In C++, you can define functions within structs to encapsulate both data and behavior, allowing for a more organized structure where related functionalities are grouped together.
Here's a simple example:
#include <iostream>
struct Rectangle {
int width, height;
int area() {
return width * height;
}
};
int main() {
Rectangle rect;
rect.width = 5;
rect.height = 3;
std::cout << "Area: " << rect.area() << std::endl; // Output: Area: 15
return 0;
}
What are Structs in C++?
Structs, short for structures, are a user-defined data type in C++ that group related variables under a single name. They are particularly useful for creating complex data types that can encapsulate multiple properties.
Basic Syntax of a Struct in C++
The syntax for defining a struct involves the `struct` keyword, followed by the struct name and a set of curly braces containing the member variables. Here’s a simple example:
struct Person {
std::string name;
int age;
};
In this example, `Person` is a struct containing two member variables: `name` and `age`.
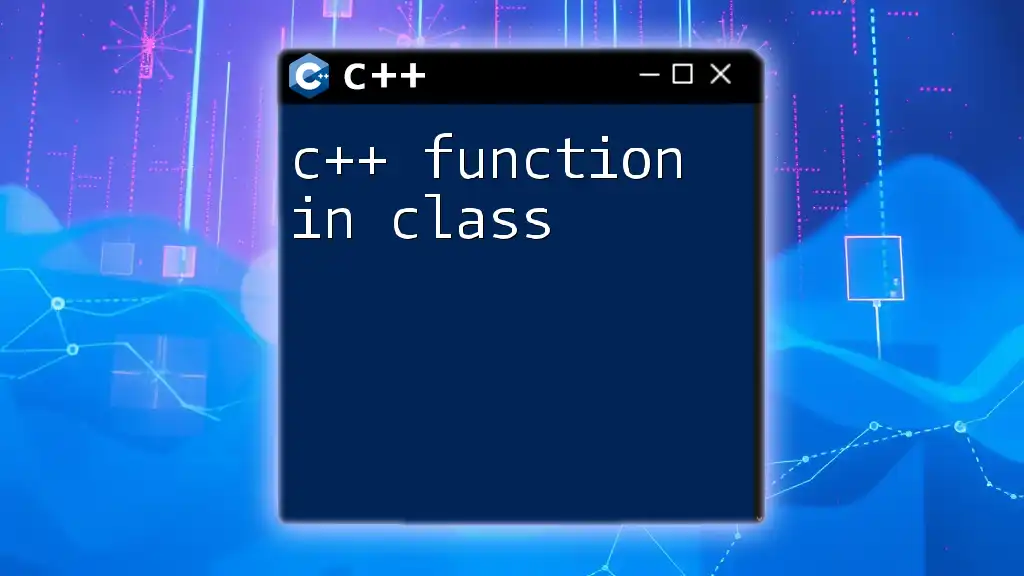
The Role of Functions in C++
Functions play a crucial role in C++ programming. They are blocks of code designed to perform a specific task, which can be called upon multiple times throughout your program, thereby reducing redundancy. Using functions aids maintainability and clarity in your code.
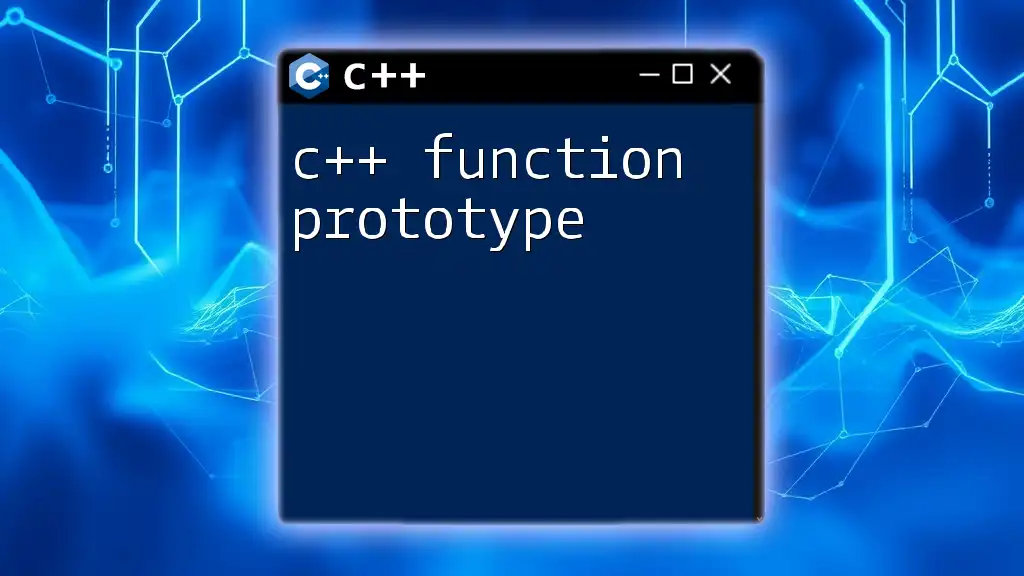
Understanding C++ Functions in Structs
Defining Functions within Structs
In C++, you can define functions inside a struct, thereby allowing you to associate behaviors directly with the data. These functions are known as member functions. Here’s how you can define a function within a struct:
struct Rectangle {
int width;
int height;
// Member function to calculate area
int area() {
return width * height;
}
};
In this example, the `Rectangle` struct has a member function called `area()`, which calculates and returns the area of the rectangle based on its `width` and `height`.
