The C++ foundation refers to the basic principles and syntax necessary for understanding and utilizing the C++ programming language effectively.
Here's a simple example demonstrating the basic structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++: A Brief Introduction
What is C++?
C++ is a powerful, high-performance programming language that is widely used in systems software, application software, and game development. Created by Bjarne Stroustrup at Bell Labs in the early 1980s, C++ builds upon the foundational concepts of the C language, adding support for object-oriented programming (OOP), which allows for creating software that is modular and reusable.
The significance of C++ in modern programming cannot be overstated. It serves as the backbone for many popular applications and frameworks, making it a key language for any software developer’s toolkit.
Key Features of C++
C++ is equipped with numerous features that enhance its utility:
- Object-Oriented Programming (OOP): This paradigm helps organize and structure large codebases through encapsulation, inheritance, and polymorphism. It encourages code reuse and simplicity in programming.
- Low-Level Memory Manipulation: C++ offers direct access to hardware and memory management, allowing for high-performance computing and fine-tuning system resources.
- Rich Library Support: The Standard Template Library (STL) provides a plethora of containers and algorithms that can simplify everyday programming tasks.
- Performance Efficiency: C++ allows for optimizations that make it suitable for performance-critical applications, ensuring that code runs efficiently.
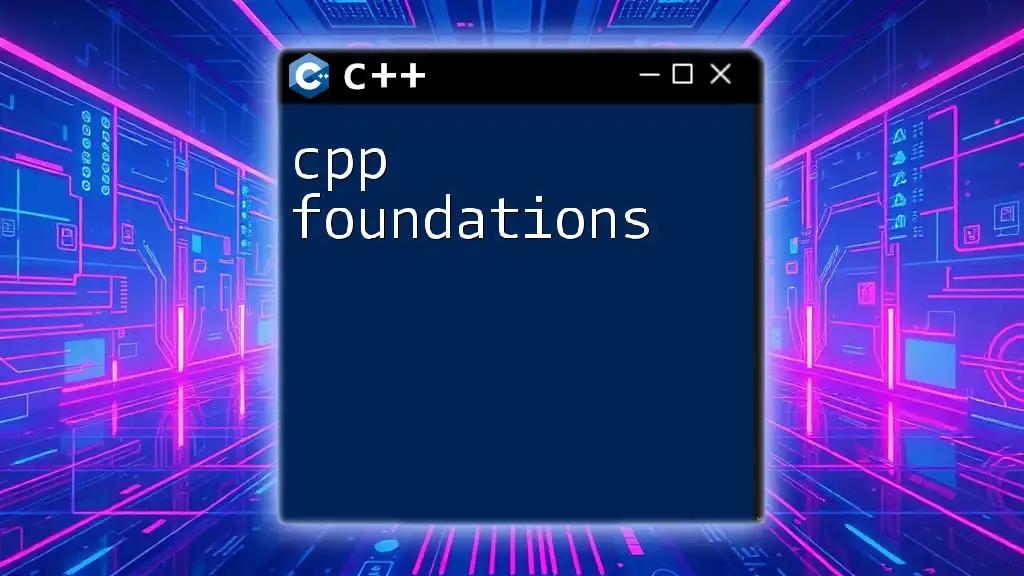
Core Concepts of C++
Variables and Data Types
Variables are fundamental components in C++ that store data to be manipulated during program execution. The most common data types include:
- int: Stores integers, both positive and negative.
- float: A single-precision floating-point data type.
- double: A double-precision floating-point data type for more precision.
- char: Represents single characters.
- string: Used for storing sequences of characters.
For example, you can declare and initialize variables like so:
int age = 30;
float salary = 50000.50;
char initial = 'A';
string name = "John Doe";
Understanding the scope (the visibility of variables) and lifetime (how long a variable exists in memory) of these variables is crucial while programming.
Control Structures
Conditional Statements
Conditional logic allows programs to execute specific blocks of code based on certain conditions. The most common control structures include if, else if, and else statements.
