2D arrays in C++ are arrays of arrays that allow you to store data in a grid-like structure, typically used for matrices and multi-dimensional data.
#include <iostream>
using namespace std;
int main() {
int matrix[3][4] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 4; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Understanding C++ Two Dimensional Array
Two-dimensional arrays are essentially arrays of arrays, allowing storage of data in a grid format. Think of them as a table, where you can access data using two indices: one for the row and one for the column. This is particularly useful in various programming scenarios, such as representing matrices for mathematical calculations, creating grids for games, or managing image data.
Syntax for Declaring 2D Arrays in C++
Declaring a 2D array in C++ is simple and follows a standard syntax. Here's how you can do it:
data_type array_name[rows][columns];
For example, if you want to create a 3-row by 4-column integer matrix, you would write:
int matrix[3][4];
This statement allocates memory for a 3x4 matrix of integers.
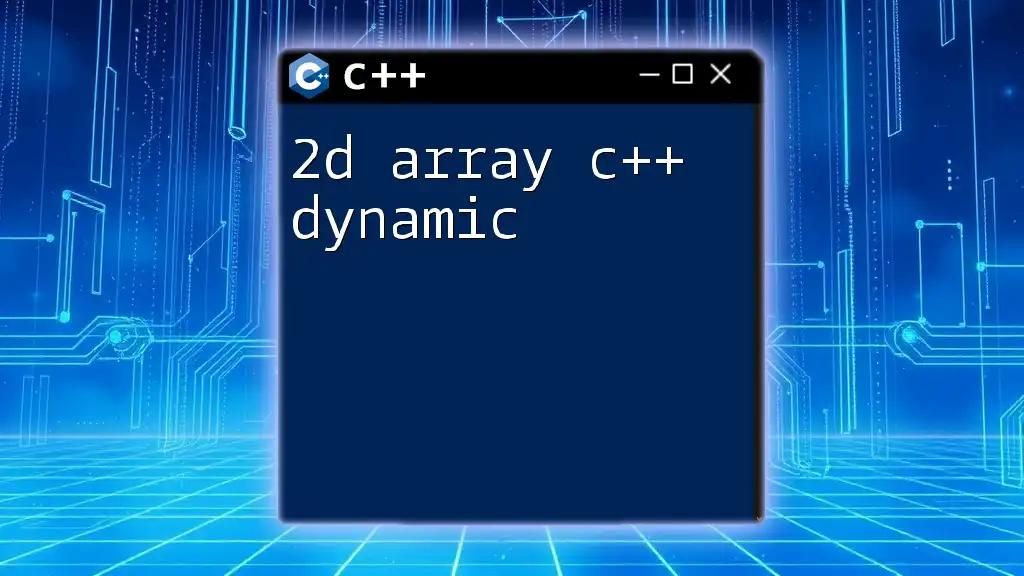
Initializing 2D Arrays in C++
Static Initialization of C++ 2D Arrays
Static initialization allows direct assignment of values to the array upon declaration. This is particularly useful when you know the values beforehand. Here's how you can initialize a 2D array statically:
int matrix[2][3] = {
{1, 2, 3},
{4, 5, 6}
};
In this example, the first row contains integers 1, 2, and 3, while the second row contains 4, 5, and 6.
Dynamic Initialization of 2D Arrays in C++
Dynamic allocation is done using pointers and `new` operator, which is helpful when the size of the array is not known at compile time or for large datasets.
