In C++, a string is a sequence of characters used to store and manipulate text, and it is typically represented using the `std::string` class from the `<string>` library.
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
What is a String in C++?
A string in C++ is a sequence of characters used to represent text. It allows programmers to handle, manipulate, and modify textual data effectively. Unlike arrays of characters in C, which are static and can lead to memory issues, C++ introduces the `std::string` class that offers dynamic memory allocation and a robust set of functionalities.
Differences Between C-style Strings and C++ Strings
C-style strings are arrays of characters terminated by a null character (`\0`). They require manual memory management and string manipulation can be error-prone. On the other hand, C++ strings encapsulate character data in an object, providing safer features such as automatic memory handling, comprehensive manipulative functions, and ease of usage.
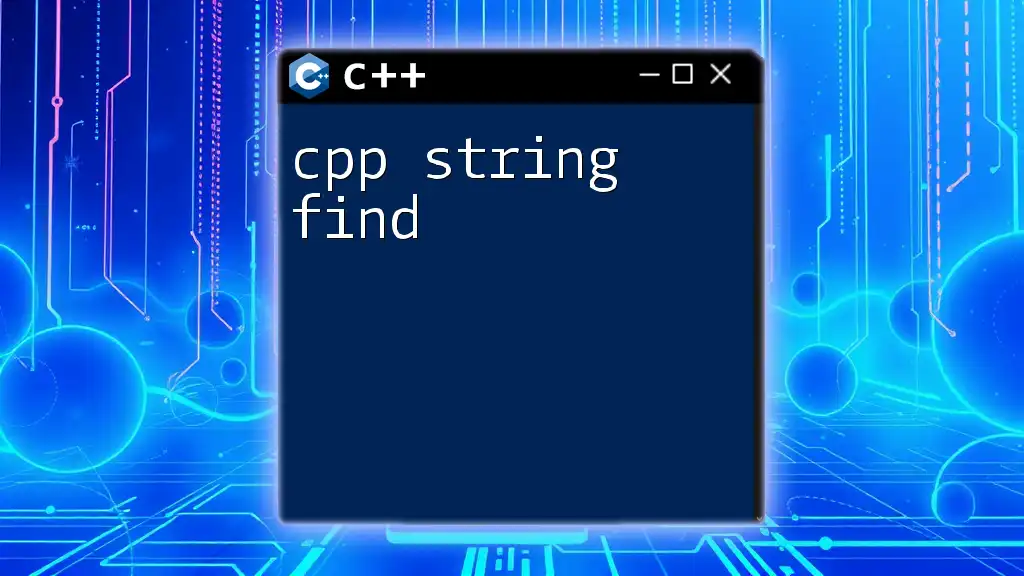
The C++ String Class
The `std::string` class, included in the `<string>` header, serves as the foundational type for storing and managing strings in C++. It brings inherent features such as dynamic sizing and rich functionality which facilitates various string operations.
Key Features of the String Class in C++
- Dynamic Sizing: The size of a `std::string` can automatically adjust, alleviating the need for manual memory management.
- Built-in Functions: C++ strings come with numerous member functions to manipulate text conveniently, such as concatenation, comparison, and search.
- Compatibility with STL: The `std::string` class integrates effectively with other components of the Standard Template Library (STL).
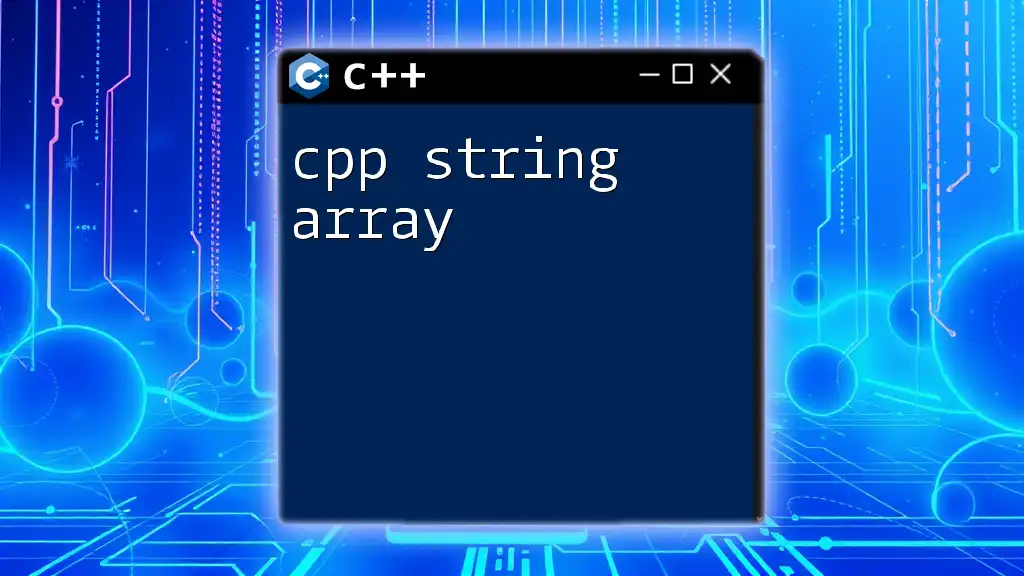
Creating Strings in C++
Declaring and Initializing Strings
Creating a string in C++ is straightforward:
std::string greeting = "Hello, World!";
This simple declaration initializes a `std::string` object with the value "Hello, World!".
