A boolean array in C++ is an array that stores boolean values (`true` or `false`), which can be used for various purposes such as flags or binary states.
Here's a simple example of a boolean array:
#include <iostream>
int main() {
bool flags[5] = {true, false, true, false, true}; // Declare a boolean array
for (int i = 0; i < 5; i++) {
std::cout << "flags[" << i << "] = " << std::boolalpha << flags[i] << std::endl; // Print each value
}
return 0;
}
Understanding Boolean Arrays in C++
What is a Boolean Array?
A boolean array in C++ is a type of array that stores boolean values, which can either be `true` or `false`. In programming, arrays are a collection of similar types of elements. In the case of boolean arrays, they hold multiple boolean values, allowing for efficient storage and manipulation of conditions or flags.
Why Use Boolean Arrays?
Boolean arrays serve various purposes in programming due to their compactness and efficiency. Here are some reasons for their use:
- Simplicity: They provide a straightforward way to manage multiple yes/no conditions.
- Efficiency: When dealing with binary states, boolean arrays minimize memory usage.
- Flexibility: They can be easily manipulated using loops and logical operations, making them ideal for applications like state representation, flagging systems, and more.
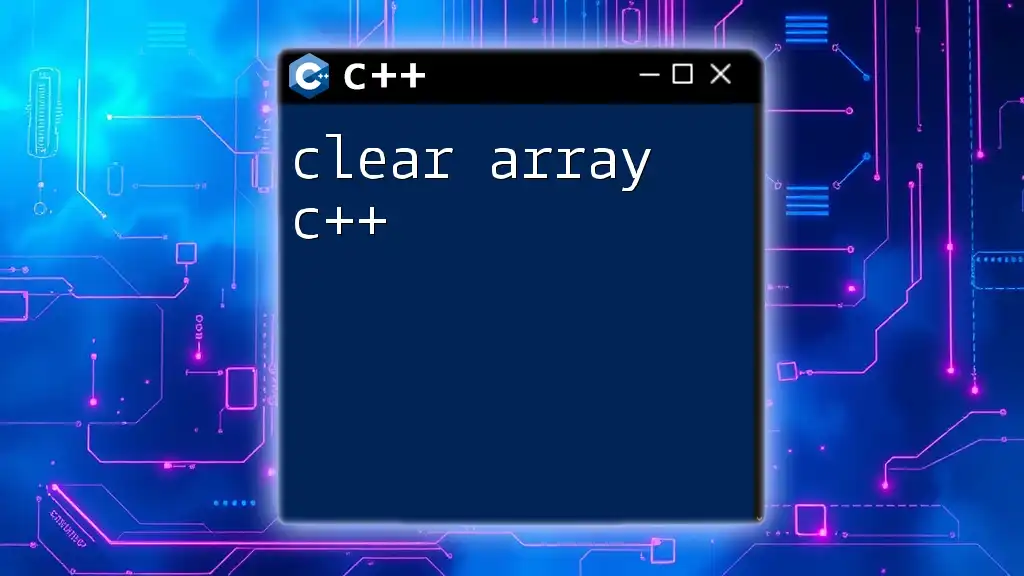
Declaring a Boolean Array in C++
Syntax for Declaring Boolean Arrays
To declare a boolean array in C++, the syntax is simple:
bool arrayName[arraySize];
For example, to declare a boolean array named `flags` of size 5, the declaration would look like this:
bool flags[5];
Initializing Boolean Arrays
You can initialize a boolean array at the time of declaration in different ways:
- Using a list of values:
bool flags[5] = {true, false, true, false, true};
- Assigning values individually:
bool flags[5];
flags[0] = true;
flags[1] = false;
flags[2] = true;
flags[3] = false;
flags[4] = true;
Both methods effectively create a boolean array, but initialization at the declaration can save effort and ensure clarity.
