The `std::accumulate` function in C++ is used to calculate the sum or total of a range of elements by applying a binary operation, typically addition.
#include <iostream>
#include <vector>
#include <numeric> // for std::accumulate
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int total = std::accumulate(numbers.begin(), numbers.end(), 0);
std::cout << "Total: " << total << std::endl; // Output: Total: 15
return 0;
}
What is `accumulate`?
The `accumulate` function is a part of the Standard Template Library (STL) in C++, specifically included in the `<numeric>` header. Its primary purpose is to perform accumulation or aggregation on a range of values, making it a powerful tool for data processing.
When comparing `accumulate` to other aggregation functions like `reduce` or `sum`, it stands out due to its flexibility. It allows not only addition but also various user-defined operations through the use of custom functions or lambdas.
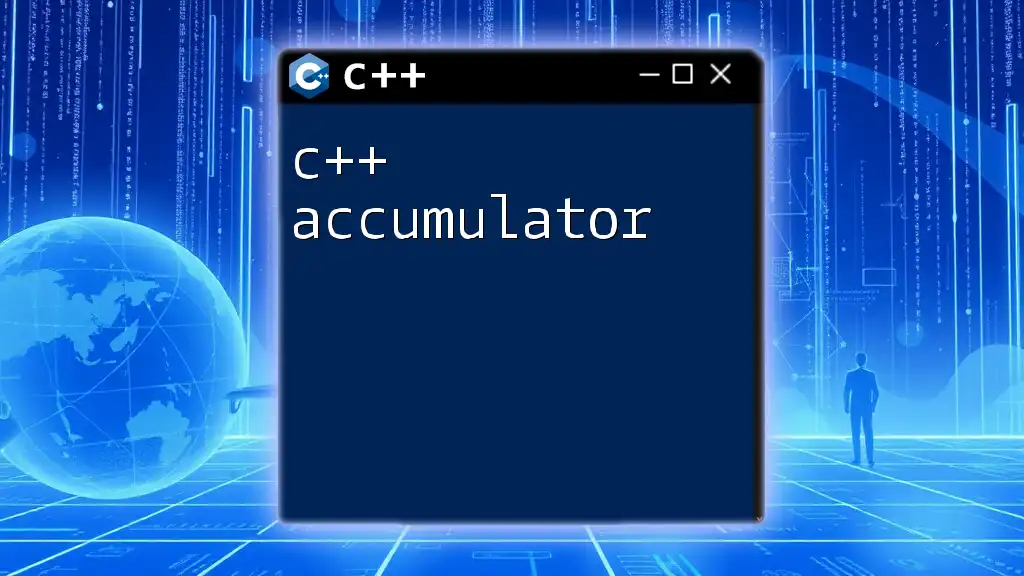
Understanding the Syntax of `accumulate`
The function signature for `accumulate` is as follows:
template<typename InputIterator, typename T>
T accumulate(InputIterator first, InputIterator last, T init);
Explanation of the Parameters
- `InputIterator first`: This parameter points to the beginning of the range you want to process.
- `InputIterator last`: This parameter points to the end of the range.
- `T init`: This is the initial value for the accumulation. It serves as a base value upon which the rest of the values from the range will be added or manipulated.
By understanding the syntax, you begin to see how `accumulate` can be leveraged for various data types and operations.