int** matrix;
int rows = 3, columns = 4;
matrix = new int*[rows]; // Allocate memory for rows
for (int i = 0; i < rows; ++i) {
matrix[i] = new int[columns]; // Allocate memory for each column in the respective row
}
After the array is no longer needed, it’s essential to free the allocated memory to prevent memory leaks:
for (int i = 0; i < rows; ++i) {
delete[] matrix[i]; // Free each row
}
delete[] matrix; // Free the array of pointers
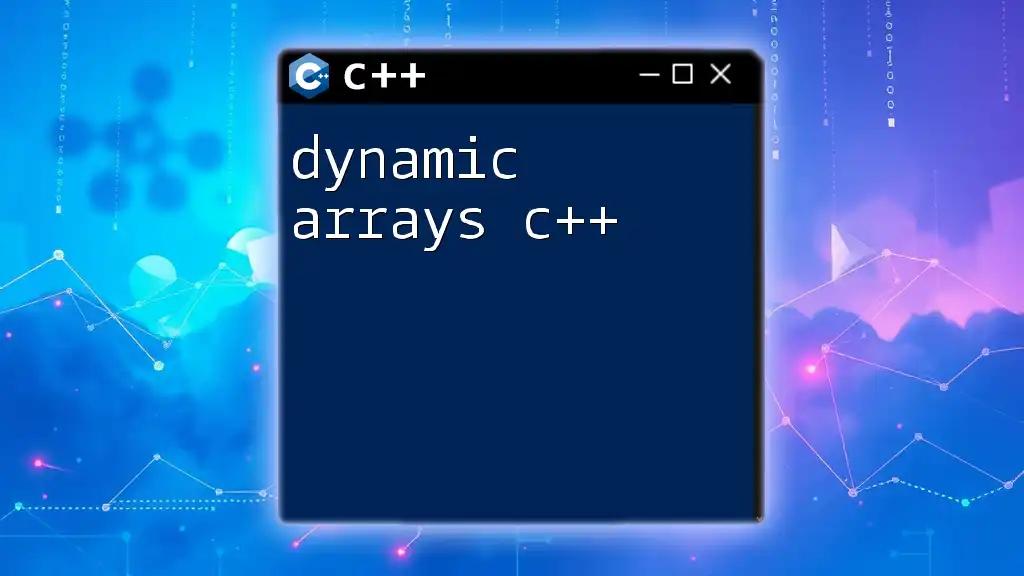
Accessing Elements in 2D Arrays
How to Access Elements in a C++ 2D Array
You can access individual elements in a 2D array in C++ using the syntax `array_name[row_index][column_index]`. For example, to access the element located at the first row and second column:
int val = matrix[0][1]; // Accesses the element at row 0, column 1
Modifying Elements in C++ 2D Arrays
Modifying the value of an element is just as straightforward. You can assign a new value using the same indexing method. Here’s an example:
matrix[1][2] = 10; // Changes the element at row 1, column 2 to 10
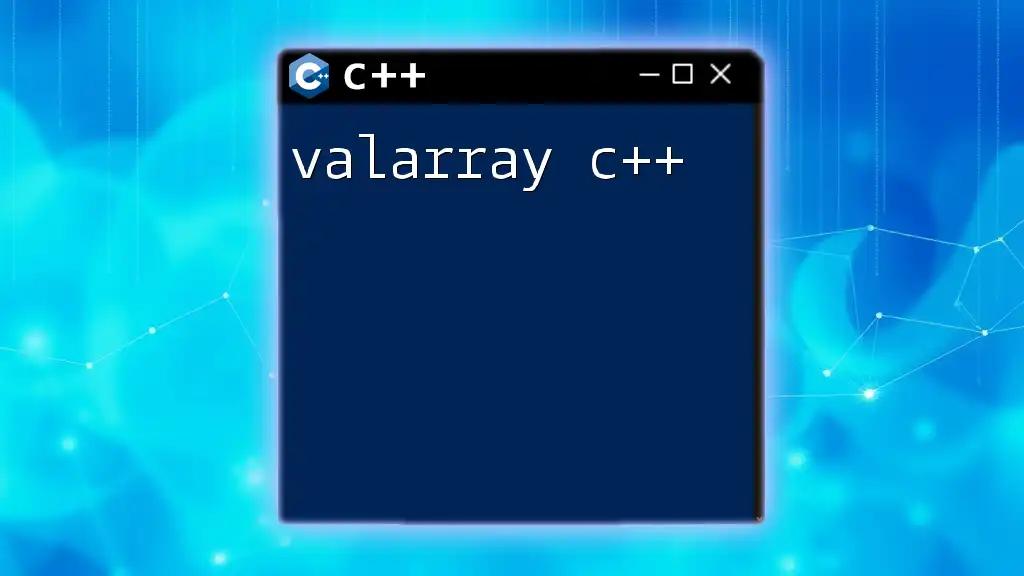
Iterating Through 2D Arrays in C++
Using Nested Loops to Traverse 2D Arrays
To process or display each element, nested loops are commonly used. The outer loop iterates through the rows, while the inner loop iterates through the columns. Here’s an example of printing the values of a 2D array:
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < columns; ++j) {
cout << matrix[i][j] << " "; // Print each element followed by a space
}
cout << endl; // New line after each row
}
Range-Based For Loops with C++ 2D Arrays
In modern C++, you can leverage range-based for loops to simplify the iteration process. Here’s how you can do it:
for (auto& row : matrix) {
for (auto& element : row) {
cout << element << " "; // Print each element in the row
}
cout << endl; // New line after each row
}
This method enhances code readability and reduces potential mistakes associated with index-based access.