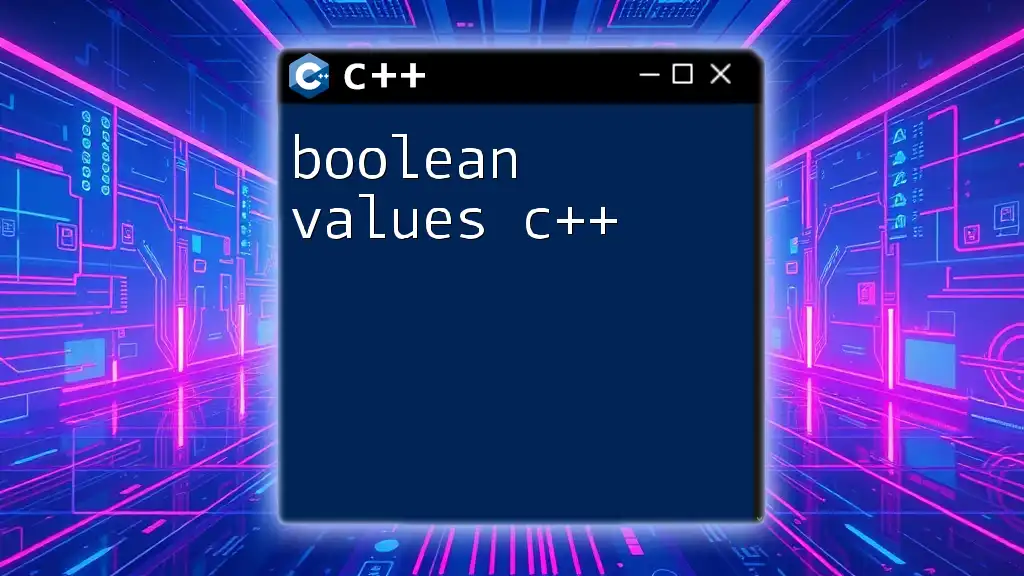
Accessing and Modifying Boolean Arrays
Accessing Elements in a Boolean Array
Accessing individual elements in a boolean array is done using the index of the element. For example, to access the first element from the `flags` array, you will use:
bool firstFlag = flags[0]; // This will be 'true'
Modifying Elements in a Boolean Array
Modifying a boolean array is straightforward. You can simply assign a new value to any index:
flags[1] = true; // Modify the second element to 'true'
After executing this line, `flags[1]` will now store `true` instead of `false`.
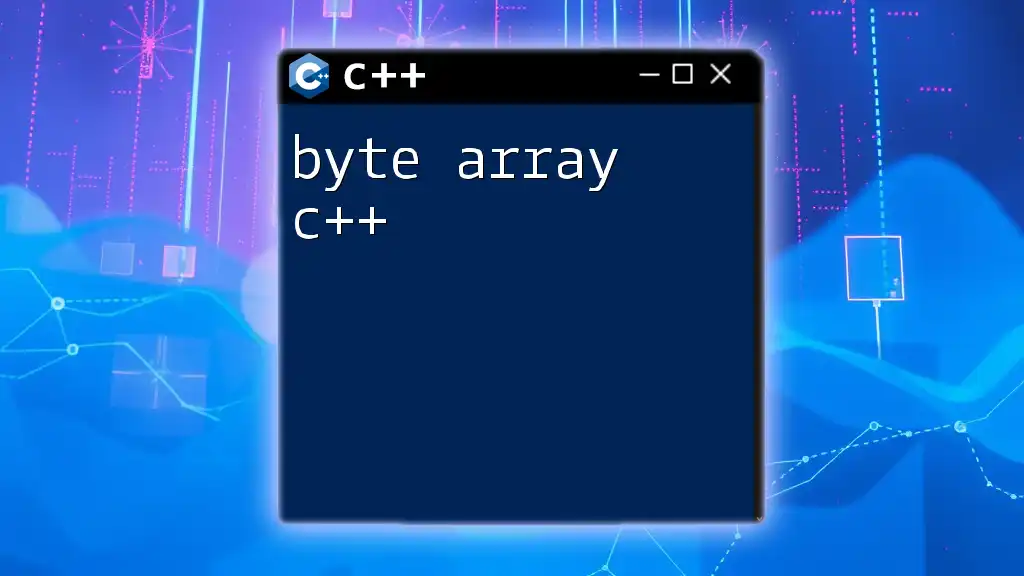
Common Operations on Boolean Arrays
Iterating through a Boolean Array
Looping through a boolean array to access or manipulate its elements is common. For instance, you can use a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << "Flag at index " << i << " is " << flags[i] << std::endl;
}
This loop will print the value of each element in the `flags` array.
