CPP tutoring focuses on providing efficient and concise instruction for mastering C++ commands, enabling learners to quickly grasp essential concepts for programming success.
Here's a simple example of using a C++ command to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Tutoring
What is C++ Tutoring?
C++ tutoring is a specialized form of education focused on teaching individuals how to effectively use the C++ programming language. With a personalized approach, C++ tutors aim to cater to the unique learning needs of each student. The significance of personalized learning cannot be overstated; it allows students to progress at their own pace and tackle areas of difficulty one-on-one with an expert.
In today’s technology-driven landscape, learning C++ is highly beneficial. The language is known for its efficiency and performance, making it a preferred choice for applications in gaming, systems programming, applications requiring high performance, and even in domains like machine learning and artificial intelligence.
Who Can Benefit from C++ Tutoring?
Numerous individuals can derive immense value from C++ tutoring, including:
- Beginners: Those who are new to programming can find C++ an excellent starting point to grasp fundamental programming concepts.
- Intermediate Programmers: Individuals who possess basic programming skills can enhance their knowledge and tackle more complex projects.
- Professionals: Those looking to transition into software development or enhance their current skill set will find C++ tutoring invaluable.
- Students: Learners preparing for exams, coding interviews, or academic projects can significantly benefit from focused tutoring sessions.
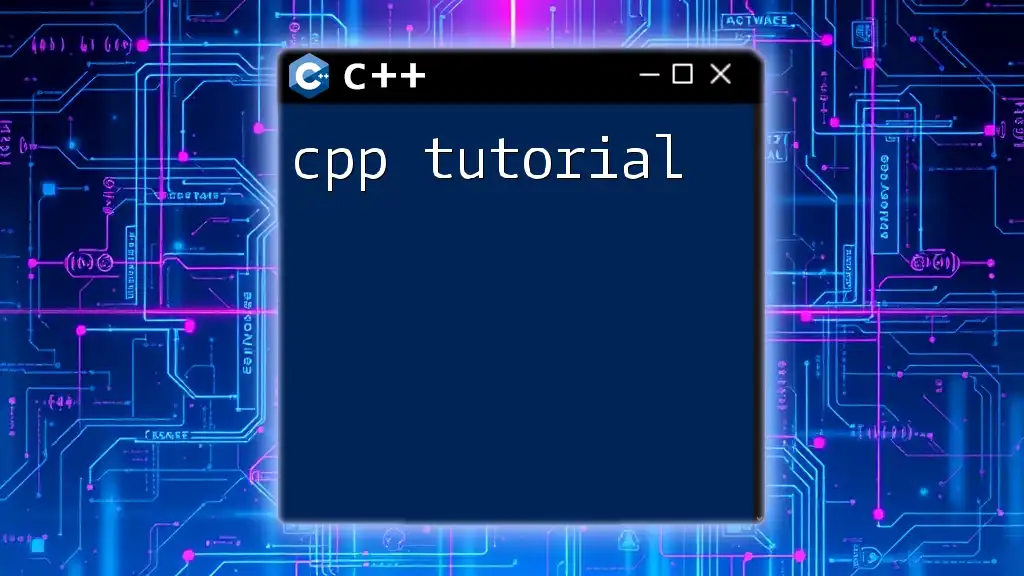
The Structure of Effective C++ Tutoring
One-on-One Tutoring vs Group Sessions
When it comes to C++ tutoring, one-on-one sessions allow for a tailored educational experience where the tutor can focus solely on the student's needs, adjusting the pace and content as necessary. This personalized approach helps in building confidence and deeper understanding.
Group sessions can also be beneficial, fostering a collaborative learning environment. They provide opportunities for students to learn from each other and tackle challenges through discussion and teamwork. However, they may not be ideal for everyone, particularly those needing more individualized attention.
Online Tutoring Platforms
There are numerous platforms available that specialize in online tutoring for C++. These platforms can create a flexible and accessible environment for students. Advantages include the ability to select tutors based on specific needs, schedule lessons at convenient times, and often access a plethora of resources.
Selecting the right platform is essential. Look for one that offers comprehensive support, allows for direct communication with tutors, and includes interactive tools like code sharing and screen sharing for enhanced collaboration.
Tailoring Learning Paths
Assessing individual learning styles is vital in C++ tutoring. Some students may grasp concepts better through visual aids, while others might learn better through hands-on practice. Building a custom syllabus that aligns with these styles will foster a productive learning environment.
Setting clear, achievable goals is also crucial. This not only maintains motivation but also allows for the measurement of progress, which can be highly rewarding and encouraging for students.
