A one-dimensional array in C++ is a linear collection of elements of the same type, accessed using a single index, allowing for efficient storage and manipulation of data.
Here’s a simple code snippet demonstrating the declaration, initialization, and access of a one-dimensional array in C++:
#include <iostream>
int main() {
int arr[5] = {10, 20, 30, 40, 50}; // Declaration and initialization
std::cout << arr[2]; // Accessing the third element (30)
return 0;
}
What is a One-Dimensional Array?
A one-dimensional array is a linear collection of elements of the same data type stored in contiguous memory locations. Unlike other data structures like vectors or linked lists, arrays provide a fixed-size storage mechanism that allows quick access to elements via their index.
In a simple analogy, think of a one-dimensional array as a row of boxes, where each box can hold a single value. The position of each box is identified by an index, beginning from 0. This fundamental understanding is crucial for effective programming in C++.
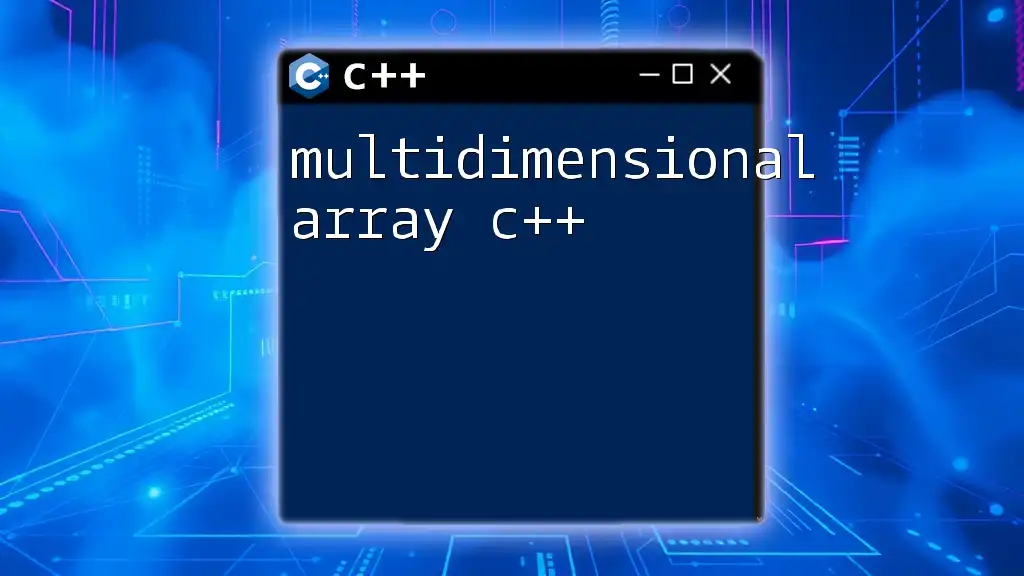
Declaring One-Dimensional Arrays
Syntax for Declaration
To declare a one-dimensional array in C++, you follow a straightforward syntax:
data_type array_name[array_size];
For instance, if you want to declare an array of integers with a size of 5, you'd write:
int numbers[5];
Types of Declaration
You can also declare and initialize an array simultaneously. This is done using the syntax:
data_type array_name[array_size] = {value1, value2, ...};
For example:
int numbers[5] = {1, 2, 3, 4, 5};
Default Values for Arrays
It's essential to understand default values assigned to uninitialized arrays. When you declare an array without initializing it, such as:
int numbers[5]; // Contains garbage values
The contents of `numbers` will be indeterminate, often referred to as garbage values. This emphasizes the importance of always initializing your arrays.