Importance of Member Functions in Structs
Member functions enhance the encapsulation of data by allowing you to operate on the member variables directly. This leads to more organized and modular code. They enable data abstraction by hiding the implementation details and exposing a simple interface that other parts of your program can use.
Member Functions vs. Global Functions
A key difference between member functions and global functions lies in their association with data. Member functions belong to a specific instance of a struct, while global functions operate independently.
Consider the following example comparing the two:
struct Circle {
double radius;
// Member function
double area() {
return 3.14 * radius * radius;
}
};
// Global function
double globalCircleArea(double radius) {
return 3.14 * radius * radius;
}
In this case, the `area()` function is a member function tied specifically to a `Circle` instance, while `globalCircleArea()` operates independently, taking the radius as an argument. Utilizing member functions often leads to clearer and easier-to-understand code when working with data types.
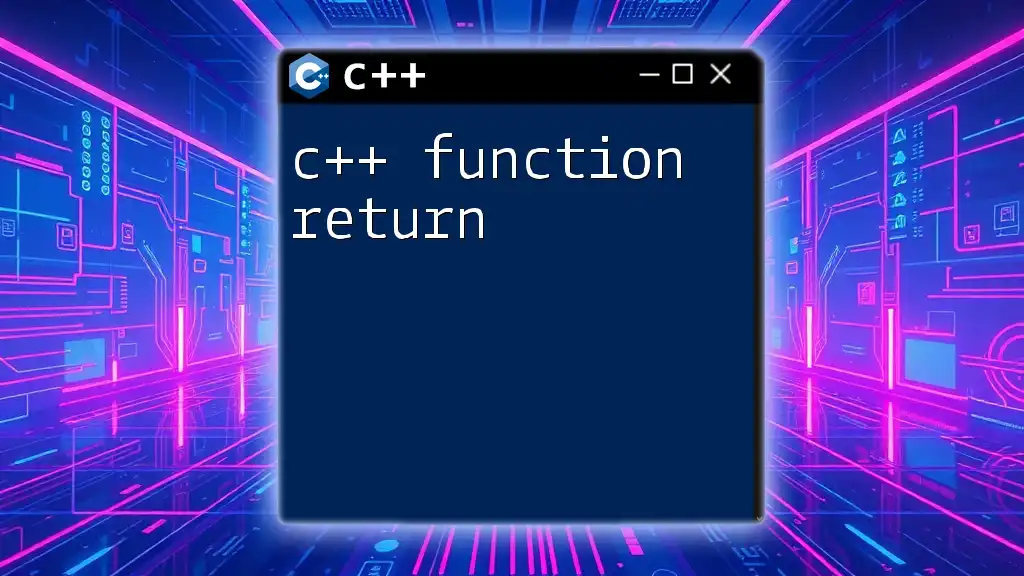
Struct Function C++: Practical Applications
Use Case: Structs with Member Functions
Structs combined with member functions are incredibly versatile. Consider a scenario where you want to model a `Car`.
struct Car {
std::string brand;
int year;
void startEngine() {
std::cout << brand << " engine started." << std::endl;
}
};
In this example, the `Car` struct includes a `startEngine` member function that outputs a message indicating the car’s engine has started. This encapsulation provides clarity and allows you to group both data and related behavior.
