The `cpp` command for copying a string involves using the `strcpy` function from the C standard library to duplicate the contents of one string into another.
Here's an example in C++:
#include <iostream>
#include <cstring>
int main() {
char source[] = "Hello, World!";
char destination[50];
strcpy(destination, source);
std::cout << "Copied String: " << destination << std::endl;
return 0;
}
Understanding C++ Strings
What are C++ Strings?
In C++, strings are used to store and manipulate sequences of characters. C++ provides two main types of strings: C-style strings and C++ `std::string`.
-
C-style strings are essentially arrays of characters terminated by a null character (`\0`). They require careful memory management and handling, as failure to properly manage the null terminators can lead to undefined behavior.
-
C++ `std::string`, on the other hand, is a more sophisticated string handling class that provides several built-in functionalities, such as automatic memory management, concatenation, and easy resizing. This makes `std::string` a far more robust and user-friendly option compared to C-style strings.
The benefits of using `std::string` include:
- Built-in safety and convenience, such as automatic resizing.
- Rich functions that simplify common operations (e.g., substring, find, and replace).
Why String Copying is Important
String copying is a fundamental operation in programming, particularly in scenarios where you need to manipulate or duplicate text data without altering the original. Some common real-world tasks include:
- User input handling: Often, input needs to be processed without modifying the original input.
- Data manipulation: When working with large datasets, creating copies can prevent unintended side effects when modifying strings.
- File and data storage: Strings often have to be copied for logging or saving user data.
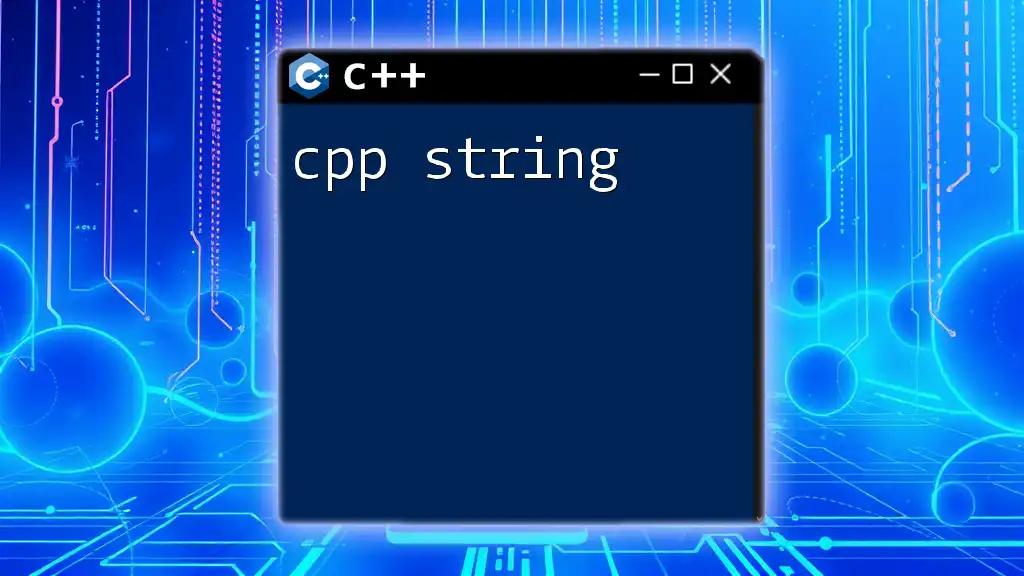
C++ String Copy Methods
Using `std::string::copy()`
The `std::string::copy()` function is designed for copying a part of a string into a character array. This approach allows for finer control over how much of the string is copied and where it’s stored.
Syntax:
string& copy(char* s, size_type n, size_type pos = 0) const;
- `s`: Pointer to the destination character array.
- `n`: Number of characters to copy.
- `pos`: The starting position in the string (default is 0).