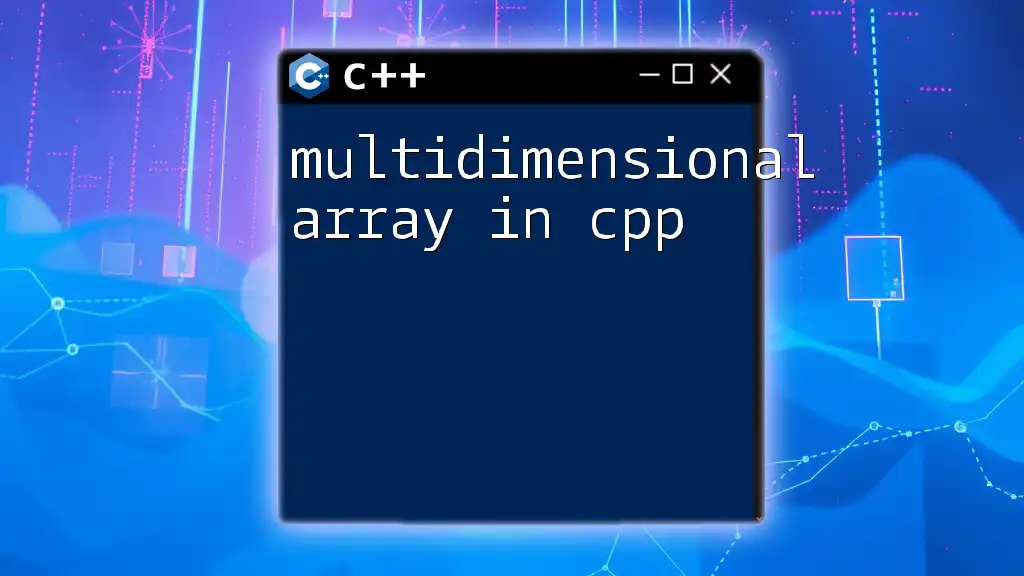
Accessing Elements in a One-Dimensional Array
Indexing Basics
Array elements are accessed using their index, which starts at 0. For example, the first element of the array `numbers` is accessed with `numbers[0]`, the second with `numbers[1]`, and so forth.
Syntax for Accessing Elements
You can not only access array elements but also modify them. The syntax to access and change an element looks like this:
array_name[index] = new_value;
Here's an example to demonstrate this:
numbers[0] = 10; // Changes the first element to 10
Common Errors in Accessing Arrays
Accessing an array with an index that exceeds its bounds leads to undefined behavior. For example, if you have an array of size 5 and attempt to access `numbers[5]`, your program may crash or produce unexpected results. Being cautious about index values is vital for avoiding such errors.
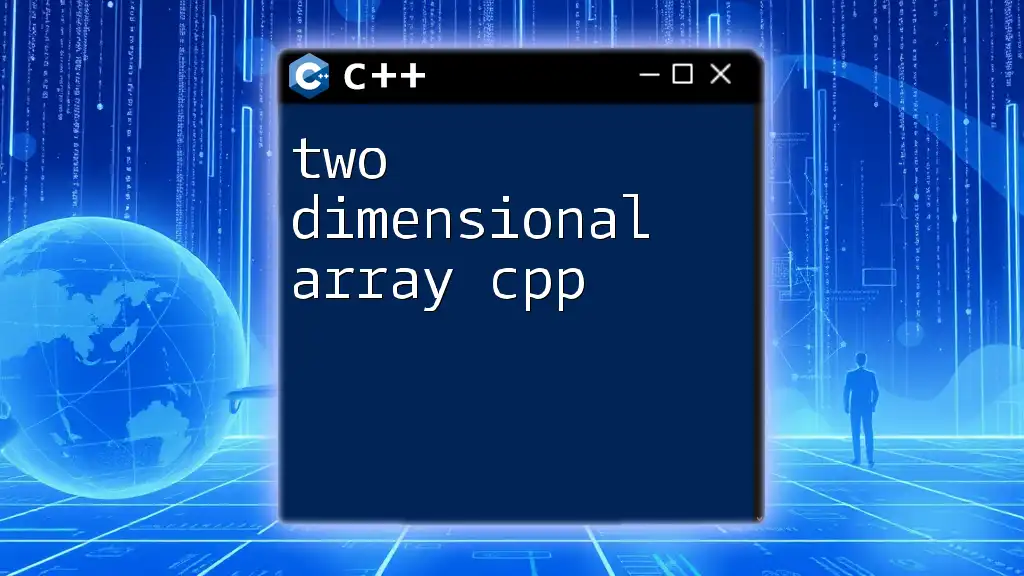
Looping Through One-Dimensional Arrays
Using For Loop
One of the most common techniques for iterating through an array is using a for loop. The following example prints all elements in the `numbers` array:
for (int i = 0; i < 5; i++) {
cout << numbers[i] << " ";
}
Using Range-based For Loop
In modern C++, you can use a range-based for loop, which simplifies syntax and enhances readability. The equivalent operation to print the `numbers` array using this method looks like this:
for (auto &num : numbers) {
cout << num << " ";
}
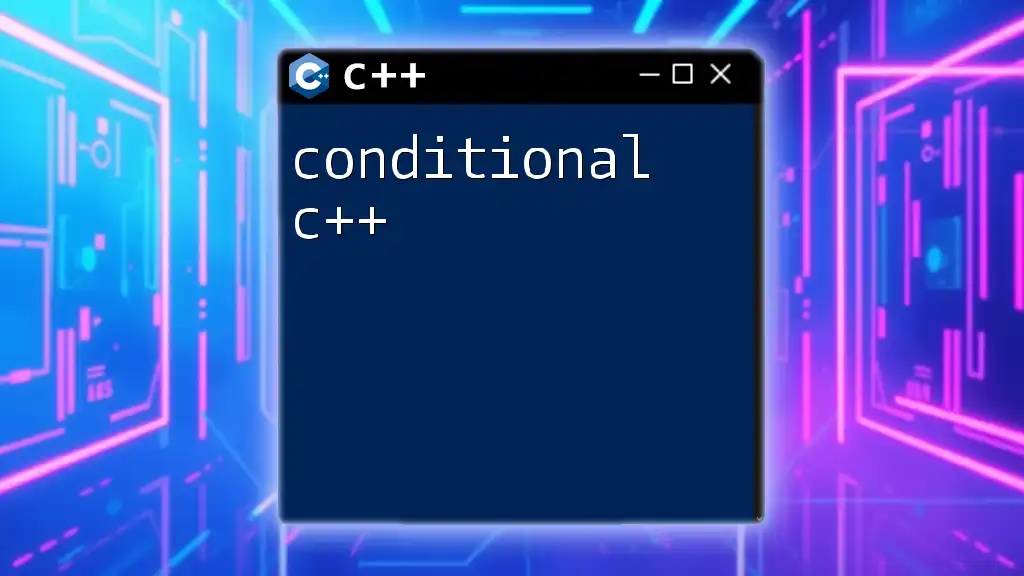
