In C++, the `unsigned` keyword is used to define variables that can only hold non-negative integer values, effectively doubling the positive range of the variable compared to its signed counterpart.
Here’s an example of using `unsigned` in C++:
#include <iostream>
int main() {
unsigned int positiveNumber = 42;
std::cout << "The unsigned number is: " << positiveNumber << std::endl;
return 0;
}
Why Use Unsigned in C++?
Using unsigned integers in C++ provides several advantages. These types increase the range of positive values that can be stored, effectively enhancing performance in scenarios where the domain of possible values is strictly non-negative.
Benefits of Using Unsigned Integers
- Performance Considerations: Unsigned types often use less memory and can optimize performance, especially in applications dealing with large quantities of data or intensive calculations.
- Use Cases: Unsigned types are ideal for variables that count items or index arrays. Since these operations naturally avoid negative numbers, using unsigned types is more semantically appropriate.
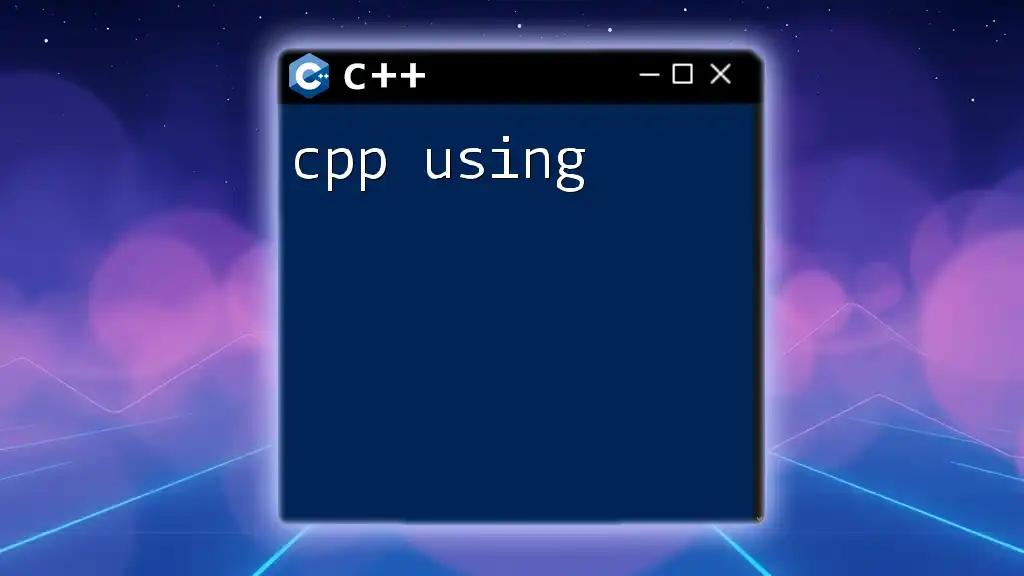
Understanding Unsigned Types
What Are Unsigned Integers?
In C++, an unsigned integer is a data type that can only represent non-negative whole numbers. Unlike signed integers, which can represent both negative and positive values, signed integers have a range that is split equally between negative and positive values. The key difference is the absence of negative values in unsigned types, effectively doubling the maximum positive range within the same byte size.
Common Unsigned Types in C++
C++ offers several types of unsigned integers to cater to different needs:
- `unsigned int`: Generally used for non-negative integers.
- `unsigned short`: Typically provides a smaller size, suitable for smaller numbers.
- `unsigned long`: Offers a larger range than `unsigned int`.
- `unsigned long long`: Provides the widest range of values among the unsigned types.
Here’s a simple illustration of variable declarations using unsigned types:
unsigned int x = 10;
unsigned long y = 1234567890;
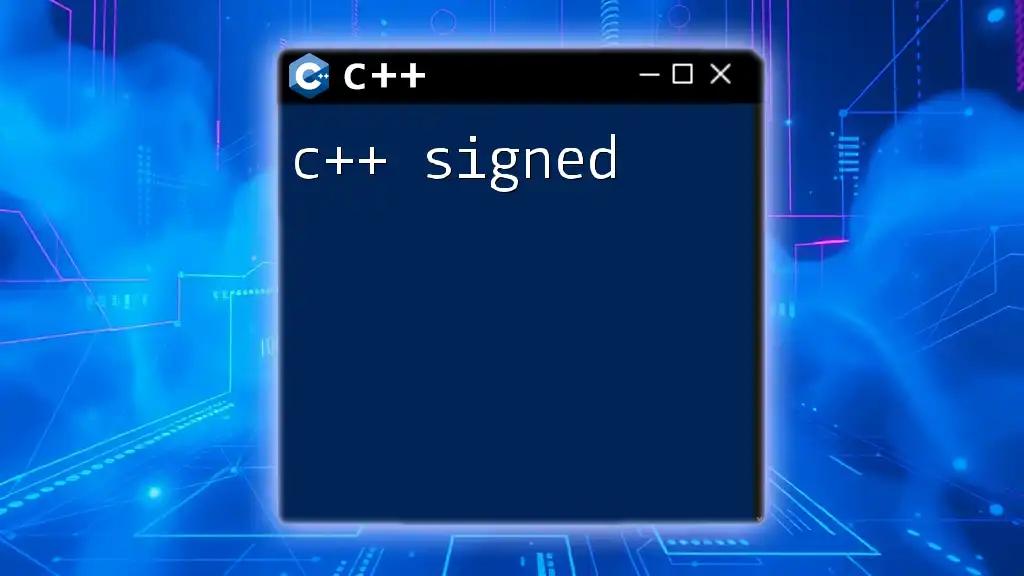
How to Declare Unsigned Variables
Declaring unsigned variables in C++ is straightforward. You simply prefix the data type with the keyword `unsigned`. The syntax is consistent across different unsigned types.
Here's how you can declare some unsigned variables:
unsigned short count = 0;
unsigned long totalMoney = 5000;
![Understanding Unsigned Int in C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fu%2Funsigned-int-cpp.webp&w=1080&q=75)
Operations on Unsigned Integers
Basic Operations
When performing arithmetic operations with unsigned integers, standard operations like addition, subtraction, multiplication, and division work just fine. However, since these types do not allow negative values, care must be taken especially with subtraction.
For example:
unsigned int a = 5;
unsigned int b = 10;
unsigned int sum = a + b; // sum is 15
Special Operations and Considerations
An important aspect to note is the behavior of unsigned integers during operations that can lead to overflow. Since the unsigned type does not accommodate negative values, underflowing an unsigned variable leads to wrapping around its maximum limit.
Take a look at this example:
unsigned int value = 0;
value = value - 1; // This will wrap around to the maximum value an unsigned int can hold
Understanding Underflows
When performing operations that could go below zero, understanding this wrapping behavior is crucial. Developers must anticipate edge cases where calculations may unexpectedly yield large numbers instead of negative values.

