To delete a dynamically allocated array in C++, you should use the `delete[]` operator followed by the array variable name. Here's a code snippet demonstrating how to do it:
int* myArray = new int[10]; // dynamically allocate an array of 10 integers
// ... use the array ...
delete[] myArray; // delete the allocated array to free up memory
Understanding Arrays in C++
Definition and Purpose of Arrays
In C++, an array is a collection of variables of the same type that are accessed using a single variable name. Arrays provide a way to store multiple items of the same type together, making data management efficient. For example, if you want to store the scores of students, using an array allows you to group all the scores under a single variable.
Understanding arrays is crucial because they form the backbone of data management in many applications, particularly when dealing with data of fixed size.
Types of Arrays in C++
Arrays in C++ can broadly be categorized into two types:
-
Static Arrays: These arrays have a fixed size that is determined at compile time. They are allocated in the stack memory. For instance:
int scores[5]; // static array of size 5
-
Dynamic Arrays: These arrays are created at runtime using pointers and can change in size during program execution. This typically involves using the `new` keyword for allocation and requires explicit deletion to manage memory effectively. Here's how you might create a dynamic array:
int* scores = new int[5]; // dynamic array of size 5

Memory Management in C++
Importance of Memory Management
In C++, managing memory is a critical skill because the language does not provide automatic garbage collection. This puts the responsibility of allocating and deallocating memory on the programmer. If you create a dynamic array and fail to release its memory, you risk memory leaks, which can lead to reduced performance or program crashes.
How Memory Leaks Occur
Memory leaks occur when dynamically allocated memory is no longer accessible but has not been released. Over time, this can consume all available memory, leading to unpredictable behavior in your program.

Deleting Arrays in C++
The `delete` Operator
In C++, when you allocate memory dynamically, you also have the responsibility to free that memory once you're done using it. This is accomplished using the `delete` operator. For single variables, the syntax is simply `delete variablePointer;`. However, for dynamically allocated arrays, you must use the `delete[]` operator.
Syntax for Deleting an Array in C++
The correct syntax for deleting a dynamically allocated array is:
delete[] arrayPointer;
This notation tells the compiler to deallocate the entire block of memory that was allocated for that array. Understand the importance of this distinction; using just `delete arrayPointer;` can lead to undefined behavior, as it may not properly free the memory space.
Example of Deleting a Dynamic Array
Here’s how to correctly allocate and delete a dynamic array in C++:
int* myArray = new int[5]; // Dynamic allocation
for (int i = 0; i < 5; ++i) {
myArray[i] = i * 10; // Populate array
}
// Use the array...
delete[] myArray; // Correct way to delete the array
In this example, we allocate memory for an array of five integers, populate that array, and finally, we ensure to free the memory with `delete[]`. This is a crucial process in preventing memory leaks and ensuring efficient memory management.
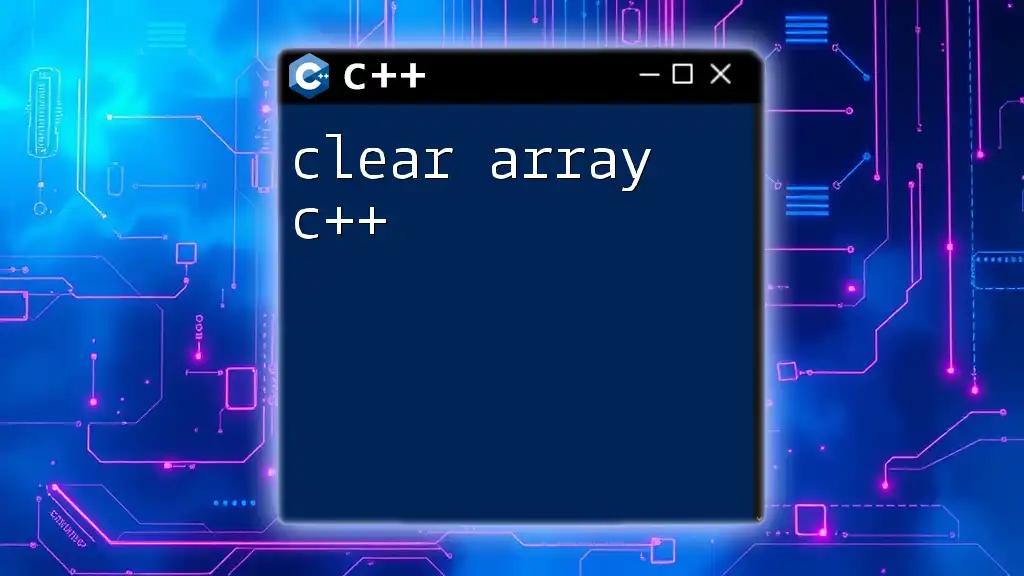
Common Mistakes When Deleting Arrays
Forgetting to Use `delete[]`
One of the most common pitfalls in C++ programming is forgetting to use `delete[]` when deleting a dynamic array. When you use just `delete`, it may not free the entire block of memory allocated for the array:
int* myArray = new int[5];
// ... populate and use myArray
delete myArray; // Incorrect: Leads to memory leak
By using `delete`, you are only telling the compiler to deallocate memory for the first element, which can lead to significant memory leaks.
