In C++, summation can be accomplished by iterating through a range of numbers and aggregating them into a total using a simple loop.
#include <iostream>
int main() {
int sum = 0;
for (int i = 1; i <= 10; ++i) {
sum += i;
}
std::cout << "The sum of the first 10 numbers is: " << sum << std::endl;
return 0;
}
Understanding Summation Basics
What is Summation?
C++ summation is the mathematical process of adding a sequence of numbers or mathematical terms. In programming, understanding how to efficiently sum elements within arrays, lists, or any collection is fundamental for various algorithms and data manipulations. Summation serves as the backbone of many algorithms, from simple ones, like finding the total of an array, to complex ones used in data analytics and statistical modeling.
Mathematical Notation
In mathematics, summation is usually represented using the sigma notation (∑), which succinctly expresses the process of summing a series of components. For example, the summation \( \sum_{i=1}^{n} i \) refers to adding all integers from 1 to n. This mathematical concept easily translates to programming, where we often apply loops or recursive methods to perform similar tasks.
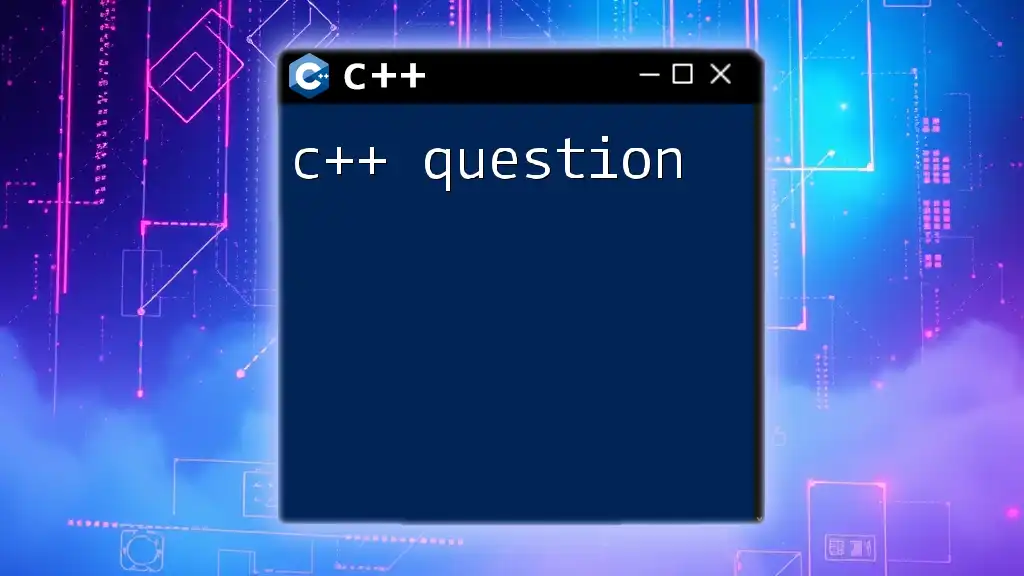
Summation Techniques in C++
Using Loops for Summation
For Loops for Summation
The for loop is one of the most intuitive ways to perform summation in C++. By iterating through an array or sequence, you can accumulate the sum easily.
Here's a simple example using a for loop:
int sumForLoop(int arr[], int size) {
int sum = 0;
for (int i = 0; i < size; i++) {
sum += arr[i];
}
return sum;
}
In the above code, we declare an integer variable `sum` initialized to 0. The loop iterates over each element of the array `arr`, adding each element to `sum`.
While Loops for Summation
A while loop functions similarly but offers flexibility in iteration control. It's useful in scenarios where the termination condition may not be strictly based on a count.
Example of using a while loop for summation:
int sumWhileLoop(int arr[], int size) {
int sum = 0;
int i = 0;
while (i < size) {
sum += arr[i];
i++;
}
return sum;
}
This code achieves the same outcome as the for loop but emphasizes clarity in iteration management by using an explicit counter `i`.
Recursive Functions for Summation
Recursion is another powerful technique to compute summation, especially useful for problems that can naturally be divided into smaller subproblems.
Here's how you can implement summation recursively:
int sumRecursive(int arr[], int size) {
if (size <= 0) return 0; // Base case
return arr[size - 1] + sumRecursive(arr, size - 1); // Recursive call
}
In this method, we check for the base case where the size is zero or negative. If not, we return the last element of the array and add it to the recursive call, which reduces the size each time until reaching the base case.
