In C++, a pointer is a variable that stores the memory address of another variable, allowing for direct memory access and manipulation.
int main() {
int value = 5; // Declare an integer variable
int* ptr = &value; // Declare a pointer and assign it the address of value
*ptr = 10; // Modify the value at the address stored in ptr
return 0;
}
What are Pointers?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are a powerful feature of C++ that allow for efficient memory management and manipulation of data structures. Understanding pointers is crucial for any C++ programmer, as they are involved in dynamic memory allocation, function calls, and more.
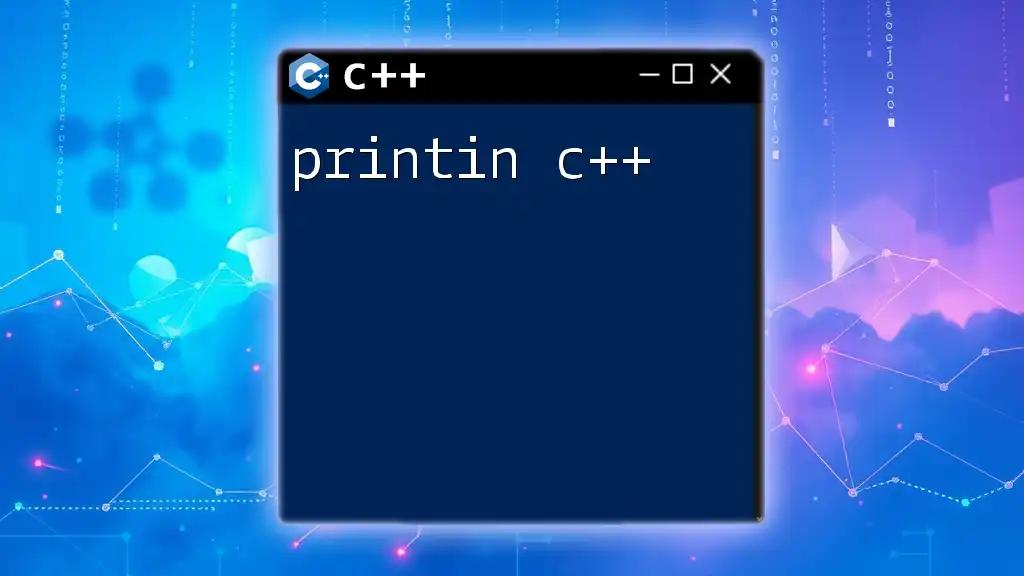
Memory in C++
To fully understand pointers, it's important to grasp the fundamental concepts of memory in C++. Memory is divided into two primary areas:
-
Stack Memory: This is where local variables are stored. Memory allocation is temporary and automatically managed, meaning it is allocated when the function is called and deallocated when it exits.
-
Heap Memory: This is used for dynamic memory allocation. Unlike stack memory, variables in heap memory remain until they are explicitly deallocated by the programmer.
Why Use Pointers?
Pointers offer several advantages in C++:
-
Dynamic Memory Allocation: Pointers enable the allocation of memory during runtime using `new`, which is essential for creating data structures such as linked lists and dynamic arrays.
-
Efficient Manipulation of Arrays and Strings: Pointers can be used to traverse arrays and manipulate strings efficiently without the need to copy data.
-
Passing Variables by Reference: Functions in C++ can pass variables by reference using pointers, allowing modifications to the original variable without returning a value.

Declaring and Initializing Pointers
To work with pointers, you first need to declare them. The syntax for declaring a pointer involves using an asterisk (*). For example:
int* ptr; // Declaring a pointer to an integer
After declaring a pointer, you need to initialize it with the address of a variable. The address-of operator (`&`) is used to get the memory address:
int x = 10;
ptr = &x; // Now ptr points to the address of x

Dereferencing Pointers
Derekferencing a pointer means accessing the value stored at the address the pointer points to. You can dereference a pointer using the asterisk (*) operator, as shown below:
std::cout << *ptr; // This outputs the value of x, which is 10
Understanding dereferencing is crucial because it allows you to interact with the data indirectly.

