A C++ function alias allows you to create a new name for an existing function, making it easier to use or improving code readability.
#include <iostream>
// Original function
void greet() {
std::cout << "Hello, World!" << std::endl;
}
// Function alias
using Hello = void(*)();
int main() {
Hello greeting = greet; // Creating an alias
greeting(); // Using the alias to call the function
return 0;
}
What is a C++ Function Alias?
A C++ function alias serves as an alternate name for a function, making it easier to reference and manipulate within code. Function aliases can greatly enhance code readability and promote the reuse of functionalities, especially when dealing with complex function signatures.
Distinctive Features of Function Aliases
- Simplification of Code: Instead of writing out long function pointer types repeatedly, developers can use aliases to create shorter, more manageable names.
- Improved Readability: Code becomes easier to understand when developers can express their intentions succinctly with meaningful names.
- Encouragement of Code Reuse: Aliases allow for greater modularity, encouraging developers to reuse functions without needing to redefine complex types.
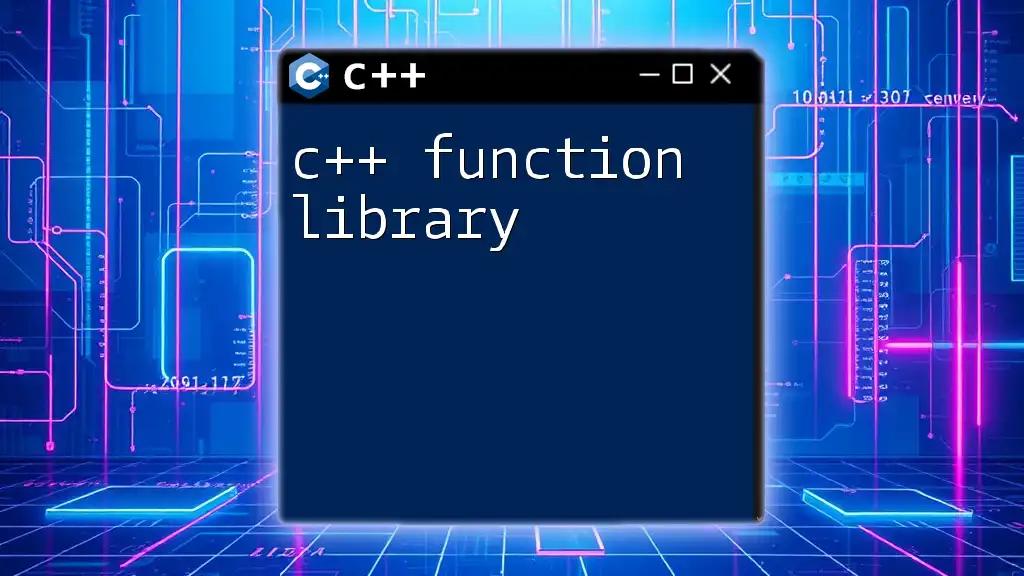
How to Create a Function Alias
Using `using` Declaration
One of the most straightforward ways to create a function alias in C++ is through the `using` keyword. This method simplifies function pointer declarations and enhances code clarity.
Syntax Structure:
using AliasName = FunctionType;
Example: Simple function alias using `using`:
using FuncAlias = void(*)(int);
void sampleFunction(int x) {
// implementation
}
FuncAlias myFunc = sampleFunction;
In this example, `FuncAlias` serves as an alias for any function that takes an `int` parameter and returns `void`. This allows for easy manipulation of function pointers without repeating the entire declaration each time.
Using Typedefs
Traditionally, C++ developers have utilized the `typedef` keyword to create function aliases. Although `typedef` is considered somewhat outdated compared to `using`, it remains prevalent in legacy codebases.
Syntax Structure:
typedef FunctionType AliasName;
Example: Creating a function alias with typedef:
typedef void (*FuncType)(double);
void anotherFunction(double y) {
// implementation
}
FuncType anotherFunc = anotherFunction;
This example employs `typedef` to create an alias called `FuncType`, which represents a pointer to any function that takes a `double` parameter and returns `void`.
