In C++, pointers can be used to access elements of an array, allowing for efficient manipulation of array data through their memory addresses.
Here's a code snippet demonstrating how to use pointers with arrays in C++:
#include <iostream>
using namespace std;
int main() {
int arr[] = {10, 20, 30, 40, 50};
int* ptr = arr; // Pointer to the first element of the array
for (int i = 0; i < 5; i++) {
cout << *(ptr + i) << " "; // Accessing array elements using pointer arithmetic
}
return 0;
}
Understanding Pointers and Arrays in C++
What is a Pointer?
A pointer in C++ is a variable that holds the memory address of another variable. Pointers provide powerful capabilities for dynamic memory management and allow for efficient data manipulation. To declare a pointer, you use the `*` symbol, which signifies that the variable is a pointer type.
For example, declaring an integer pointer can be done as follows:
int *p;
Importance of Pointers
Pointers are crucial in C++ programming for several reasons:
- Memory Management: Pointers give direct control over memory allocation and deallocation. This is essential for creating efficient and performant applications, especially when working with large amounts of data.
- Function Efficiency: When passing large data structures like arrays to functions, pointers can be used to avoid copying the entire structure, which is more efficient.
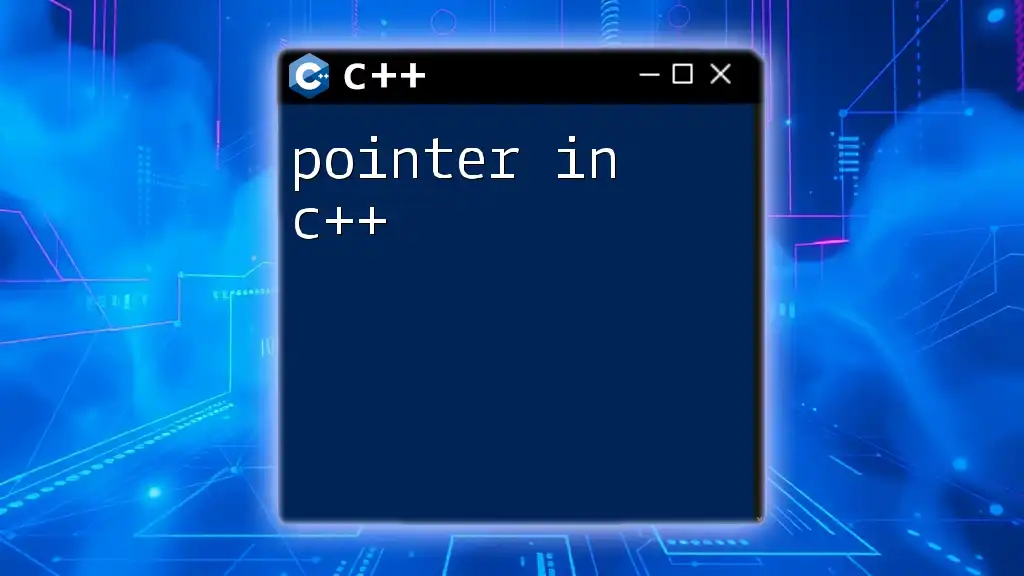
Introduction to Arrays in C++
What is an Array?
An array in C++ is a collection of elements of the same type stored in contiguous memory locations. Arrays allow for easy data management and access. The basic syntax for declaring an array is as follows:
int arr[5]; // Declaration of an integer array of size 5
Arrays can be single-dimensional or multi-dimensional, with single-dimensional arrays being the most common.
Memory Layout of Arrays
In C++, when you declare an array, memory is allocated sequentially. For instance, if you declare an integer array of size 5:
int arr[5];
The memory layout will look something like this:
Memory Address | Value
------------------------
0x001 | arr[0]
0x002 | arr[1]
0x003 | arr[2]
0x004 | arr[3]
0x005 | arr[4]
This layout is critical to understanding how to manipulate arrays using pointers.