Copying and Assigning Strings
C++ strings support straightforward assignment and copying, ensuring that string manipulation is simple and intuitive:
std::string str1 = "Example";
std::string str2 = str1; // Copy Constructor
In this example, `str2` becomes a copy of `str1`, and both strings exist independently in memory, thus preventing unintentional data corruption.
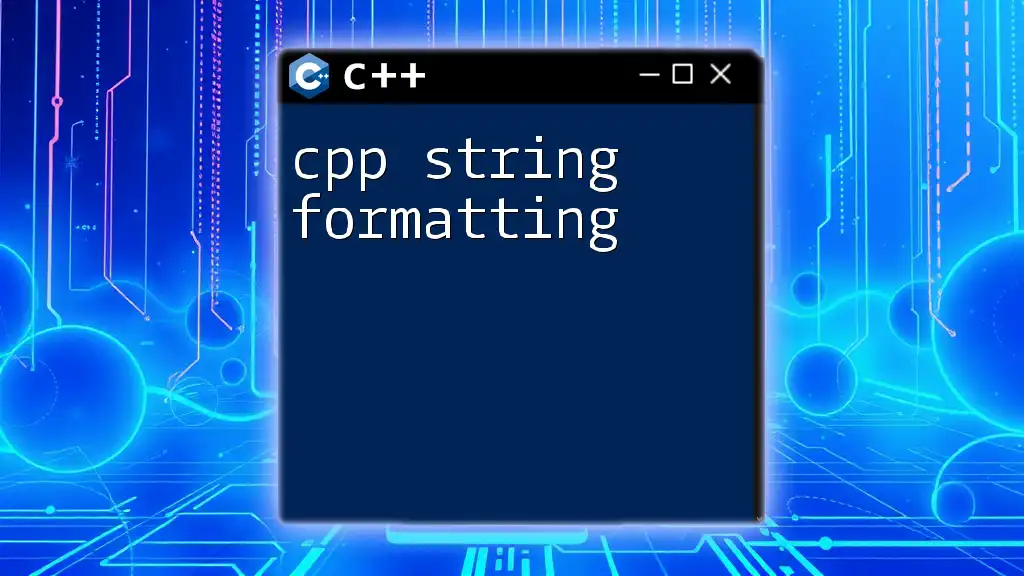
Common String Operations
Concatenation of Strings
Concatenation joins two or more strings into one. C++ provides the `+` operator for this purpose:
std::string first = "Hello, ";
std::string last = "World!";
std::string full = first + last; // Concatenation
With this syntax, `full` now holds "Hello, World!".
Finding Length of a String
The `length()` member function retrieves the number of characters in a string:
std::string name = "Programming";
std::cout << name.length(); // Outputs: 11
This feature is essential when validating user input or performing operations that require exact string sizes.
Accessing Characters in a String
You can access individual characters using the index operator `[]`:
char firstChar = name[0]; // Accessing the first character
This shows how to retrieve characters by their position, enhancing text processing capabilities.
