A C++ scheduler is a system that manages the execution of tasks or processes based on specific timing or resource availability.
#include <iostream>
#include <thread>
#include <chrono>
void scheduledTask() {
std::cout << "Task executed!" << std::endl;
}
int main() {
std::this_thread::sleep_for(std::chrono::seconds(5)); // Delay for 5 seconds
scheduledTask(); // Execute scheduled task
return 0;
}
What is a C++ Scheduler?
A CPP Scheduler is a system or framework that manages the execution of tasks in C++ programs based on specific conditions and timing requirements. It allows developers to schedule different functions and methods to run at designated times or under certain circumstances. The importance of scheduling lies in its ability to optimize resource utilization and enhance the performance of applications by efficiently managing the order and timing of task executions.
In C++, scheduling is employed in various scenarios, such as managing background tasks in GUI applications, implementing game loops, handling asynchronous operations, and managing multiple threads in concurrent programming.
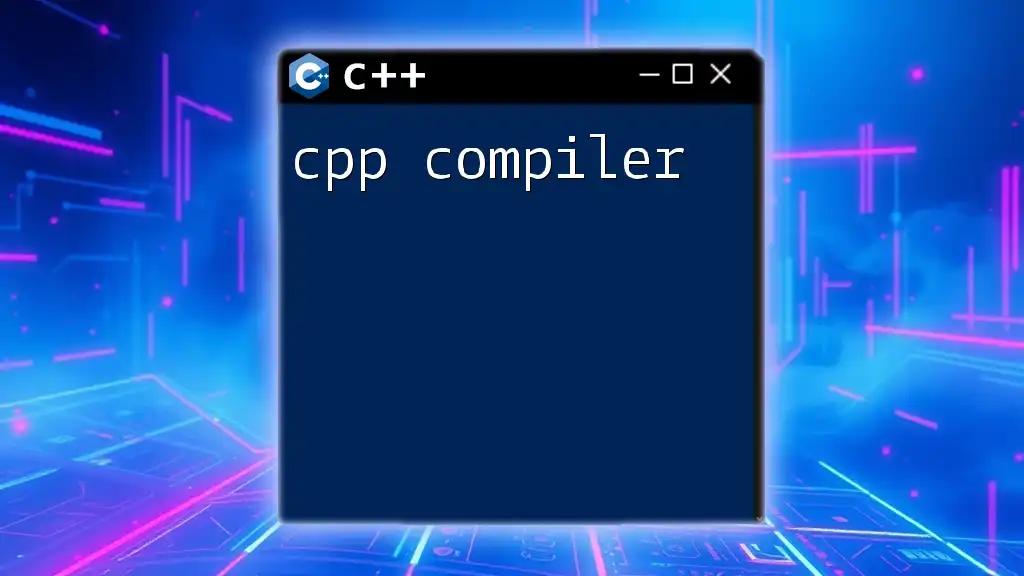
Types of Scheduling
Preemptive Scheduling
Preemptive scheduling allows the system to interrupt a currently running task to start or resume another task based on priority or timing criteria. In this approach, the operating system decides when to take control from one task and assign it to another.
Advantages
- Responsiveness: Enhances responsiveness to real-time events.
- Fairness: Ensures that all tasks receive CPU time, promoting fairness in resource allocation.
Disadvantages
- Complexity: Requires more intricate mechanisms for context switching and synchronization.
- Overhead: More resources are spent on managing task switching.
Use Cases in C++ Programming
Preemptive scheduling is suitable for applications that need to respond to time-sensitive events, such as multimedia applications and real-time systems.
Example Code Snippet
// Preemptive Scheduling Example
#include <iostream>
#include <thread>
#include <chrono>
void task1() {
while (true) {
std::cout << "Task 1 running." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
}
}
void task2() {
while (true) {
std::cout << "Task 2 running." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
}
}
int main() {
std::thread t1(task1);
std::thread t2(task2);
t1.join();
t2.join();
return 0;
}
Cooperative Scheduling
Cooperative scheduling, on the other hand, relies on tasks to voluntarily yield control periodically or when idle. In this approach, each task must be designed to cooperate with others by yielding execution when appropriate.
Advantages
- Simplicity: Easier to implement with less overhead and fewer context-switching operations.
- Deterministic: Provides predictable execution order since tasks yield control explicitly.
Disadvantages
- Responsiveness Issues: If a task does not yield, it can block others, leading to performance bottlenecks.
- Complex Task Management: Requires careful design to ensure that all tasks eventually receive CPU time.
