A multidimensional array in C++ is an array that contains other arrays, allowing you to store data in a table format, such as rows and columns.
Here’s a simple example of a 2D array:
#include <iostream>
int main() {
int arr[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 4; ++j) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
What is a Multidimensional Array?
A multidimensional array in C++ is essentially an array of arrays. The most commonly used form is the 2D array, which can be visualized as a table with rows and columns. In general, the dimensionality of an array means how many indices you need to access an element within it.
Differences between One-Dimensional and Multidimensional Arrays
-
One-Dimensional Arrays: These can be thought of simply as a list of elements accessed with a single index. For example, `arr[0]` will give you the first element.
-
Multidimensional Arrays: In contrast, multidimensional arrays require multiple indices. For instance, in a 2D array `arr[i][j]`, you must specify both the row index `i` and the column index `j`. This allows for a structured way to manage and manipulate data that naturally fits into a tabular format.
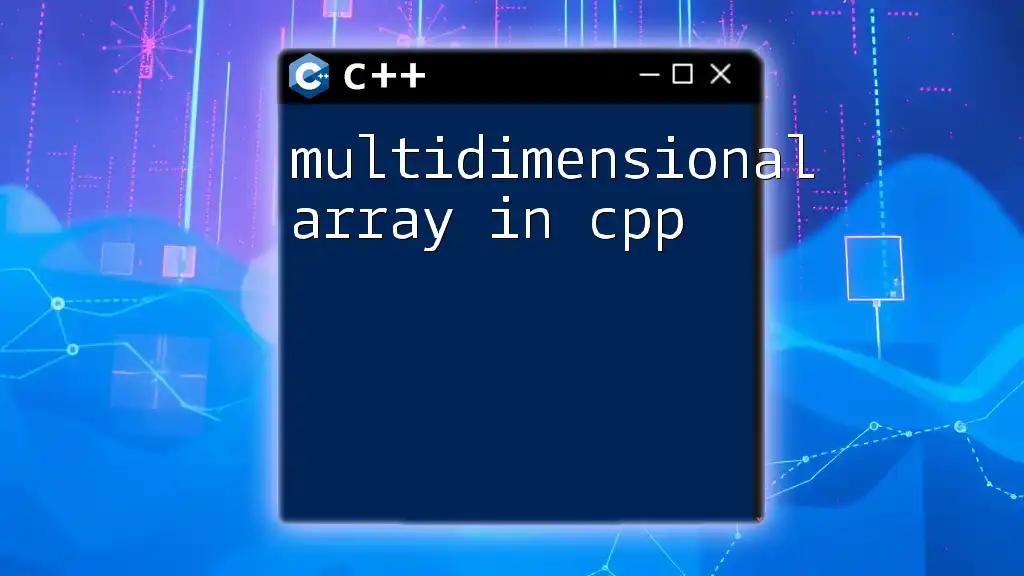
Importance of Multidimensional Arrays in Programming
Multidimensional arrays play a vital role in various programming contexts. They are commonly used for:
-
Data Representation: When modeling relationships between data points, such as in the case of matrices in linear algebra or grids in games.
-
Image Processing: Pixels in an image can be represented as a 2D array where each element corresponds to a pixel's color value.
-
Scientific Computing: In simulations and modeling, multidimensional arrays allow for the representation of complex datasets.