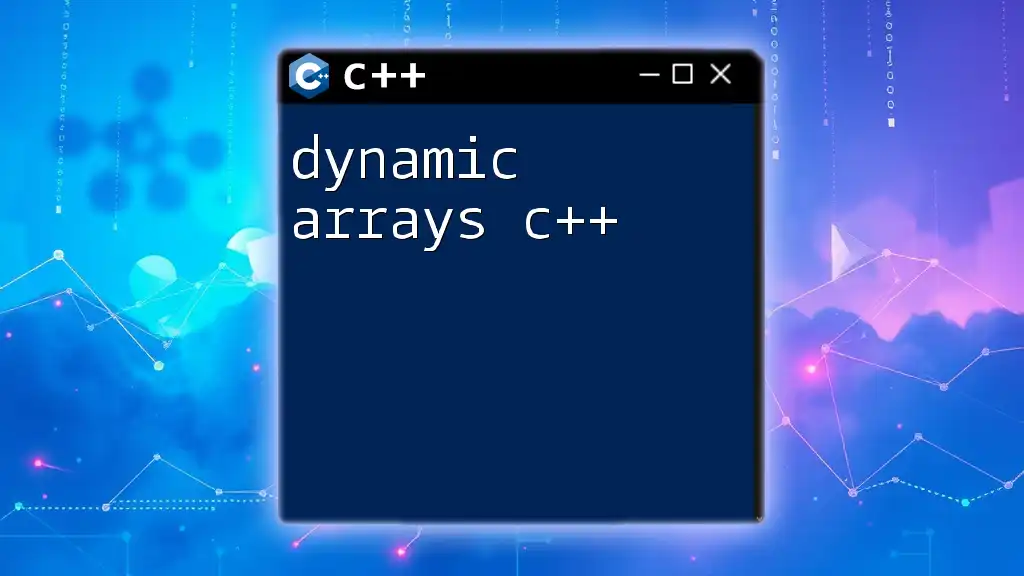
The Relationship Between Pointers and Arrays in C++
How Arrays and Pointers are Linked
Arrays and pointers are closely related; an array name acts as a pointer to its first element. When you pass an array to a function, you are actually passing a pointer to its first element.
For instance:
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
}
When calling `printArray(arr, 5);`, `arr` represents the address of the first element `arr[0]`.
Pointer to an Array in C++
To declare a pointer to an array, you specify the type of the elements, followed by the `*` symbol and the name of the pointer. The syntax looks like this:
int (*p)[5];
This declaration means that `p` is a pointer to an array of 5 integers. You can access elements in the array through the pointer as follows:
int arr[5] = {1, 2, 3, 4, 5};
int (*p)[5] = &arr; // Pointer to the array
cout << (*p)[2]; // Outputs 3
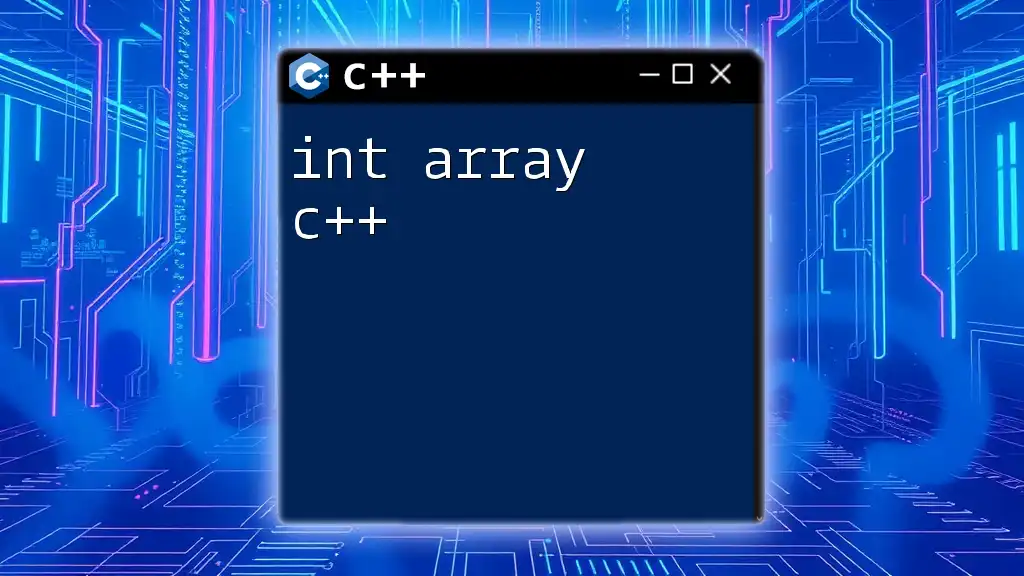
Working with Pointers and Arrays
Accessing Array Elements Using Pointers
One of the most powerful features of pointers is accessing array elements directly. You can perform pointer arithmetic to traverse the array. For example:
int arr[5] = {10, 20, 30, 40, 50};
int *p = arr; // Pointer to the first element of the array
for (int i = 0; i < 5; i++) {
cout << *(p + i) << " "; // Outputs each element using pointer
}
This demonstrates that you can access each element by simply incrementing the pointer, which is more efficient than using index-based access.
