In C++, a byte array is typically defined using `std::array<uint8_t, N>` where `N` is the size of the array, allowing for efficient storage and manipulation of binary data.
Here’s a code snippet demonstrating how to define and initialize a byte array:
#include <array>
#include <cstdint>
#include <iostream>
int main() {
std::array<uint8_t, 5> byteArray = {0xFF, 0x00, 0xAB, 0x3C, 0x7D};
for (const auto& byte : byteArray) {
std::cout << std::hex << static_cast<int>(byte) << " ";
}
return 0;
}
Understanding the Basics of Byte Arrays in C++
What is a Byte?
A byte is a fundamental unit of digital information that consists of 8 bits. In C++, bytes are often represented by the `unsigned char` data type, which can hold values ranging from 0 to 255. Understanding bytes and how they interact with various data types is crucial when learning about byte arrays in C++. Byte arrays can store collections of byte values and are particularly useful for low-level programming tasks such as memory management and data serialization.
Declaring Byte Arrays in C++
To declare a byte array in C++, you typically use the following syntax:
unsigned char byteArray[10]; // Declaration of a byte array with 10 elements
In this case, `byteArray` is an array that can hold 10 bytes. It is important to choose the size of your byte array thoughtfully, as undersized arrays may lead to buffer overflow issues, while oversized arrays can waste memory.
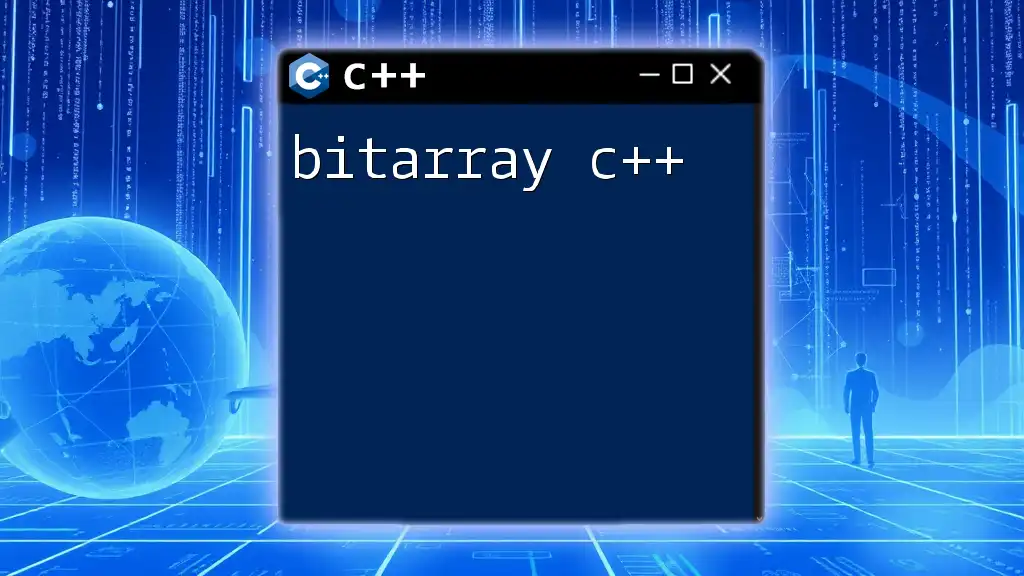
Accessing and Manipulating Byte Arrays
Accessing Byte Array Elements
Accessing elements in a byte array is done through indexing, similar to accessing elements in other array types. For example:
byteArray[0] = 255; // Assigning value to the first element
Here, we assign the maximum value a byte can hold to the first element of the array. It’s crucial to remember that array indexing starts at zero, meaning accessing an index out of bounds can cause undefined behavior.
Modifying Byte Array Elements
Once you’ve assigned values to your byte array, modifying those values is straightforward:
byteArray[1] = byteArray[0] / 2; // Modifying the second element
In this example, we take the value of the first element, perform a calculation, and then store the result in the second element. Such manipulations highlight the simplicity and flexibility of working with byte arrays.