Using the Standard Library Algorithms
C++ offers powerful standard library functions that can streamline summation operations. One such function is `std::accumulate`, which resides in the `<numeric>` header.
Using `std::accumulate` for summation is highly efficient:
#include <numeric>
#include <vector>
int sumWithAccumulate(const std::vector<int>& numbers) {
return std::accumulate(numbers.begin(), numbers.end(), 0);
}
This method is not only concise but also leverages optimizations provided by the C++ standard library, making it a preferred option for many developers.
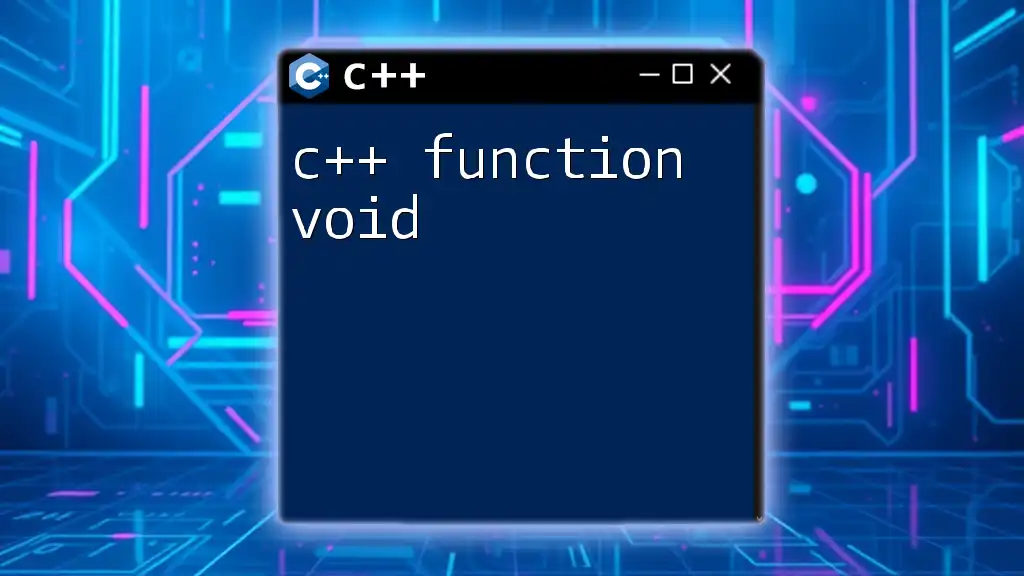
Special Cases of Summation
Summation of Integers
A notable formula in mathematics is that of the summation of the first n integers, expressed as \( S_n = \frac{n(n + 1)}{2} \). This formula can simplify computations greatly as it provides a direct calculation rather than using iterative methods.
Here's how to implement it in C++:
int sumOfFirstN(int n) {
return (n * (n + 1)) / 2;
}
Summation of Series
Summation also plays a critical role in various series, such as arithmetic and geometric series.
For an arithmetic series, where each term increases by a common difference, you can compute the sum as follows:
int sumArithmeticSeries(int n, int a, int d) {
return n / 2 * (2 * a + (n - 1) * d);
}
In this example, `n` represents the number of terms, `a` is the first term, and `d` is the common difference.