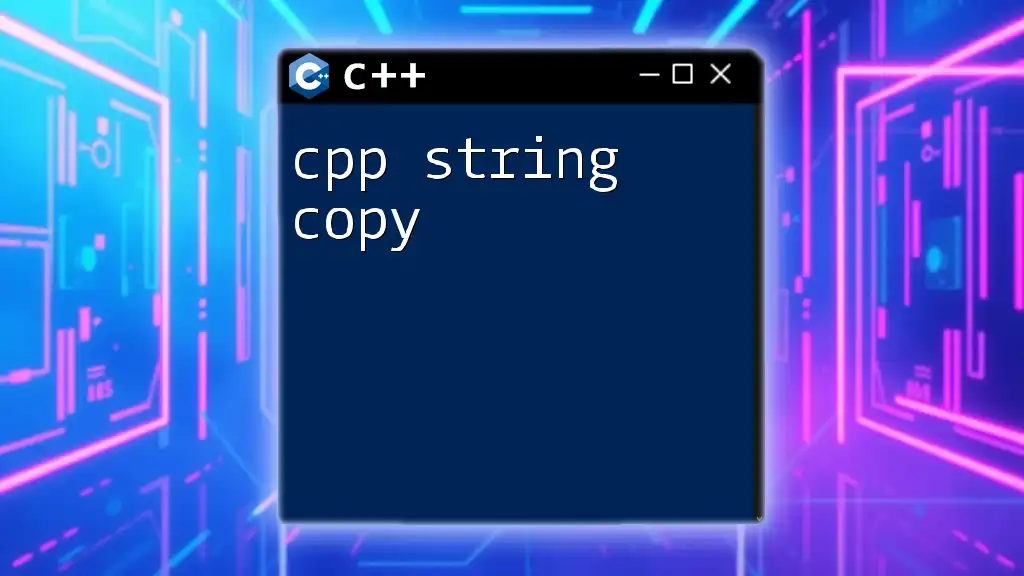
Modifying Strings
Inserting and Erasing Characters
The `insert()` method allows you to add characters at a specific position:
std::string message = "Hello!";
message.insert(5, " C++"); // "Hello C++!"
Conversely, the `erase()` method removes characters from a string:
message.erase(5, 4); // "Hello!"
These functions provide powerful tools for dynamically adjusting string content.
Replacing Substrings
The `replace()` method allows modification of part of a string:
message.replace(0, 5, "Goodbye"); // "Goodbye!"
Manipulating substrings in this manner opens up numerous possibilities for text editing.
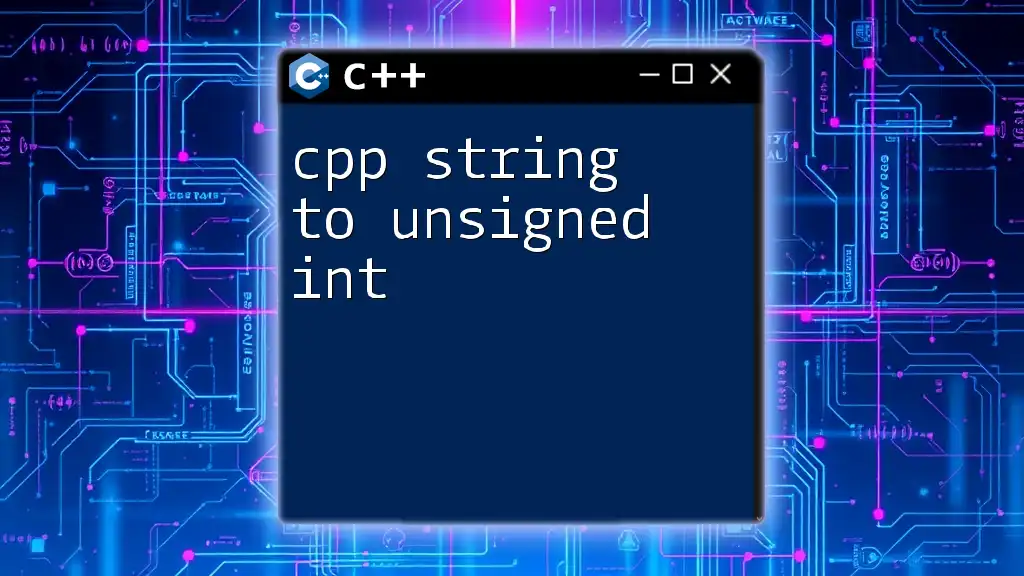
String Comparison
Comparing strings is effortless in C++. You can determine equality or lexicographical order using relational operators:
std::string strA = "Apple";
std::string strB = "Banana";
bool result = (strA == strB); // false
Such comparisons enable functionalities such as sorting and searching within textual data.
