A bidirectional iterator in C++ allows traversal of a container in both forward and backward directions, making it useful for various data structures such as lists and deques.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
auto it = myList.begin();
// Forward iteration
for (; it != myList.end(); ++it) {
std::cout << *it << " "; // Output: 1 2 3 4 5
}
std::cout << std::endl;
// Backward iteration
--it; // Move to last element
for (; it != myList.begin(); --it) {
std::cout << *it << " "; // Output: 5 4 3 2 1
}
std::cout << *it; // Output: 1
return 0;
}
What are Iterators?
In C++, iterators serve as a powerful abstraction layer for traversing elements in a collection like arrays or other containers. Think of iterators as generalized pointers that provide a way to navigate through the elements without exposing the underlying data structure.
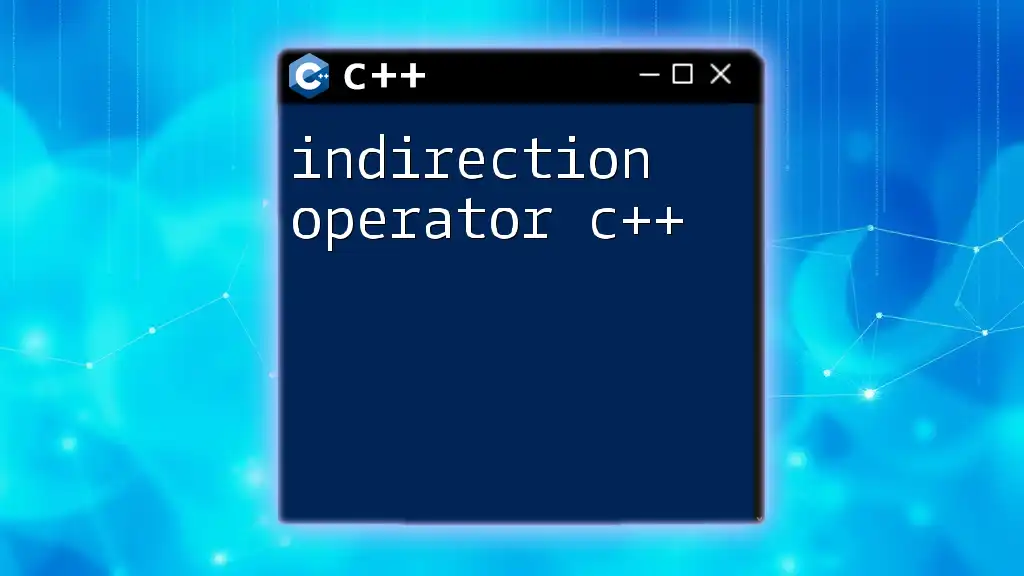
Types of Iterators
Understanding the various types of iterators is crucial as they cater to different use cases. Here are key types to consider:
- Input Iterators: Allow reading elements once and only in a forward direction.
- Output Iterators: Enable writing elements once and only in a forward direction.
- Forward Iterators: Allow reading and writing elements in a forward direction and can traverse multiple times.
- Bidirectional Iterators: Can move both forward and backward, making them versatile for traversing collections.
- Random-Access Iterators: Provide constant-time access to any element, akin to array indexing.
Choosing the right iterator type influences the efficiency and clarity of your code.