Common Operations on One-Dimensional Arrays
Finding the Length of an Array
You may often need to know the length of an array. This can be calculated in C++ as follows:
int length = sizeof(numbers) / sizeof(numbers[0]);
This snippet uses the `sizeof` operator to determine the total size of the array in bytes divided by the size of a single element, giving you the count of elements in the array.
Searching for an Element
Searching through an array can be accomplished using various algorithms. The linear search algorithm is a simple approach:
bool found = false;
for (int i = 0; i < length; i++) {
if (numbers[i] == searchValue) {
found = true;
break;
}
}
This example looks for `searchValue` within the `numbers` array and sets `found` to true if it is located.
Sorting an Array
Sorting an array is another vital operation. Below is an implementation of the Bubble Sort algorithm:
for (int i = 0; i < length - 1; i++) {
for (int j = 0; j < length - i - 1; j++) {
if (numbers[j] > numbers[j + 1]) {
swap(numbers[j], numbers[j + 1]);
}
}
}
This simple yet effective sorting method compares adjacent elements and swaps them into the correct order.
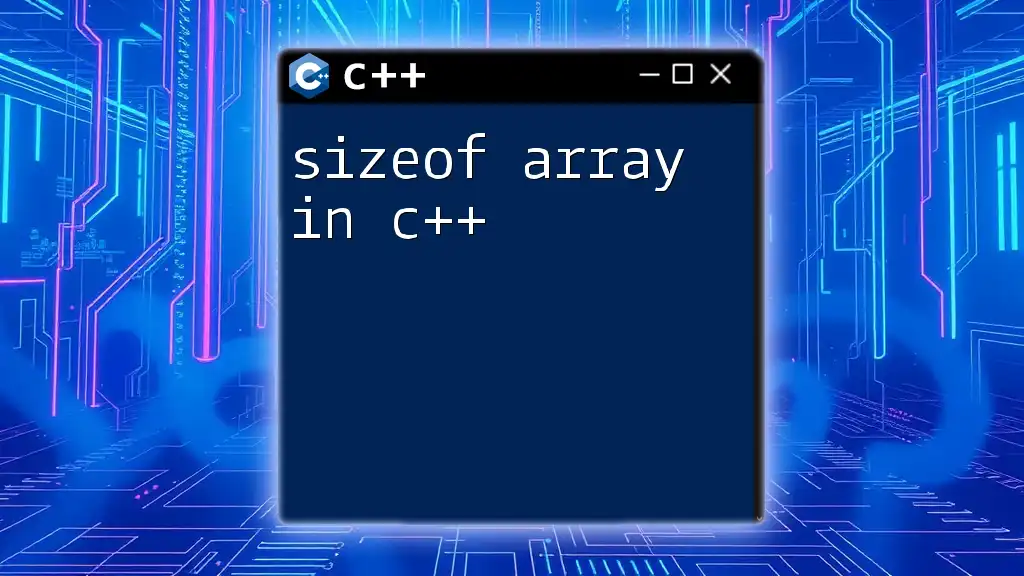
Practical Applications of One-Dimensional Arrays
One-dimensional arrays serve multiple practical purposes in programming. Here are a few common use cases:
- Storing Grades: Arrays can efficiently hold a fixed number of student grades.
- Maintaining Lists: Arrays are excellent for tracking items, like inventory lists.