Pointer Array C++
A pointer array is an array that contains pointers to other variables or data. Here’s how you can declare a pointer array:
int* arr[5]; // Array of 5 integer pointers
Here’s a simple example of using a pointer array:
int a = 10, b = 20, c = 30;
int* arr[3] = {&a, &b, &c};
for (int i = 0; i < 3; i++) {
cout << *arr[i] << " "; // Outputs 10 20 30
}
In this case, `arr` is an array of pointers, where each pointer points to an integer variable.
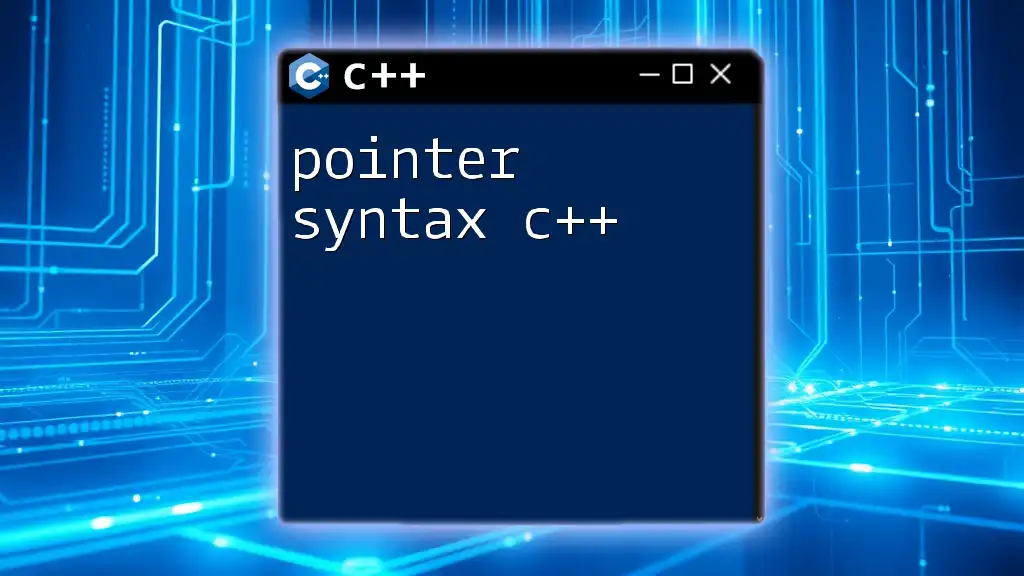
Practical Applications of Pointers and Arrays
Dynamic Arrays Using Pointers
Dynamic arrays allow for flexible memory management. You can create an array whose size is determined at runtime using pointers. Here’s an example:
int n;
cout << "Enter the size of the array: ";
cin >> n;
int *arr = new int[n]; // Dynamically allocate an array of size n
// Use the array
for (int i = 0; i < n; i++) {
arr[i] = i * 10;
}
// Deallocate memory
delete[] arr;
In this example, we allocate memory for an array using `new` and deallocate it using `delete[]`.
Multi-dimensional Arrays and Pointers
When dealing with multi-dimensional arrays, pointers provide a powerful mechanism to navigate through the data. A two-dimensional array can be represented as pointers to pointers. For instance:
int rows = 3, cols = 4;
int **arr = new int*[rows]; // Allocate memory for rows
for (int i = 0; i < rows; i++) {
arr[i] = new int[cols]; // Allocate memory for columns
}
// Accessing elements
arr[1][2] = 5;
for (int i = 0; i < rows; i++) {
delete[] arr[i]; // Deallocate memory for columns
}
delete[] arr; // Deallocate memory for rows
In this code snippet, we allocate memory dynamically for a 2D array.