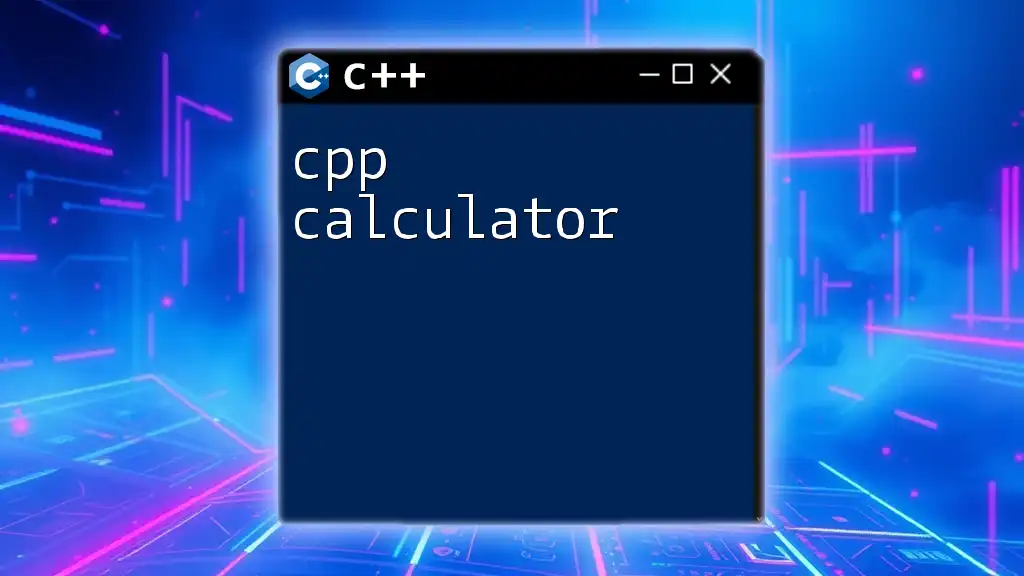
Including the Necessary Headers
To use `accumulate`, you must include certain headers in your program:
#include <numeric> // for std::accumulate
#include <vector> // for std::vector
#include <iostream> // for std::cout
Including `<numeric>` is essential as it contains the definition of `accumulate`. Without this header, your code will not recognize the function.
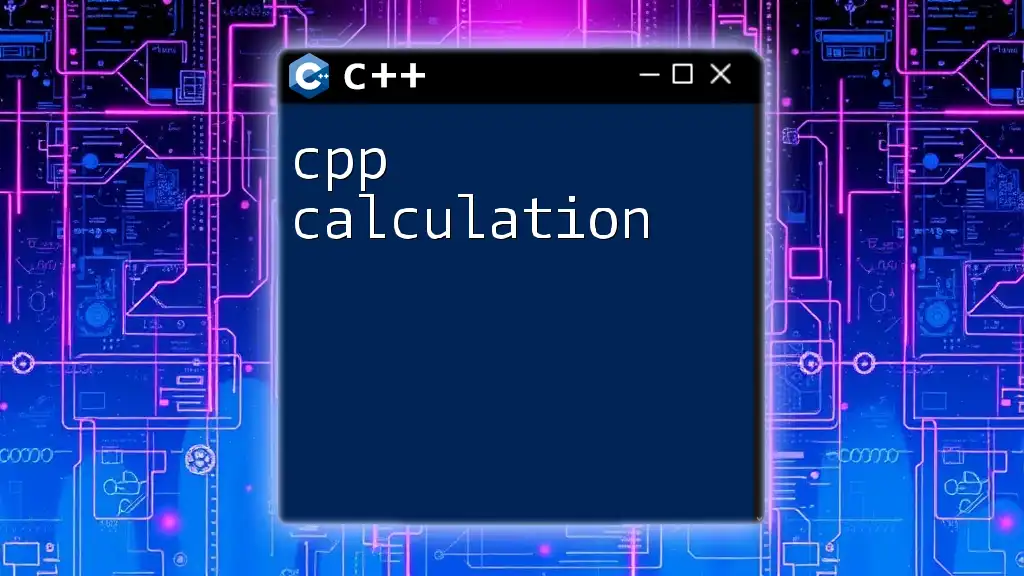
Basic Usage of `accumulate`
Simple Summation
The simplest usage of `accumulate` is to sum the elements of a numeric vector. For example:
#include <iostream>
#include <numeric>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int sum = std::accumulate(vec.begin(), vec.end(), 0);
std::cout << "Sum: " << sum << std::endl; // Output: Sum: 15
return 0;
}
In this example, `std::accumulate` takes the beginning and end of the vector and an initial value of `0`. The output, 15, is the sum of all the elements in the vector. Understanding how `accumulate` processes ranges allows you to use it effectively in a variety of scenarios.
Advanced Usage Examples
Using an Initial Value
The initial value can change the result of the accumulation. For instance:
int sumWithInitial = std::accumulate(vec.begin(), vec.end(), 10);
std::cout << "Sum with Initial: " << sumWithInitial << std::endl; // Output: Sum: 25
In this case, `10` is added to the accumulated total, resulting in a different output. Understanding how the initial value impacts results is crucial when using `accumulate`.