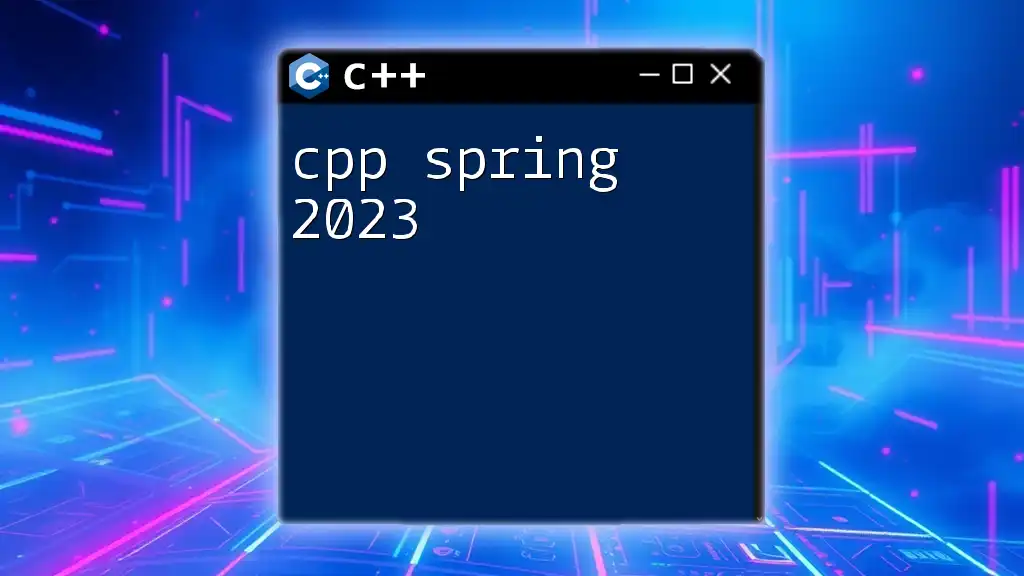
String Conversion
Converting Between C-style Strings and C++ Strings
In C++, converting between C-style strings and `std::string` is simple:
const char* cstr = "Hello, World!";
std::string cppStr = std::string(cstr); // Conversion to C++ String
This conversion ensures you can use C-style strings without sacrificing the benefits of the C++ string class.
Numeric to String and String to Numeric Conversion
C++ provides functions like `std::to_string()` and `std::stoi()` for numeric conversions:
int num = 42;
std::string numStr = std::to_string(num);
int convertedNum = std::stoi(numStr);
This functionality is very useful when processing numeric input and output as strings.
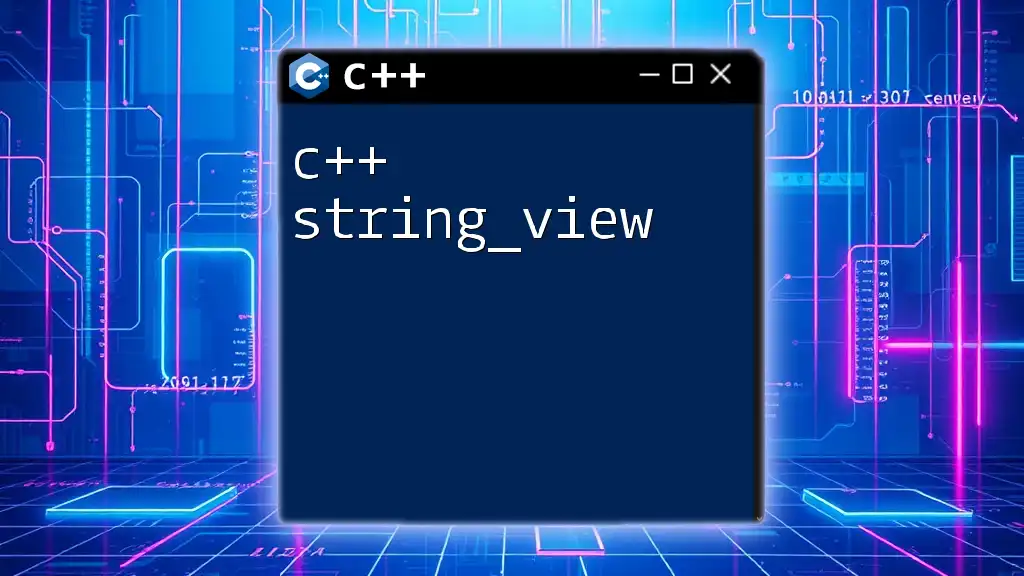
String Operations with Standard Algorithms
The compatibility of `std::string` with standard algorithms allows you to leverage a multitude of STL functions to manipulate strings. For example, you can use `std::sort` with strings just as easily as with other containers.
std::string data = "bacd";
std::sort(data.begin(), data.end()); // Sorts characters in ascending order
Utilizing standard algorithms enhances the efficiency and readability of your code.