Deleting an Array Multiple Times
Another danger is trying to delete the same array multiple times. This action results in undefined behavior, which may cause your program to crash or behave unexpectedly:
int* myArray = new int[5];
delete[] myArray;
// At this point, myArray is no longer valid
delete[] myArray; // Incorrect: Double deletion leads to undefined behavior
To avoid this, set the pointer to `nullptr` after deletion:
delete[] myArray;
myArray = nullptr; // Now it's safe to delete again
Using `delete` on Static Arrays
Remember that static arrays are managed automatically by C++. Using `delete` on a static array will result in a compilation error or undefined behavior:
int myArray[5];
delete[] myArray; // Incorrect: Causes a compilation error
Static arrays should be allowed to go out of scope automatically, and there is no need to manually delete them.
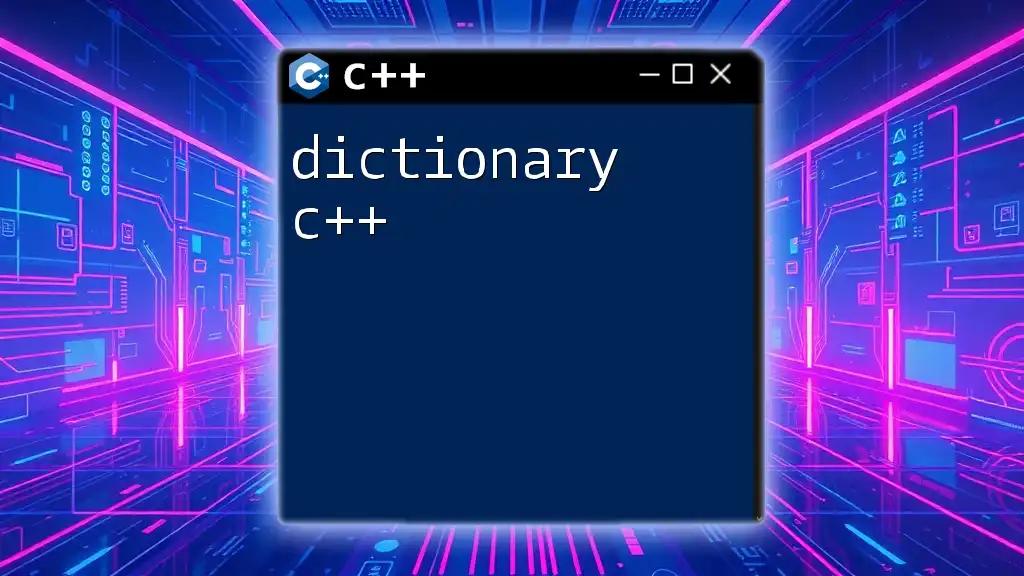
Best Practices for Deleting Arrays in C++
Smart Pointers and Their Advantages
To mitigate the risks related to manual memory management, consider using smart pointers. C++ has introduced smart pointers in the standard library which help automate the process of memory management.
- `std::unique_ptr`: This smart pointer retains sole ownership of an object through a pointer and automatically deletes the object when the unique pointer goes out of scope. Here’s how you can use it for arrays:
#include <memory>
std::unique_ptr<int[]> mySmartArray(new int[5]); // Automatically managed
By using smart pointers, you can help ensure that memory is freed when it's no longer needed, avoiding common pitfalls associated with manual memory management.
When to Use Dynamic Arrays
Dynamic arrays are suitable when you don’t know the exact size of data at compile time or when dealing with large amounts of data that exceed stack memory limitations. They offer flexibility in terms of resizing and improve the efficiency of memory usage for certain applications.
However, if the size is known and fixed, prefer using static arrays for their simplicity and automatic lifetime management.
