In C++, function parameters can be passed by value or by reference, enabling different behaviors in how changes to the parameters affect the original variables.
Here's a code snippet illustrating both styles:
#include <iostream>
void byValue(int x) {
x = x + 10;
}
void byReference(int &y) {
y = y + 10;
}
int main() {
int a = 5;
int b = 5;
byValue(a); // 'a' remains 5
byReference(b); // 'b' changes to 15
std::cout << "a: " << a << ", b: " << b << std::endl; // Output: a: 5, b: 15
return 0;
}
Types of Function Parameter Passing
Pass by Value
Passing parameters by value means that a copy of the variable is made when it is passed to the function. Any changes made to the parameter inside the function do not affect the original variable.
When to use pass by value:
- Suitable for small data types (like integers or chars) where performance is not a major concern.
- Provides safety since the original data remains unchanged.
Example:
void passByValue(int x) {
x = x + 10;
std::cout << "Inside function: " << x << std::endl;
}
int main() {
int a = 5;
passByValue(a);
std::cout << "Outside function: " << a << std::endl; // Output: 5
return 0;
}
Explanation of Example: In this example, when the variable `a` is passed to `passByValue`, a copy is made. The original variable remains unchanged even though it is modified within the function.
Pass by Reference
Passing by reference allows the function to operate directly on the original variable by creating an alias to it. Any changes made to the parameter will affect the actual argument.
When to use pass by reference:
- When you want to avoid copying large objects, thus improving performance.
- When you need to modify the original variable.
Example:
void passByReference(int &x) {
x = x + 10;
std::cout << "Inside function: " << x << std::endl;
}
int main() {
int a = 5;
passByReference(a);
std::cout << "Outside function: " << a << std::endl; // Output: 15
return 0;
}
Explanation of Example: Here, the function `passByReference` modifies the original variable `a` since `x` is a reference to `a`. Changes made within the function are reflected outside of it.
Pass by Pointer
When passing by pointer, the address of the variable is passed to the function. This allows for direct manipulation of the original variable through pointer dereferencing.
When to use pass by pointer:
- Useful when dealing with dynamic memory allocation, as it allows flexibility in memory management.
- Essential when you need to indicate that a function can modify the passed variable.
Example:
void passByPointer(int *x) {
*x = *x + 10;
std::cout << "Inside function: " << *x << std::endl;
}
int main() {
int a = 5;
passByPointer(&a);
std::cout << "Outside function: " << a << std::endl; // Output: 15
return 0;
}
Explanation of Example: In this case, we pass the address of `a` to `passByPointer`. The function dereferences the pointer to modify the original value. Thus, the change is reflected outside of the function.
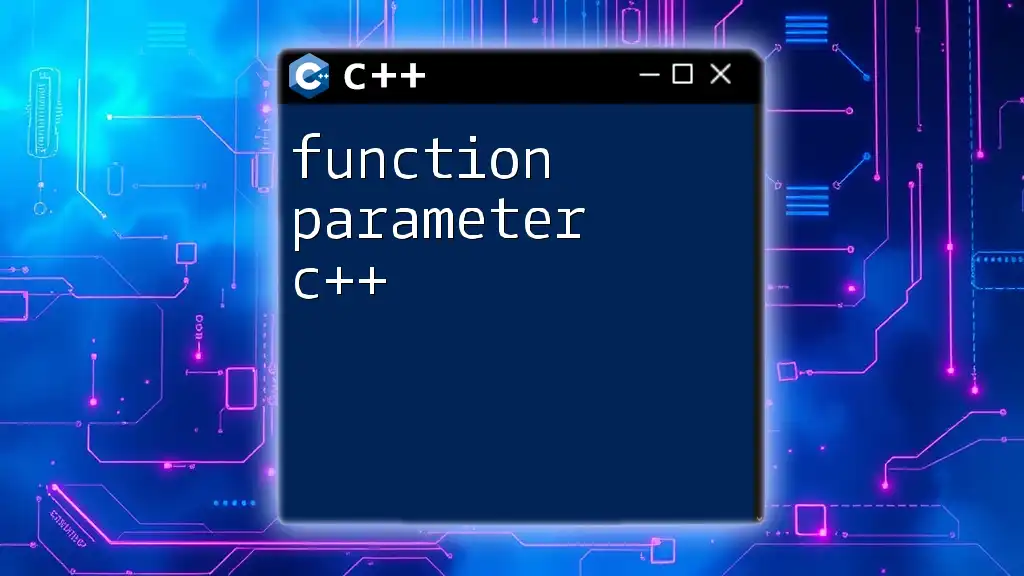
Comparison of Parameter Passing Methods
Pros and Cons of Pass by Value
-
Advantages:
- It prevents modification of original data, reducing risks of unintended side effects.