Here’s a simple example:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
The switch-case statement is another way to handle multiple conditions effectively, improving code readability.
Loops
Loops allow for repeated execution of a block of code. The main types of loops in C++ include:
- for loops: Best for a known number of iterations.
- while loops: Executes based on a condition.
- do-while loops: Guarantees execution at least once.
Here’s an example of a for loop:
for (int i = 0; i < 5; i++) {
cout << "Iteration: " << i << endl;
}
Each loop type has its specific use cases, and understanding these will greatly enhance your coding proficiency.
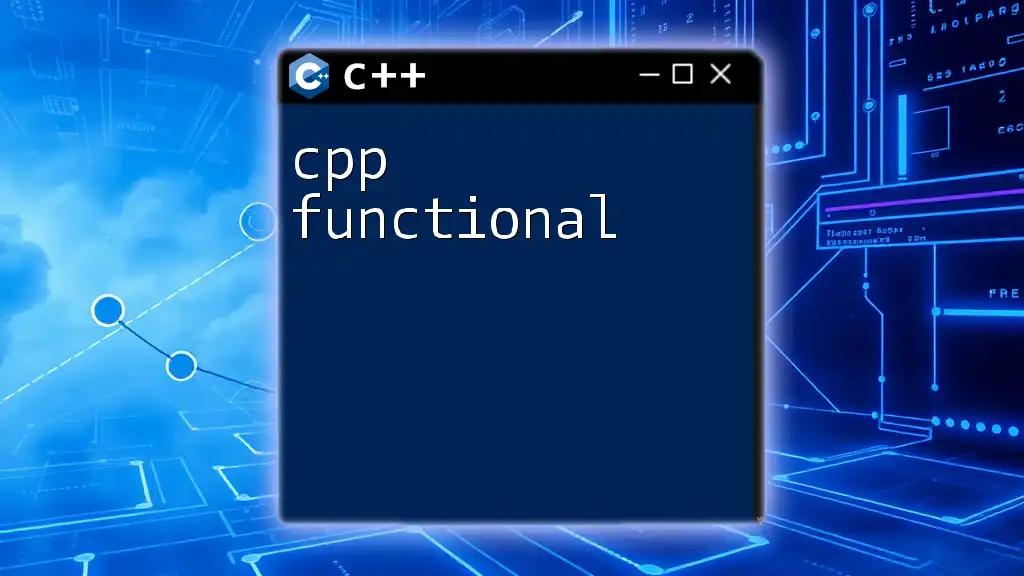
Object-Oriented Programming in C++
Classes and Objects
Classes serve as blueprints for creating objects and encapsulating data and functions. An object is an instance of a class.
Here’s how you can define a basic class:
class Car {
public:
string brand;
void honk() {
cout << "Honk! Honk!";
}
};
It’s essential to understand access specifiers such as public, private, and protected, which control data access permissions in a class.
Inheritance
Inheritance is a fundamental concept allowing one class to derive properties from another. It promotes code reusability.
In C++, several types of inheritance exist, including single, multiple, and hierarchical inheritance. Here’s a simple example demonstrating inheritance:
class Vehicle {
public:
void drive() { cout << "Driving"; }
};
class Bike : public Vehicle {
public:
void ringBell() { cout << "Ring Ring"; }
};
With inheritance, `Bike` can use the methods of `Vehicle`, enhancing code efficiency and organization.
Polymorphism
Polymorphism allows functions to operate in different ways based on their parameters. It comes in two types: compile-time (method overloading) and run-time (method overriding).
Here’s an example of method overloading, where the same function name operates differently based on the type of argument passed:
void display(int i) {
cout << "Integer: " << i;
}
void display(string s) {
cout << "String: " << s;
}
Understanding and utilizing polymorphism is vital in writing adaptable and maintainable code.