Use Cases in C++ Programming
This type of scheduling is often utilized in systems with limited resources, such as embedded systems and simple game engines.
Example Code Snippet
// Cooperative Scheduling Example
#include <iostream>
#include <thread>
#include <chrono>
void task1() {
std::cout << "Task 1 starting..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(3));
std::cout << "Task 1 completed." << std::endl;
}
void task2() {
std::cout << "Task 2 starting..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Task 2 completed." << std::endl;
}
int main() {
task1();
task2();
return 0;
}

Building a Simple C++ Scheduler
Basic Concepts
Before diving into implementing a C++ Scheduler, it's important to understand the fundamental concepts related to multithreading in C++. Threads are essential for executing tasks concurrently. To create a scheduler, you'll primarily use components from the C++ Standard Library, including:
- `<thread>`: For creating and managing threads.
- `<mutex>`: For ensuring safe access to shared resources.
- `<condition_variable>`: For synchronizing threads.
Implementation Steps
Step 1: Create a Task Queue
The task queue acts as a storage for the tasks that need to be executed. It allows the scheduler to manage tasks efficiently.
Step 2: Implement Methods to Add and Execute Tasks
You will need to develop the core functionality that allows adding tasks to the queue and triggering their execution.
Example Code Snippet
#include <iostream>
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <functional>
std::queue<std::function<void()>> tasks;
std::mutex mtx;
std::condition_variable cv;
void scheduler() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !tasks.empty(); });
auto task = tasks.front();
tasks.pop();
lock.unlock();
task(); // Execute task
}
}
void addTask(std::function<void()> task) {
std::lock_guard<std::mutex> lock(mtx);
tasks.push(task);
cv.notify_one();
}
int main() {
std::thread schedulerThread(scheduler);
addTask([] { std::cout << "Running task 1" << std::endl; });
addTask([] { std::cout << "Running task 2" << std::endl; });
schedulerThread.join();
return 0;
}

Advanced C++ Scheduling Techniques
Thread Pools
Thread pools are an advanced technique in scheduling, designed to optimize task execution by reusing a fixed number of threads to execute multiple tasks. This approach minimizes the overhead of repeatedly creating and destroying threads.
Benefits of Using Thread Pools in C++
- Efficiency: Reduces the overhead of thread management by maintaining a pool of active threads.
- Scalability: Can handle numerous tasks concurrently without overwhelming the system.
Example of Implementing a Thread Pool Scheduler
The following example demonstrates a simple implementation of a thread pool that can enqueue and execute tasks concurrently.
Example Code Snippet
#include <iostream>
#include <vector>
#include <queue>
#include <thread>
