In C++, a function can be passed as a parameter to another function, allowing for higher-order programming and function customization.
Here’s a simple example demonstrating this concept:
#include <iostream>
using namespace std;
void process(int x, void (*func)(int)) {
func(x);
}
void square(int x) {
cout << x * x << endl;
}
int main() {
process(5, square); // Outputs: 25
return 0;
}
Understanding Functions in C++
What is a Function?
A function is a block of code designed to perform a specific task. Functions enable modularity, allowing you to break down a complex problem into smaller, manageable pieces. The syntax of a simple C++ function looks like this:
return_type function_name(parameter_list) {
// function body
}
Types of Functions
Functions can be categorized into two main types:
- Built-in Functions: These are predefined functions provided by C++ libraries, such as `cout` and `cin` for input and output.
- User-defined Functions: Functions created by the programmer for specific tasks. They provide customization, allowing for tailored solutions in your code.
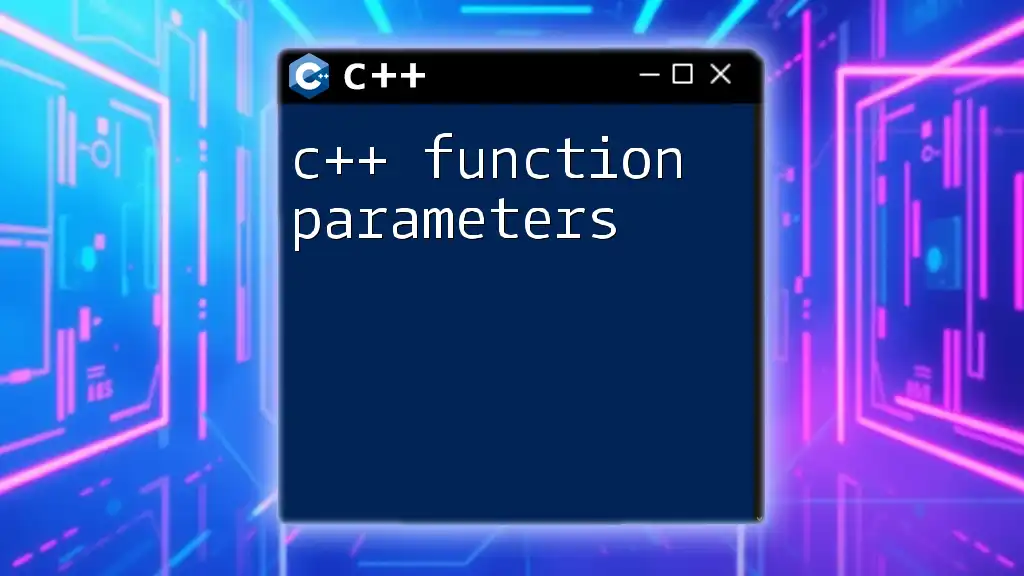
Functions as First-Class Citizens
Definition of First-Class Functions
First-class functions are functions that can be treated like any other variable. This means they can be passed as arguments to other functions, returned from functions, and assigned to variables. In C++, functions achieve first-class status primarily through function pointers and references.
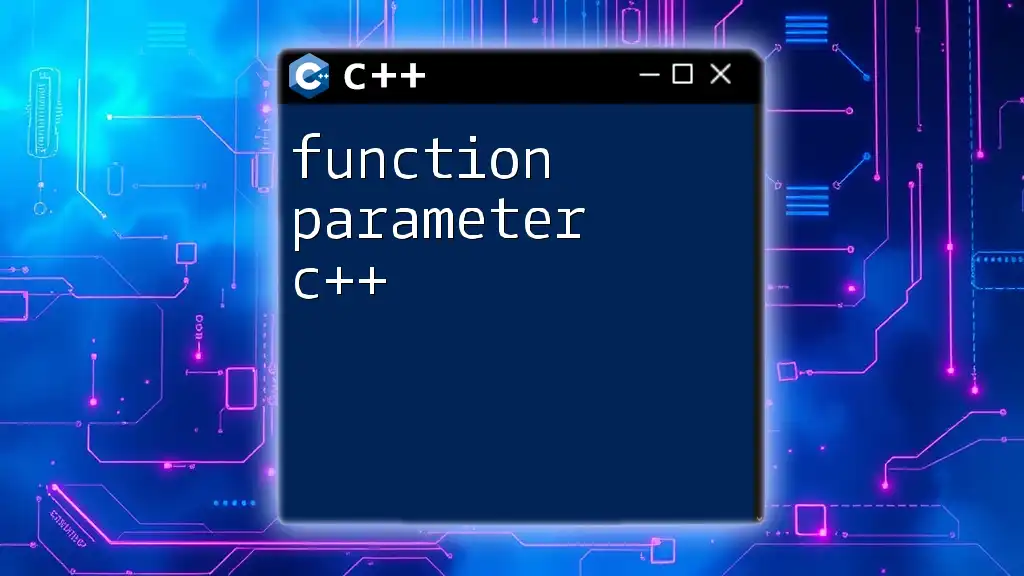
Passing Functions as Arguments
Why Pass Functions as Arguments?