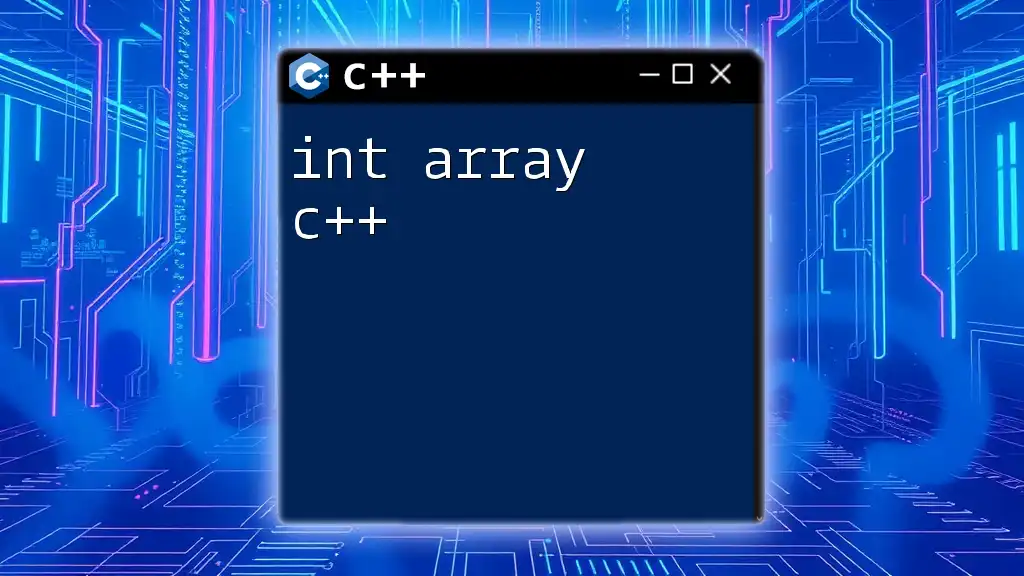
Common Use Cases for Byte Arrays in C++
Buffers and Data Transmission
One of the most common use cases for byte arrays in C++ is in networking and data transmission. Byte arrays serve as buffers that temporarily store data being sent or received over a network socket.
For example, when receiving data from a socket, you might use a byte array to hold the incoming byte stream. The following code illustrates this concept:
unsigned char buffer[256]; // Buffer for incoming data
recv(socket, buffer, sizeof(buffer), 0); // Receive data into the buffer
Here, `recv` populates the byte array `buffer` with data from a connected socket. Understanding how to manage such byte arrays is vital for effective network communication.
File Operations
Another important application of byte arrays is in file handling. You can read from and write to binary files using byte arrays, enabling you to handle raw data efficiently.
Consider this example of reading from a binary file:
std::ifstream file("data.bin", std::ios::binary);
file.read(reinterpret_cast<char*>(byteArray), sizeof(byteArray));
file.close();
In this snippet, the file `data.bin` is read into the `byteArray`, bridging the gap between file storage and memory representation. Binary file operations often rely on byte arrays for their seamless handling of raw byte data.
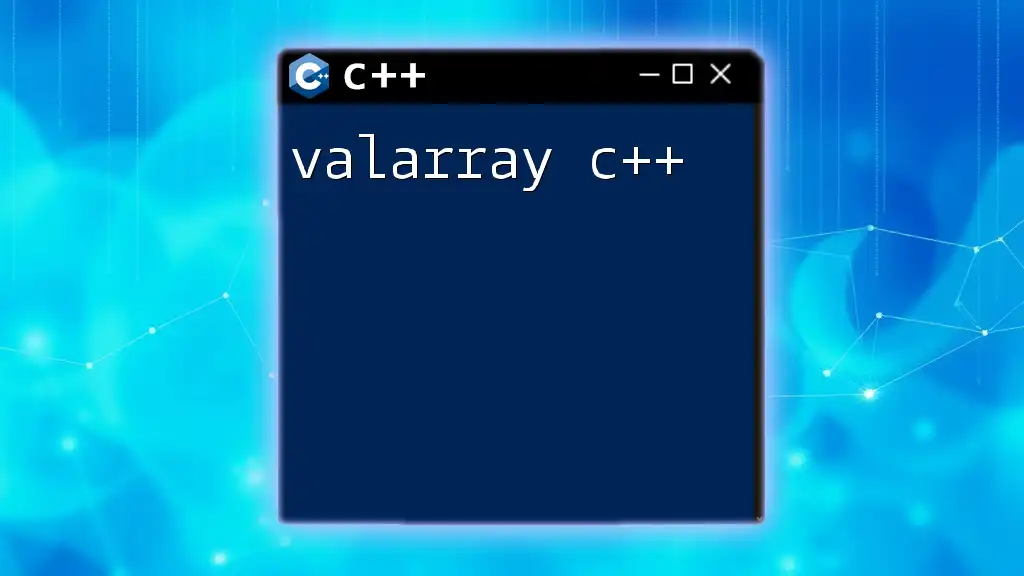
Converting Between Data Types and Byte Arrays
Integer to Byte Array Conversion
Converting integers to byte arrays is essential when you need to manipulate binary representations. C++ allows for bit manipulation to achieve this.