- Simpler to understand as no additional syntax (like pointers) is required.
-
Disadvantages:
- Performance overhead can be significant for large data objects because it involves copying the entire object.
Pros and Cons of Pass by Reference
-
Advantages:
- Efficient for large objects since it only passes a reference, reducing the overhead.
- Facilitates modification of the original variable directly.
-
Disadvantages:
- Risk of unintended changes to the original variable, which can introduce bugs if not managed carefully.
Pros and Cons of Pass by Pointer
-
Advantages:
- Offers maximum flexibility with dynamic memory management.
- Can manage arrays and dynamic data structures efficiently.
-
Disadvantages:
- Involves additional complexity in syntax and semantics, such as risk of null pointer dereferencing.
- Increased chance of memory leaks if not managed properly.
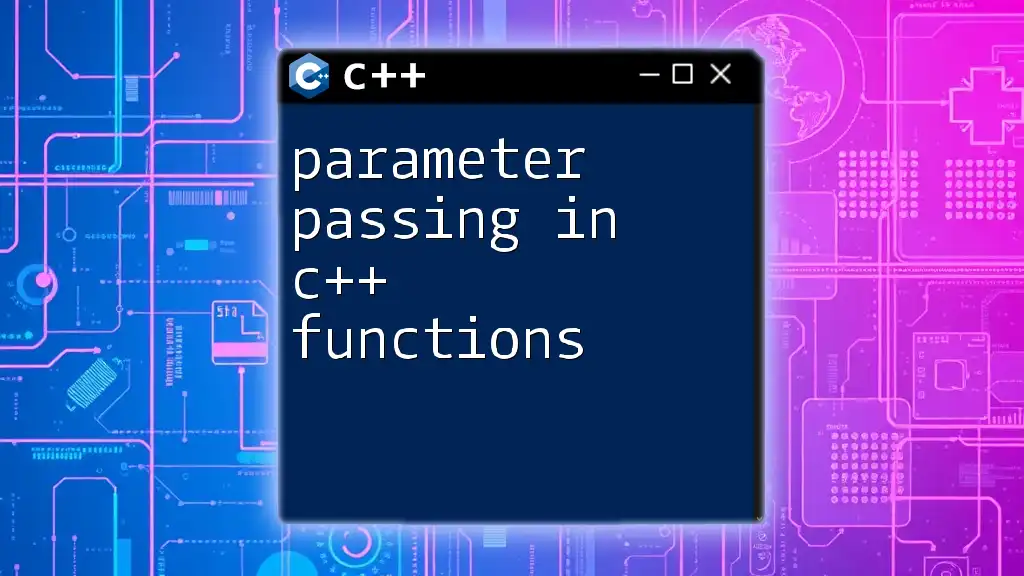
Practical Examples in C++
Using Pass by Value in Calculations
Here’s a practical example of using pass by value for a simple function that computes the sum of two numbers.
Example:
int add(int x, int y) {
return x + y;
}
int main() {
int a = 5, b = 10;
std::cout << "Sum: " << add(a, b) << std::endl; // Output: 15
return 0;
}
In this case, `a` and `b` are passed by value, and their sum does not change the originals.
Using Pass by Reference for Swapping Values
A common use case for passing by reference is swapping two values. This allows the function to swap the integers in place.
Example:
void swap(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 1, y = 2;
swap(x, y);
std::cout << "x: " << x << ", y: " << y << std::endl; // Output: x: 2, y: 1
return 0;
}
Using Pass by Pointer with Dynamic Memory
This example illustrates how to manipulate an array using pointers for dynamic memory allocation.