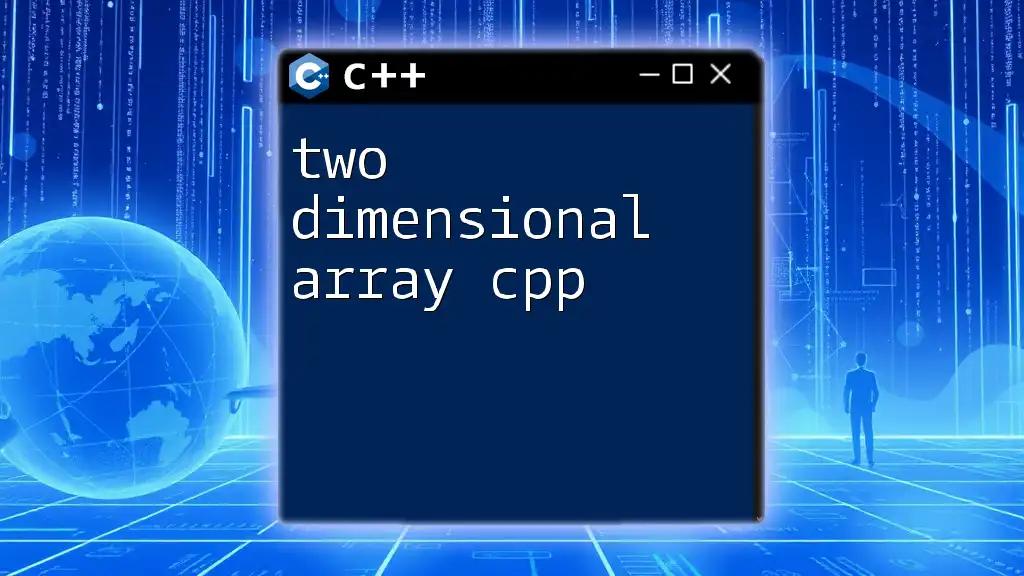
Basics of C++ Multidimensional Arrays
Declaring a Multidimensional Array in C++
Declaring a multidimensional array in C++ involves defining its size as per its dimensions. The syntax is straightforward:
int arr[3][4]; // Declares a 2D array with 3 rows and 4 columns
Each element can be accessed via two indices reflecting the row and column positions.
Initializing Multidimensional Arrays
Initialization can be performed in multiple ways:
-
Static Initialization: Here, you specify values at the time of declaration.
int arr[2][3] = {{1, 2, 3}, {4, 5, 6}}; // Initializes with values
-
Dynamic Initialization: This can happen at runtime, usually requiring loops to populate the array.
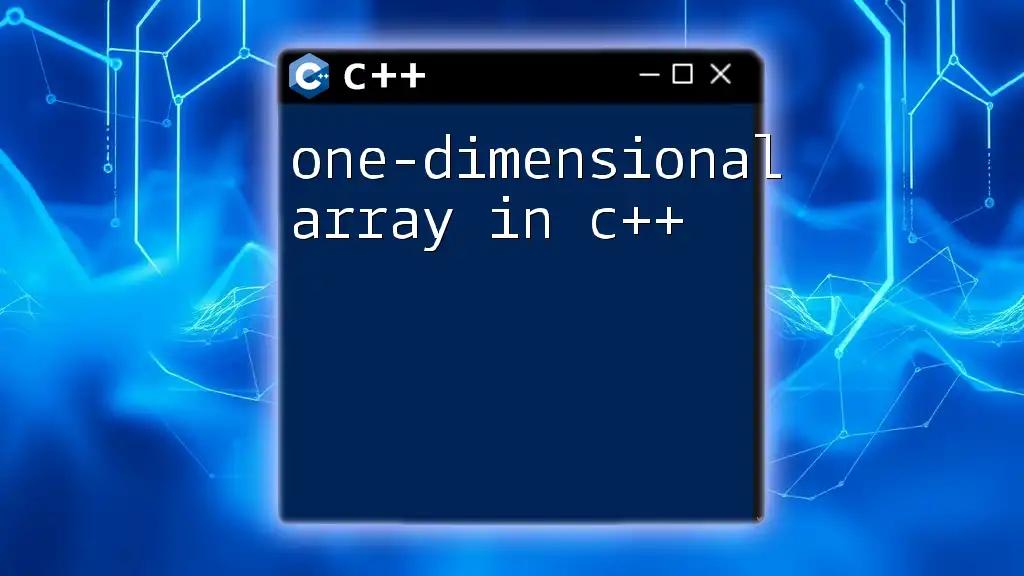
Accessing and Modifying Multidimensional Arrays
Accessing Elements in a C++ Multidimensional Array
Element access involves using both row and column indices. For example:
cout << arr[1][2]; // Outputs the third element of the second row
Important Note: Remember that array indexing in C++ starts from 0!
Modifying Elements in a Multidimensional Array
Just like accessing elements, modifying them is straightforward. You can change an array element with a simple assignment:
arr[0][1] = 10; // Changes the second element of the first row to 10
This simplicity allows for quick adjustments and updates to the data.

