In C++, an array can be passed to a function for processing, allowing you to manipulate its elements within that function.
Here's a simple example demonstrating a function that calculates the sum of elements in an array:
#include <iostream>
using namespace std;
void sumArray(int arr[], int size) {
int sum = 0;
for (int i = 0; i < size; i++) {
sum += arr[i];
}
cout << "Sum: " << sum << endl;
}
int main() {
int myArray[] = {1, 2, 3, 4, 5};
int size = sizeof(myArray) / sizeof(myArray[0]);
sumArray(myArray, size);
return 0;
}
What is an Array?
An array is a collection of elements identified by index or key. In C++, an array is a data structure that allows you to store multiple items of the same data type in a single variable. This feature makes arrays particularly convenient for managing large amounts of data and simplifies code when working with lists of related values.
Arrays are essential in many programming scenarios where you need to perform operations on a collection of items. By utilizing arrays, you can access and modify the data efficiently using indices.
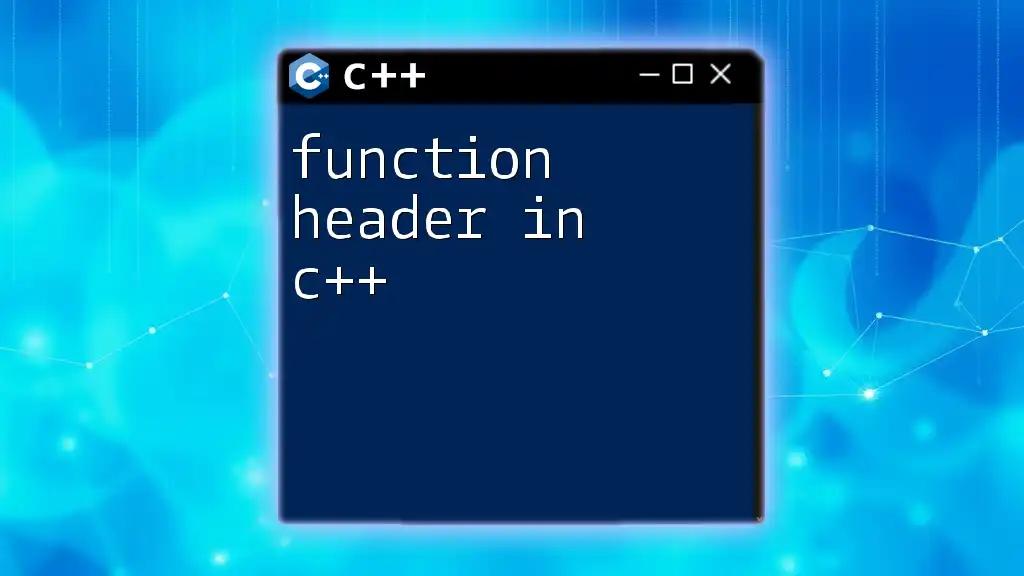
Types of Arrays in C++
One-dimensional arrays
One-dimensional arrays are the simplest form of arrays, where elements are stored in a single linear structure. For instance, you may store a list of integers like this:
int numbers[5] = {1, 2, 3, 4, 5};
In the above example, `numbers` is an array that can hold five integer values.
Two-dimensional arrays
Two-dimensional arrays can be visualized as a table or grid, where data is arranged in rows and columns. Here's an example:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
This `matrix` holds nine integers, arranged into three rows and three columns.
Multi-dimensional arrays
Multi-dimensional arrays extend higher dimensions like three-dimensional structures (cubes), which can be complex to manage. For example:
int cube[2][2][2] = {{{1, 2}, {3, 4}}, {{5, 6}, {7, 8}}};
Given the complexity of multi-dimensional arrays, they are less commonly used than one-dimensional or two-dimensional arrays.
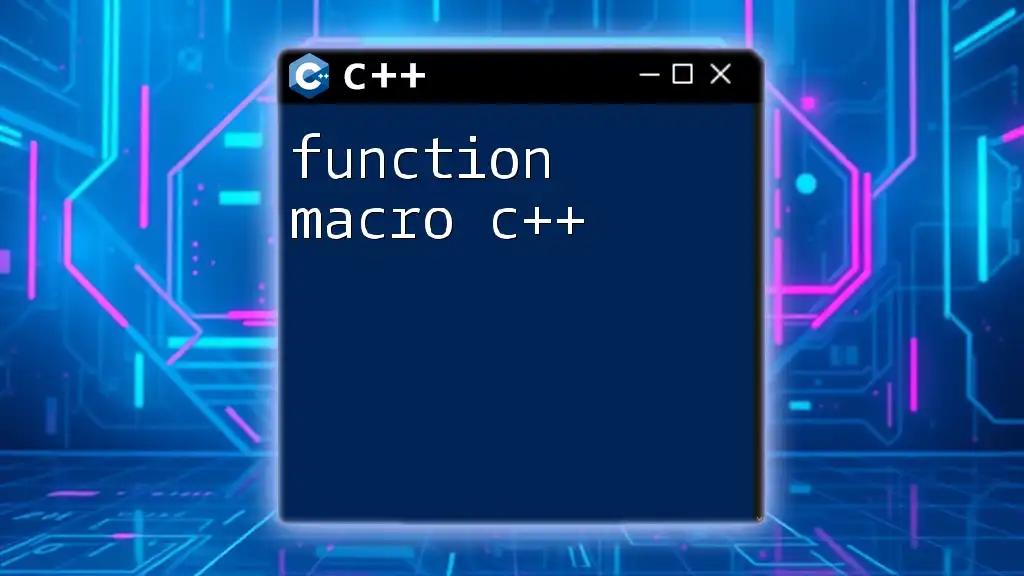
Basics of Array Functions in C++
Understanding Array Functions
Array functions in C++ are used to perform operations on arrays. Their primary purpose is to abstract operations, making code cleaner and more efficient. By utilizing functions, you allow for reuse, which ultimately reduces the chance of errors in your code.
