A two-dimensional array in C++ is essentially an array of arrays, allowing you to store data in a grid format using rows and columns. Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
int arr[3][4] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
// Display the 2D array
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 4; j++) {
cout << arr[i][j] << " ";
}
cout << endl;
}
return 0;
}
Understanding Two Dimensional Arrays
What is a Two Dimensional Array?
A two-dimensional array is essentially an array of arrays. It is a data structure that allows you to store elements in a grid-like format defined by rows and columns. This structure is particularly useful for representing data in a matrix form—such as mathematical matrices, tables, or game boards—where elements are logically arranged in two dimensions.
Key Characteristics of 2D Arrays in C++
Two-dimensional arrays in C++ come with several key characteristics:
-
Memory Allocation: When you declare a two-dimensional array, C++ allocates memory for the array elements in a contiguous block. This is beneficial for performance, especially when accessing elements sequentially as it optimizes cache utilization.
-
Data Storage: Each element in a 2D array can be accessed using two indices: the first for the row and the second for the column. This intuitive setup helps in organizing and managing data efficiently.
-
Comparison with One-Dimensional Arrays: While you can represent a 2D structure in a one-dimensional array, using a 2D array simplifies the code, enhances readability, and reduces the chances of errors.
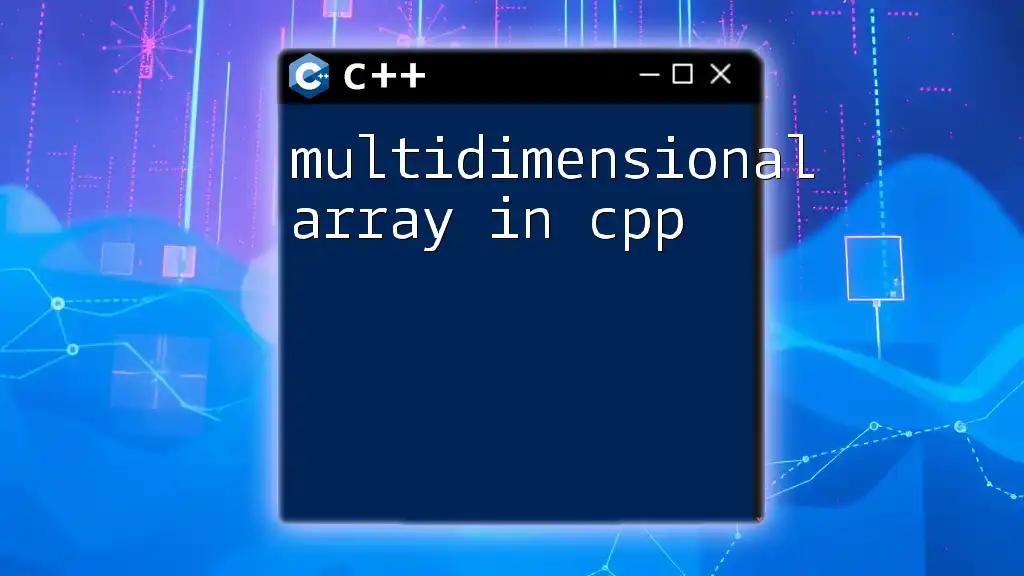
How to Make a 2D Array in C++
Syntax for Declaring a 2D Array
To declare a two-dimensional array in C++, use the following syntax:
type arrayName[rowSize][columnSize];
- `type`: The data type of the array elements (int, float, etc.),
- `rowSize`: The number of rows in the array,
- `columnSize`: The number of columns in the array.
Example of Declaring a 2D Array
Here is a code snippet that demonstrates how to declare a 2D array:
int arr[3][4]; // A 2D array with 3 rows and 4 columns
In this example, `arr` can hold a total of 12 integers (3 rows × 4 columns).