Common Operations on Multidimensional Arrays
Looping Through Multidimensional Arrays
To iterate through a multidimensional array effectively, you will need nested loops. For instance, using a 2D array:
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
This example prints all elements in a structured manner, showcasing how you can navigate through each dimension.
Passing Multidimensional Arrays to Functions
When it comes to function parameters, multidimensional arrays can be passed, though you need to specify dimensions correctly:
void printArray(int arr[][3], int rows) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < 3; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
}
In this case, the number of columns must be specified so the function knows how to navigate through the array.
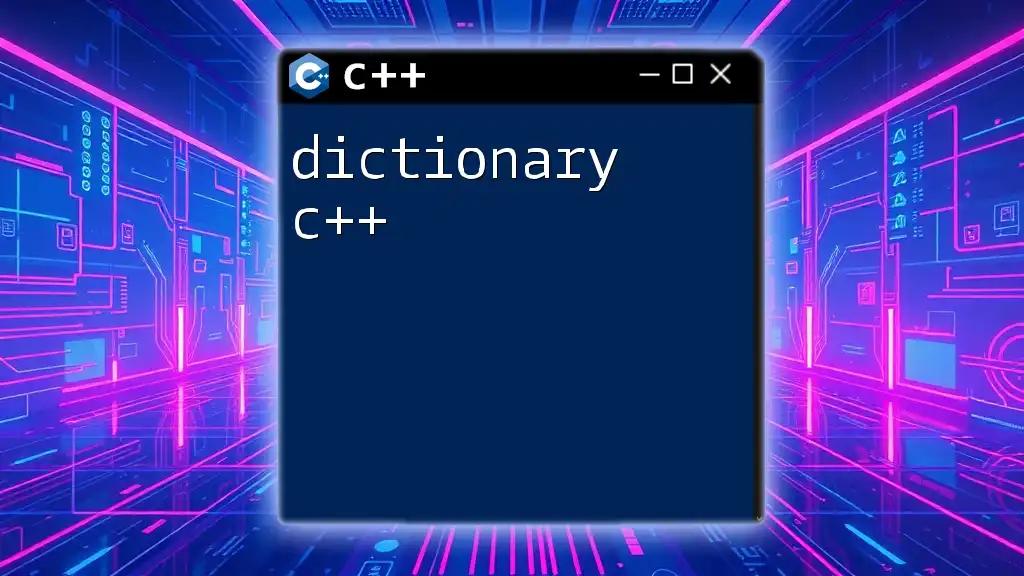
Advanced Topics in Multidimensional Arrays
Dynamic Multidimensional Arrays
If you require arrays of variable sizes, dynamic memory allocation comes into play. For instance, to create a dynamic multidimensional array:
int** arr;
arr = new int*[rows];
for (int i = 0; i < rows; i++)
arr[i] = new int[cols];
This creates an array where the number of rows and columns can be determined at runtime. Just remember to clean up the dynamically allocated memory with `delete`.
Multidimensional Arrays of Different Sizes
You can also create jagged arrays, where each row can have a different number of columns. This is done through a similar dynamic memory allocation technique:
int** jaggedArray = new int*[rows];
for (int i = 0; i < rows; i++) {
jaggedArray[i] = new int[cols[i]]; // cols[i] can vary for each row
}
This offers flexibility but requires careful management to avoid memory leaks.