Passing functions as arguments can help enhance the flexibility and reusability of your code. This allows you to define the behavior of your functions at runtime, which is particularly useful in scenarios like callbacks or custom sorting.
C++ Passing Function as Parameter
In C++, you can pass a function as a parameter to another function. The syntax for doing so generally involves using function pointers. This involves specifying the type of the function pointer, including its return type and parameters.
Function Pointer
What is a Function Pointer?
A function pointer is a variable that can store the address of a function. It allows you to call the function indirectly. Here’s how you can declare a function pointer:
return_type (*pointer_name)(parameter_type);
For example, to declare a pointer to a function that takes two integers and returns an integer:
int (*operation)(int, int);
Syntax of Function Pointers in C++
Declaring and using function pointers can be succinctly expressed. Here’s a small example:
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b;
}
int main() {
int (*funcPtr)(int, int) = add;
cout << "Sum: " << funcPtr(2, 3) << endl; // Outputs: Sum: 5
return 0;
}
How to Pass Function as Argument in C++
Passing Function Pointers
To pass function pointers to another function, you define the parameter type as a pointer to the function type. Here’s a detailed example:
#include <iostream>
using namespace std;
void printMessage(const char* message) {
cout << message << endl;
}
void executeFunction(void (*func)(const char*), const char* msg) {
func(msg);
}
int main() {
executeFunction(printMessage, "Hello, World!");
return 0;
}
In this example:
- `executeFunction` takes a function pointer `func` as an argument and a string message `msg`.
- It then calls the provided function with the message.
Passing Member Functions
Passing member functions in C++ requires a slightly different approach because you need to account for the class context. An effective way to manage this is to use `std::function` and `std::bind`, which simplify the handling of member functions.
Using std::function to Pass Functions
What is std::function?
`std::function` is a general-purpose polymorphic function wrapper in C++. It can encapsulate any callable target, which can be a free function, a lambda, or a member function.
Example of std::function
Here’s how you can encapsulate functionality with `std::function`:
#include <iostream>
#include <functional>
using namespace std;
void operateOnNumber(int x, function<void(int)> func) {
func(x);
}
int main() {
operateOnNumber(5, [](int n) { cout << "Number: " << n << endl; });
return 0;
}
In this case, `operateOnNumber` receives an `int` and a `std::function` as parameters, enabling the flexibility to use any function that matches the expected signature.
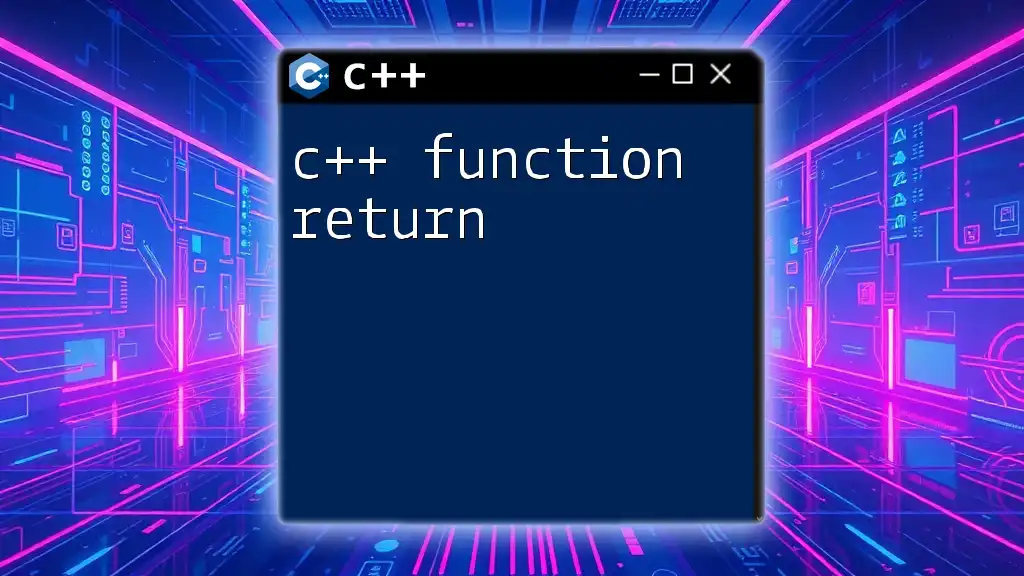
Practical Applications
Using Functions as Callbacks
Callbacks play a crucial role in asynchronous programming in C++. A callback function is a function that is passed to another function as an argument and is executed after a certain event occurs. This pattern is particularly prevalent in event-driven programming, such as GUI applications.