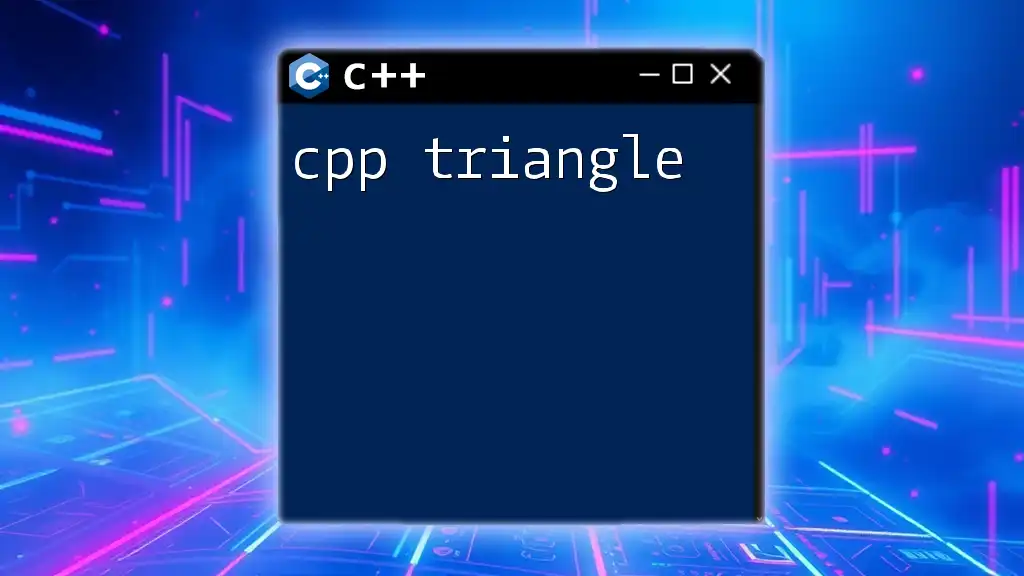
String Manipulation Best Practices
When working with C++ strings, it is crucial to adopt efficient usage patterns. Consider the following recommendations:
- Minimize Copies: Be aware when passing strings to functions. Use references to avoid unnecessary copies, e.g., `void processString(const std::string& str)`.
- Use `std::move()` Wisely: In newer C++ standards, leveraging `std::move()` can optimize string transfers when returning from functions.
Understanding string performance is also vital. For applications where performance is paramount, analyze the difference between using `std::string` and simpler C-style strings, particularly in high-performance or resource-constrained environments.
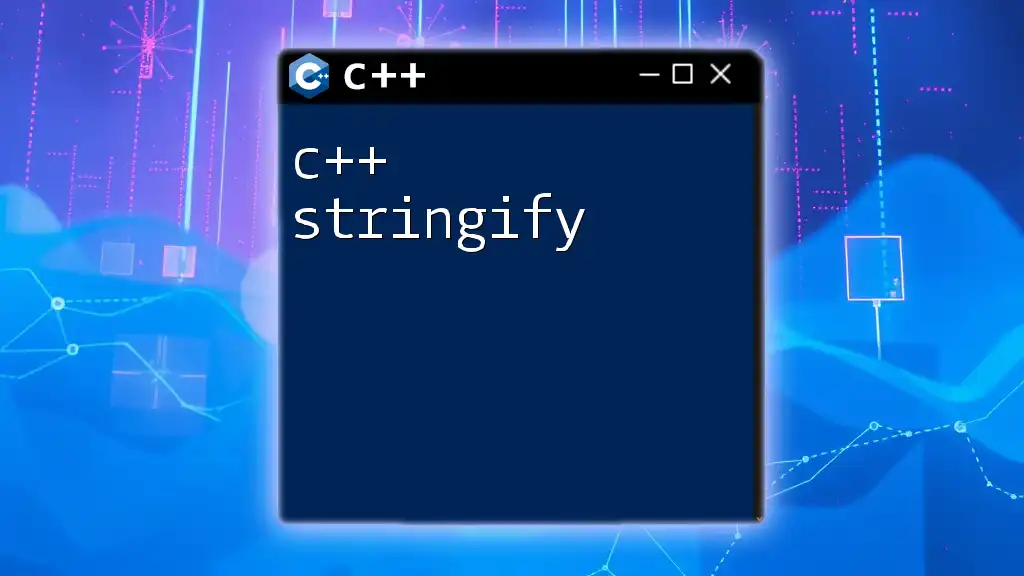
Conclusion
As you can see, working with a cpp string is a fundamental skill in C++. Mastering these concepts and operations will empower you to tackle myriad text-processing tasks efficiently. By leveraging string manipulation capabilities and best practices, you become a more proficient C++ programmer, well-equipped to handle various programming challenges.
