In C++, you can convert a `std::string` to an `unsigned int` using the `std::stoul` function, which stands for "string to unsigned long" and is designed for this purpose.
Here's a code snippet demonstrating this conversion:
#include <string>
#include <iostream>
int main() {
std::string strNumber = "1234";
unsigned int number = std::stoul(strNumber);
std::cout << "The unsigned int is: " << number << std::endl;
return 0;
}
Understanding the Basics of C++ Strings and Unsigned Integers
What is a C++ String?
In C++, a string is a sequence of characters that is used to represent text. Strings are represented as objects of the `std::string` class, which is part of the C++ Standard Library. Unlike C-style strings (character arrays), `std::string` provides numerous utility functions that make handling strings much easier, such as concatenation, substring extraction, and searching.
Common use cases for strings in programming include:
- User input for forms or command-line interfaces.
- Data storage and manipulation from files or databases.
- Networking applications where textual data is exchanged.
What is an Unsigned Integer?
An unsigned integer is a data type that can store non-negative whole numbers only. Unlike signed integers, which can represent both positive and negative values, unsigned integers range from 0 to a maximum positive value determined by the number of bits used. This makes unsigned integers particularly useful in applications where only non-negative values are needed.
Differences between signed and unsigned integers:
- Signed integers can store negative values, while unsigned integers cannot.
- Unsigned integers have a larger positive range for the same number of bits.
Use cases for unsigned integers include:
- Counting items where negative counts do not make sense.
- Indices for array access.
- Bit manipulation tasks.
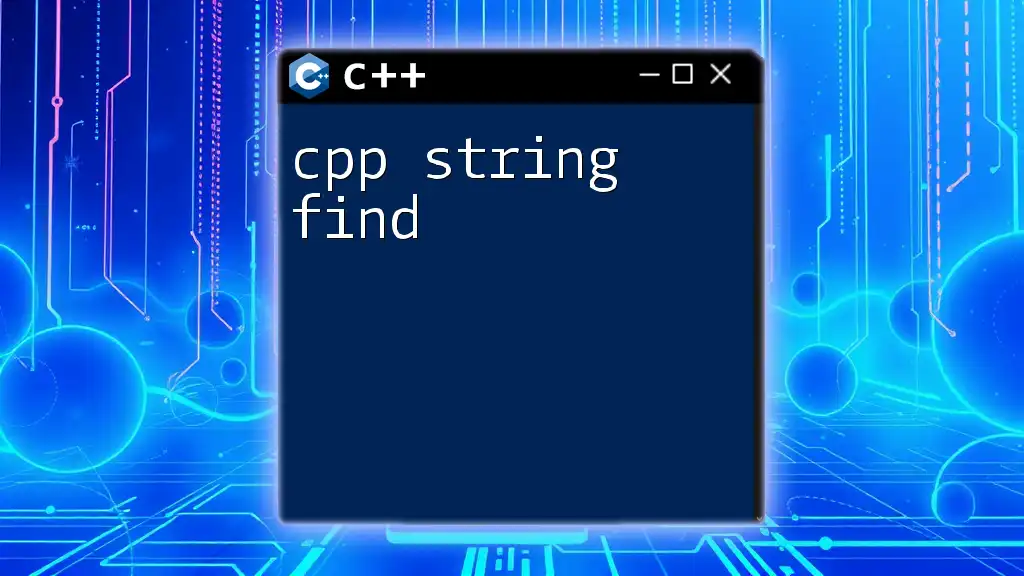
Why Convert C++ Strings to Unsigned Integers?
Converting C++ strings to unsigned integers is a common requirement in various programming scenarios. Some common situations where conversion may be needed include:
- Input validation: When you need to ensure that user input is a valid non-negative number before processing it.
- Mathematical operations: Strings often need conversion before performing arithmetic or comparisons.
- Data parsing from files or streams: Reading numerical data as strings requires conversion to perform operations on those values.
Handling user input effectively is crucial. By converting strings to unsigned integers, you can ensure your program behaves as expected while maintaining data integrity.