- Search Simplification: Sorted arrays enable binary search, which drastically reduces the search time.
By leveraging one-dimensional arrays effectively, you can streamline many programming tasks in C++.
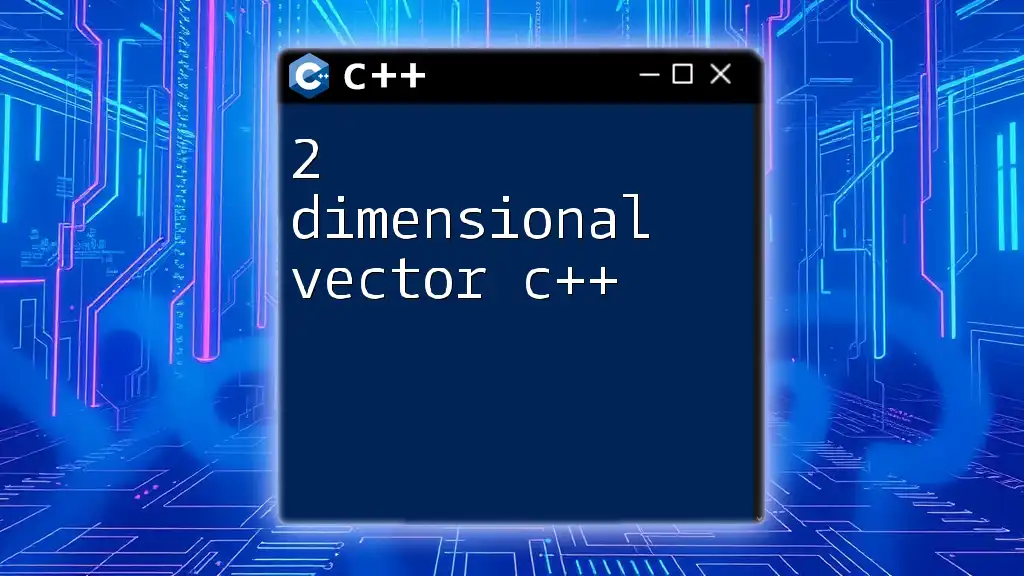
Conclusion
Understanding the concept of a one-dimensional array in C++ is indispensable for any budding programmer. Mastery of array declaration, access, looping, and various operations empowers you to write more efficient and organized code.
To truly grasp these concepts, practice implementing one-dimensional arrays in different scenarios. Be sure to follow the blog for more tutorials and insights on C++ programming!
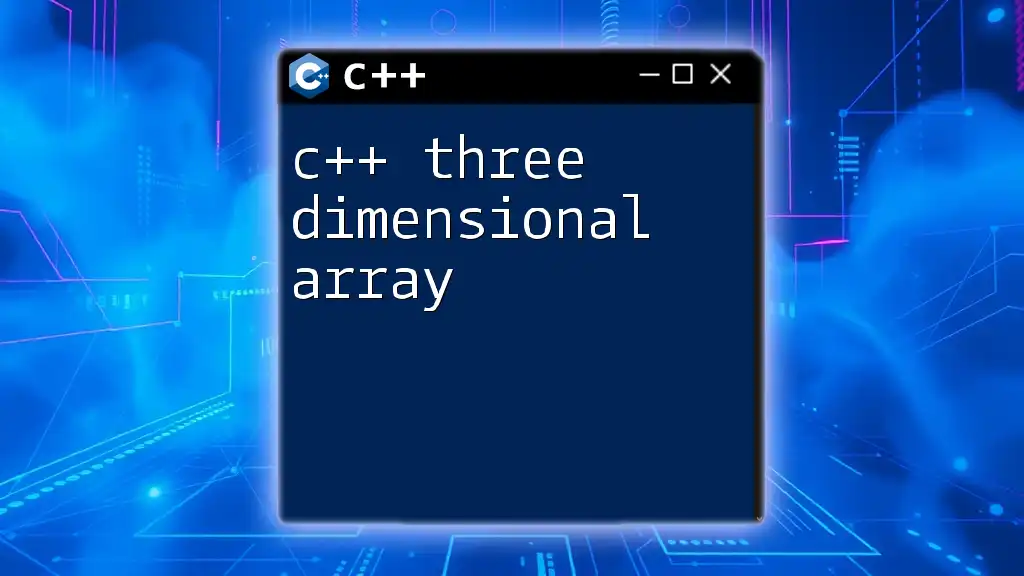
Additional Resources
To further enrich your understanding of one-dimensional arrays and C++, consider exploring documentation and online tutorials. Recommended readings include C++ Primer and Effective C++, both of which provide profound insights into array usage and best practices in C++.