Accumulating Complex Data Types
`accumulate` can also be applied to vectors containing more complex data types, such as structs. For example:
struct Product {
std::string name;
double price;
};
double totalCost(std::vector<Product> products) {
return std::accumulate(products.begin(), products.end(), 0.0,
[](double sum, const Product& p) {
return sum + p.price;
});
}
In this example, we're summing up the prices of products stored in a vector of structs. The custom lambda function allows you to define how the accumulation operates on each item in the vector. This flexibility is one of the strengths of using `cpp accumulate`.
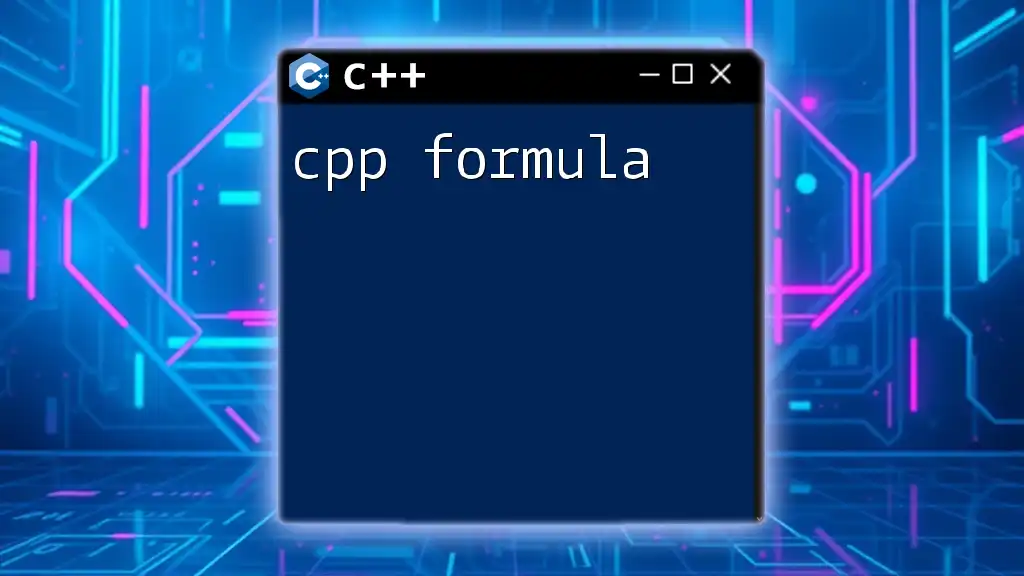
Customizing the Accumulation Operation
Using Lambda Functions
Lambdas provide an excellent way to customize the behavior of `accumulate`. You can define custom operations directly inline. Consider the following code:
double customSum = std::accumulate(vec.begin(), vec.end(), 0.0, [](double sum, int value) {
return sum + value * 2; // Doubling the sum
});
In this example, instead of simply summing the values, we double each value before adding it to the total. This demonstrates the powerful customization that `accumulate` offers through lambda expressions.
Using Function Pointers
You can also use traditional functions to customize the accumulation process. This method may appeal to those who prefer more structured code. Here’s how it looks:
double multiply(double sum, int value) {
return sum + value * 2; // Similar logic as the lambda
}
double customSumUsingFunctionPointer = std::accumulate(vec.begin(), vec.end(), 0.0, multiply);
This approach allows you to separate the logic from the main operation, which can enhance readability in more extensive codebases.