Using Operator Overloading in Structs
Operator overloading allows you to define how certain operators behave with user-defined types. This is particularly useful in structs when you want to define custom behavior for operations like addition or subtraction. Here’s an example of overloading the `+` operator for a `Vector` struct:
struct Vector {
int x, y;
// Operator overloading for the + operator
Vector operator+(const Vector& v) {
return {x + v.x, y + v.y};
}
};
This example allows you to add two `Vector` instances using the `+` operator. You could write:
Vector v1 = {2, 3};
Vector v2 = {5, 7};
Vector result = v1 + v2; // result will be (7, 10)
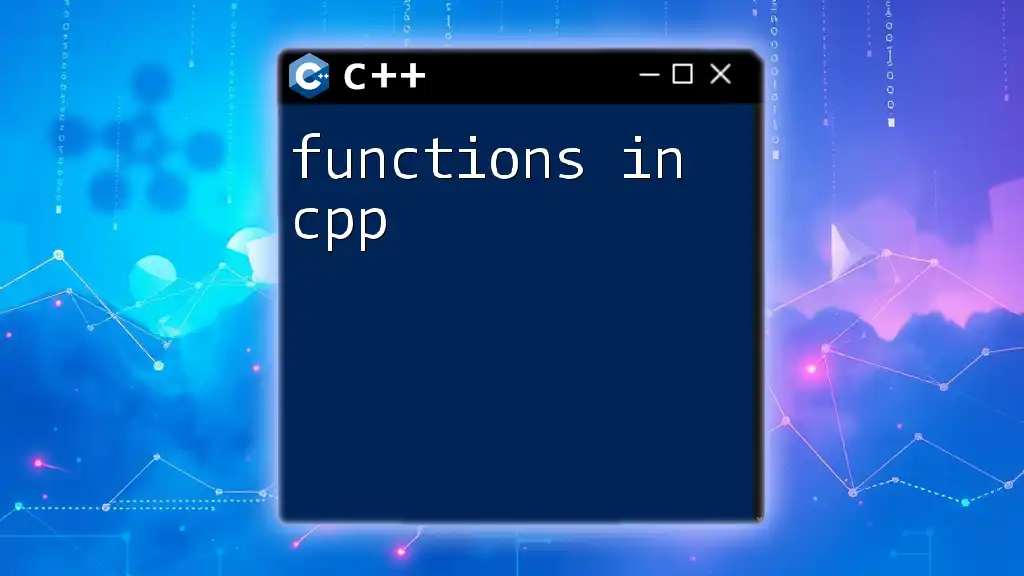
Best Practices for Functions in Structs
Coding Conventions and Readability
When defining functions within structs, clarity and readability should be your top priority. Use descriptive names for both structs and their member functions to clearly communicate their purpose. Consistent naming conventions can significantly enhance understanding for anyone reviewing your code.
When to Use Structs with Functions
Opt for structs with functions when you need to associate behavior directly with data or when dealing with related data types. This approach leads to better-organized code. However, be cautious of performance implications and unnecessary complexity. It's essential to ensure that using a struct in this manner makes logical sense for your application's requirements.
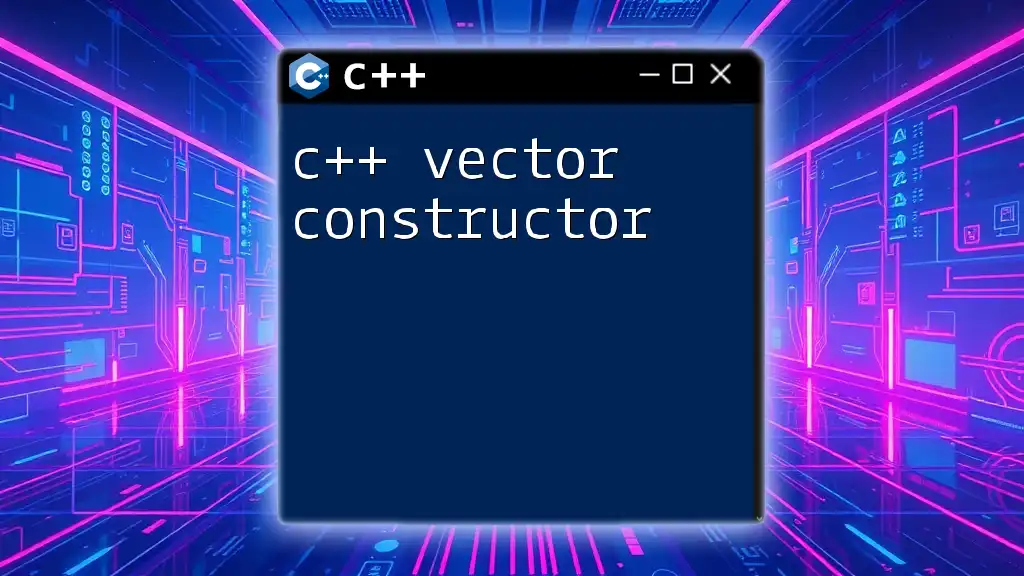
Conclusion
Understanding how to use C++ functions in structs opens up a world of modular programming techniques and data organization. Structs not only allow for the grouping of related data but also enable the encapsulation of methods that manipulate that data.
By encapsulating behavior within structs, you can enhance both the maintainability and readability of your code. As you practice coding with functions in structs, consider applying these principles to real-world scenarios, making your programs easier to understand and manage.
For further exploration of C++ programming concepts and best practices, continuous learning and hands-on practice are not just recommended—they are essential for mastery.