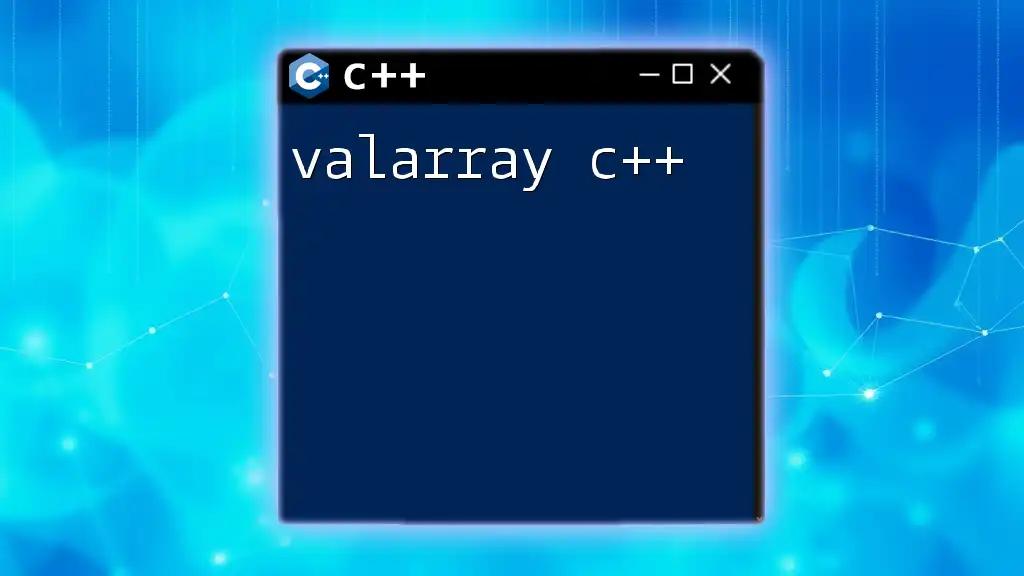
Conclusion
Properly managing dynamically allocated arrays is vital in C++ programming, particularly to avoid memory leaks and undefined behaviors. Understanding the differences between static and dynamic arrays, how to use the `delete[]` operator, and adhering to best practices such as using smart pointers can significantly improve your programming efforts.
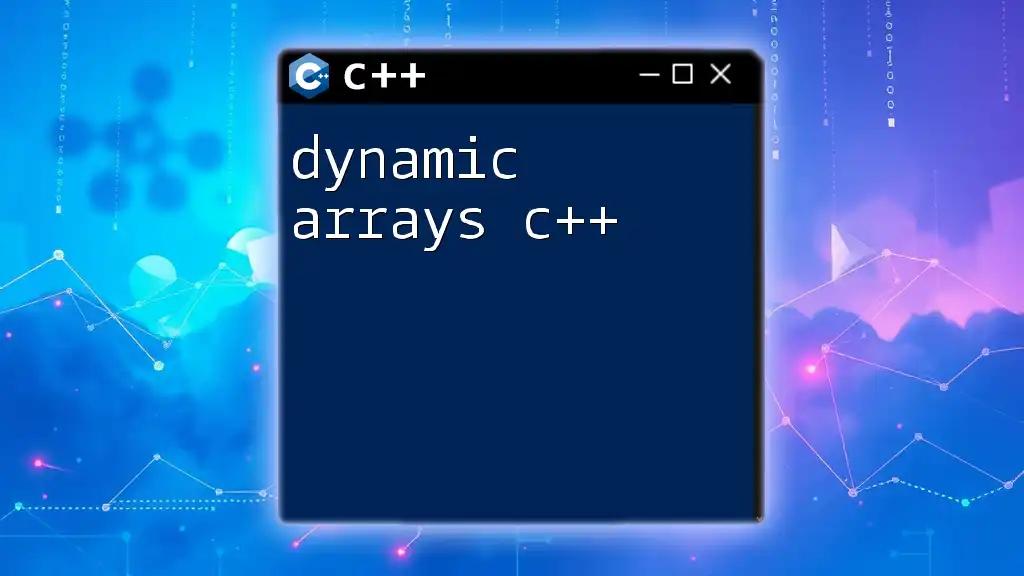
Call to Action
We encourage you to practice creating and deleting arrays in your own C++ projects. If you have any comments, questions, or experiences related to deleting arrays in C++, share them with us! Join our community for more tips and tutorials on mastering C++ programming.