Code Example:
#include <iostream>
#include <string>
int main() {
std::string original = "Hello, World!";
char buffer[50];
original.copy(buffer, 13); // Copy first 13 characters
buffer[13] = '\0'; // Null-terminate the copied string
std::cout << buffer << std::endl; // Output: Hello, World!
return 0;
}
In this example, the `copy()` method is used to transfer a specified number of characters to a buffer. Remember to null-terminate the buffer to avoid undefined behavior when it is read.
Using Assignment Operator
C++ provides a straightforward method for string copying using the assignment operator. This is the most common and easiest way to copy strings in C++.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string original = "Hello, World!";
std::string copied = original; // Using assignment operator
std::cout << copied << std::endl; // Output: Hello, World!
return 0;
}
When using the assignment operator, the entire string is copied seamlessly. This method is highly recommended for simplicity and readability.
Using the `std::string` Constructor
Another effective way to copy strings is via the constructor of the `std::string` class. This approach is both clear and practical, especially when initializing a new string variable.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string original = "Hello, World!";
std::string copied(original); // Using constructor
std::cout << copied << std::endl; // Output: Hello, World!
return 0;
}
This constructor-based copying is typically both efficient and expressive. It’s a great way to create a copy while indicating the intent clearly.
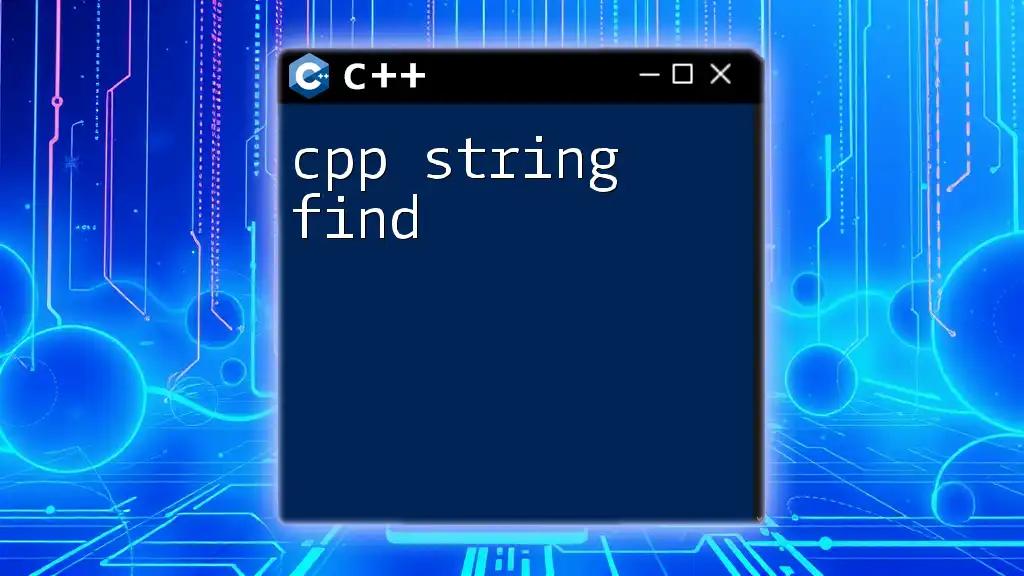
Special Cases in String Copying
Copying Strings with Substr
The `std::string::substr()` function allows you to create a substring, effectively copying just a portion of the original string. This can be particularly useful for handling specific segments of larger strings.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string original = "Hello, World!";
std::string partialCopy = original.substr(0, 5); // Copy the first 5 characters
std::cout << partialCopy << std::endl; // Output: Hello
return 0;
}
In this example, the `substr()` method extracts and creates a new string containing only "Hello". Use cases for partial string copying may include file processing, user commands, and data cleaning.
Dealing with Dynamic Memory
C++ also enables dynamic memory management, necessary to handle strings that may be created during runtime. When copying strings that reside in dynamically allocated memory, it's crucial to manage memory carefully to avoid memory leaks.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string *original = new std::string("Hello, World!");
std::string copied = *original; // Copying pointed string
std::cout << copied << std::endl; // Output: Hello, World!
delete original; // Prevent memory leak
return 0;
}
It’s essential to always free dynamically allocated memory when it's no longer needed to prevent memory leaks. This is fundamental to writing efficient, reliable C++ programs.