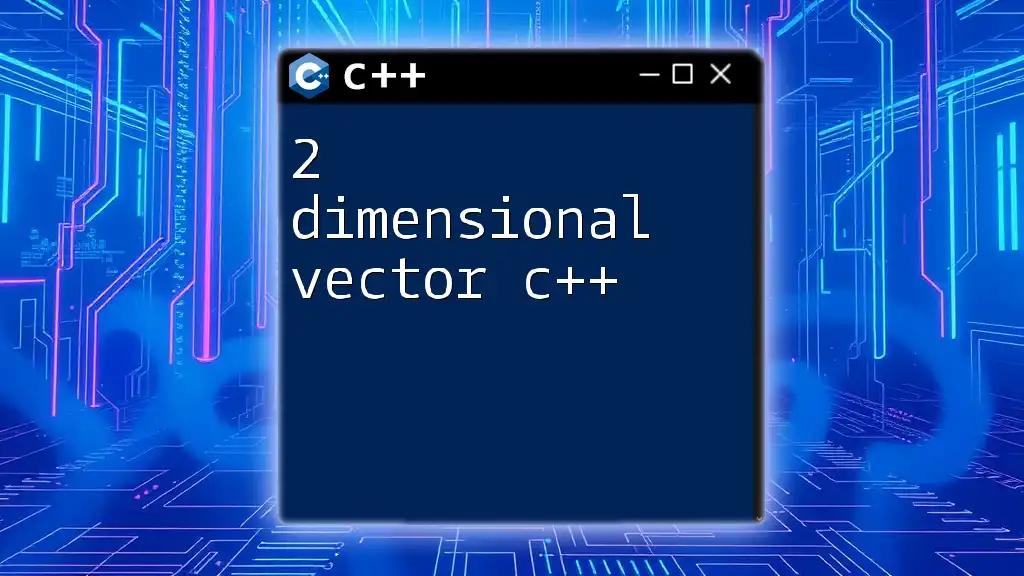
Practical Examples of Multidimensional Arrays in C++
Example 1: Matrix Addition
Matrix addition can be implemented simply with nested loops. Given two matrices of the same size, the result can be calculated as follows:
int matrixA[2][3] = {{1, 2, 3}, {4, 5, 6}};
int matrixB[2][3] = {{7, 8, 9}, {10, 11, 12}};
int result[2][3];
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
result[i][j] = matrixA[i][j] + matrixB[i][j];
}
}
This encapsulates the basic logic of addition — summing corresponding elements.
Example 2: Finding the Transpose of a Matrix
Finding the transpose involves swapping rows with columns. For a square matrix, it looks like this:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int transpose[3][3];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
transpose[j][i] = matrix[i][j];
}
}
This procedure effectively rearranges the matrix to a transposed state.
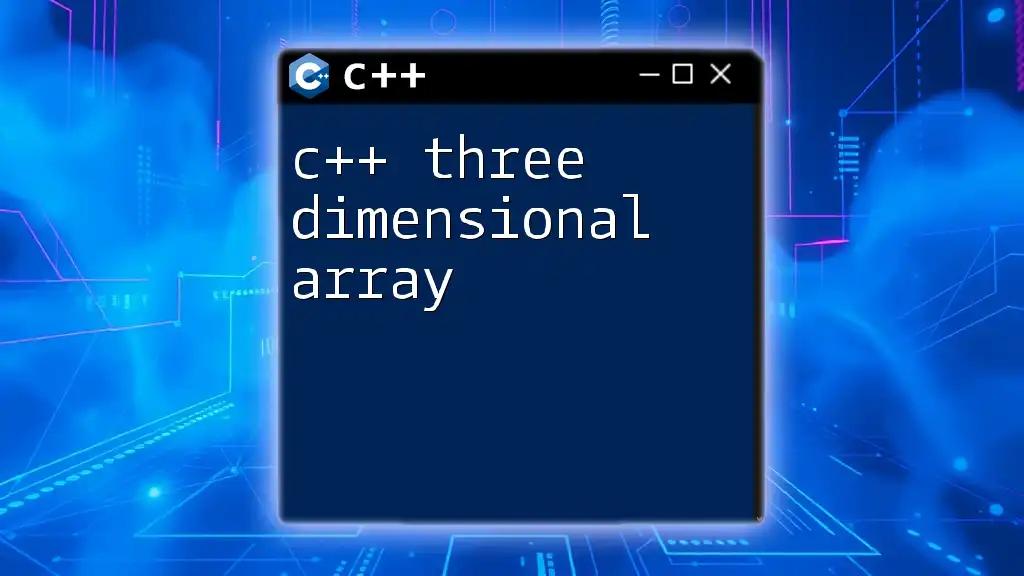
Conclusion
In summary, the multidimensional array in C++ serves as a powerful construct that enhances data representation and management in programming. From basic declaration and initialization to dynamic memory techniques and practical applications like matrix operations, multidimensional arrays prove indispensable in various scenarios. Explore them further and implement these concepts in your work for effective and logical data handling.

Additional Resources
- For deeper insights, consider referencing textbooks focused on C++ programming or checking online platforms like educational websites and documentation sites to bolster your understanding of multidimensional arrays and their applications in real-world scenarios.