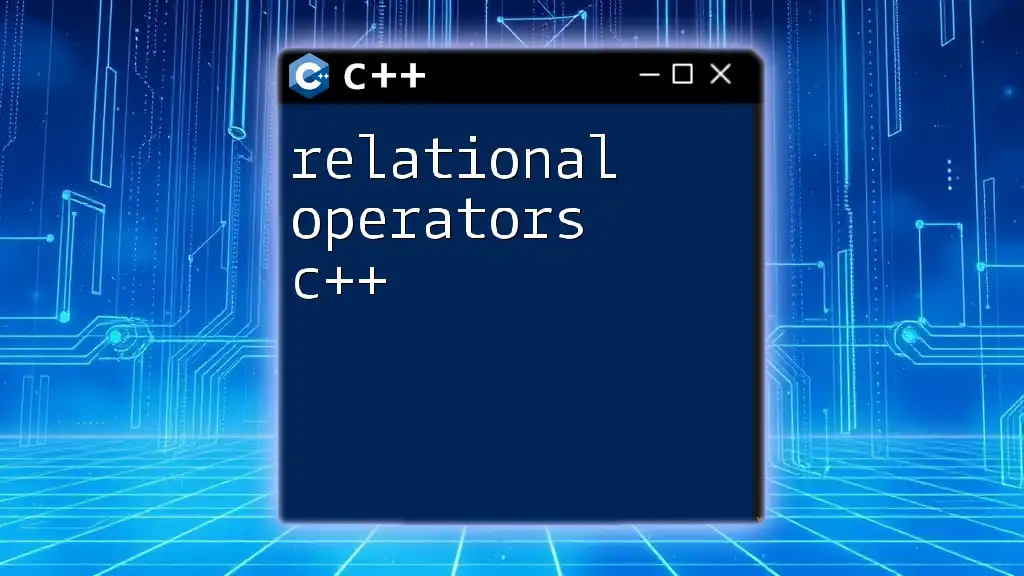
Understanding Bidirectional Iterators
Definition of Bidirectional Iterator
A bidirectional iterator extends the capabilities of forward iterators by allowing traversal in both forward and backward directions. This flexibility is particularly useful when working with data structures where you might need to backtrack.
Containers in the C++ Standard Library that support bidirectional iterators include:
- `std::list`
- `std::set`
- `std::map`
Characteristics of Bidirectional Iterators
Unlike input or output iterators, bidirectional iterators enable you to:
- Traverse elements both in increasing and decreasing order.
- Utilize mixed operations (e.g., increment `++it` or decrement `--it`).
Comparative Advantage: When working with a bidirectional iterator, you do not need to create a new iterator for backward traversal as seen in forward iterators. This feature provides a seamless coding experience when working with certain algorithms.
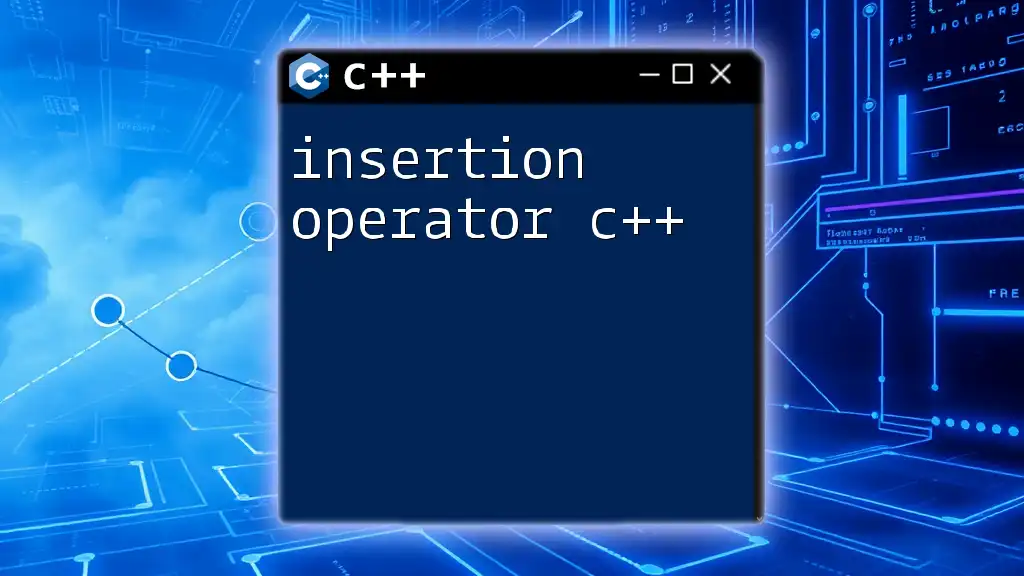
How to Use Bidirectional Iterators
Introducing the Bidirectional Iterator Syntax
The syntax for using bidirectional iterators is straightforward. You instantiate an iterator, typically associated with a container, and move through the elements as needed. This approach benefits from safety and increased readability.
Example with the Standard Library
Here's a practical example demonstrating how to leverage bidirectional iterators using the `std::list` container:
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
// Creating bidirectional iterators
std::list<int>::iterator it = myList.begin();
// Traversing forward
for (; it != myList.end(); ++it) {
std::cout << *it << " ";
}
std::cout << std::endl;
// Traversing backward
for (it = myList.end(); it != myList.begin();) {
--it; // Move backwards through the list
std::cout << *it << " ";
}
return 0;
}
In this example, you create a list of integers, traverse it forward, and then backtrack through the elements using the bidirectional iterator `it`. This bidirectional capability allows you to explore the data structure efficiently.