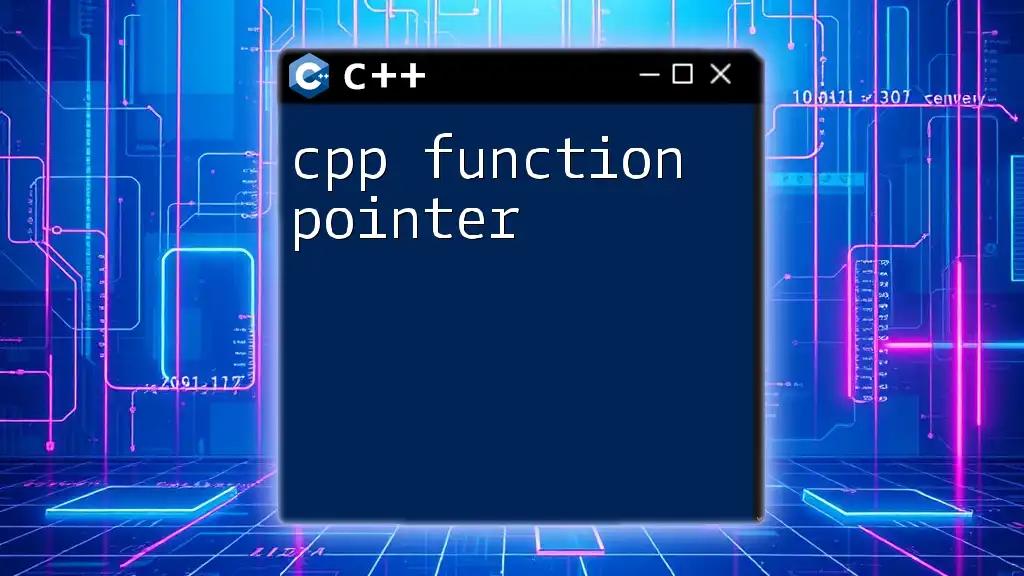
Memory Management in C++
Dynamic Memory Allocation
Dynamic memory allocation allows programs to request and release memory during runtime, which is crucial for managing resources efficiently.
C++ uses the keywords new and delete for allocating and deallocating memory. Here’s how it works:
int* arr = new int[5];
// Use the array...
delete[] arr; // Free the memory
Properly managing dynamic memory is crucial to avoid memory leaks, which can degrade application performance.
Smart Pointers
Smart pointers provide automated memory management, reducing the risk of memory leaks and dangling pointers. C++11 introduced various smart pointers:
- unique_ptr: Maintains sole ownership of a resource.
- shared_ptr: Allows multiple pointers to share ownership of a resource.
- weak_ptr: Provides a non-owning reference to prevent circular references.
Here’s an example of using a unique_ptr:
unique_ptr<int> p1(new int(10));
cout << *p1; // Accessing value
Using smart pointers simplifies memory management and enhances program stability.
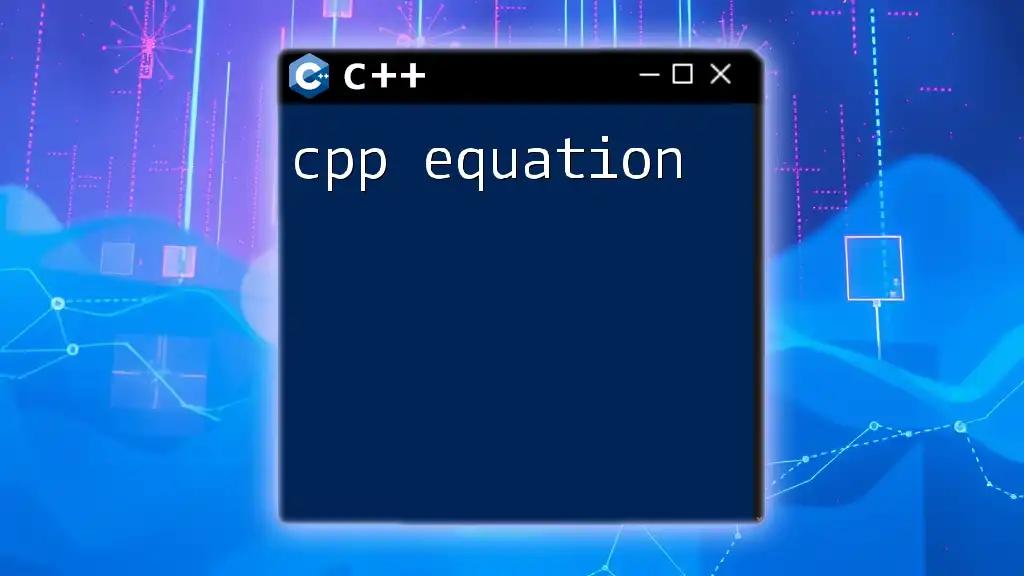
Practical Applications of C++
Game Development
C++ is a dominant language in the game development industry due to its performance and versatility. It’s the primary language used in popular game engines like Unreal Engine, allowing developers to create immersive experiences with rich graphics.