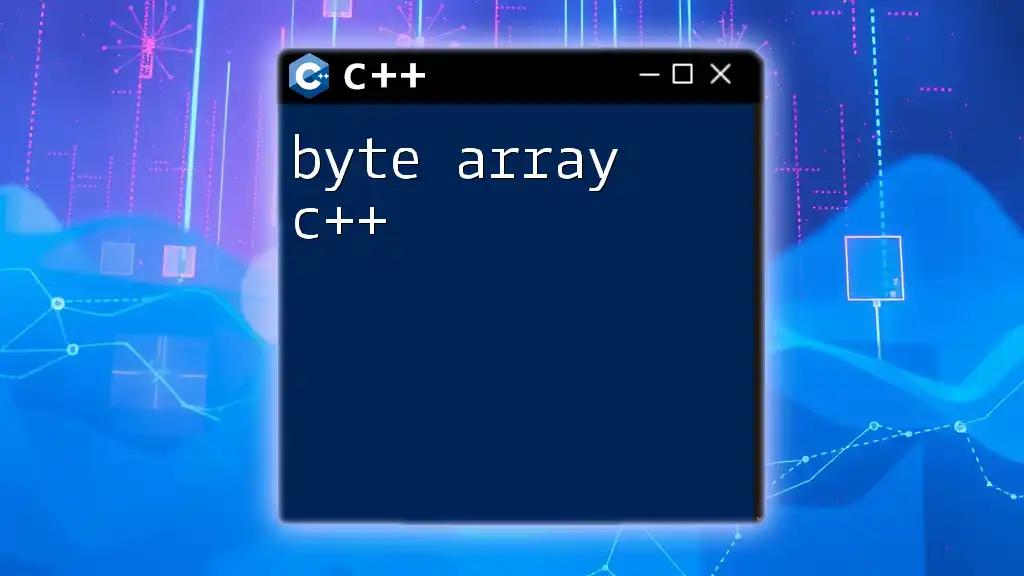
Common Use Cases for 2D Arrays in C++
Representing Matrices
Matrix representation is a fundamental aspect of many applications in mathematics and engineering. For instance, to perform matrix operations like addition, you can define two matrices and iterate through them to compute the result.
Example of matrix addition:
int result[3][3]; // Declaring a result matrix for 3x3 addition
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
result[i][j] = matrixA[i][j] + matrixB[i][j]; // Adding corresponding elements
}
}
Creating Grids for Games
In game development, 2D arrays in C++ can effectively represent grids for maps or game boards, enabling you to manage game states and interactions. For example, a chessboard can be represented using an 8x8 grid:
char chessboard[8][8]; // Each element can hold 'B' for Black, 'W' for White or 'E' for empty
Storing and Manipulating Images
2D arrays can represent pixel data in images, where each array element corresponds to a pixel's color value. Manipulating individual pixels can achieve effects like filters or transformations:
// Example of setting a pixel color