Logical Operations with Boolean Arrays
Performing logical operations on boolean arrays can simplify complex decision-making processes. For instance, you can use logical conditions:
if (flags[0] && flags[1]) {
std::cout << "Both flags are true!" << std::endl;
}
Counting True or False Values
Counting how many values in a boolean array are `true` can be pivotal in many algorithms. You can achieve this using a simple function:
int countTrue(bool arr[], int size) {
int count = 0;
for (int i = 0; i < size; i++) {
if (arr[i]) {
count++;
}
}
return count;
}
This function iterates through the array and increments the `count` variable for each `true` value it encounters.
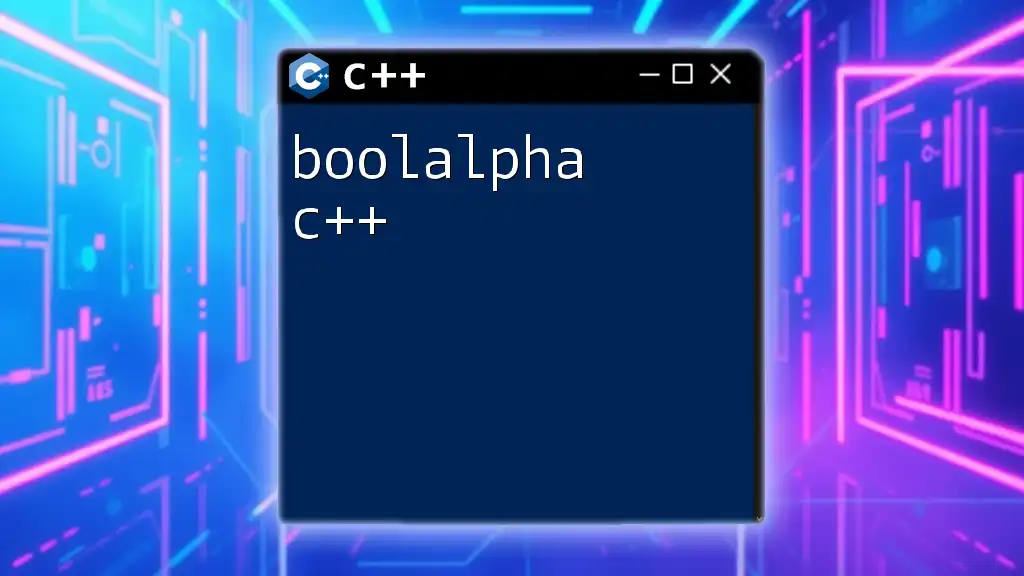
Practical Applications of Boolean Arrays
Use Case: Binary Flags
Boolean arrays can effectively represent binary flags — this is especially useful in configurations or settings. For example, a boolean array could indicate the availability of multiple features in a software application:
bool features[3] = {true, false, true}; // Feature 1 and Feature 3 are enabled
Use Case: Boolean Matrix
Boolean arrays can also be used to create matrices, often employed in graph algorithms to represent adjacency matrices. For instance, an adjacency matrix for a graph can be implemented as:
bool adjacencyMatrix[4][4] = {
{false, true, false, false},
{true, false, true, false},
{false, true, false, true},
{false, false, true, false}
};
In this example, the graph has four nodes, and the `true` values represent connections between nodes.