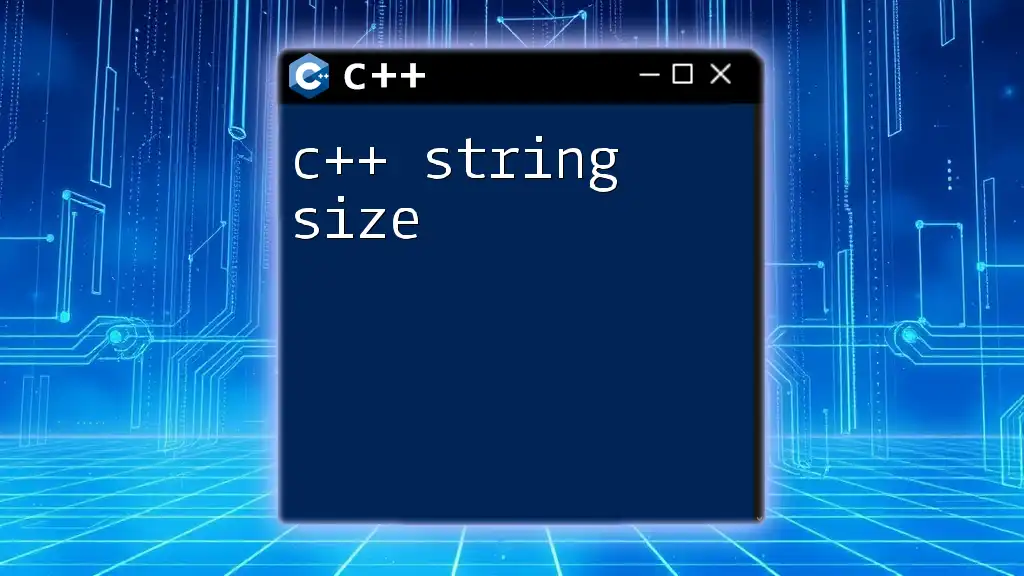
Additional Resources
To deepen your understanding of C++ strings, explore further reading, tutorials, and official documentation that delve deeper into their functionalities and use cases.
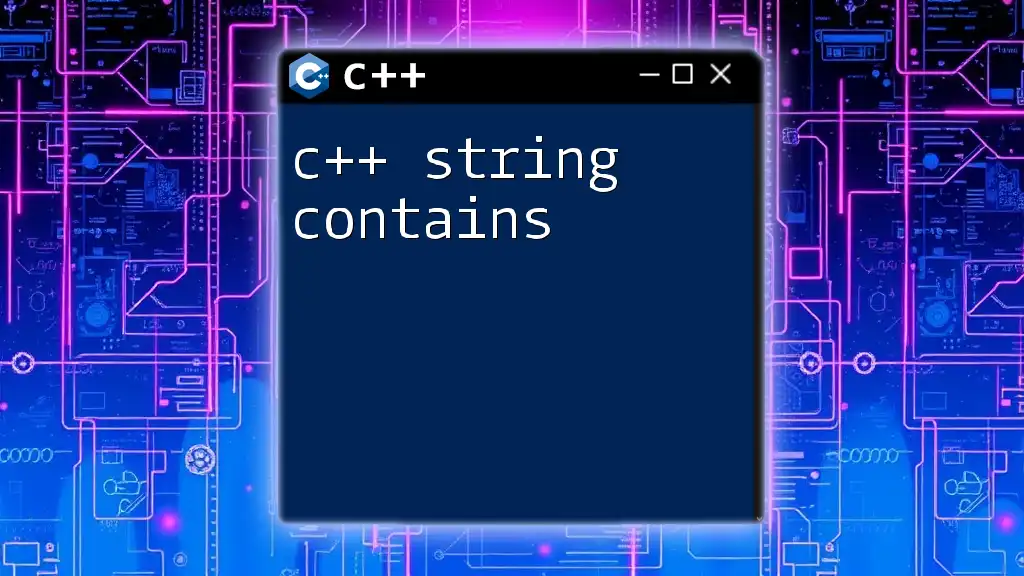
Call to Action
Challenge yourself with practical exercises in C++ string manipulation! Apply these concepts in your projects to enhance your programming capability and understanding of C++.