Example:
void updateArray(int *arr, int size) {
for (int i = 0; i < size; ++i) {
arr[i] += 5; // Add 5 to each element
}
}
int main() {
int size = 5;
int *arr = new int[size]{1, 2, 3, 4, 5};
updateArray(arr, size);
for (int i = 0; i < size; ++i) {
std::cout << arr[i] << " "; // Output: 6 7 8 9 10
}
delete[] arr; // Important to free memory
return 0;
}
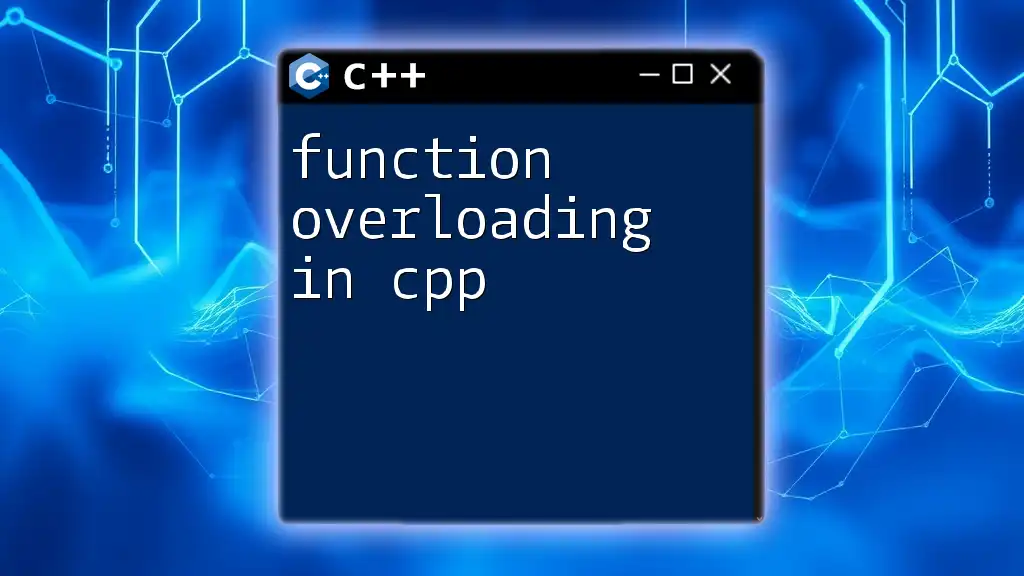
Advanced Concepts
Using const with Function Parameters
`const` can be employed to enhance safety and efficiency by preventing modifications to input parameters.
Example:
void display(const int x) {
std::cout << "Value: " << x << std::endl;
}
void increment(const int &x) {
// x++; // Error! Cannot modify a const reference
}
Here, using `const` signifies that the function promises not to modify the parameter, lending clarity and aiding in avoiding accidents.
Default Parameters
C++ allows function parameters to have default values, which can enhance the flexibility of function calls.
Example:
void display(int x, int y = 10) {
std::cout << "x: " << x << ", y: " << y << std::endl;
}
int main() {
display(5); // y takes the default value
display(5, 15); // Both values are provided
return 0;
}
Variadic Functions in C++
For functions that require a variable number of arguments, variadic functions are effective. This can be achieved using ellipsis (`...`) in parameter definitions.
Example:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
int main() {
printNumbers(3, 1, 2, 3); // Output: 1 2 3
return 0;
}
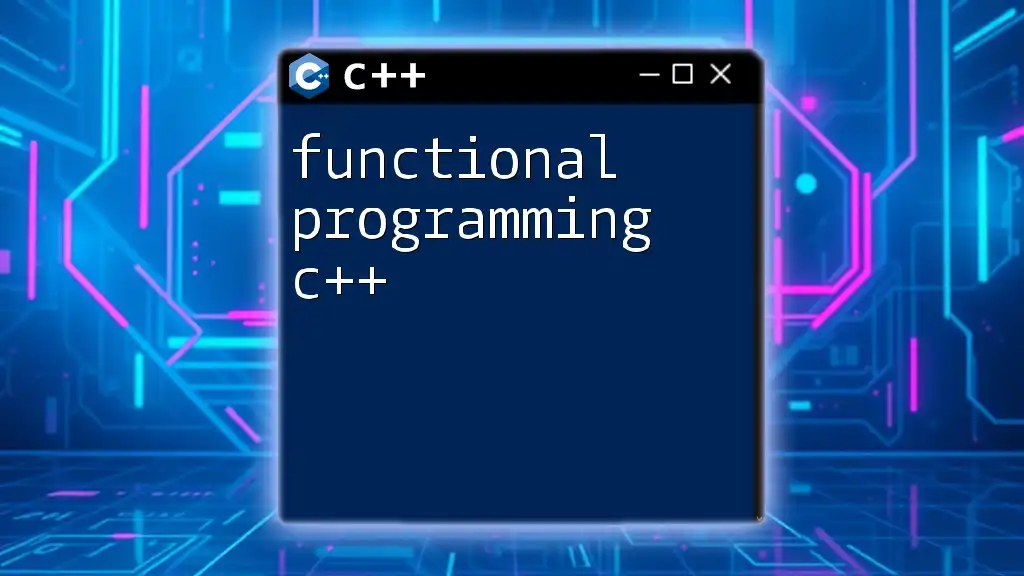
Conclusion
In understanding function parameter passing in C++, we see that the choice between pass by value, pass by reference, and pass by pointer significantly influences both performance and safety. It’s crucial to select the appropriate method based on the needs of the application, maintaining a balance between efficiency and code clarity.
Best Practices for Parameter Passing
- Use pass by value for simple types where immutability is paramount.
- Choose pass by reference when you need to modify an already existing variable or deal with large data types without overhead.
- Opt for pass by pointer when working with dynamic data structures.
Following these guidelines ensures that your code remains clean, efficient, and safe, making your functions both powerful and understandable.