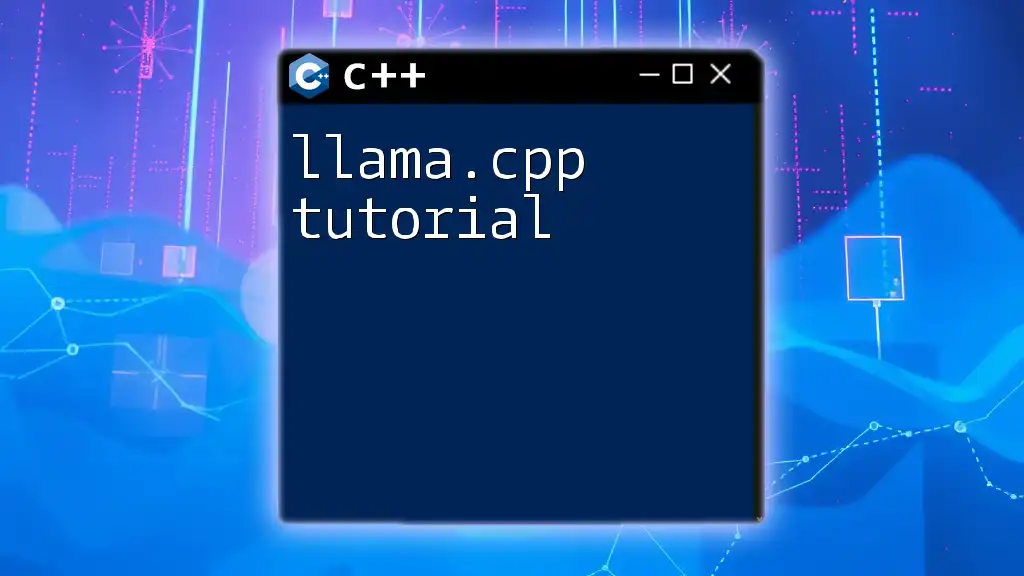
Core C++ Concepts to Cover in Tutoring
Fundamentals of C++
Introduction to C++ Syntax
Every C++ program begins with the essential structure of the language. Understanding the basic components is crucial. For instance, consider the classic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet, `#include <iostream>` is a preprocessor directive that includes the input-output stream library, allowing the use of `std::cout`. The `main` function is the entry point of the program, and `std::endl` is used to insert a new line. Understanding this syntax forms the foundation for further learning.
Variables and Data Types
C++ offers several built-in data types, including integers, doubles, characters, etc. Variables serve as containers for these data types. For example, declaring and initializing variables can be illustrated as follows:
int age = 25;
double salary = 50000.50;
These examples demonstrate how variables store information. Understanding data types enhances programming practices by enabling programmers to make informed decisions about memory usage and program efficiency.
Control Statements
Conditional Statements (if-else, switch)
Conditional statements allow for dynamic decision-making in programs. The `if-else` structure provides the means to execute different code based on specific conditions. Here’s a simple application of this concept:
int number = 10;
if (number > 0) {
std::cout << "Positive" << std::endl;
}
The flow control of such statements empowers programmers to write code that adapts to various situations.
Loops (for, while, do-while)
Loops facilitate the execution of code multiple times, which is fundamental in programming. Each loop type serves a specific purpose. A `for` loop is used when the number of iterations is known ahead of time:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
Understanding when to use each type of loop is critical for efficient programming practices.
Functions and Scope
Creating and Using Functions
Functions encapsulate code blocks for reuse, improving code organization. A simple function is outlined below:
int add(int a, int b) {
return a + b;
}
This function accepts two integer parameters and returns their sum. Understanding functions leads to cleaner, more maintainable code.
Scope and Lifetime of Variables
Variables can exist in different scopes, affecting their visibility and lifetime. Local variables are accessible within the function they are declared in, while global variables can be accessed site-wide. Here’s an example:
int globalVar = 10;
void display() {
int localVar = 5;
std::cout << globalVar << ", " << localVar << std::endl;
}
Recognizing the importance of scope helps prevent variable shadowing and unintended behavior in programs.
Object-Oriented Programming (OOP) in C++
Basics of OOP Concepts
C++ is an object-oriented programming language, meaning it is built around the concepts of encapsulation, inheritance, and polymorphism. These principles promote modularity and reusability. Here’s a brief illustration of a class:
class Car {
public:
std::string brand;
int year;
void display() {
std::cout << brand << " " << year << std::endl;
}
};
Understanding how to define and instantiate classes forms the backbone of advanced C++ programming.
Working with Classes and Objects
Instantiating a class and accessing its methods is crucial for utilizing OOP principles. Here’s how to work with the `Car` class defined earlier:
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2022;
myCar.display();
This example demonstrates object instantiation and method calls, which are foundational in designing robust, object-oriented applications.