Syntax for Function Declaration
When declaring a function that works with arrays, it's important to specify the argument type and size. Here's a simple example:
void processArray(int arr[], int size);
In this declaration, `arr[]` represents an array of integers, and `size` indicates the number of elements in the array. This syntax is fundamental in creating functions that manipulate arrays.
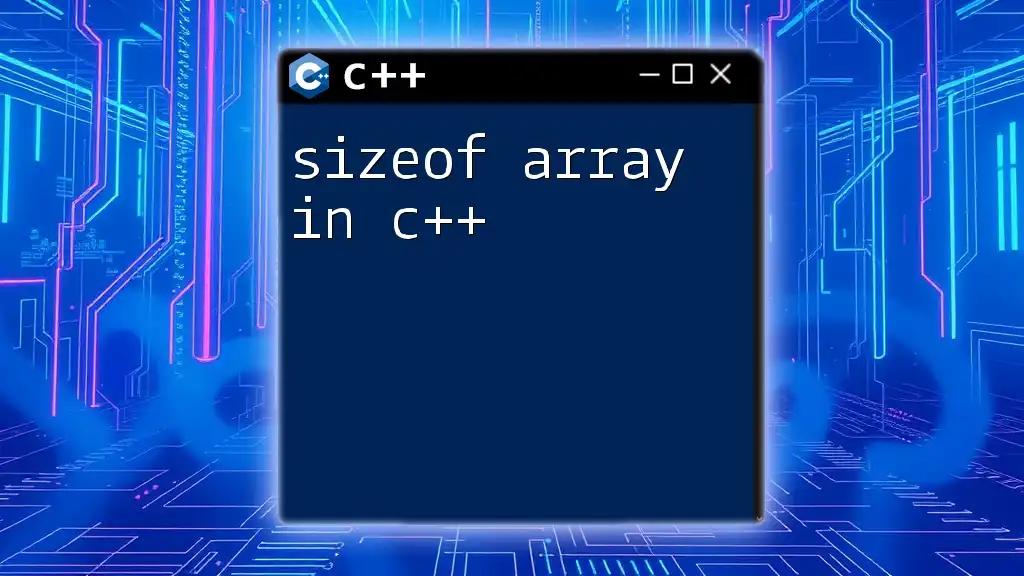
Creating Functions that Handle Arrays
Passing Arrays to Functions
Arrays in C++ are passed to functions by reference, meaning that no new copy of the array is created. This feature helps maintain memory efficiency. Here's an example that demonstrates passing an array to a function:
#include <iostream>
void displayArray(int arr[], int size) {
for (int i = 0; i < size; i++)
std::cout << arr[i] << " ";
}
In this example, the `displayArray` function prints each element of the array. Notice that we do not pass the size of the array as part of the array itself; instead, we supply its size as a separate argument. This is essential for controlling loops and preventing out-of-bounds errors.
Returning Arrays from Functions
Returning arrays from functions can be challenging because you cannot return an array directly. The common approach is to return a pointer to dynamically allocated memory. Here's an example:
int* createArray(int size) {
int* arr = new int[size];
return arr;
}
In this example, `createArray` dynamically allocates an array of integers in memory. Remember: when using `new`, you must manage memory allocation and deallocation. Failure to delete the array later on can lead to memory leaks.