Custom Sorting Functions
Functions as parameters can be particularly useful when implementing custom sorting strategies using `std::sort`. The standard library allows you to pass custom functions as comparators. Here’s an example:
#include <algorithm>
#include <vector>
#include <iostream>
using namespace std;
bool compare(int a, int b) {
return a > b;
}
int main() {
vector<int> nums = {2, 1, 4, 3};
sort(nums.begin(), nums.end(), compare);
for (int num : nums) {
cout << num << " "; // Outputs: 4 3 2 1
}
return 0;
}
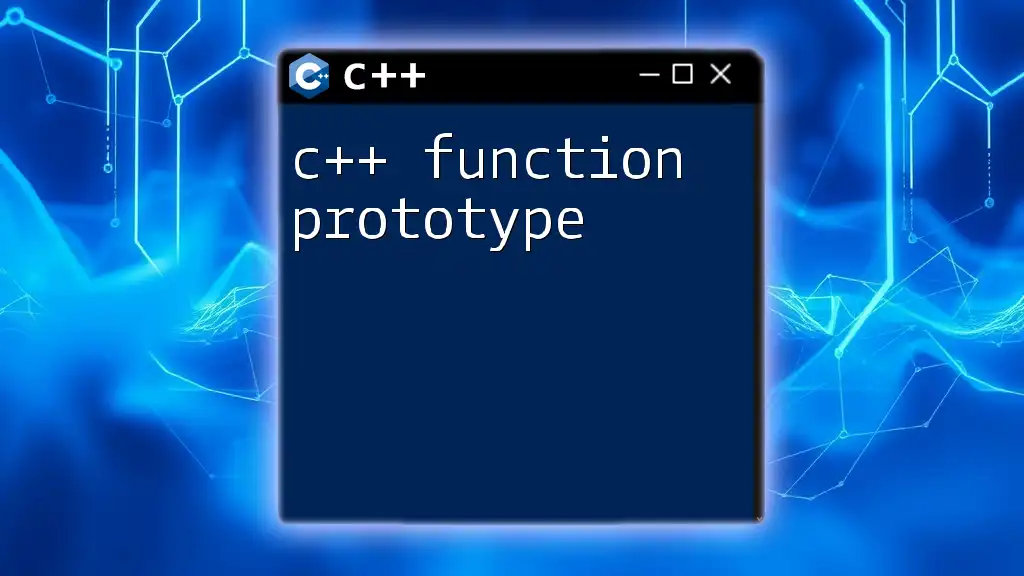
Best Practices
When to Use Function Parameters
Using functions as parameters can dramatically increase the flexibility and maintainability of your code. However, it’s crucial to be mindful of proper usage patterns. Avoid passing functions that depend on local variables in the calling scope to prevent undefined behavior.
Performance Considerations
The choice between function pointers, functors, and lambdas can impact performance. While function pointers offer simplicity, modern C++ encourages the use of `std::function` for its versatility despite the minor overhead it introduces. It’s good practice to measure performance in the context of your specific application.