Systems Programming
As a systems programming language, C++ allows for interfacing with hardware and performing low-level tasks. This makes it a preferred choice for operating system development and creating embedded systems, providing high control over system resources.
Application Development
C++ is extensively used in developing desktop applications. Its ability to create graphical interfaces can be powered by frameworks such as Qt and wxWidgets, enabling developers to build sophisticated user interfaces that enhance user experience.
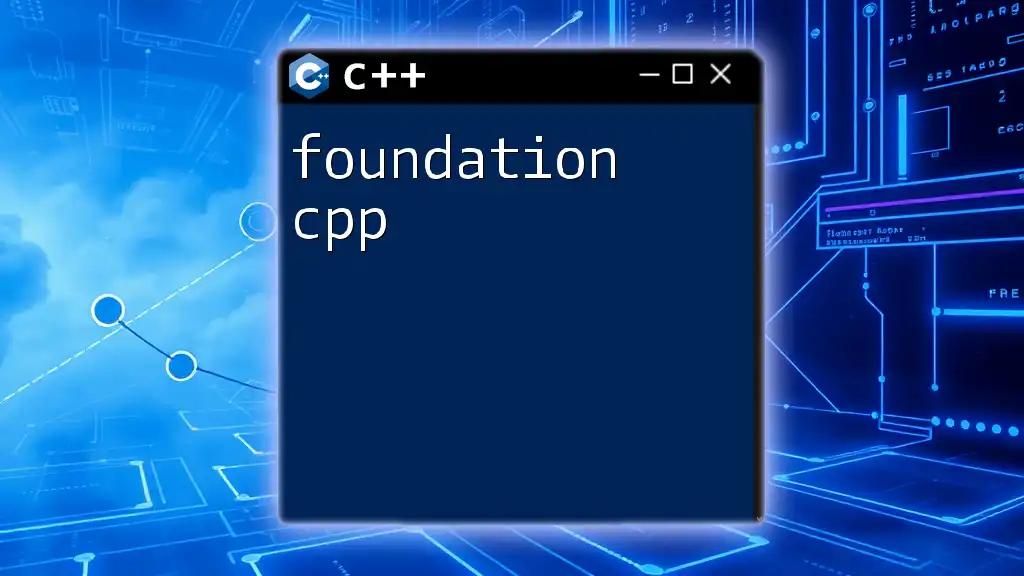
Conclusion
Why Learn C++?
C++ offers a rich set of features enabling developers to write efficient, high-performance code. Understanding its foundational principles will not only enhance your programming skills but also open doors to various career opportunities in software development.
Additional Resources
To further your journey in mastering C++, consider exploring recommended books, websites, and online courses. Joining community forums and discussion groups can also provide valuable support and insights from fellow learners and professionals in the field.
By laying a solid C++ foundation, you empower yourself to tackle advanced programming challenges and contribute effectively to various software projects.