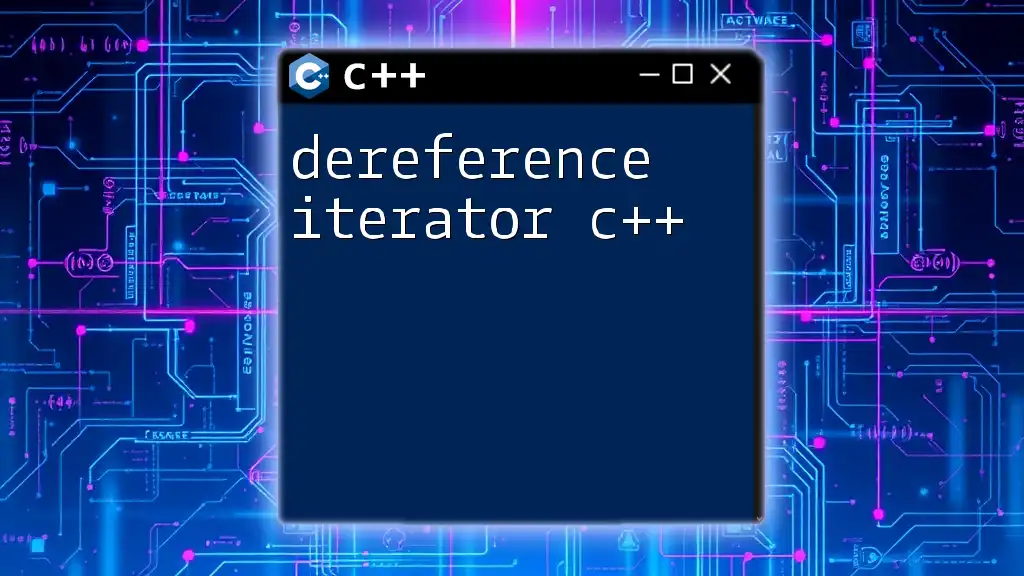
Underlying Mechanism of Bidirectional Iterators
How Bidirectional Iterators Work
Bidirectional iterators utilize pointer-like mechanics to access elements. Each iterator includes methods such as `++it` for moving to the next element and `--it` for returning to the previous element. This functionality is crucial for enabling full traversal of containers.
The Importance of Iteration Control
Controlling the iteration is key to working with collections. The increment (`++`) and decrement (`--`) operations enable developers to navigate through an entire sequence with precise control:
- Stability: The performance of bidirectional iterators typically remains stable across operations, much better than multiple individual iterations.
- Performance: Utilizing bidirectional iterators can yield performance improvements, especially when integrated with STL algorithms.
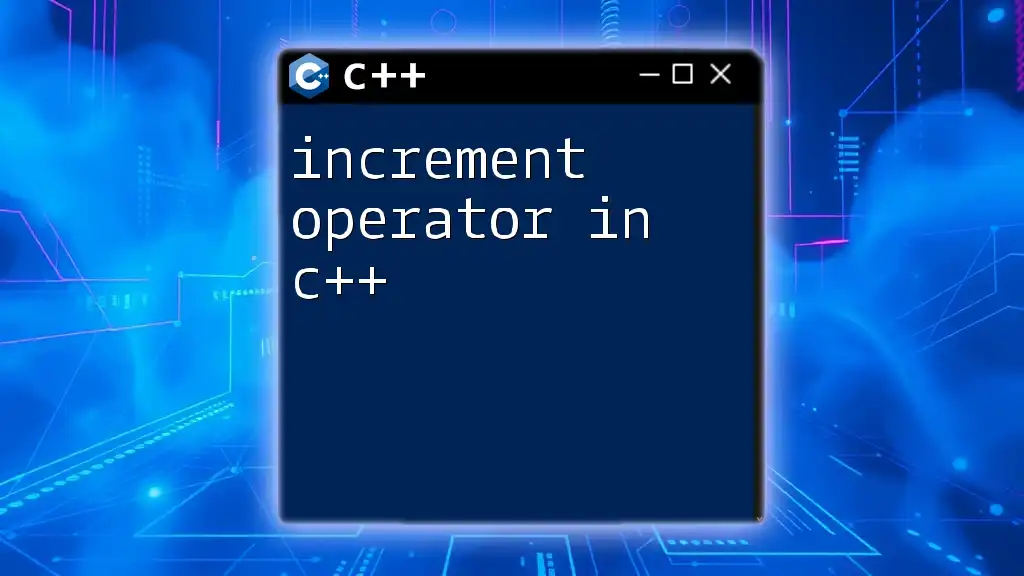
Real-world Applications of Bidirectional Iterators
Use in Standard Template Library (STL) Containers
Bidirectional iterators are commonly employed in STL containers like `std::list`, `std::set`, and `std::map`. They are particularly handy when you need to maintain the order of the elements while allowing for reverse access.