image[x][y] = 255; // Set the pixel at coordinates (x,y) to white (depending on the color model)
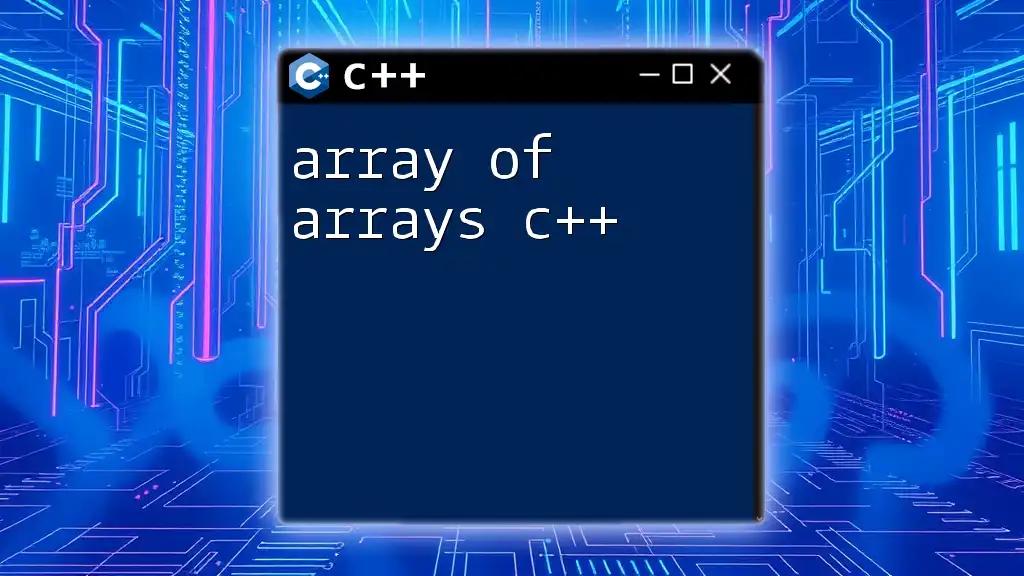
Best Practices for Using C++ 2D Arrays
Memory Management Tips
When dynamically allocating arrays, always ensure proper deallocation of memory to avoid memory leaks. Always check for successful memory allocation and handle errors gracefully. Use `nullptr` checks to ensure you're not trying to free memory that wasn’t allocated.
Performance Considerations
With the performance of 2D arrays in C++, access patterns are crucial. For instance, accessing elements in a row-major order (sequentially across rows) is more efficient due to cache locality compared to column-major order access. Be conscious of your loop structure to ensure optimal performance.
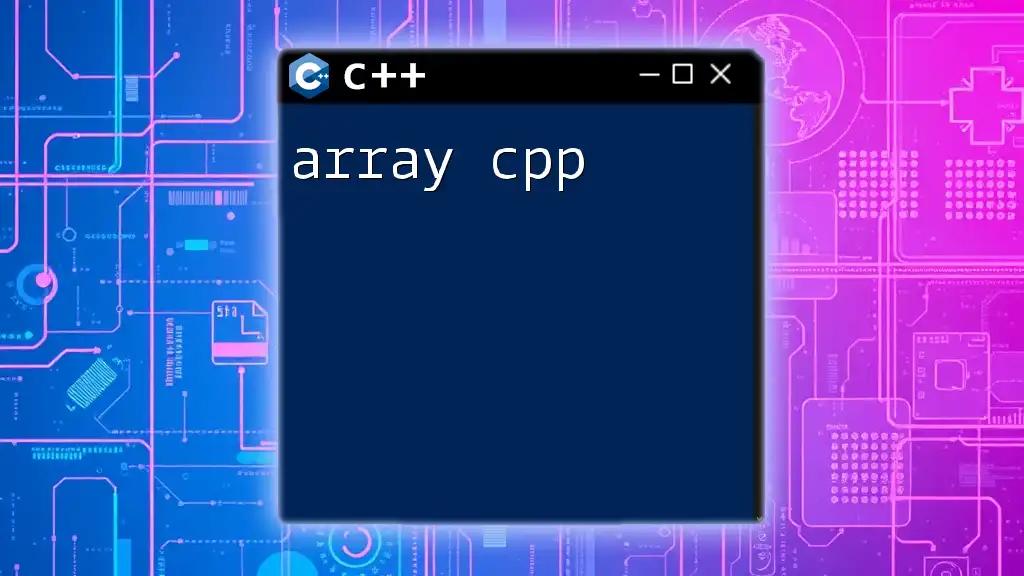
Conclusion
Understanding 2D arrays in C++ significantly enhances your ability to manage and manipulate data effectively. From dynamic allocation to traversals, mastering this topic opens doors to numerous applications in technology and programming. To further your learning, practice creating and utilizing 2D arrays in different programming scenarios.