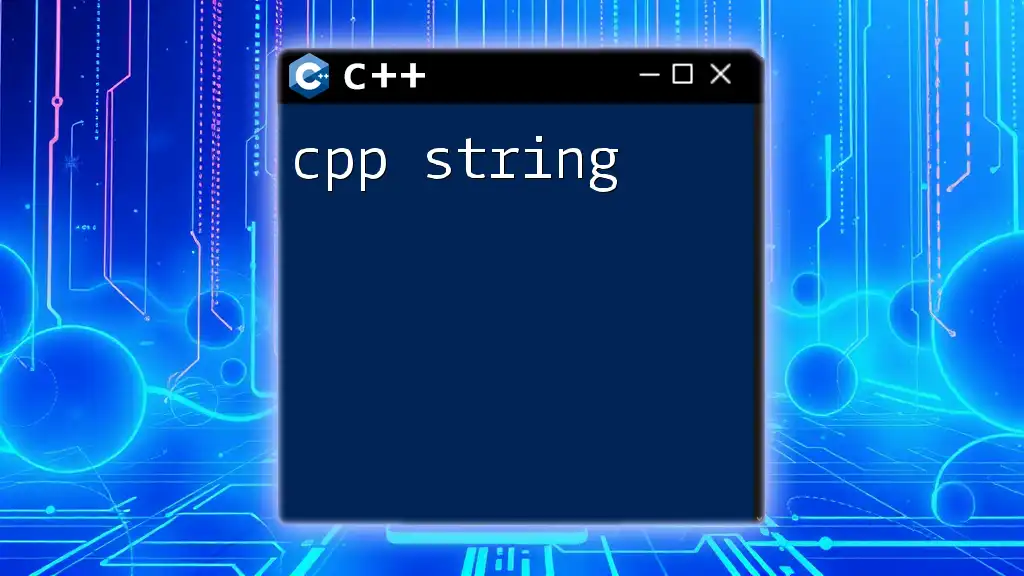
Enhancing C++ Tutoring with Practical Exercises
Coding Challenges for Different Skill Levels
Incorporating diverse coding challenges into C++ tutoring can significantly enhance learning. For beginners, simple tasks like printing numbers or basic algorithms work well. Intermediate challenges can involve manipulating data structures or solving logic puzzles. Resources like LeetCode and HackerRank provide a plethora of problems catering to various skill levels.
Projects to Reinforce Learning
Hands-on projects (such as building a calculator, a simple game, or a personal diary application) enable students to apply their knowledge, reinforcing what they’ve learned in a practical context. Real-world applications of programming concepts solidify understanding and improve retention.
Frequent Mistakes to Avoid
As students learn C++, they often encounter common hurdles. Typical pitfalls include misunderstanding pointers, syntax errors, and poor memory management. Educating students on how to effectively troubleshoot and debug their code enhances their problem-solving capabilities, making them more proficient programmers.
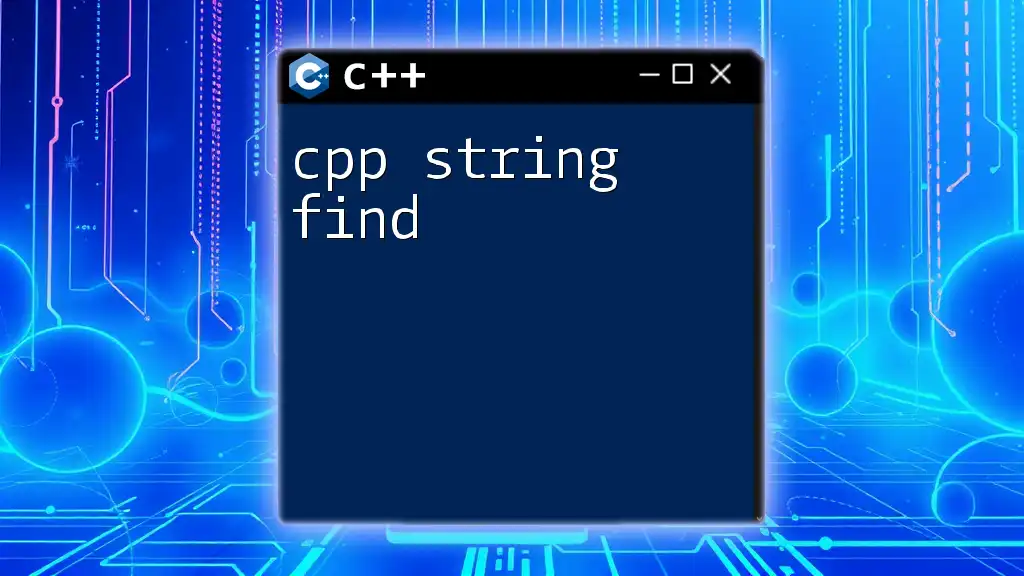
Evaluating Progress in C++ Tutoring
Setting Milestones and Goals
Establishing defined milestones helps students stay motivated and focused. By breaking their journey into smaller, achievable segments, they can monitor their progress and celebrate small victories along the way.