For instance:
int num = 1024;
byteArray[0] = (num >> 24) & 0xFF; // Get the first byte
In this example, we shift `num` 24 bits to the right and mask it with `0xFF` to extract the most significant byte. Such conversions are crucial in applications like serialization and network communication.
Byte Array to String Conversion
Sometimes you'll need to convert a byte array to a string, especially when dealing with text data. This can be achieved as follows:
std::string byteString(reinterpret_cast<char*>(byteArray), sizeof(byteArray));
This snippet constructs a string from the byte array, effectively allowing you to manipulate or display the byte data as text.
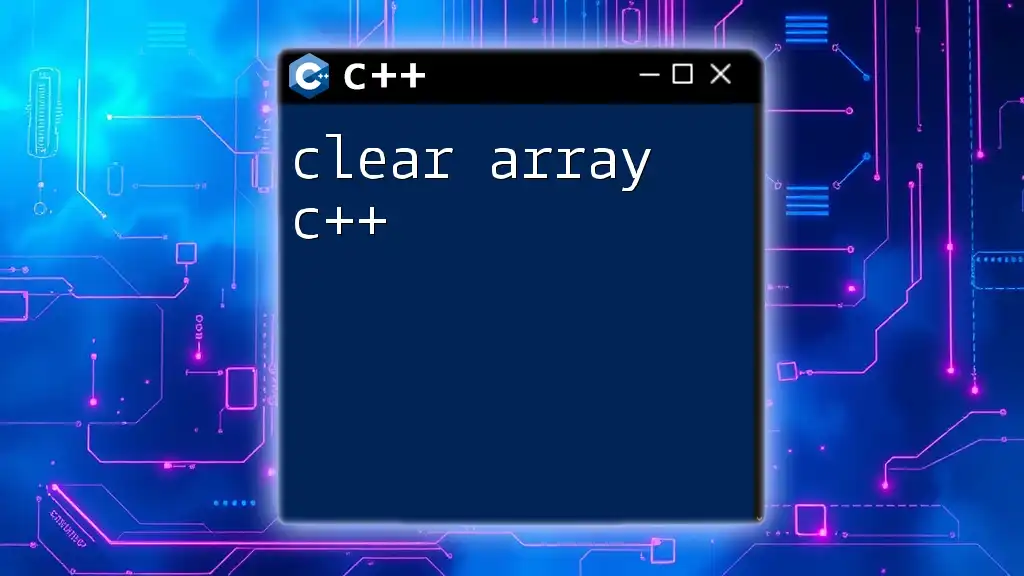
Best Practices for Using Byte Arrays in C++
Memory Management Considerations
Memory management is critical when dealing with byte arrays in C++. Always ensure that the size of the byte array is appropriate for your application context. Pay attention to the following:
- Avoid buffer overflows by performing bounds checks when accessing elements.
- Use smart pointers or containers like `std::vector` for dynamic byte arrays, which automatically manage memory and reduce the risk of leaks.
Performance Optimization
To optimize performance when using byte arrays:
- Prefer using static arrays when the size is known at compile time for improved speed and reduced allocation overhead.
- Choose dynamic arrays cautiously, as they can introduce additional complexity and overhead due to memory allocation.