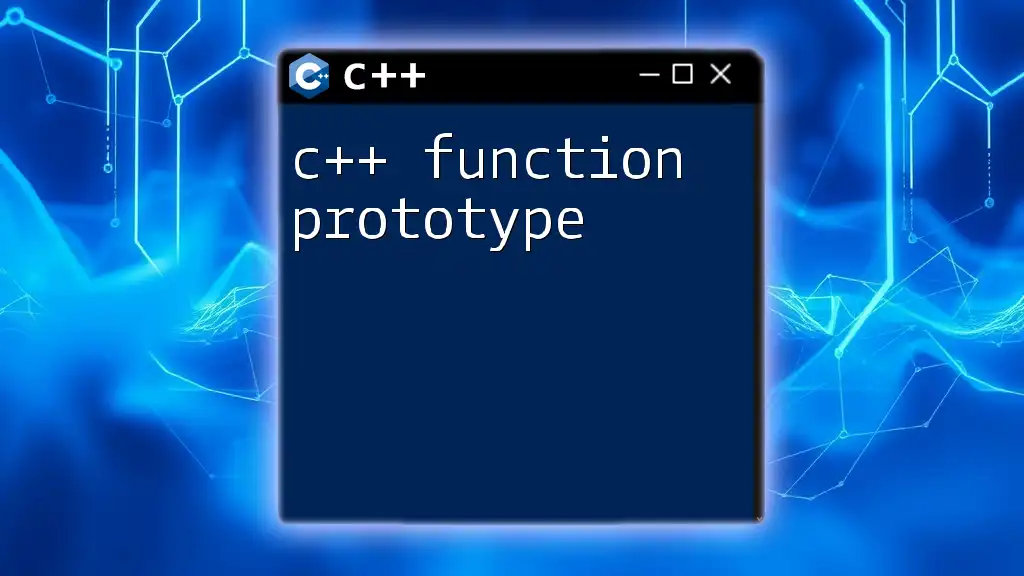
Performance Considerations
Time Complexity of Summation Algorithms
When dealing with summation in C++, it’s essential to consider the time complexity associated with different methods. For conventional iterative methods like loops, the time complexity is \( O(n) \), where n is the number of elements to sum. This means the time taken grows linearly with the number of elements.
However, recursive methods may introduce a higher overhead, especially regarding function call stack space, making them less efficient for large input sizes.
Memory Usage
In terms of memory usage, iterative approaches are generally more space-efficient compared to recursive methods, which may consume considerable stack space especially with deep recursive calls. Therefore, opting for iterative methods for large data sets can lead to better performance.
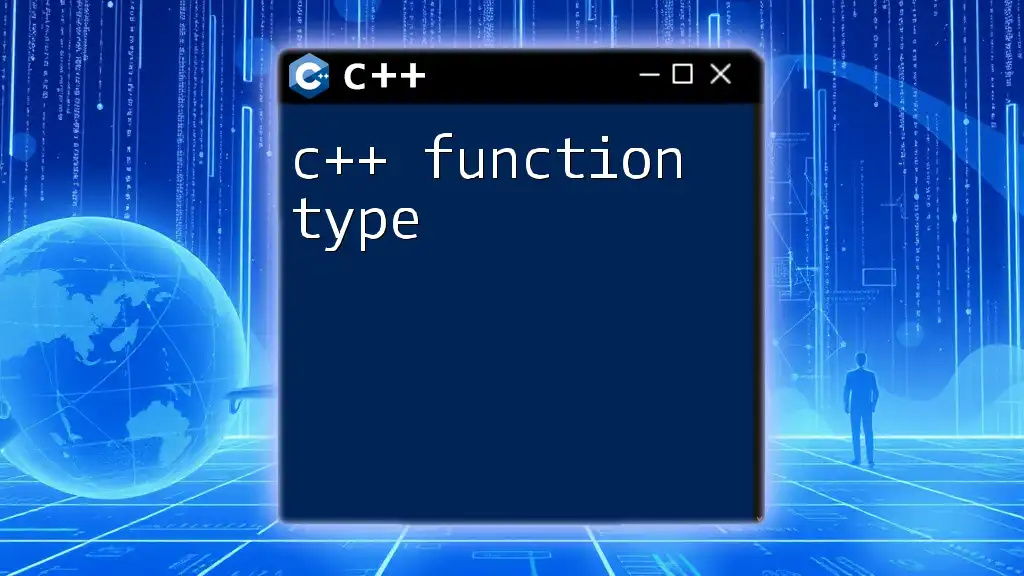
Real-World Applications of Summation in C++
Financial Calculations
In financial calculations, summation is crucial. For instance, to calculate total sales, expenses, or revenue over a certain period, you rely heavily on summation methods. By summing daily transactions, businesses can gain better insights into their performance.
Data Analysis
Summation is also fundamental in data analysis. Whether you are aggregating data for statistical measures (like mean, median) or analyzing trends over time, mastering C++ summation techniques enables you to manipulate data efficiently and accurately.