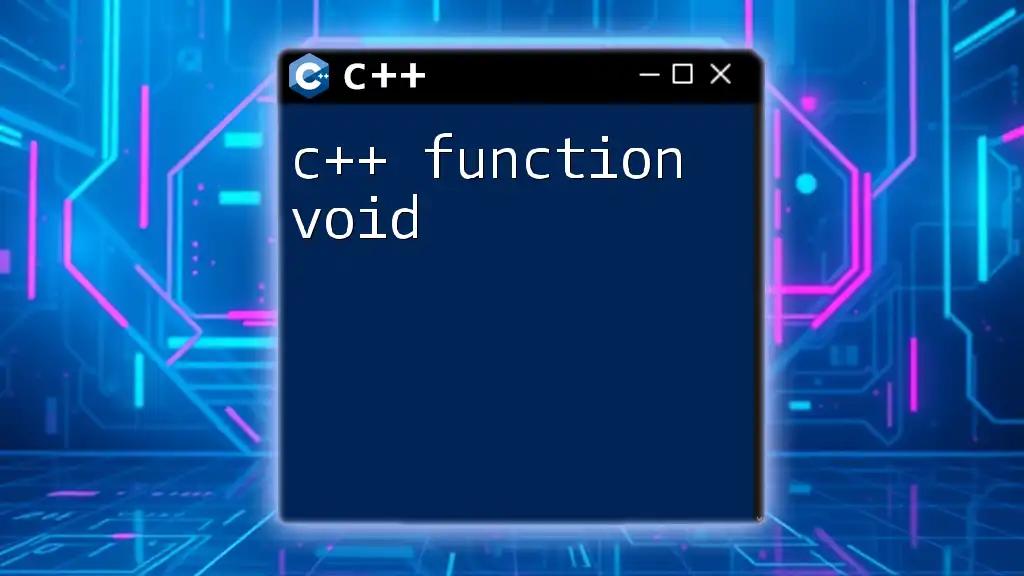
Practical Applications of Function Aliases
Enhancing Code Clarity
Function aliases can significantly contribute to code clarity, especially in scenarios where functions serve as callbacks. The representation of complex function pointer types can be simplified, allowing developers to focus more on the logic rather than the syntax.
Example: Using function aliases in callbacks:
using Callback = void (*)(int);
void callbackFunction(int z) {
// implementation
}
void registerCallback(Callback cb) {
// implementation
}
In this scenario, the alias `Callback` defines a specific function signature, making it easy to declare and register callback functions without confusion.
Simplifying Function Pointer Declarations
When dealing with lengthy function pointer types, using aliases provides a clear benefit by decluttering declarations and making them easier to manage and read. This practice is especially useful in complex projects where multiple functions may share similar signatures.
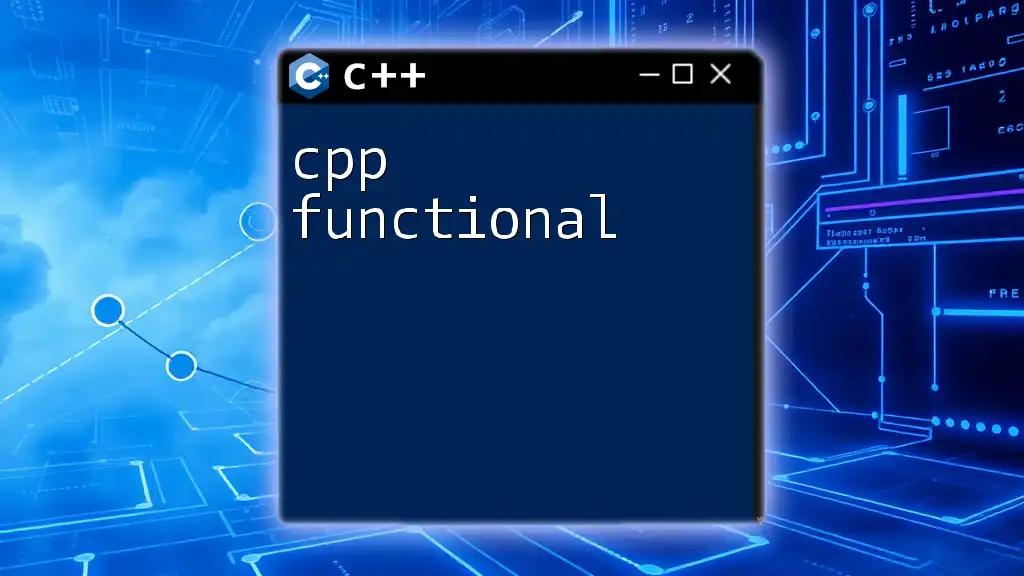
Risks and Limitations of Using Function Aliases
Potential Confusion
While function aliases can enhance clarity, overusing them can lead to confusion. If a codebase has many aliases, developers may struggle to remember what each alias represents, thereby negating some benefits of using them in the first place.