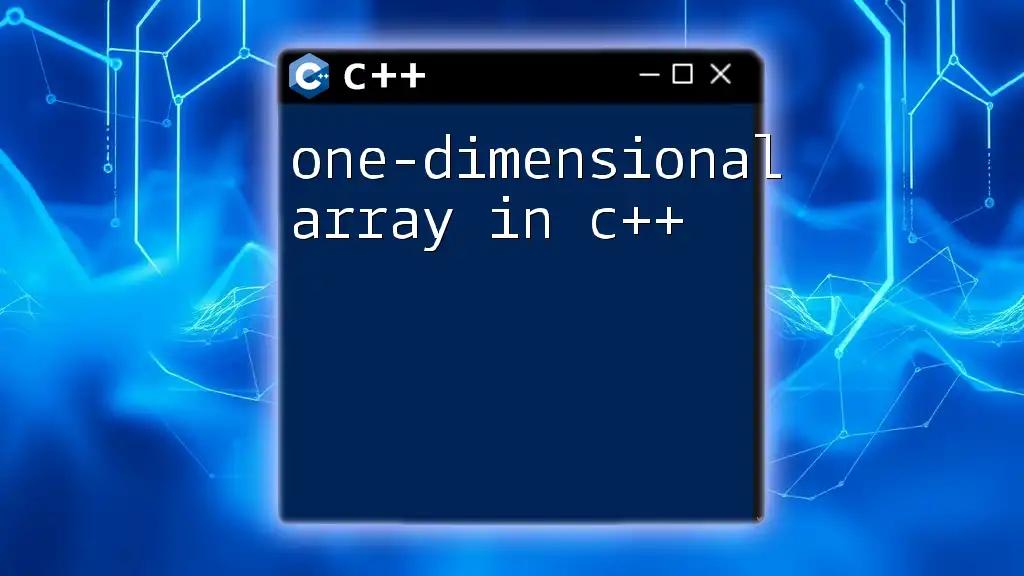
Initializing a Two Dimensional Array
Using Braces for Initialization
You can initialize a two-dimensional array directly using braces:
int arr[2][3] = {{1, 2, 3}, {4, 5, 6}};
In this case, the first row contains the values 1, 2, 3, and the second row contains 4, 5, 6.
Assigning Values After Declaration
Alternatively, you can assign values to the array after it has been declared:
int arr[2][3]; // Declaration
arr[0][0] = 1;
arr[0][1] = 2; // Assigning values
arr[0][2] = 3;
arr[1][0] = 4;
arr[1][1] = 5;
arr[1][2] = 6;
Here, we declare the array first and then assign values to each element explicitly.
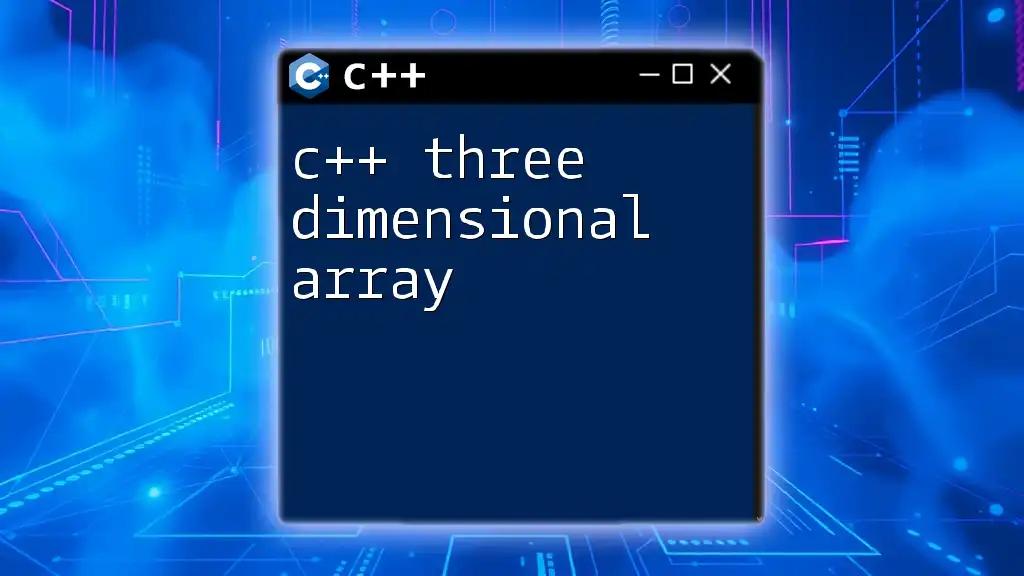
Accessing Elements in a 2D Array
Indexing Methodology for 2D Arrays
Accessing elements in a 2D array requires you to specify the row index and the column index. For example, `arr[i][j]` accesses the element located at the i-th row and j-th column.
Example of Accessing Elements
To display an element from the array, you might use:
std::cout << arr[0][1]; // Accessing specific element
This line of code would output 2, the element located at the first row and second column of the array.

Iterating Through a 2D Array
Using Nested Loops
To iterate through each element of a 2-dimensional array, you can utilize nested loops. The outer loop traverses the rows, while the inner loop traverses the columns.
Here’s how you can do it:
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
std::cout << arr[i][j] << " ";
}
std::cout << std::endl;
}
This code snippet will print all the elements in the 2D array in a structured format.
