In C++, you can pass a two-dimensional array to a function by specifying the array type along with the size of the second dimension in the function parameters. Here's a code snippet demonstrating this:
#include <iostream>
void printArray(int arr[][3], int rows) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < 3; ++j) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
}
int main() {
int myArray[2][3] = {{1, 2, 3}, {4, 5, 6}};
printArray(myArray, 2);
return 0;
}
Understanding 2D Arrays in C++
What is a 2D Array?
A 2D array is a data structure that allows the storage of data in a tabular format, which can be visualized as a matrix of rows and columns. Unlike a 1D array that holds a linear sequence of elements, a 2D array is ideal for scenarios requiring organization of data in two dimensions, such as a table or a grid.
For instance, a 2D array representing a classroom seating arrangement might look like this:
Col1 Col2 Col3
Row1 A B C
Row2 D E F
Row3 G H I
Declaring a 2D Array
To declare a 2D array in C++, you can specify the number of rows and columns like this:
int array[3][4]; // 3 rows, 4 columns
This declaration creates a 2D array that can hold a total of 12 integers.
Initializing a 2D Array
You can initialize a 2D array during declaration by providing the initial values enclosed in curly braces. Here's an example:
int arr[3][4] = { {1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12} };
This creates a 2D array with three rows and four columns, initialized with specified values.
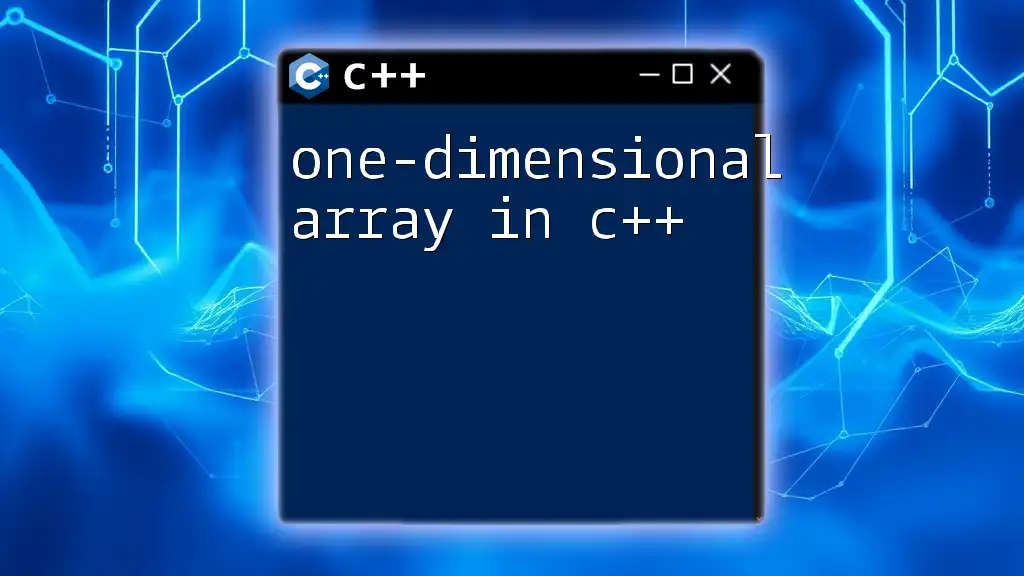
Passing a 2D Array to a Function
Why Pass 2D Arrays to Functions?
Passing two-dimensional arrays to functions is essential for enhancing code organization and memory efficiency. It allows you to isolate functionality (like processing or displaying the array) into different functions, thus making your code cleaner and reusable.
Syntax for Passing a 2D Array
The syntax for passing a 2D array to a function can be written as follows:
void myFunction(int arr[][4]); // Note: the number of columns must be specified
It’s critical to define the column size when declaring the function parameter. This information helps the compiler to understand the memory layout of the 2D array.
Complete Example of Passing 2D Arrays to Functions
Passing a 2D Array with Fixed Size
Here’s a code snippet that demonstrates how to pass a fixed-size 2D array to a function and print its elements:
#include <iostream>
void printArray(int arr[][4], int rows) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < 4; ++j) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
}
int main() {
int array[3][4] = { {1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12} };
printArray(array, 3);
return 0;
}
In this example, the `printArray` function takes a 2D array and its row count, iterating through to print each value.
Passing a 2D Array with Variable Columns
When working with variable-sized 2D arrays, you can define the function with a pointer to an array. This is particularly useful for dynamic arrays where column sizes are not known during compile time.
Here's how you can achieve this:
#include <iostream>
void printArray(int (*arr)[4], int rows) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < 4; ++j) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
}
The use of `int (*arr)[4]` indicates that `arr` is a pointer to an array containing four integers per row.
Using std::vector for Dynamic 2D Arrays
Introduction to std::vector
A more modern and flexible way to manage 2D arrays in C++ is to use the `std::vector`. Vectors can dynamically resize themselves and avoid manual memory management issues associated with raw arrays.
