Understanding how to declare and initialize a variable in C++ is essential for beginners; for example, you can define an integer variable as follows:
int number = 5; // Declares an integer variable named 'number' and initializes it with the value 5
Why Are C++ Questions Important?
Mastering C++ through questions and problem-solving is crucial for anyone looking to grasp the intricacies of programming. C++ is widely used in various fields, including system/software development, game development, and performance-critical applications. By engaging with real-world scenarios and common questions, learners can develop their coding skills more effectively.
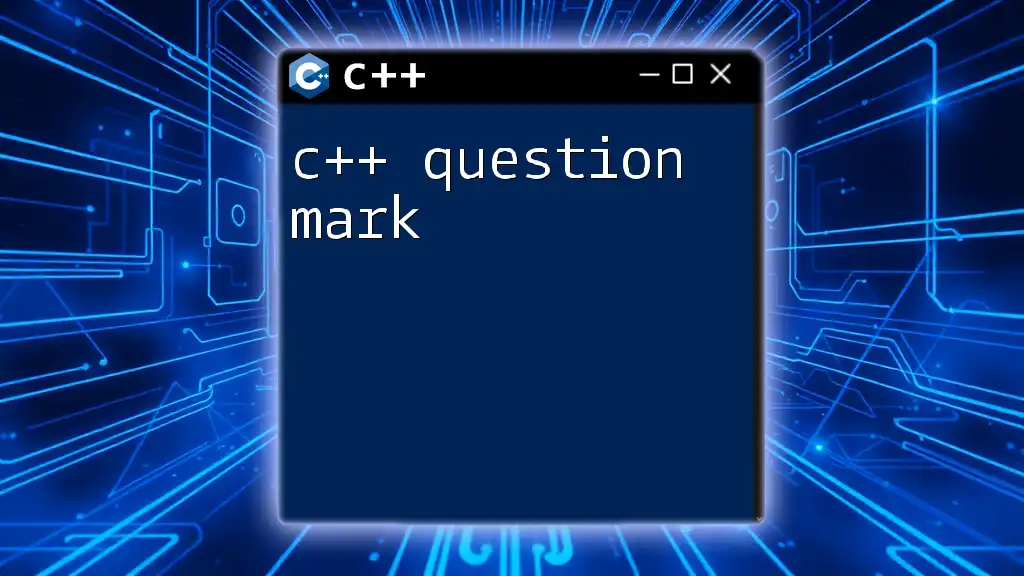
Common C++ Questions and Challenges
Understanding Syntax and Semantics
Syntax questions often arise for beginners grappling with C++. These questions typically relate to basic rules and structure.
One common syntax error is a missing semicolon at the end of a statement. For example:
int main() {
std::cout << "Hello, World!" // Missing semicolon
}
Semantics questions, on the other hand, focus on whether the code does what it is supposed to do. Understanding the difference between syntax (the form of the code) and semantics (the meaning behind it) is essential for debugging and effective programming.
Data Types and Variables
C++ provides several built-in data types like `int`, `float`, `char`, and `bool`. Understanding these types allows programmers to choose the appropriate data representation.