Feedback Mechanisms
Providing immediate and constructive feedback is essential. It allows students to identify areas for improvement. Encouraging self-reflection at the end of each session can enable learners to recognize their strengths and weaknesses independently, fostering a growth mindset.
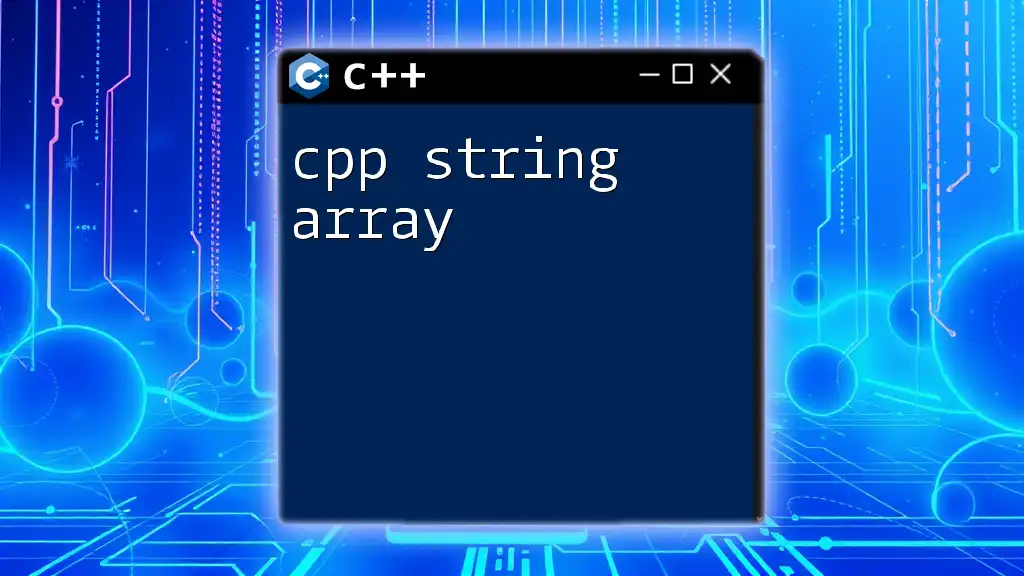
Conclusion
The Future of C++ Learning
Learning C++ cannot only advance one's programming skills but also open doors to numerous career opportunities in software development, data science, and more. The principles of C++ remain relevant as technology evolves, making it a valuable investment for future professionals.
Call to Action
If you are ready to embark on your C++ learning journey, consider enrolling in a tutoring program. Personalized C++ classes can provide the knowledge and support you need to excel in this vital programming language.
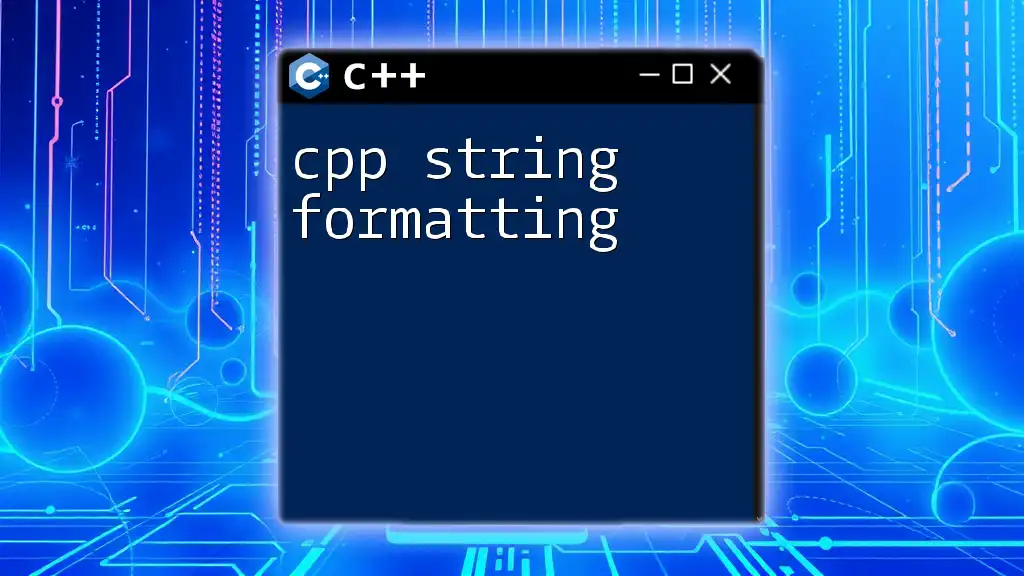
Resources for Further Learning
To continue your exploration of C++, consider diving into recommended books such as C++ Primer or online resources. Engaging with programming communities or forums can also provide valuable insights and foster connections with fellow learners.