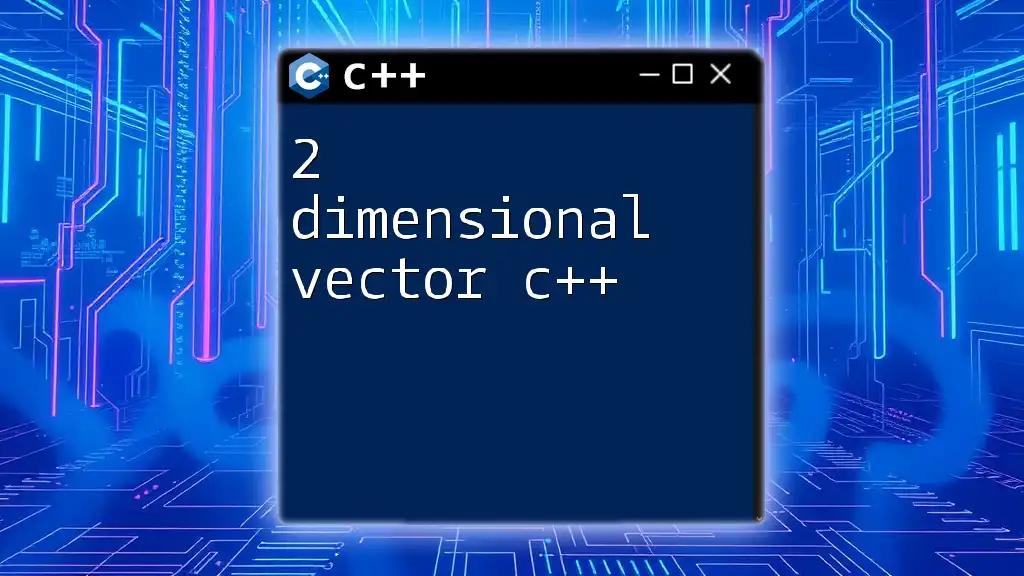
Practical Applications of 2D Arrays
Use Cases in Real-World Scenarios
Two-dimensional arrays have numerous practical applications, some of which include:
-
Image Processing: Pixels in images are often represented as a 2D array, where each element corresponds to the color of a pixel.
-
Game Development: Game boards, grids, and maps can be represented using 2D arrays, facilitating easy management of positions and objects.
-
Mathematical Computations: Many algorithms in linear algebra require matrix operations, which can be efficiently handled using 2D arrays.
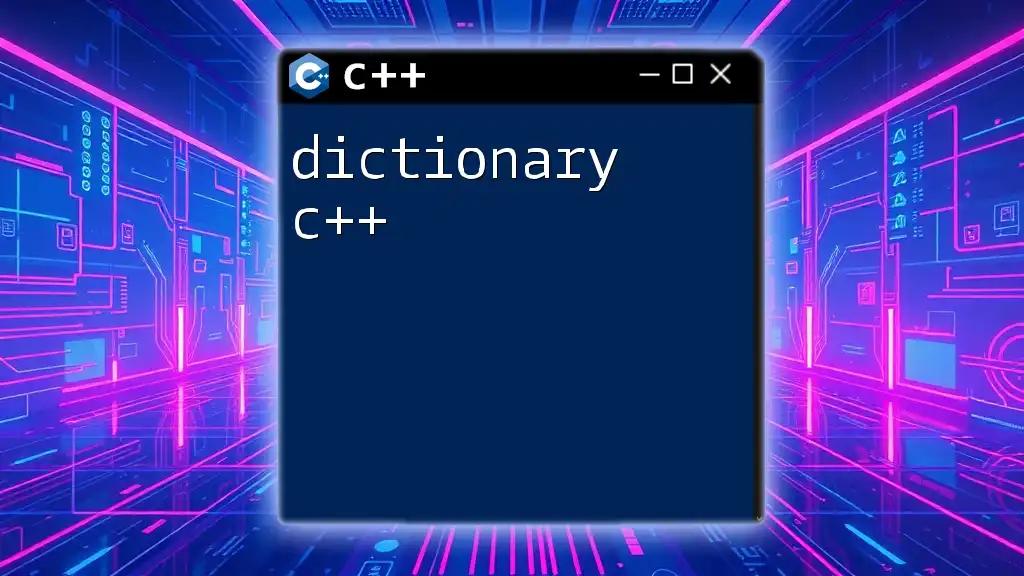
Common Errors and Troubleshooting
Frequently Encountered Issues
When working with two-dimensional arrays, common errors include:
-
Index Out of Bounds: Attempting to access an element outside of the defined array limits will result in undefined behavior.
-
Initialization Mistakes: Failing to initialize elements can lead to unexpected results when processing the array.
Tips for Avoiding Errors
To prevent issues:
- Use constants for defining row and column sizes, enhancing readability and maintainability.