To create a 2D vector, you can do the following:
#include <vector>
std::vector<std::vector<int>> vec(3, std::vector<int>(4));
This creates a 2D vector initialized with three rows and four columns.
Function to Pass std::vector
Passing a vector of vectors to a function works similarly to arrays but with improved safety and flexibility:
#include <iostream>
#include <vector>
void printVectorArray(const std::vector<std::vector<int>>& vec) {
for (const auto& row : vec) {
for (int val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
}
int main() {
std::vector<std::vector<int>> vec = {{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}};
printVectorArray(vec);
return 0;
}
This method of passing 2D arrays using `std::vector` handles memory management automatically, making your code less prone to errors.
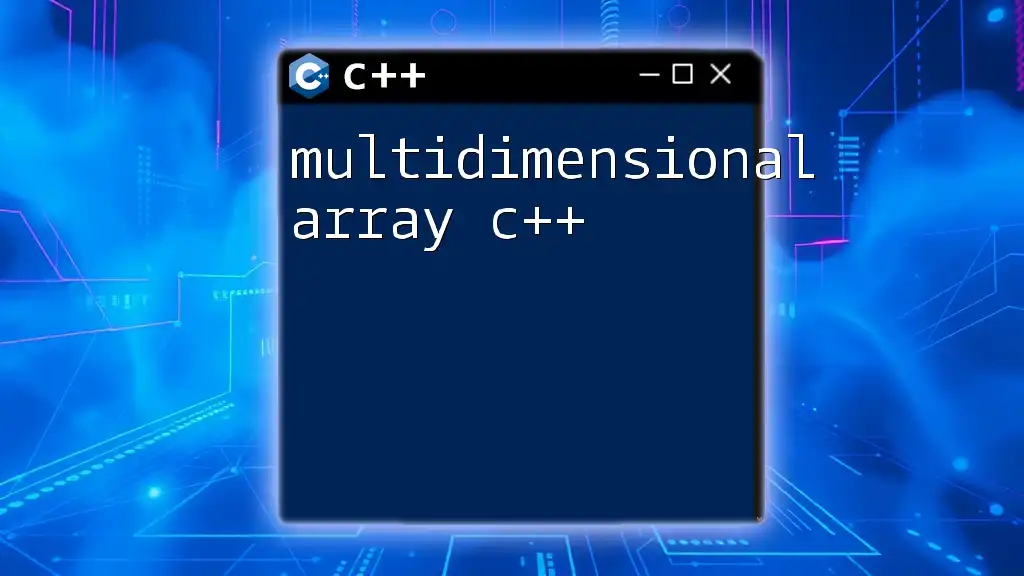
Common Issues and Solutions
Memory Management Considerations
When passing large 2D arrays, it’s important to be mindful of memory management. Passing large arrays can lead to stack overflow errors if the arrays are too large. If the array size is determined at runtime, consider using dynamic memory allocation or std::vector to manage the memory efficiently.
Compilation Errors and Debugging Tips
Common errors when passing 2D arrays can include:
- Mismatched dimensions between the function declaration and the argument passed.
- Incorrectly defined array sizes in the header.
Debugging these issues can be simplified by:
- Checking the size and type of arrays at compile time.
- Using a debugging tool to step through your code line by line.
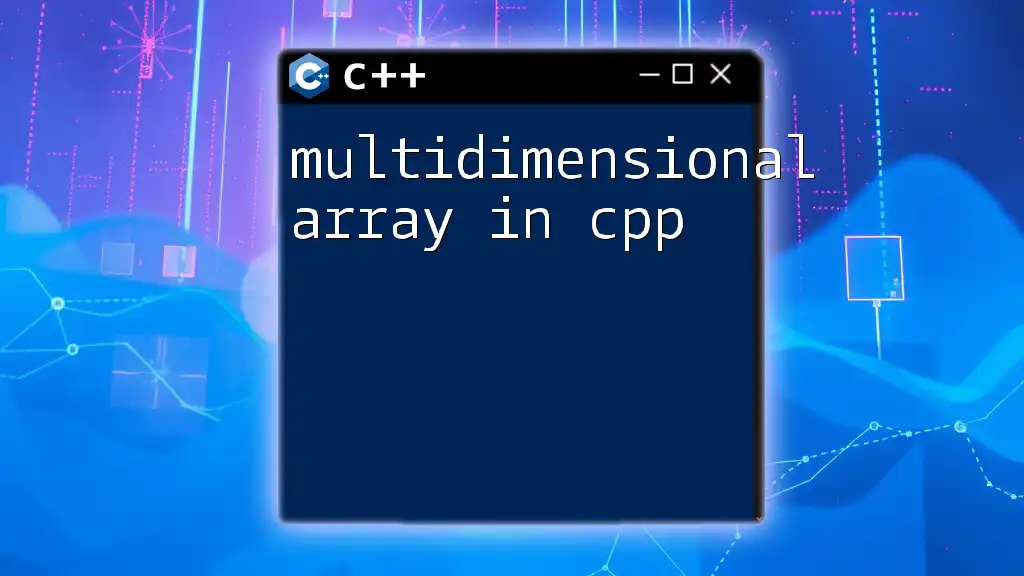
Best Practices
When to Use 2D Arrays
2D arrays are valuable when you're dealing with structured data that mimics a table or grid, such as matrices in mathematical computations, pixel data in images, or seating charts. If you need variable dimensions, consider using `std::vector` instead for its flexibility.