Debugging Challenges
Function aliases may complicate debugging. If an alias is misconfigured or improperly referenced, it could lead to runtime errors that are harder to trace back to their source. Understanding the actual underlying type rather than the alias can be crucial during debugging sessions.

Advanced Usage of Function Aliases
Function Aliases in Templates
Function aliases can also be leveraged within templates, which adds versatility and abstraction to the code.
Example: Templated function alias:
template<typename T>
using FuncTemplate = void(*)(T);
In this instance, `FuncTemplate` dynamically resolves to a function pointer type based on the template argument `T`, allowing for a flexible and generic coding practice.
Combining Function Aliases with Lambda Expressions
Function aliases can be effectively utilized with lambda expressions, enhancing the functionality and usability of callbacks and custom operations.
Example: Function alias for a lambda:
using LambdaFunc = std::function<void(int)>;
LambdaFunc myLambda = [](int a) {
// implementation
};
By wrapping a lambda expression with `std::function`, developers can create an alias that allows them to easily handle function-like objects, maintaining the benefits of type safety and closure.

Best Practices for Using C++ Function Aliases
When to Use vs. When to Avoid
It’s essential to assess when it’s appropriate to employ function aliases. While they are immensely beneficial in simplifying complex function declarations, overusing them may introduce unnecessary complexity. Developers should consider clarity and the audience of their code, ensuring that function aliases serve to improve understanding rather than impede it.
Documenting Function Aliases
Proper documentation of function aliases is critical. Each alias should be accompanied by comments that explain its purpose and usage. This practice not only aids in maintainability but also contributes to team collaboration, where different team members might interact with the code over time.

Conclusion
In summary, C++ function aliases offer a powerful tool for enhancing code readability, promoting reuse, and simplifying complex declarations. However, like any programming construct, they must be used judiciously to avoid confusion. Exploring function aliases can lead to cleaner and more efficient code, making it an invaluable practice for both beginner and seasoned C++ developers.
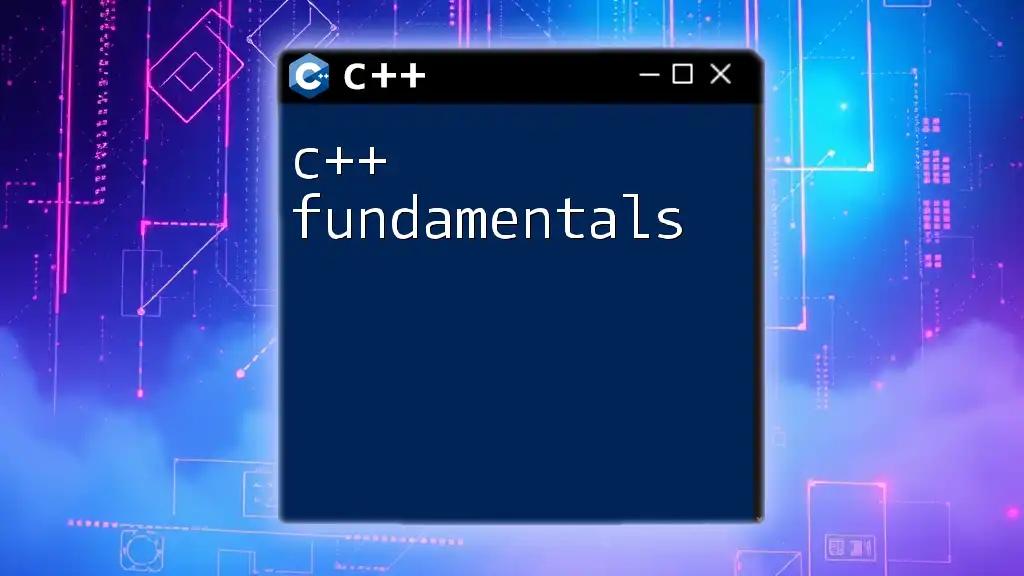
Additional Resources
For further exploration, developers can delve into articles, tutorials, and official documentation that discuss C++ functions, pointers, and best coding practices. Engaging with the broader programming community can yield beneficial insights and techniques related to function aliasing and other functionalities within C++.