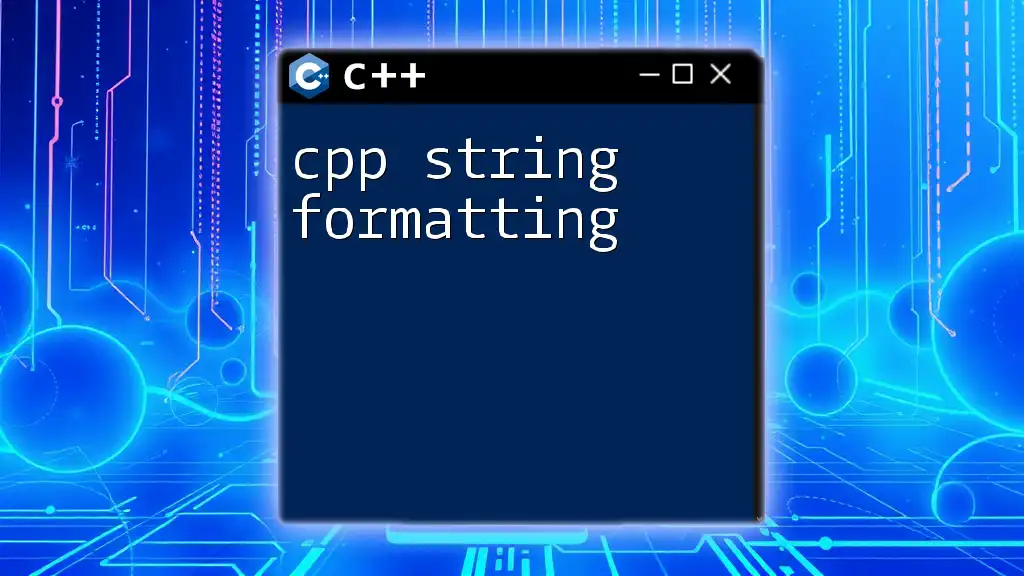
Methods for Converting String to Unsigned Integer
Using `std::stoi` Function
One of the most straightforward methods for converting a string to an unsigned integer is using the `std::stoi` function. This function converts a string to an integer while handling exceptions for invalid inputs.
Here is how to use `std::stoi` with proper error handling:
#include <iostream>
#include <string>
#include <stdexcept>
int main() {
std::string input = "12345";
unsigned int result;
try {
result = std::stoul(input); // Use std::stoul for unsigned int conversion
std::cout << "Converted value: " << result << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid input: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Input out of range: " << e.what() << std::endl;
}
return 0;
}
In this code snippet, `std::stoul` is used to ensure we convert the string to an unsigned long, which can then be safely assigned to an unsigned int, provided the value is within an acceptable range. The try-catch blocks allow you to handle potential errors gracefully, ensuring your program remains robust against invalid or out-of-range inputs.
Using `std::istringstream`
Another method for converting strings is to use `std::istringstream`, which stems from the C++ input-output stream library. This method allows you to easily extract values from a string, making it very suitable for conversions.
Here’s a code example using `std::istringstream`:
#include <iostream>
#include <sstream>
int main() {
std::string input = "67890";
unsigned int output;
std::istringstream(input) >> output; // Extracting unsigned int directly
std::cout << "Converted value: " << output << std::endl;
return 0;
}
In this snippet, we create an `istringstream` object with the string input, and then use the extraction operator (`>>`) to convert it to an unsigned integer. This approach is straightforward, but note that it does not handle errors directly; you should ensure the input string is valid before attempting the extraction.
Using C-style Functions (e.g., `strtoul`)
For those who prefer a more traditional C approach, the `strtoul` function can be utilized to convert a string to an unsigned long integer. This function provides a level of control over error checking as well.
Here’s how to implement it in C++:
#include <iostream>
#include <cstdlib>
int main() {
const char* input = "54321";
char* endPtr;
unsigned long output = std::strtoul(input, &endPtr, 10);
if (*endPtr != '\0') {
std::cerr << "Conversion error." << std::endl;
} else {
std::cout << "Converted value: " << output << std::endl;
}
return 0;
}
Using `strtoul`, you can specify the base for the conversion (here, base 10 for decimal). The `endPtr` allows you to check if the entire string was valid for conversion. If not, you will know that an error occurred, which can help you in debugging and improving user experience.