Here's a brief showcasing usage in `std::set`:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
// Bidirectional iterator for std::set
std::set<int>::iterator it = mySet.begin();
// Forward traversal
for (; it != mySet.end(); ++it) {
std::cout << *it << " ";
}
std::cout << std::endl;
// Backward traversal
for (it = mySet.end(); it != mySet.begin();) {
--it; // Move backward through the set
std::cout << *it << " ";
}
return 0;
}
When to Use Bidirectional Iterators
Bidirectional iterators are advantageous when:
- You need to traverse elements in both directions without generating additional iterators.
- You're interacting with data structures like lists or sets where order matters, and you need to access predecessors.
Leveraging bidirectional iterators can enhance the clarity and efficiency of your code significantly.
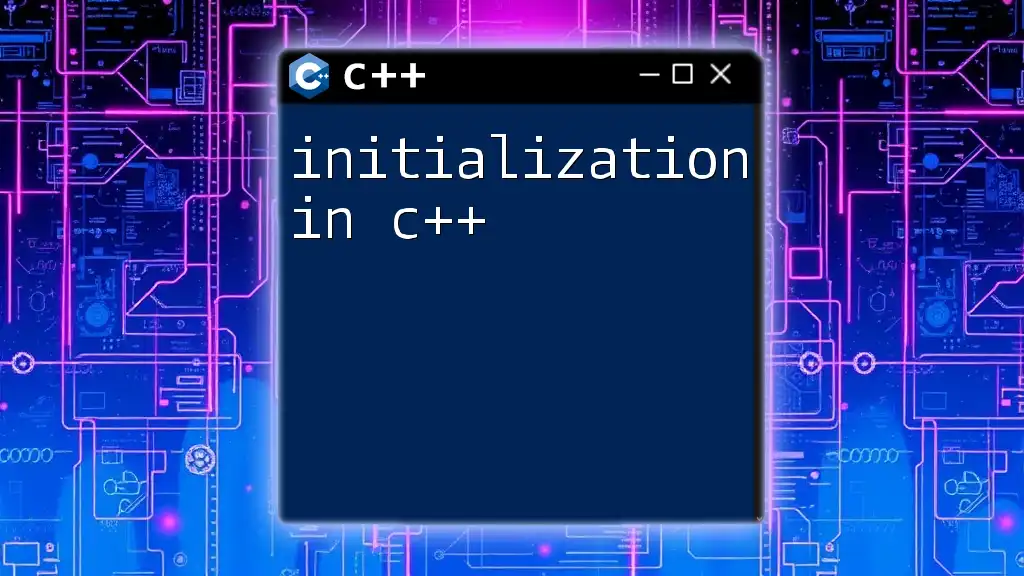
Common Mistakes and Best Practices
Common Errors with Bidirectional Iterators
When utilizing bidirectional iterators, a few common pitfalls to be cautious of include:
- Iterator Validity: Always check whether your iterator is valid before dereferencing it to avoid runtime errors.
- Boundary Cases: Misunderstanding the iterator limits can cause infinite loops or segmentation faults.
Best Practices in Using Bidirectional Iterators
To maximize the effectiveness of bidirectional iterators:
- Initialization: Always initialize your iterators correctly before use.
- End Checks: Rigorously check whether your iterator has reached the end to maintain stability in your loops.
- Use STL Algorithms: Prefer using STL algorithms designed for iterators to improve readability and performance.