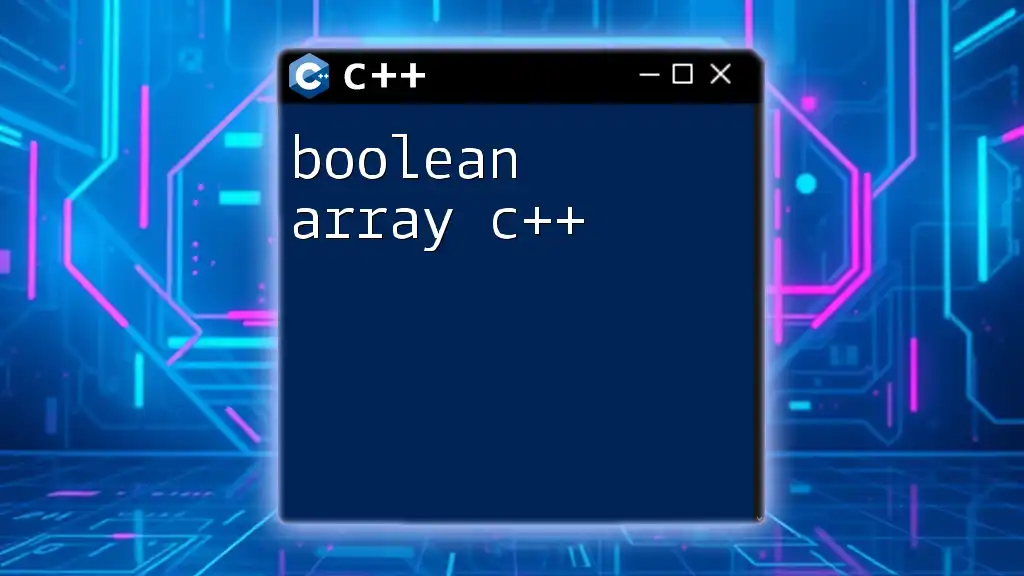
Debugging Byte Arrays
Common Errors with Byte Arrays
Working with byte arrays can introduce several common pitfalls:
- Array Out of Bounds: Accessing or modifying elements outside the declared size can lead to undefined behavior.
- Type Mismatches: Using incompatible types can cause incorrect data to be processed or interpreted.
For instance, indexing an array beyond its limit can cause segmentation faults or corruption of adjacent memory.
Debugging Techniques
To effectively debug byte arrays:
- Use nullptr checks and array size conditions before performing operations to catch errors before they occur.
- Leverage tools such as gdb, integrated debugging environments, and assertions to trace and inspect the state of your byte array during execution.
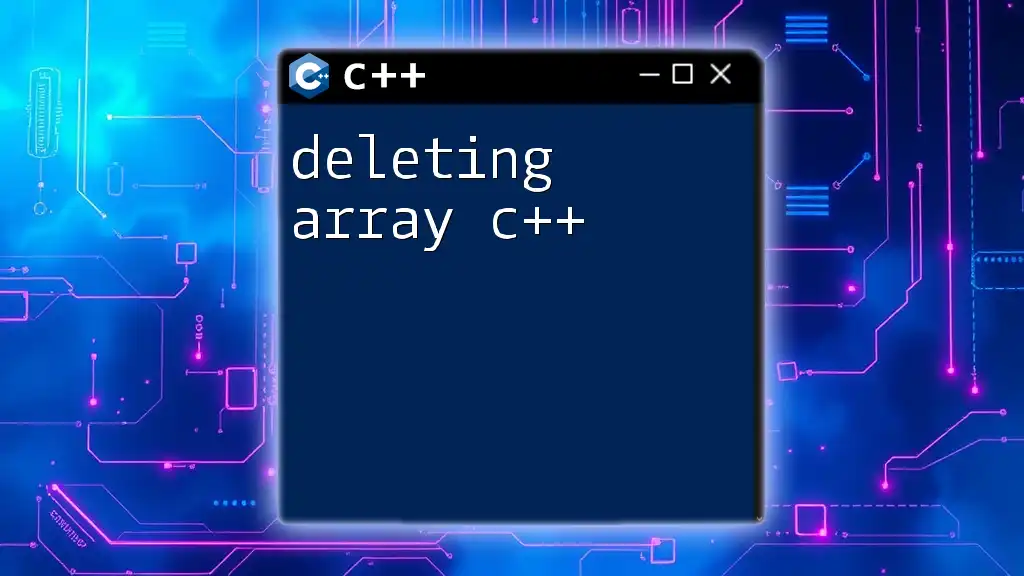
Conclusion
Understanding and effectively utilizing byte arrays in C++ is paramount for many technical applications, from file handling to network programming. By mastering the principles outlined above, you can enhance your programming skills and develop a deeper comprehension of memory management and performance optimization.