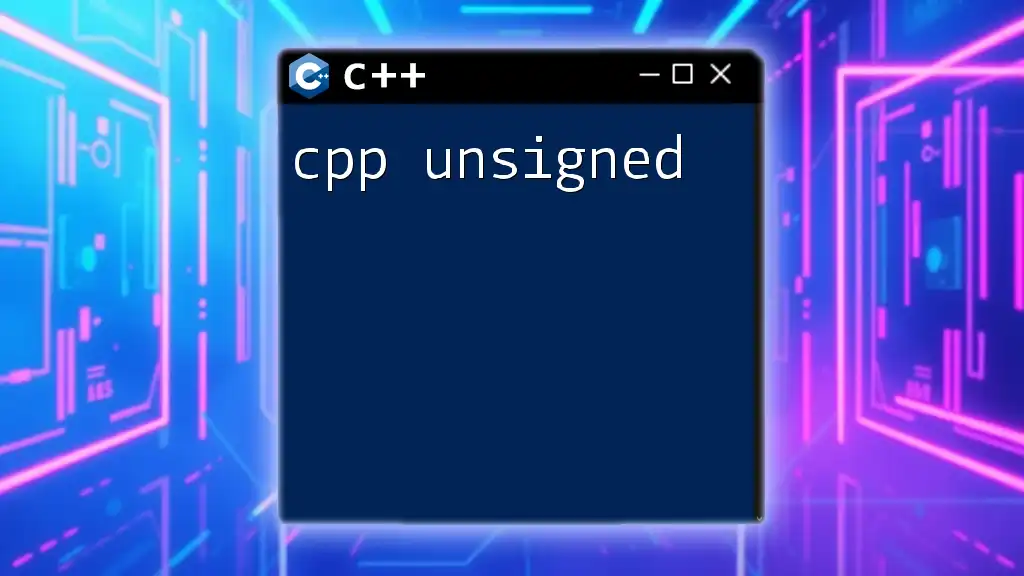
Error Handling in Conversion
Common Errors and Their Solutions
When converting strings to unsigned integers, several common errors may arise:
- Invalid inputs: If the string contains non-numeric characters, functions like `std::stoi` will throw an `invalid_argument` exception. Always validate your input before attempting conversion to prevent these errors.
- Out-of-range errors: If the string represents a number larger than what can be held in an unsigned integer, an `out_of_range` exception will occur. This is particularly relevant when dealing with user input, where the possibility of large numbers is common.
Implementing robust error handling strategies ensures your application operates smoothly, enhancing both reliability and user experience.
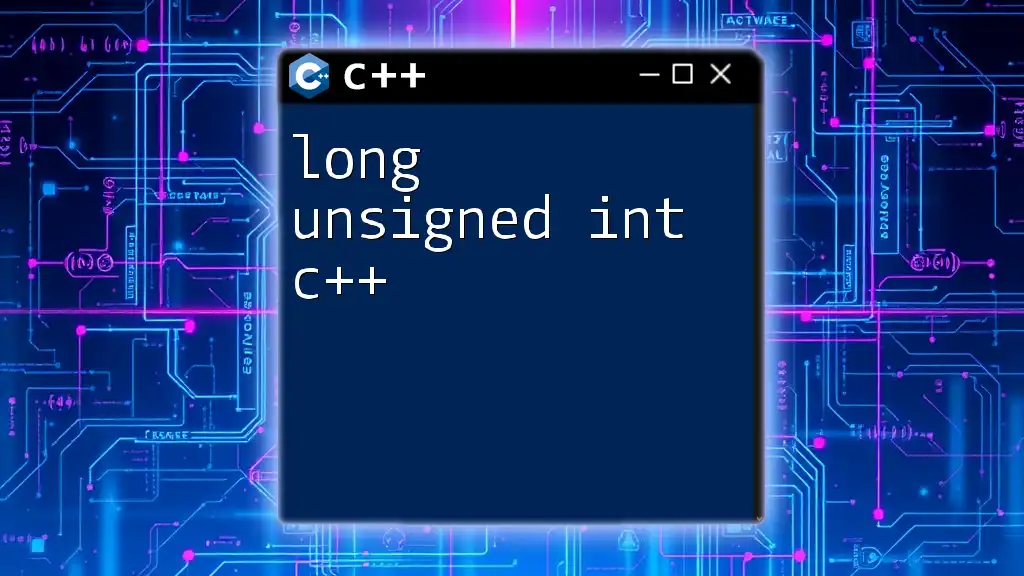
Best Practices for Converting Strings to Unsigned Integers
-
Choosing the Right Method: Depending on your input source and specific needs, choose the appropriate conversion method. For simple scenarios, `std::stoi` or `std::istringstream` may be sufficient. For more control and error handling, consider `strtoul`.
-
Ensuring Input Validation: Always validate your string inputs before attempting conversions. This can include checking for empty strings, non-numeric characters, and lengths that exceed expected limits.
-
Maintaining Code Clarity: Keep your code concise and easy to read. Use meaningful variable names and add comments to enhance clarity. This practice not only aids you in debugging but also helps others who may read your code.