- Always test array boundaries to ensure you don't exceed the defined limits during access or iteration.
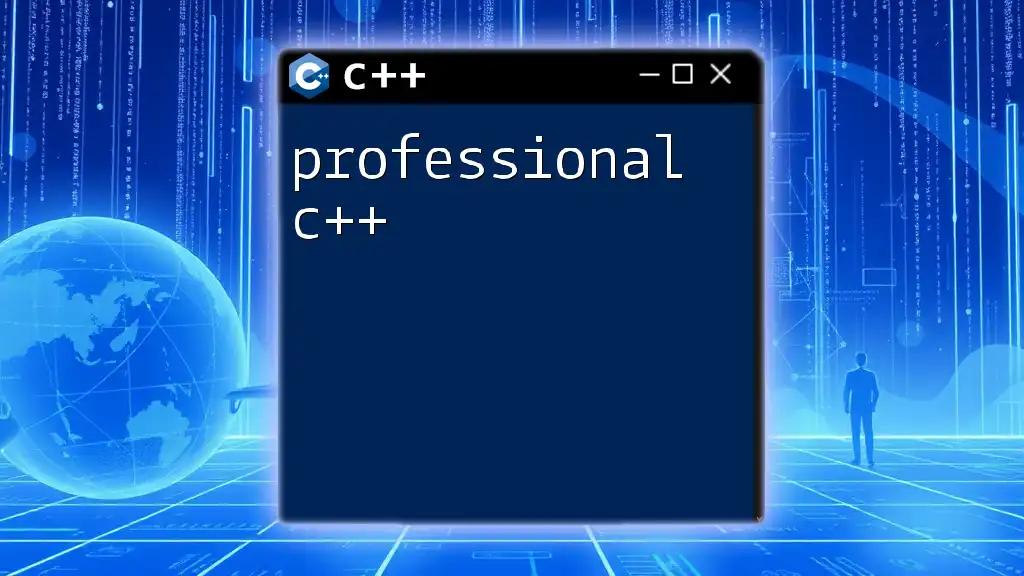
Conclusion
Mastering the use of a two-dimensional array in C++ opens the door to efficiently managing complex data structures. This powerful feature enables developers to manipulate grid-based data intuitively, paving the way for applications in various domains, from game design to complex computations. By understanding how to declare, initialize, access, and iterate through 2D arrays, you'll harness the full potential of this versatile data structure. Explore beyond this foundational knowledge, and you’ll find endless possibilities in C++ programming.
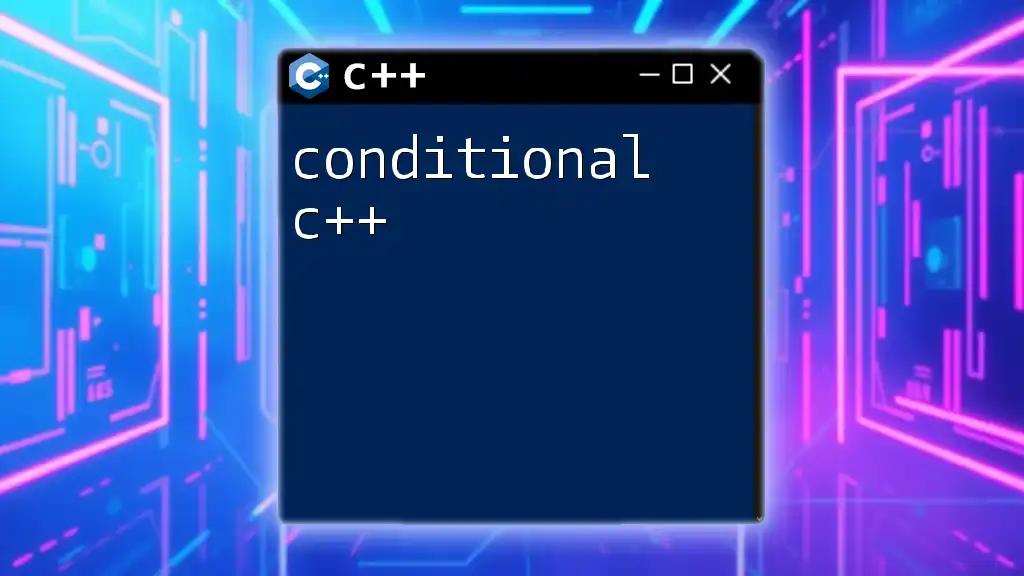
Further Reading and Resources
For additional resources, consider checking the C++ documentation, online tutorials, or specialized courses focused on advanced topics like dynamic 2D arrays or pointers in C++. Expanding your understanding will only enhance your programming skill set. Happy coding!