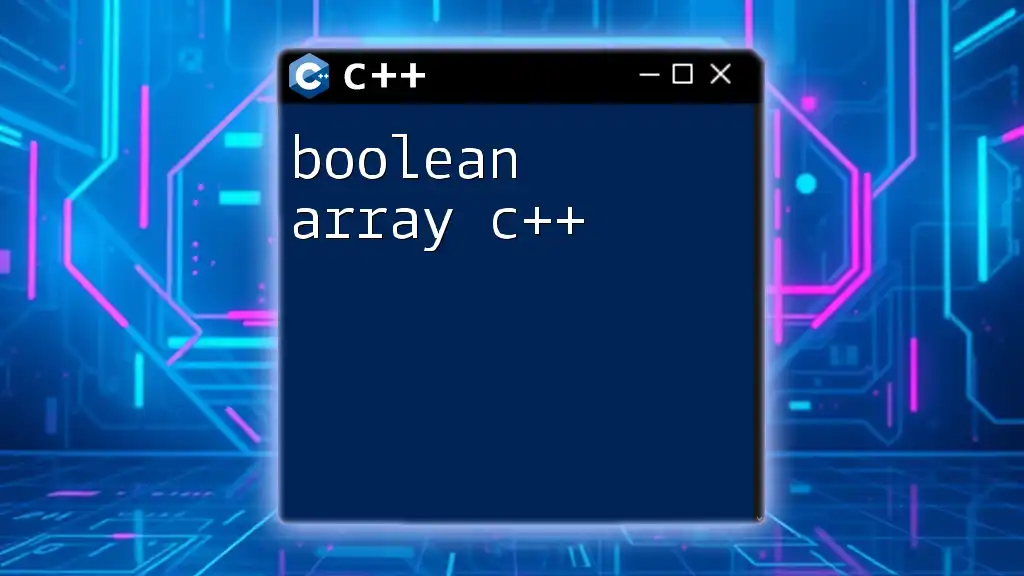
Common Pitfalls When Using Pointers with Arrays
Memory Leaks and Allocation Errors
One of the most common mistakes in C++ programming is not releasing allocated memory. Always ensure that you use `delete` to free up any memory allocated with `new`. A memory leak can cause unexpected behavior due to resource exhaustion.
Out-of-Bounds Access
Accessing memory outside the bounds of an array can lead to undefined behavior:
int arr[3] = {1, 2, 3};
cout << arr[5]; // Undefined behavior
Always check boundaries when working with pointers and arrays to prevent such issues.
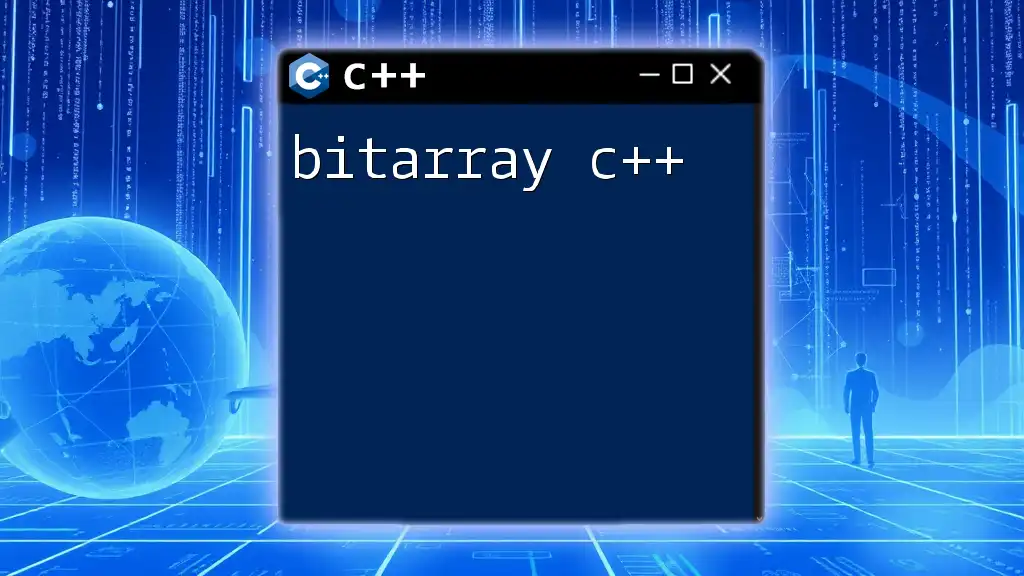
Conclusion
Recap of Key Points
The relationship between pointers and arrays in C++ is fundamental for creating efficient applications. Understanding how to manipulate arrays using pointers will enhance your programming skills.
Further Learning Resources
For those wishing to delve deeper, consider exploring comprehensive C++ programming books, online coding platforms, and tutorials focused on pointers and memory management.
Call to Action
Practice using pointers in arrays with sample projects and coding exercises. Experiment with different implementations to solidify your understanding and expertise in pointers in arrays C++.