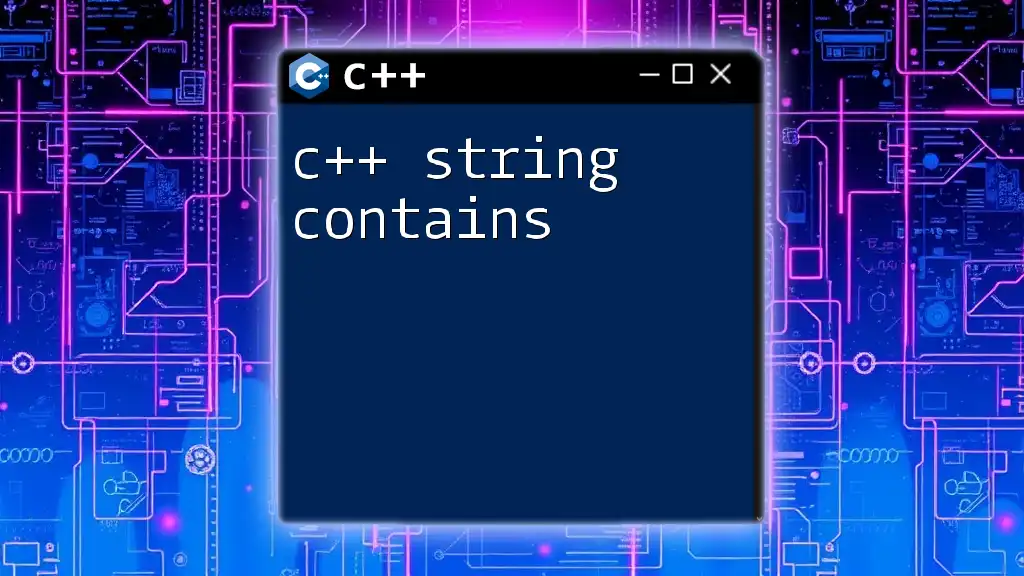
Conclusion
In summary, converting a C++ string to an unsigned integer is a valuable skill that can greatly enhance your programming toolkit. Understanding the various methods available and their applications will allow you to handle user input more effectively while ensuring robust data integrity. As you continue to develop your C++ skills, practice these conversions to gain confidence and efficiency in working with strings and integers.
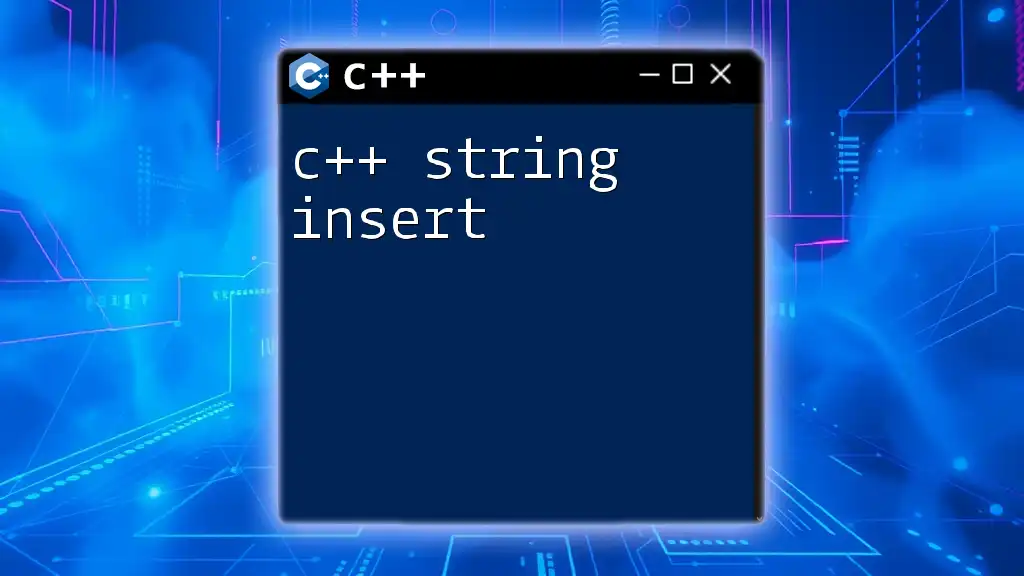
Additional Resources
Recommended Reading
Explore additional tutorials and references on C++ data types and error handling to further your understanding and expertise on this topic.
FAQs Related to String Conversion
If you have any pressing questions about string to unsigned integer conversion, consider common queries such as:
- “What happens if the string contains special characters?”
- “Is there a limit to the length of the string I can convert?”
Dive into these areas to boost your C++ command fluency!