Questions related to variables usually revolve around their scope and lifetime. For instance, a variable declared inside a function is local to that function and cannot be accessed outside of it:
void example() {
int x = 10; // x is local to this function
}
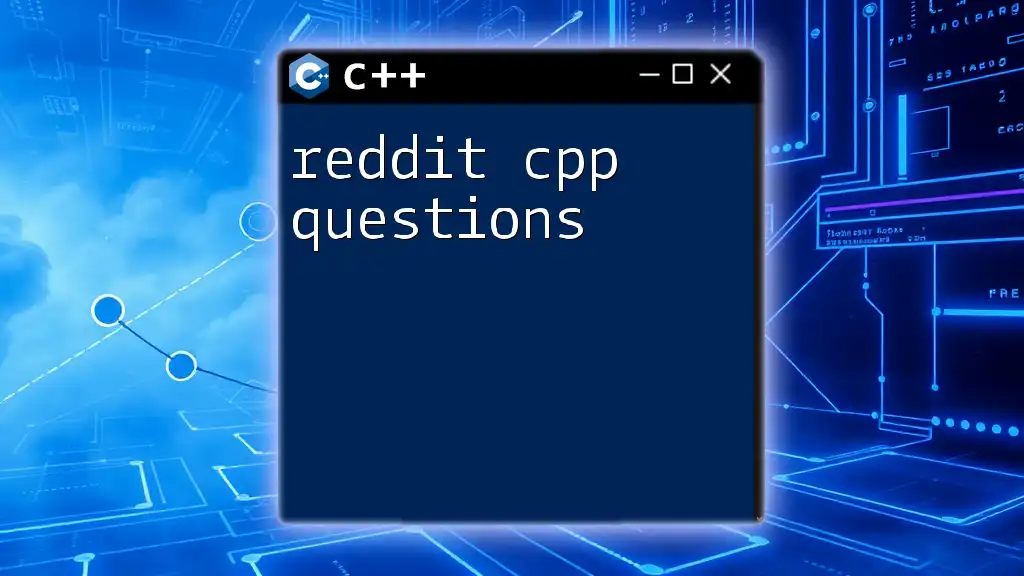
Control Structures in C++
Conditional Statements
Conditional statements, such as if-else statements, are fundamental in controlling the flow of a program. For example:
if (x > 0) {
std::cout << "Positive";
} else {
std::cout << "Non-positive";
}
The choice of using a switch statement over multiple if-else blocks can simplify code readability. A switch statement can handle multiple cases, making it a beneficial tool:
switch(day) {
case 1: std::cout << "Monday"; break;
case 2: std::cout << "Tuesday"; break;
// Other cases
}
Looping Constructs
Looping constructs, namely for, while, and do-while, allow code to execute repeatedly based on conditions. Using a `for` loop often looks like this:
for(int i = 0; i < 5; i++) {
std::cout << i;
}
While and do-while loops are useful when you don't know beforehand how many iterations you'll need. The primary distinction is that the do-while loop guarantees that the block of code will execute at least once:
while(condition) {
// Code block
}
// Do-while example
do {
// Code block
} while(condition);
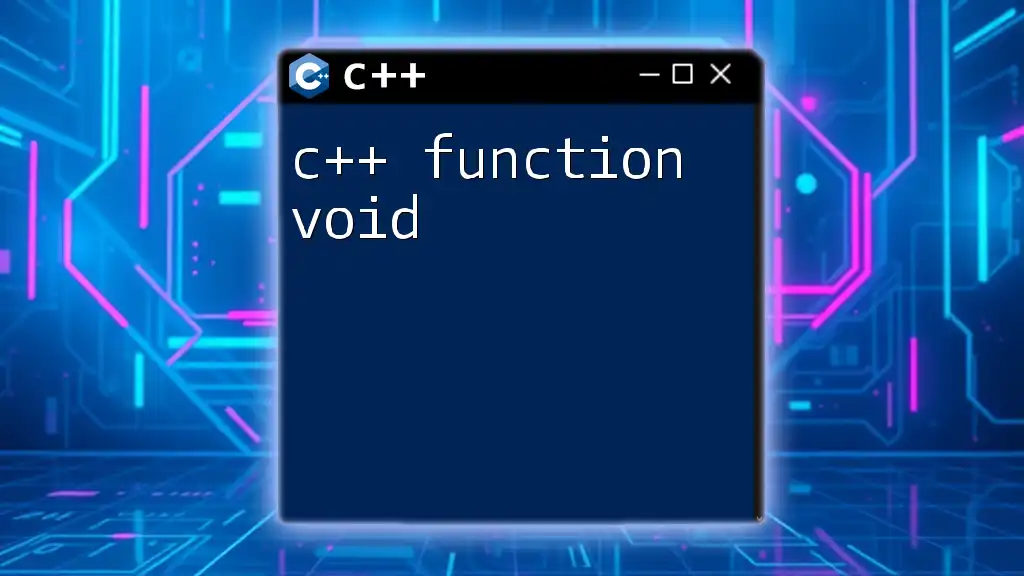
Functions and Their Usage
Defining Functions
Functions encapsulate repetitive tasks and enhance code reusability. A basic function declaration includes the type of output it returns and its parameters:
int add(int a, int b) {
return a + b;
}
Common questions about functions often address the significance of parameters and return types. Understanding how to pass variables into functions and retrieve outputs is vital for any programmer.