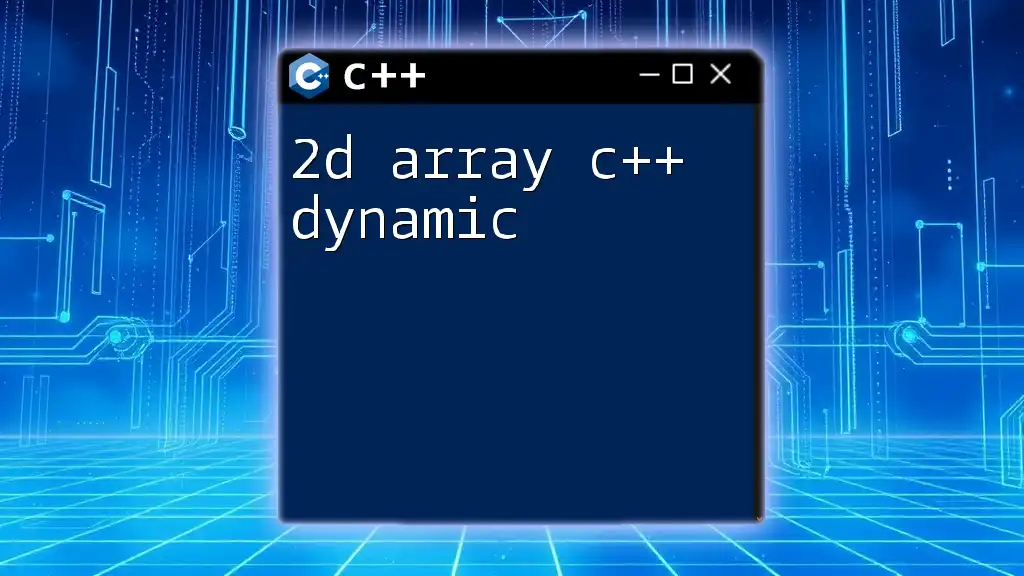
Additional Resources
For further reading, consider online resources such as C++ documentation, programming forums, and tutorials focused on low-level programming with byte arrays. Libraries like Boost or frameworks for networking can offer valuable tools and abstractions for working with byte arrays.
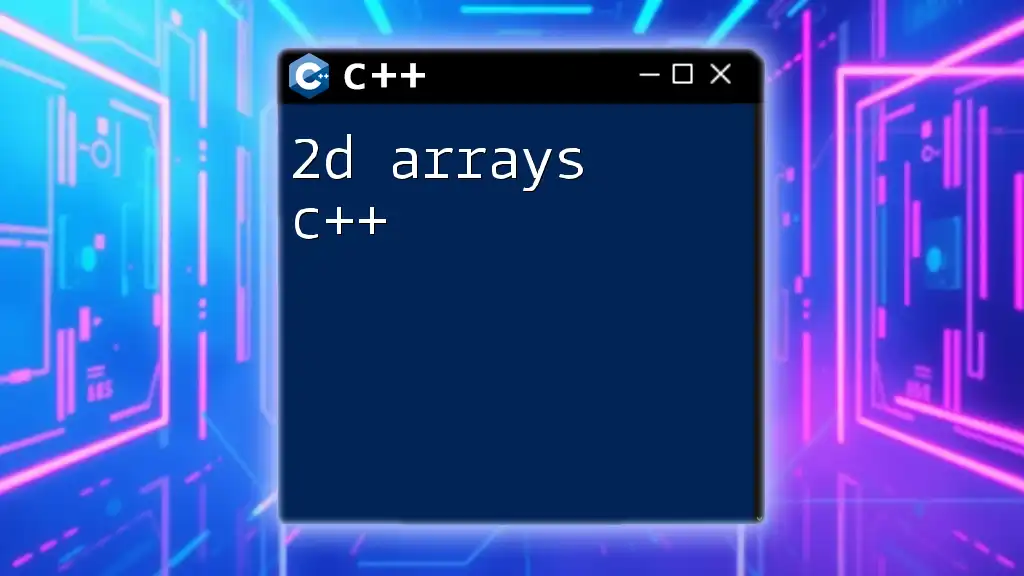
Call to Action
We encourage you to explore your own experiences with byte arrays in C++ and share your insights or challenges. Join discussions on platforms dedicated to C++ programming to broaden your understanding and application of this essential concept in your projects.