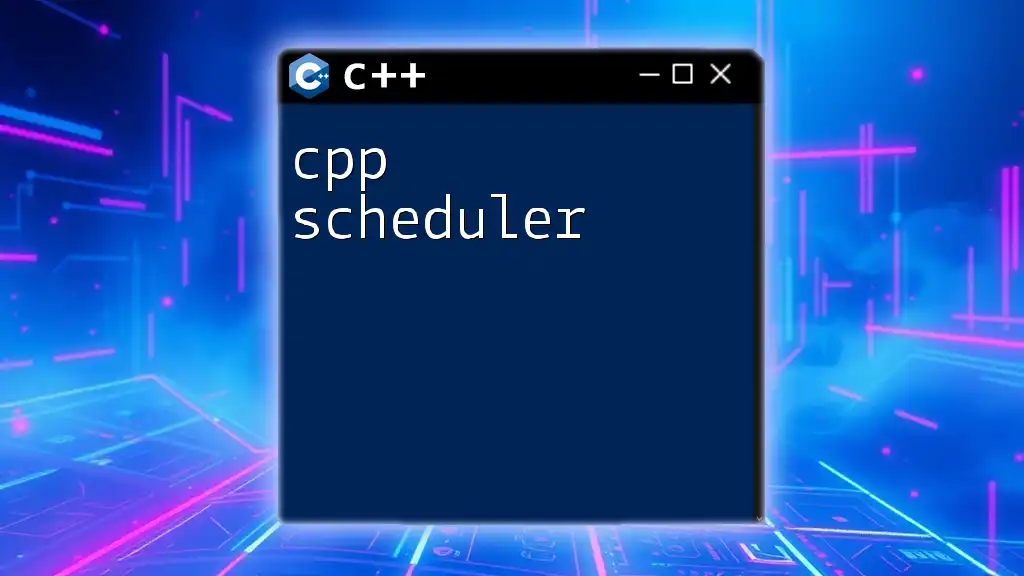
Performance Considerations
While `accumulate` is powerful, it’s essential to be aware of potential performance impacts when working with large datasets. The efficiency of accumulation depends on the operations performed, data types used, and the overall size of the input.
Choose Suitable Data Types
Using the appropriate data types, especially when dealing with floating-point operations or large integers, can greatly affect performance. It’s worth evaluating your requirements to ensure you're optimizing where necessary.
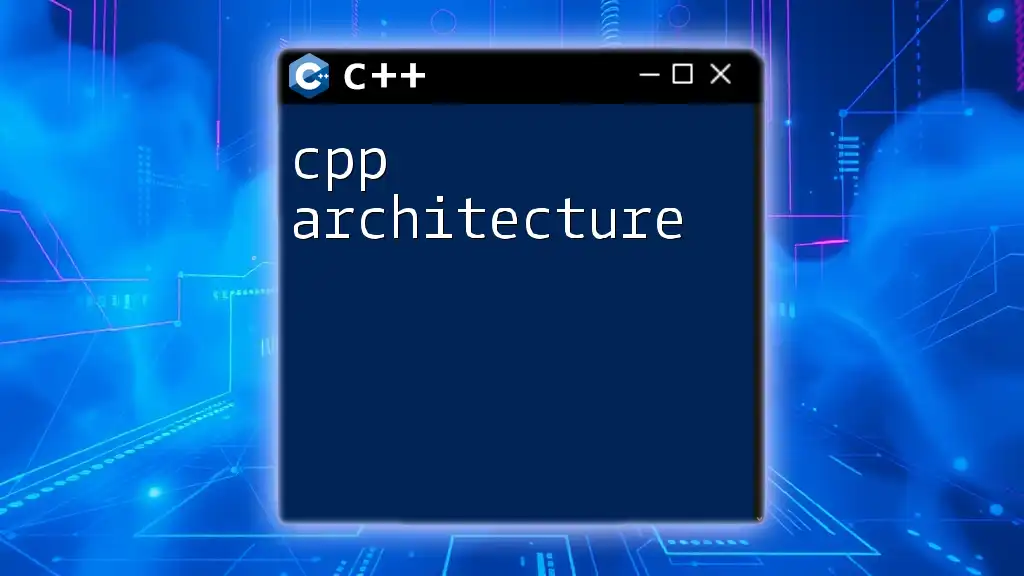
Common Mistakes and How to Avoid Them
-
Forgetting to Include `<numeric>`: This is a common pitfall, as missing this header will cause compilation errors.
-
Misunderstanding Input Range Boundaries: Ensure you grasp the concepts of iterators and the range they define. Not doing so can lead to unexpected results or errors.
-
Using Incorrect Data Types for Initialization: The initialization value should be compatible with the type of the elements being accumulated, or you'll run into conversion issues.