Common Mistakes in String Copying
Forgetting Null Terminators
One frequent mistake when working with C-style strings is overlooking null terminators. Without proper null-termination, functions expecting a string may read beyond the intended memory space, resulting in undefined behavior.
Code Snippet to Avoid:
char buffer[10];
std::string myString = "Hello!";
myString.copy(buffer, myString.size()); // Forgetting null-termination
To prevent such issues, always ensure you null-terminate your buffers. It is integral to maintain your program's stability and reliability.
Copying Large Strings
Performance can be impacted when copying large strings. Using move semantics helps reduce unnecessary memory allocation and copy overhead.
Short Example:
std::string largeString(1000000, 'A');
std::string copiedString = std::move(largeString); // Using std::move for efficiency
In this example, `std::move` efficiently transfers ownership of `largeString` to `copiedString`, minimizing performance costs associated with deep copying.
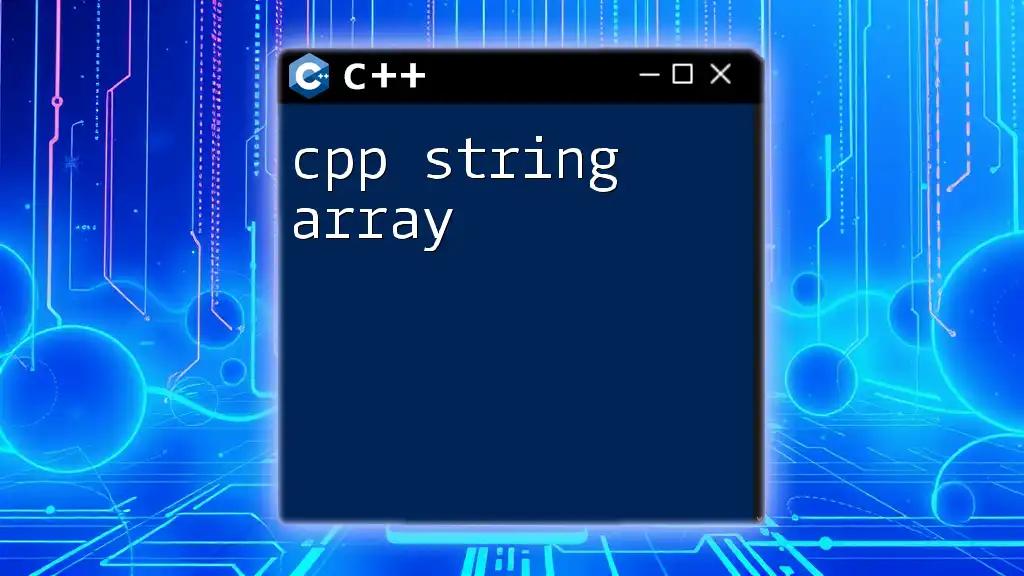
Conclusion
In summary, understanding how to perform a cpp string copy is crucial for effective string management in C++. Utilizing various methods such as `std::string::copy()`, assignment operators, or constructors allows developers to manipulate strings confidently and efficiently. Mastering these techniques will serve you well as you tackle more complex programming tasks involving string handling. Make sure to practice these methods and understand their implications in your code for cleaner and more efficient programming.

Additional Resources
For further reading and exploration, consider checking the official C++ documentation, enrolling in online C++ courses, and participating in community forums. These resources will provide you with valuable insights and assist you in your learning journey.

Frequently Asked Questions (FAQs)
What is the difference between `std::string::copy()` and the assignment operator?
- The `std::string::copy()` function is used to copy parts of a `std::string` into a character array, while the assignment operator creates a new `std::string` that is a copy of an existing one.
How can I copy a string without extra memory allocation?
- Using the move semantics with `std::move` allows you to transfer ownership of a string without incurring the cost of copying data.
Are there performance differences between copying methods?
- Yes, using the assignment operator or move semantics is generally more efficient than manual copying, especially for large strings. Always consider the context of your operations when choosing a copying method.