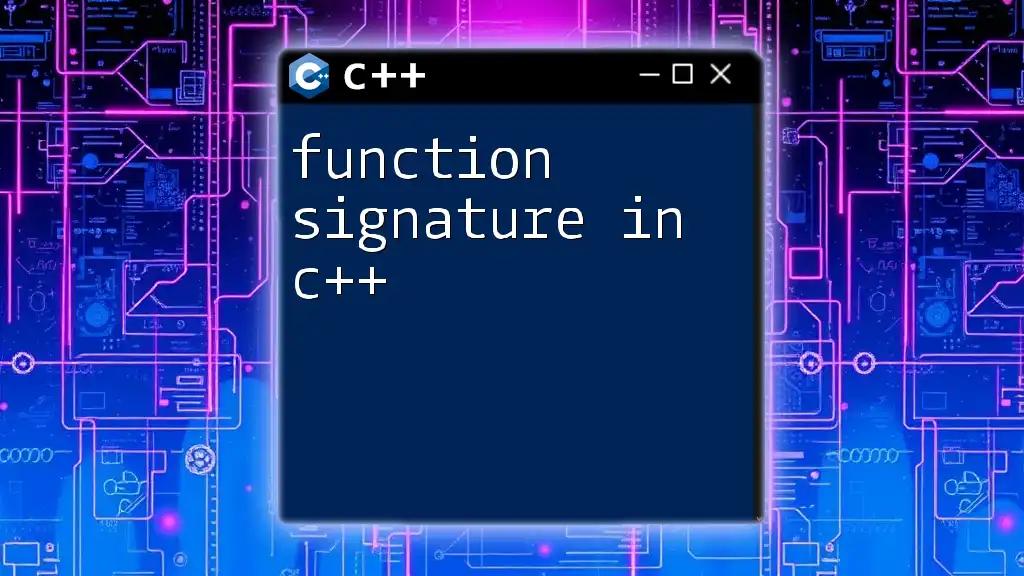
Common Array Functions in C++
Built-in Functions for Arrays
C++ offers several built-in functions for array manipulation. Using functions from libraries like `<algorithm>` and `<numeric>` helps simplify tasks, such as sorting or summing array values. For instance, the `std::sort` function allows you to sort arrays effortlessly.
Example: Sorting an Array
Sorting is a fundamental operation. Here's an example of a simple bubble sort function:
void bubbleSort(int arr[], int size) {
for (int i = 0; i < size - 1; i++)
for (int j = 0; j < size - i - 1; j++)
if (arr[j] > arr[j + 1])
std::swap(arr[j], arr[j + 1]);
}
This implementation of bubble sort iterates over the array, comparing adjacent elements and swapping them accordingly to sort the array in ascending order.

Best Practices for Using Functions with Arrays
Memory Management in Array Functions
Effective memory management is crucial in C++. Whenever you allocate memory using `new`, it is your responsibility to free that memory using `delete[]`. This practice prevents memory leaks, which can degrade performance over time. Here's how to properly deallocate an array:
delete[] arr; // Deallocating dynamically allocated array
Error Handling
Accessing an array out of bounds is a common error that can cause undefined behavior. To avoid such issues, it's wise to implement error checking:
if (index < 0 || index >= size) {
std::cerr << "Index out of bounds!";
}
This code snippet checks if the given index is within the permissible range before attempting to access the array.
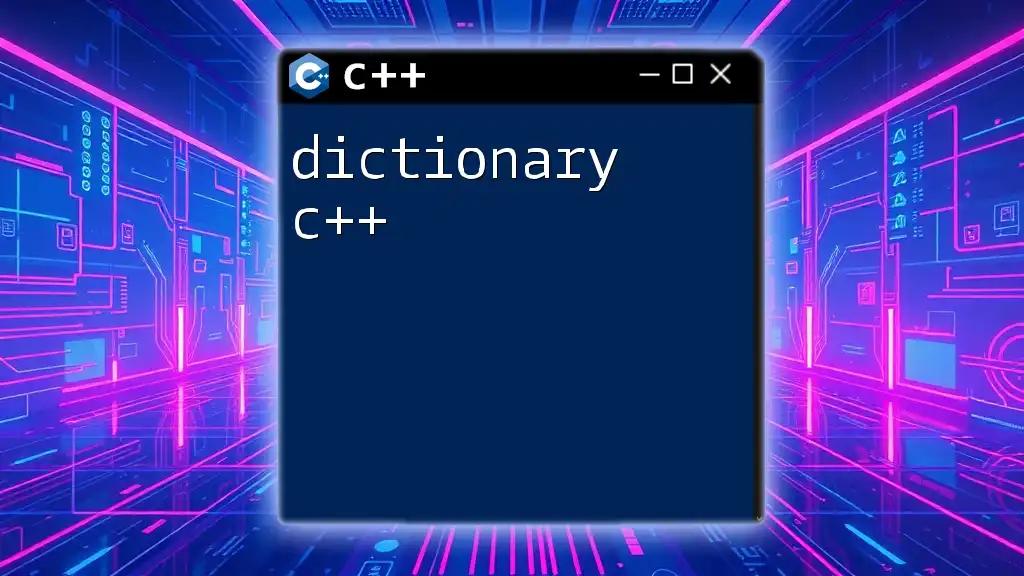
Conclusion
In summary, the function of array in C++ extends far beyond storing values; it involves creating efficient and reusable code through functions. Understanding the different types of arrays and how to manipulate them with functions is essential for any C++ programmer.
Moreover, practicing memory management and error handling will elevate your coding skills. Advanced topics like templates can further enhance your understanding of functions in managing arrays, paving the way for you to tackle more sophisticated data structures in the future.

Additional Resources
For further learning, consider exploring online tutorials, documentation, or coding platforms where you can practice your skills with array functions in C++. The depth of array manipulation potential offers great rewards to those willing to master it.