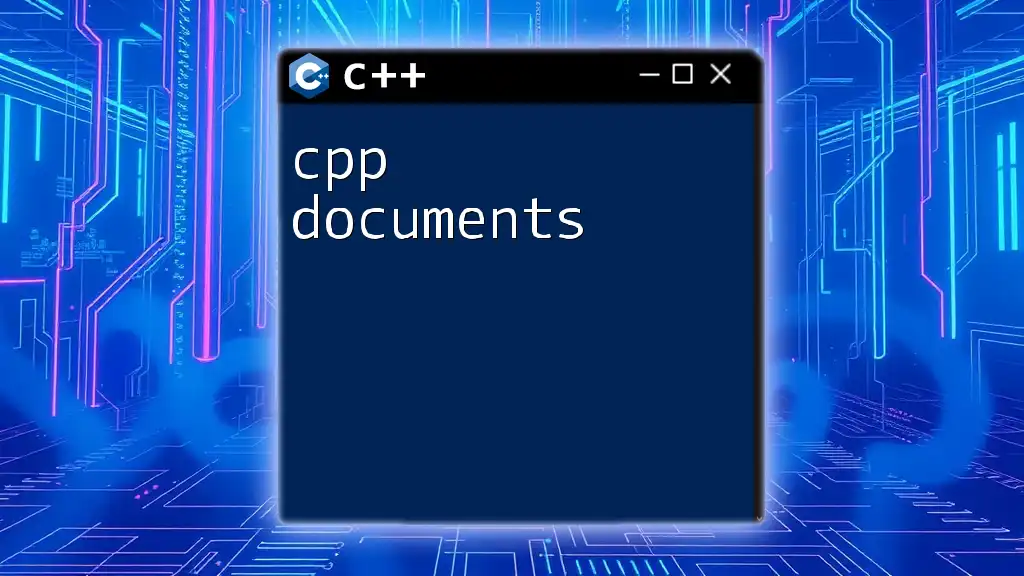
Conclusion
In summary, the C++ `accumulate` function is versatile and powerful, enabling programmers to easily aggregate data with various operations. By understanding its syntax, using it effectively with simple or complex types, and customizing its behavior, you can enhance your data processing capabilities in C++.
Practice using `cpp accumulate` in your projects, and you may uncover even more ways this function can optimize your code. Feel free to share any examples or implementations you come across!
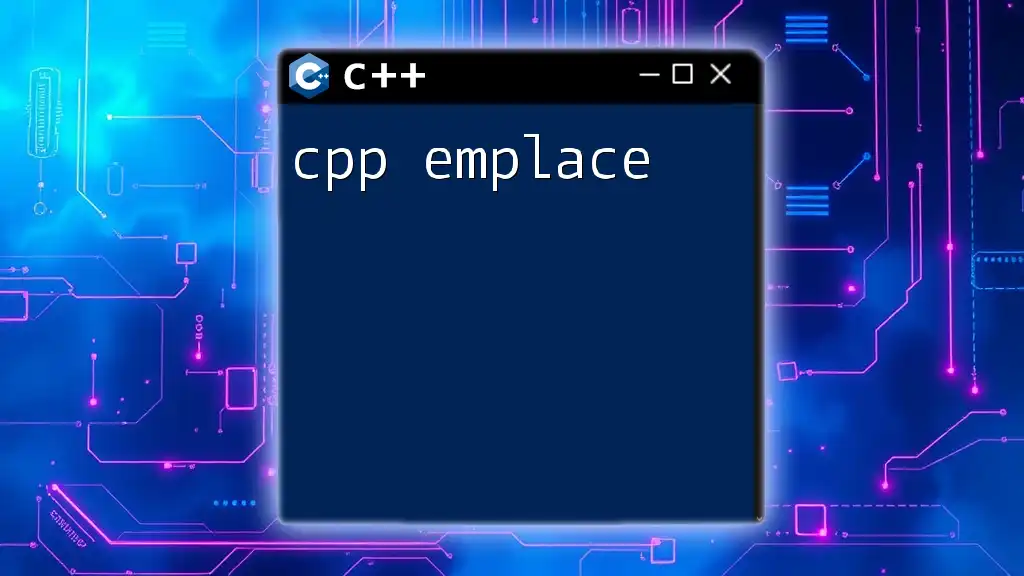
Further Resources
For additional learning, refer to the official C++ documentation on the Standard Library, and consider exploring books or online resources to deepen your understanding of C++. Don’t forget to check out our services for more practical guidance and training in C++.