Type Conversion with Unsigned Integers
Implicit Type Conversions
Unsigned types can be implicitly converted to larger data types. For instance, an operation involving an unsigned integer and a larger signed type might still succeed without explicit conversion.
Explicit Type Conversions
However, converting signed integers to unsigned types requires caution. It’s important to use explicit casting to avoid unexpected behavior, especially if negative values are involved.
Here’s how you can do this in C++:
int a = -5;
unsigned int b = static_cast<unsigned int>(a); // b will hold a large, unexpected value (usually a large positive value)

Unsigned Types and Functions
Passing Unsigned Parameters
When dealing with functions, using unsigned integers as parameters can provide clarity in what is intended. It is beneficial when you know a variable will never be negative.
Returning Unsigned Values
Returning unsigned values from functions has its advantages too. This helps enforce that the values being returned will only be non-negative:
unsigned int getPositiveNumber() {
return 42; // This function guarantees a non-negative return value
}
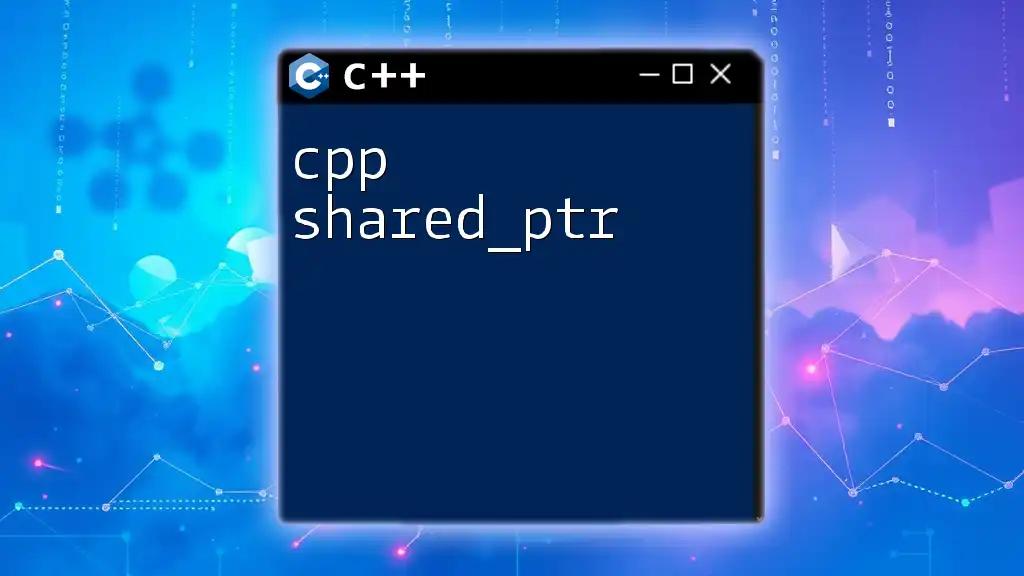
Common Pitfalls of Unsigned Integers
One of the most common pitfalls is overlooking how signed and unsigned integers interact. When an unsigned integer is combined with a signed integer in expressions, it may lead to unexpected results.
For example:
unsigned int x = 1;
x = x - 2; // This will cause x to wrap around to a very large value instead of becoming negative
Understanding how operations affect unsigned integers can avoid these potential issues, ensuring developers make better-informed decisions.

Conclusion
In summary, using cpp unsigned types is beneficial for programming practices involving non-negative integers. They help optimize memory and performance while clearly conveying intentions. As you continue to code in C++, always consider using unsigned types where applicable, and pay attention to the behaviors and pitfalls outlined to avoid unexpected results.

Additional Resources
For those looking to deepen their understanding of `cpp unsigned`, various books and online resources are available. Websites and community forums can also facilitate discussions and provide insights from other developers who have navigated similar challenges. Engaging with such resources can enhance your learning and practical application skills in C++.