Coding Style and Readability
Maintaining a clear coding style is paramount. Use meaningful names for your arrays and functions, comment your code liberally to explain complex logic, and ensure your code is well-structured. Readable code is easier to debug and maintain over time.
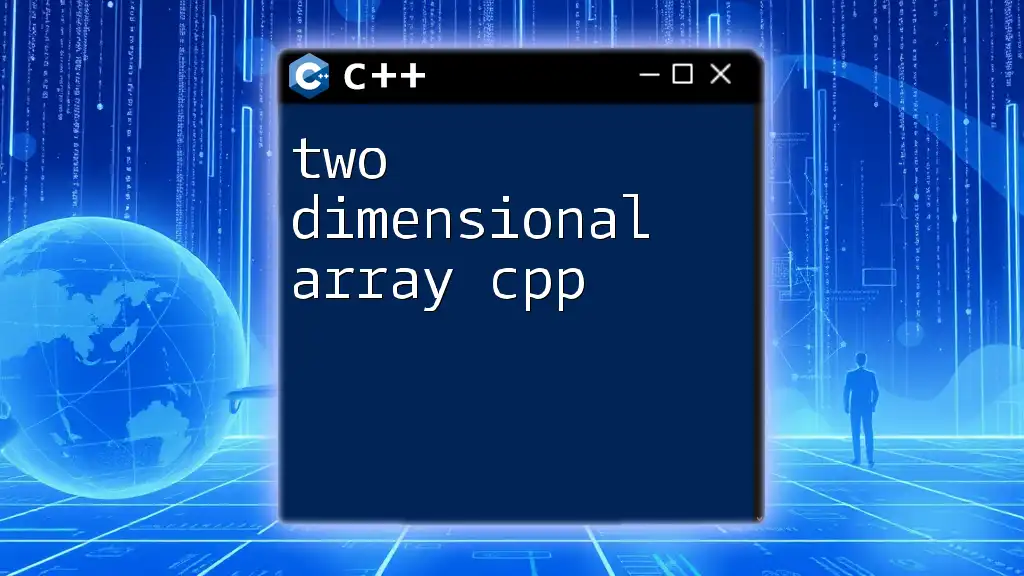
Conclusion
This article has provided a comprehensive guide on how to pass 2 dimensional arrays to functions in C++—from understanding their structure to practical implementations in code. Mastery of this topic is crucial for effective coding in C++, especially when dealing with complex data structures. Practice by writing functions that manipulate 2D arrays to solidify your understanding.
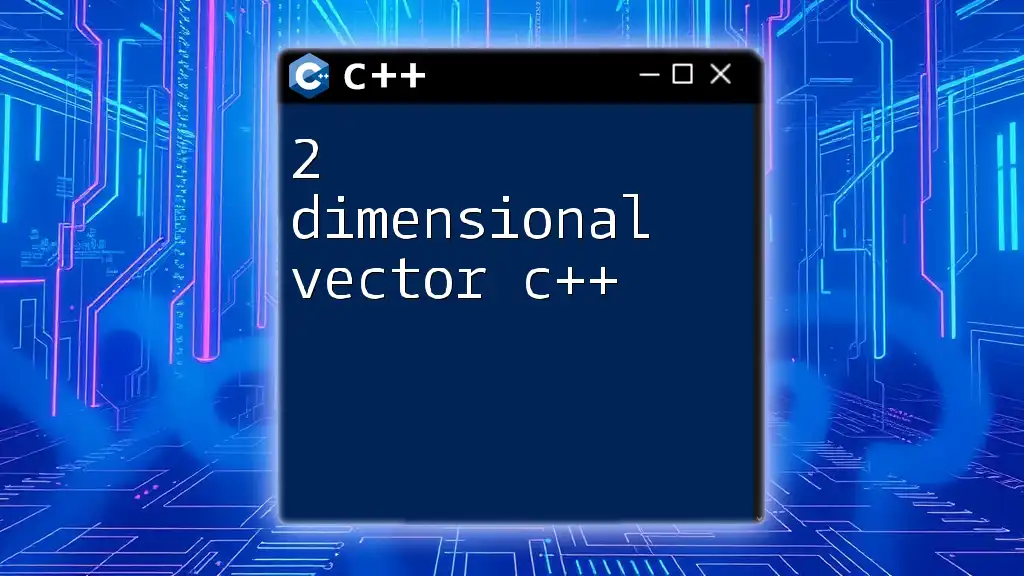
Additional Resources
For further learning, you may explore the official C++ documentation, and seek out online tutorials and textbooks that delve into the intricacies of arrays and data structures in C++.