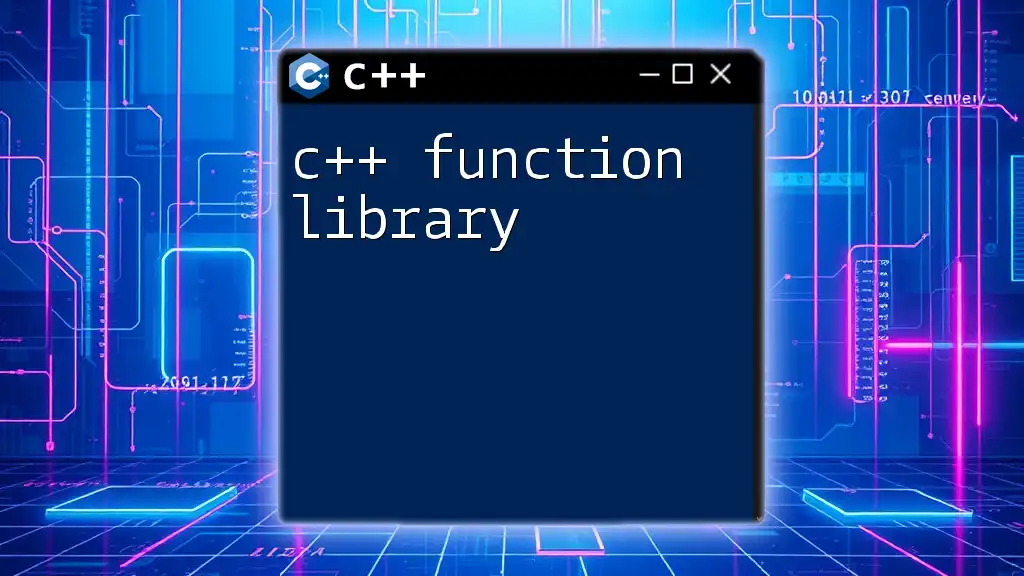
Conclusion
This guide has covered various techniques for performing C++ summation, exploring methods such as loops, recursion, and standard library functions. By understanding these techniques, you can apply them effectively in real-world programming scenarios, whether you're performing data analysis or financial calculations.
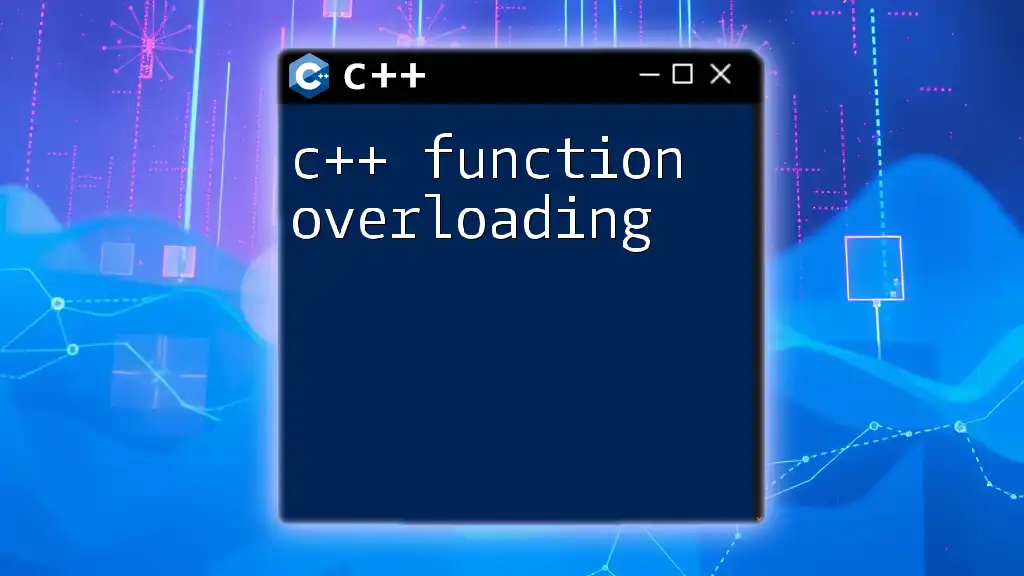
Further Reading and Resources
To deepen your understanding of C++ summation and related topics, consider exploring books and online courses focused on algorithms, data structures, and computational mathematics. Many online platforms also offer coding challenges that can reinforce the concepts covered here.
Stay curious and keep practicing your skills in C++, and you'll find summation techniques becoming second nature in your programming toolkit.