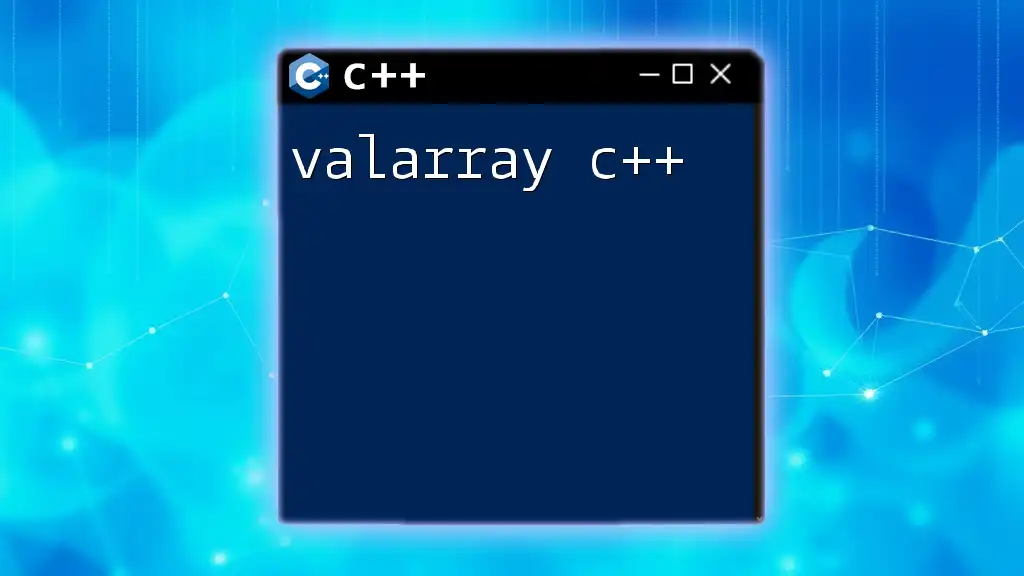
Best Practices for Using Boolean Arrays
Memory Management Considerations
While boolean arrays are efficient in terms of space, ensuring that they aren't declared with excessive size is crucial. If you know only a few flags will be utilized, consider dynamically allocating memory based on runtime requirements.
bool* dynamicFlags = new bool[numberOfFlags];
Always remember to free up dynamically allocated memory using `delete[]`.
Error Handling in Boolean Arrays
One of the common pitfalls when dealing with boolean arrays is out-of-bounds access. Always ensure that you check the size of the array before accessing indices:
if (index >= 0 && index < arraySize) {
// Safe access
bool value = flags[index];
}
This practice prevents runtime errors and ensures your program runs smoothly.
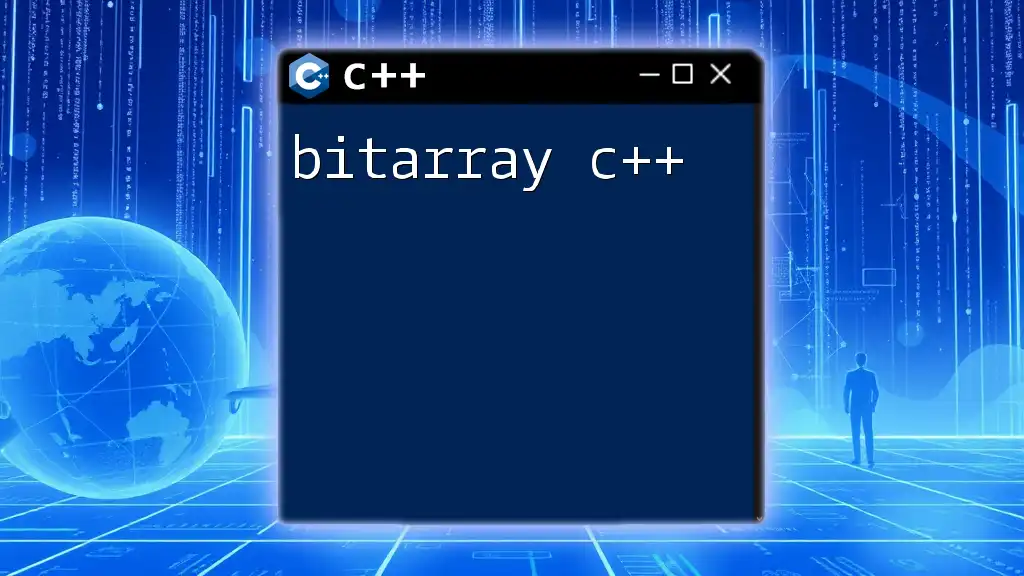
Summary and Conclusion
In summary, a boolean array in C++ is a powerful structure that facilitates the organization and manipulation of binary data efficiently. By embracing their uses, such as binary flags and boolean matrices, developers can streamline their programming practices for optimal condition handling. Always keep in mind best practices around memory management and error handling to utilize boolean arrays effectively.
Further Learning Resources
To deepen your understanding of boolean arrays and C++ programming, consider exploring various online resources, community forums, and programming books dedicated to C++. The more you practice with boolean arrays, the more intuitive they will become in your C++ programming journey.