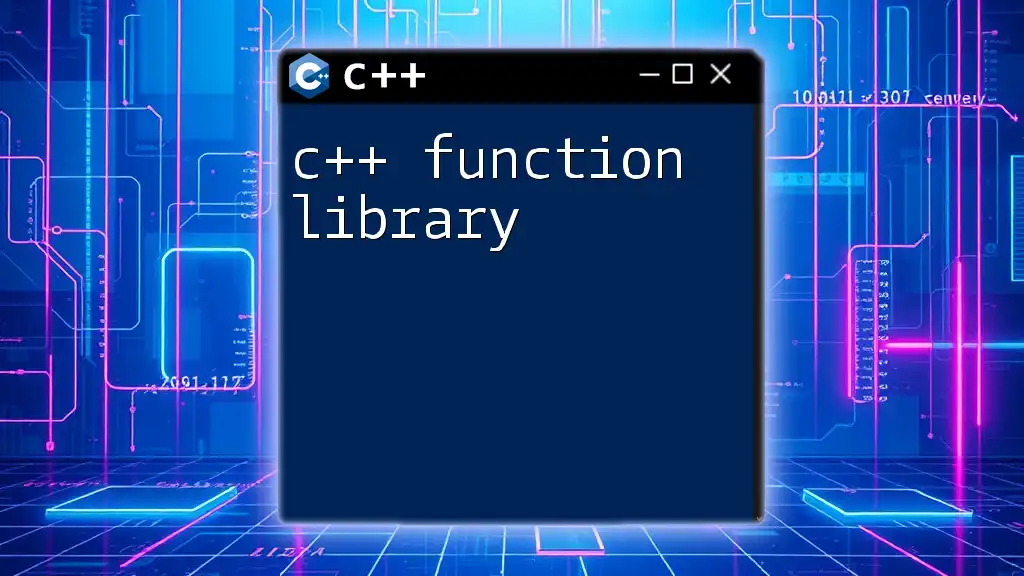
Conclusion
The concept of a C++ function as parameter introduces immense flexibility in coding, facilitating advanced programming techniques like callbacks and custom sorting. By mastering this concept, you enhance your ability to create modular, reusable, and clean code. Practicing these principles will allow you to fully leverage the power of functions within the C++ programming language. As you advance, explore more intricate topics such as functors and lambda expressions to deepen your understanding of function manipulation in C++.
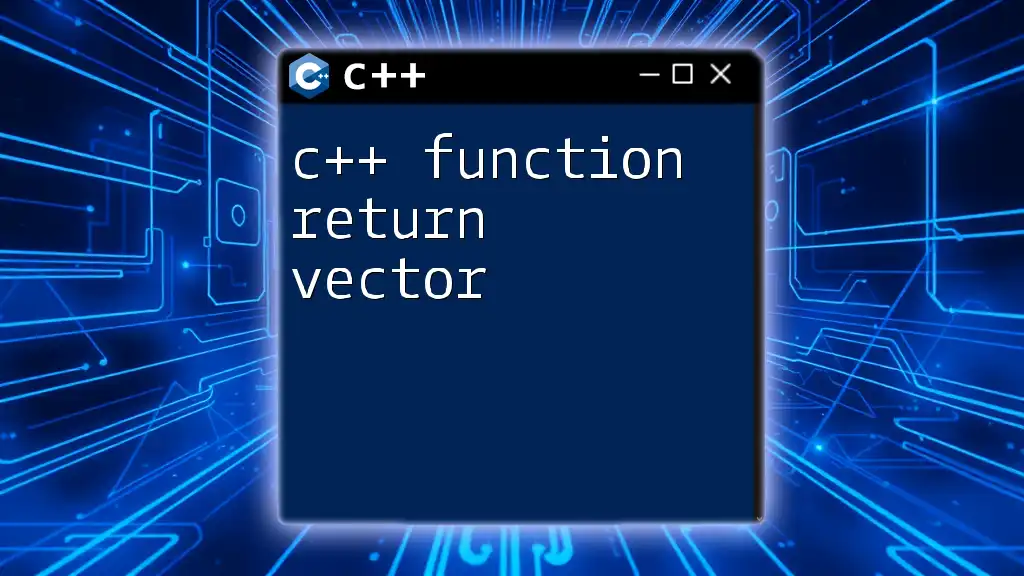
Additional Resources
For those wanting to further explore C++ functions and their parameters, consider exploring programming textbooks or online resources dedicated to advanced C++ topics. Create sample projects implementing these concepts to solidify your understanding and boost your skills.