Lambda Functions
Lambda functions are a convenient way to define anonymous functions in C++. They can simplify code, especially when used with standard library algorithms. An example of a lambda function is shown below:
auto sum = [](int a, int b) { return a + b; };
With lambda functions, you can create quick, inline functions that improve the efficiency of your code.
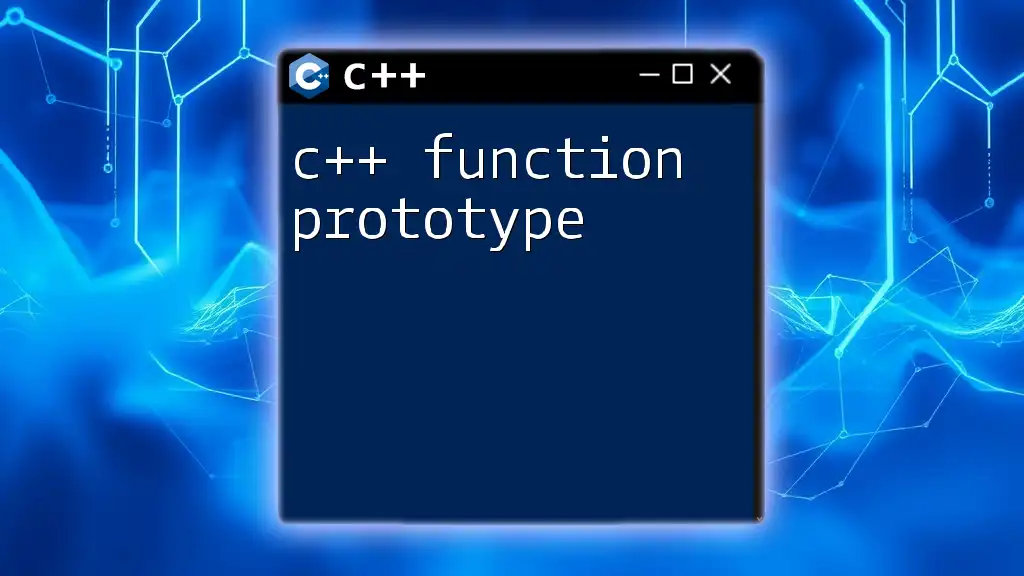
Object-Oriented Programming Questions
Classes and Objects
Classes represent user-defined data types and are a big part of C++'s Object-Oriented Programming paradigm. Here’s a simple class definition:
class Car {
public:
void start() { /* Code to start the car */ }
};
Encapsulation allows classes to hide their internal state and protect it from unintended modifications. Furthermore, inheritance enables new classes to derive properties from existing ones, promoting code reusability.
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. This concept is pivotal in achieving dynamic method resolution. Here is a simple illustration of polymorphism in C++:
class Base {
virtual void show() { std::cout << "Base"; }
};
class Derived : public Base {
void show() override { std::cout << "Derived"; }
};
In this case, the `show()` method behaves differently based on whether the object is of the base class or derived class.