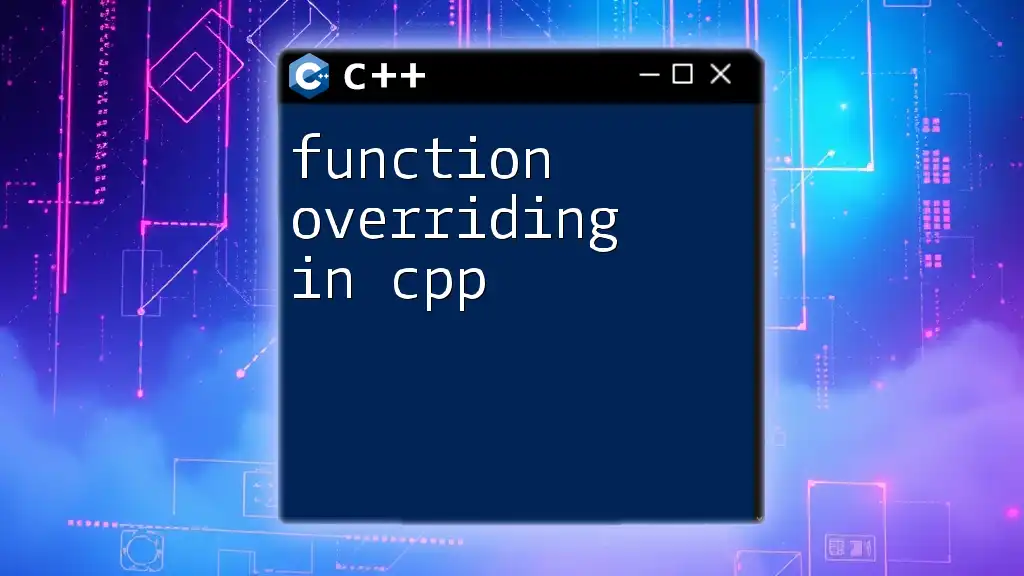
Additional Resources
While this guide offers a solid foundation, exploring books, websites, and community forums can greatly enhance your knowledge and troubleshooting skills in C++. Engage with peers and contribute to discussions to continue your learning journey in C++ and parameter passing methods.
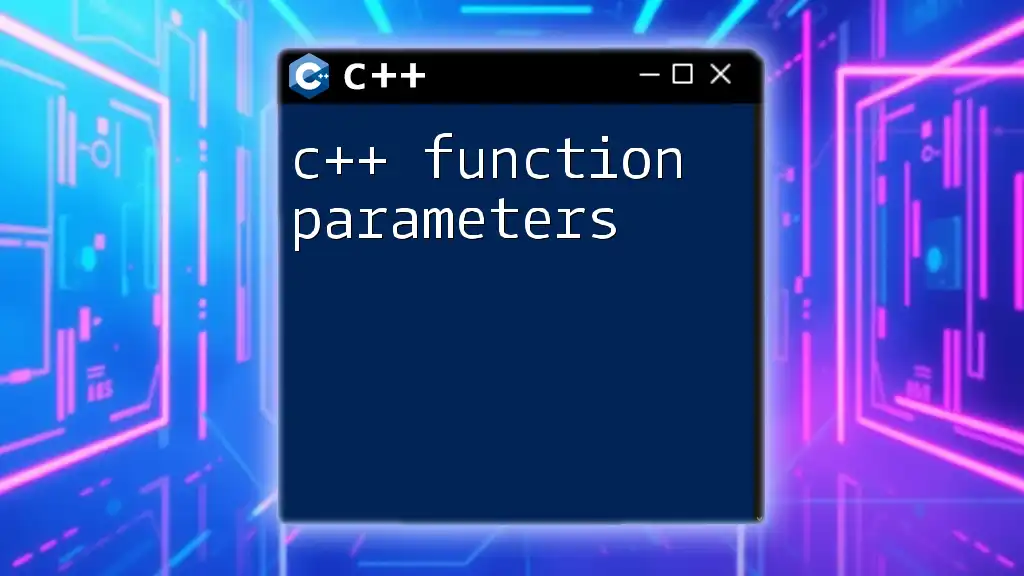
Frequently Asked Questions (FAQs)
What is the main difference between pass by reference and pass by pointer?
Pass by reference creates an alias for the variable, while pass by pointer works with the variable's memory address. References are generally safer and easier to use, eliminating the need for dereferencing.
When should I use pass by value instead of pass by reference?
Use pass by value when you need to ensure that the original data remains unchanged, especially for small, primitive types where performance overhead is negligible.
Are there performance implications of using pass by value for large objects?
Yes, for large objects, passing by value can cause significant overhead due to the copying process. In such cases, prefer pass by reference or pointer to enhance performance.