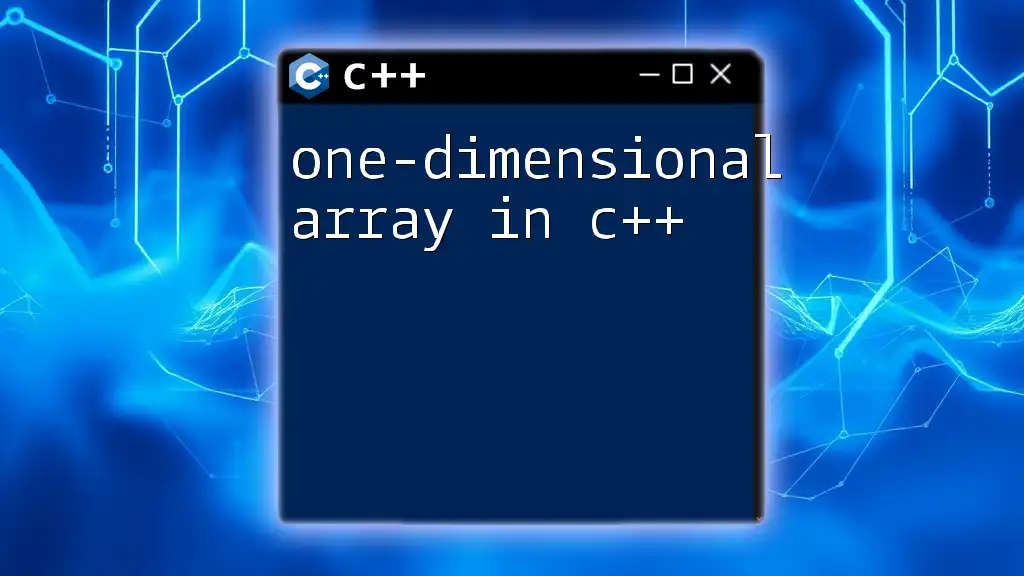
Conclusion
Bidirectional iterators are vital components of C++ programming, particularly in terms of data traversal and manipulation. They offer flexibility and efficiency, making them indispensable when manipulating collections where bidirectional access is beneficial.
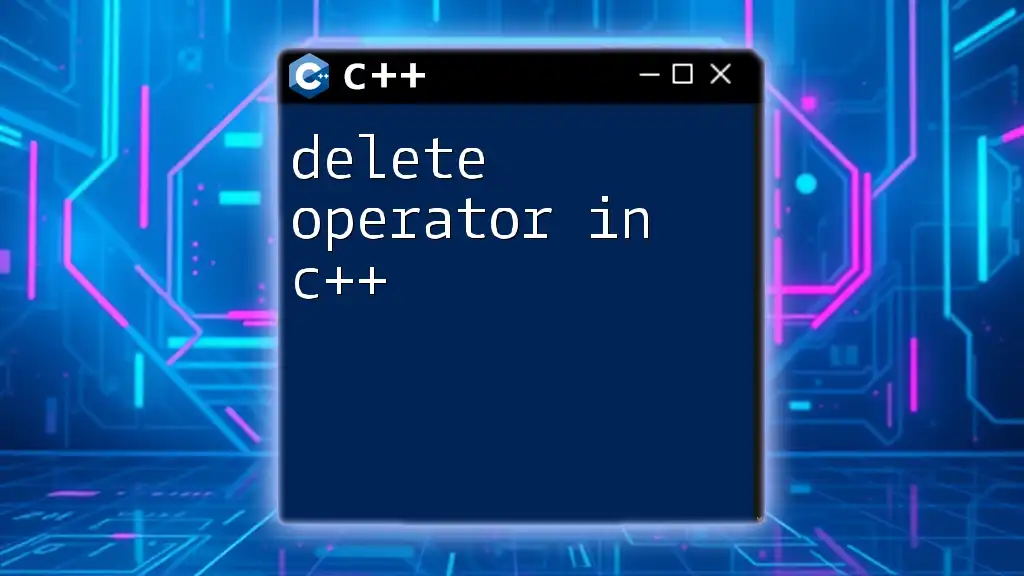
Additional Examples and Exercises
Practice Problem 1: Implementing Your Own Bidirectional Iterator
You could create a simple bidirectional iterator class over your own data structure to consolidate your understanding and apply the principles in a controlled environment.
Practice Problem 2: Enhancing Performance with Iterators
Experiment with using a combination of iterators and algorithms to build more complex data structures or functionalities, focusing on how bidirectional iterators can optimize your implementations.
Exploring bidirectional iterator in C++ not only enriches your understanding of the STL but also empowers you to write cleaner, more efficient code.