. Learning to read error messages and knowing how to troubleshoot them is crucial. For instance, a common compilation error might read:
error: 'cout' was not declared in this scope
This often indicates that the iostream library was not included correctly. By addressing the issue and ensuring necessary headers are included, you can prevent such errors.
Using Debugger Tools
To streamline your development process, utilizing debugger tools like GDB can be invaluable. Debuggers allow you to step through your code line by line, inspect variables, and control execution flow.
Leveraging Build Systems
Introduction to CMake
For larger projects or when collaborating with multiple developers, using a build system like CMake offers significant advantages. CMake is a cross-platform tool that automatically generates the build configuration for your projects.
Basic CMake Example
Here’s a simple example of a `CMakeLists.txt` file:
cmake_minimum_required(VERSION 3.10)
project(MyCPPProject)
add_executable(my_program main.cpp other.cpp)
This script specifies the minimum required version for CMake, sets the project name, and defines the executable to be built from the specified source files. CMake simplifies your building process by abstracting away many of the complexities of using traditional build tools.

Conclusion
By mastering cpp building 162, you stand to enhance your software development skills significantly. This comprehensive guide has walked you through setting up your environment, creating projects, and understanding both the basics and advanced techniques that streamline the building process. The ability to efficiently build and manage C++ projects is not just convenient; it's a fundamental skill that every C++ programmer should develop.
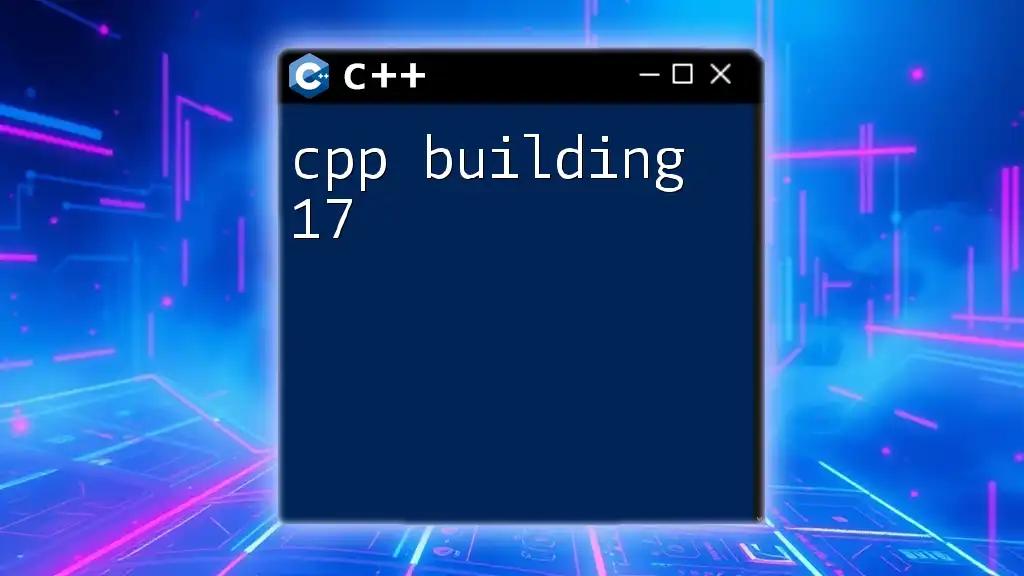
Additional Resources
For further exploration, consider delving into C++ documentation, tutorials, and community forums. Numerous books and online courses are available to deepen your knowledge, guiding you toward becoming proficient in C++ development.

Call to Action
If you're eager to enhance your skills and dive deeper into the world of C++ commands and building techniques, consider joining our courses where you can receive hands-on training and personalized support!