#include <future>
#include <functional>
#include <mutex>
#include <condition_variable>
class ThreadPool {
public:
ThreadPool(size_t);
template<class F>
auto enqueue(F&& f) -> std::future<typename std::result_of<F()>::type>;
~ThreadPool();
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable cv;
bool stop;
};
ThreadPool::ThreadPool(size_t threads)
: stop(false) {
for(size_t i = 0; i < threads; ++i)
workers.emplace_back([this] {
for(;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->cv.wait(lock, [this]{ return this->stop || !this->tasks.empty(); });
if(this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
template<class F>
auto ThreadPool::enqueue(F&& f) -> std::future<typename std::result_of<F()>::type> {
using return_type = typename std::result_of<F()>::type;
auto task = std::make_shared<std::packaged_task<return_type()>>(std::forward<F>(f));
std::future<return_type> res = task->get_future();
{
std::unique_lock<std::mutex> lock(queue_mutex);
if(stop)
throw std::runtime_error("enqueue on stopped ThreadPool");
tasks.emplace([task](){ (*task)(); });
}
cv.notify_one();
return res;
}
ThreadPool::~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
cv.notify_all();
for(std::thread &worker: workers)
worker.join();
}
int main() {
ThreadPool pool(4);
for (int i = 0; i < 8; ++i) {
pool.enqueue([i] {
std::cout << "Task " << i << " running\n";
});
}
return 0;
}
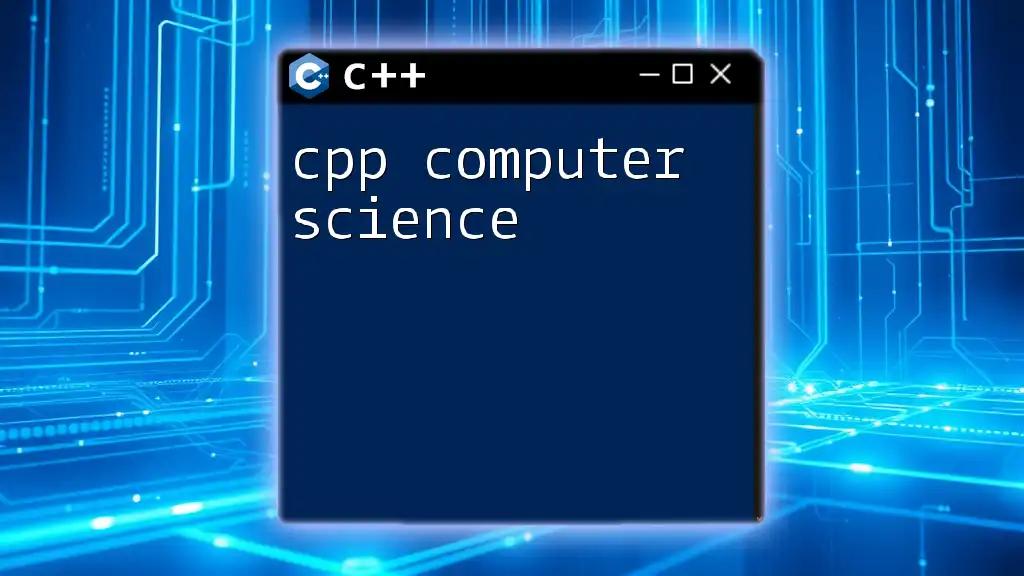
Best Practices for Scheduling in C++
When developing a C++ Scheduler, consider the following best practices to optimize your implementation:
- Use Proper Synchronization: Always ensure thread safety by using mutexes and condition variables appropriately to avoid data races and deadlocks.
- Implement Error Handling: Incorporate robust error handling to manage unexpected failures during task execution.
- Avoid Blocking Operations: Strive to minimize blocking calls in your tasks to ensure better responsiveness and performance.
- Profile and Optimize: Regularly profile your scheduler to identify bottlenecks and optimize performance.
By adhering to these practices, you can create a more efficient and maintainable C++ Scheduler.
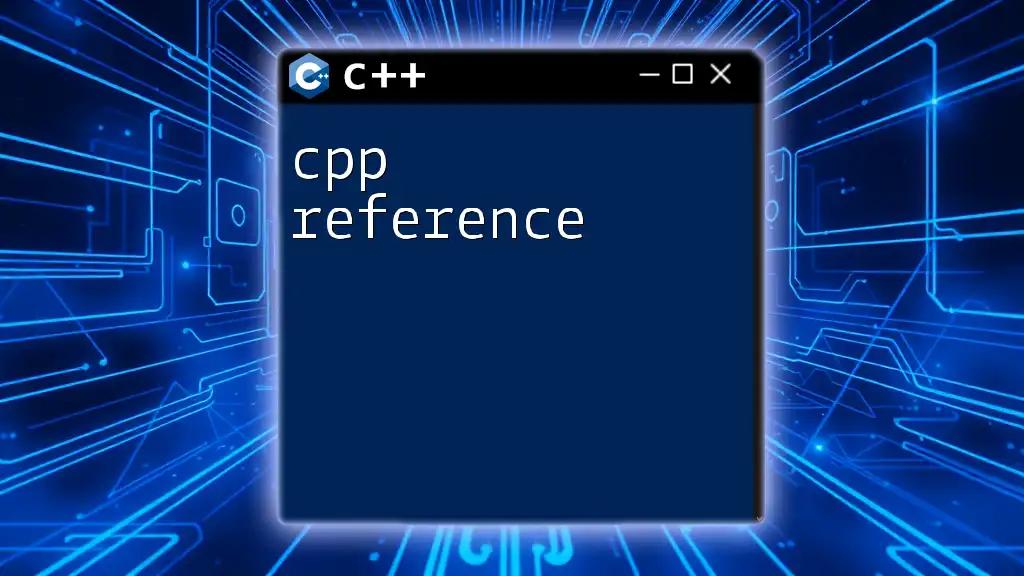
Conclusion
A CPP Scheduler plays a crucial role in managing the execution of tasks efficiently, contributing significantly to the performance and responsiveness of applications. With an understanding of different scheduling techniques, such as preemptive and cooperative scheduling, along with advanced constructs like thread pools, developers can harness the power of C++ to create highly optimized applications.
As programming paradigms and techniques continue to evolve, exploring C++ scheduling can open up new paths for improving software architecture and functionality. Embracing these strategies not only enhances existing systems but also prepares developers for the future of concurrent programming in C++.