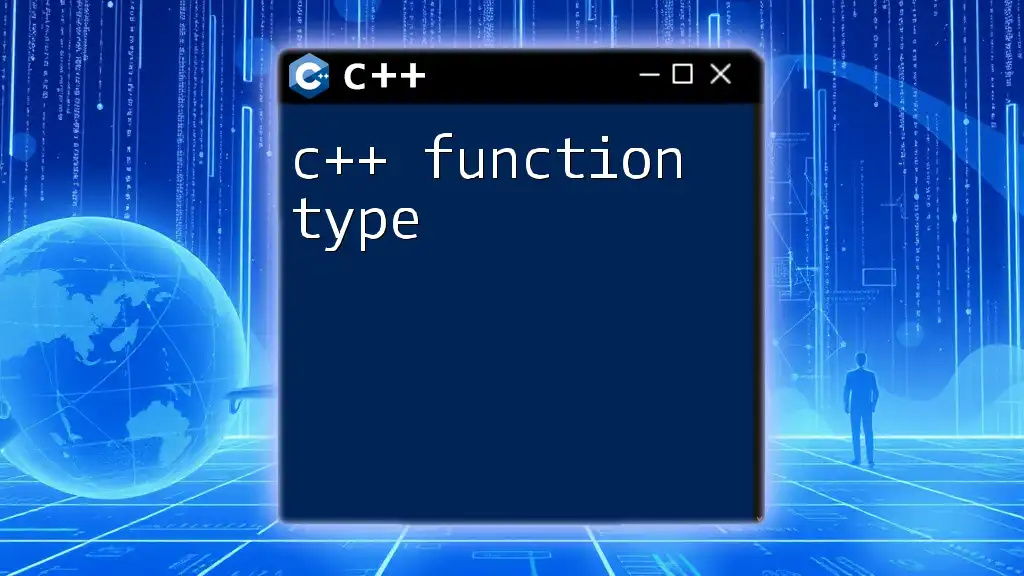
Memory Management in C++
Pointers
Pointers are variables that store the memory address of another variable. They are critical for dynamic memory management in C++. However, they may introduce vulnerabilities if not managed correctly.
int* ptr = new int; // Pointer declaration
This allocates memory for an integer but requires proper care and cleanup to avoid memory leaks.
Dynamic Memory Allocation
Dynamic memory allocation using the `new` operator allows programmers to allocate memory at runtime. Equally important is the use of the `delete` operator to avoid memory leaks:
int* arr = new int[10];
delete[] arr; // Proper memory cleanup
Failing to deallocate memory properly can lead to serious performance issues and instability in applications.
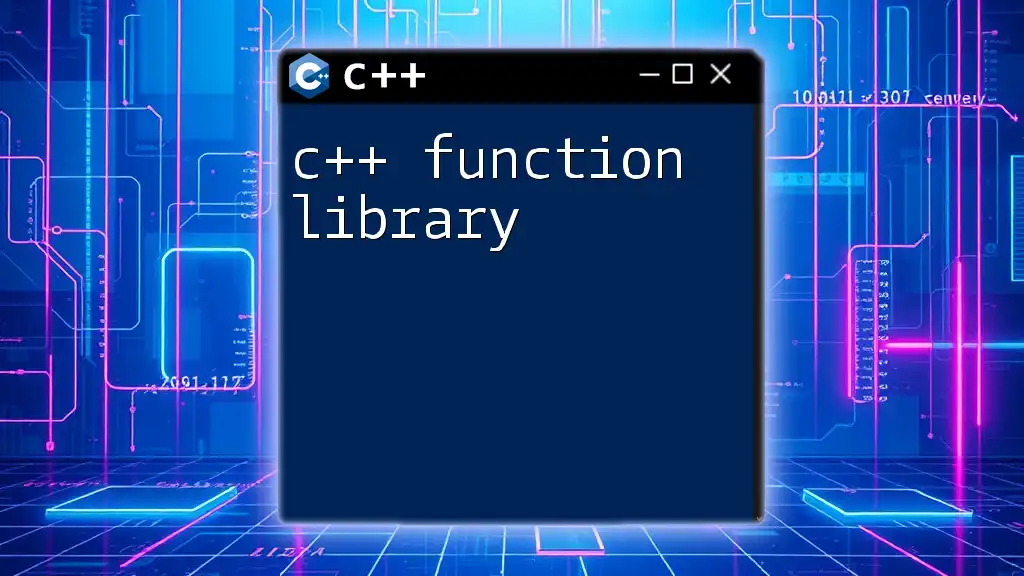
Conclusion
Engaging with C++ questions helps reinforce learning and solidifies understanding of core programming concepts. By exploring common topics and challenges in C++, programmers can enhance their skills, leading to better problem-solving abilities. To maximize your C++ proficiency, consider practicing consistently and applying these concepts in real-world scenarios. There are numerous resources available for further learning, including books, online courses, and coding challenges that can boost your programming journey.