Types of Pointers in C++
Null Pointers
A null pointer is a pointer that does not point to any valid memory location. This can help manage memory safely. You can declare a null pointer like this:
int* ptr = nullptr; // Pointer initialized to null
Void Pointers
A void pointer is a special type of pointer that can point to any data type, but it cannot be dereferenced directly. Here's how you can use a void pointer:
void* vPtr;
int x = 5;
vPtr = &x; // vPtr now holds the address of x
To use a void pointer, you typically need to cast it to the appropriate type.
Function Pointers
Function pointers allow you to store the address of a function. This is particularly useful for implementing callback functions. Here’s an example of how to declare and use a function pointer:
void myFunction() {
std::cout << "Hello from myFunction!" << std::endl;
}
void (*funcPtr)() = &myFunction; // Declaring a function pointer
(*funcPtr)(); // Calling the function through the pointer
Pointers to Pointers
A pointer to a pointer (double pointer) is a pointer that points to another pointer. Here’s an example:
int** ptrToPtr = &ptr; // ptrToPtr holds the address of ptr
Understanding pointers to pointers can be crucial, especially in complex data structures like matrices.
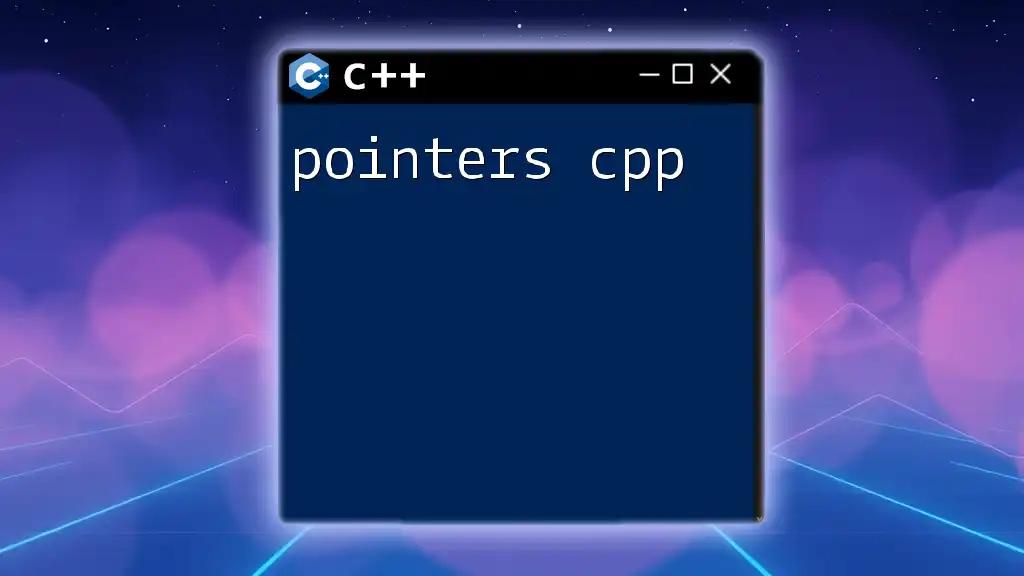
Dynamic Memory Allocation
Dynamic Memory Allocation
C++ supports dynamic memory allocation through the `new` and `delete` operators. Using `new`, you can dynamically allocate memory for variables during program runtime:
int* arr = new int[5]; // Dynamically allocated array of integers
Once you are done using the memory allocated on the heap, it’s essential to free it using the `delete` operator to avoid memory leaks:
delete[] arr; // Deallocate memory
Common Pitfalls with Dynamic Memory
When working with dynamic memory, several pitfalls can arise:
-
Memory Leaks: If you forget to deallocate memory, your program will consume more memory over time, leading to performance issues.
-
Double Deletion: Attempting to delete memory that has already been deallocated can cause undefined behavior.
-
Dangling Pointers: Pointers that reference deallocated memory are referred to as dangling pointers, which can lead to crashes if accessed.

Advanced Pointer Concepts
Smart Pointers
Smart pointers are part of the C++ Standard Library and help manage dynamic memory automatically, reducing the risk of memory leaks. They include types such as `std::unique_ptr`, `std::shared_ptr`, and `std::weak_ptr`. Here’s an example of using `std::unique_ptr`:
#include <memory>
std::unique_ptr<int> smartPtr(new int(10)); // Automatically deallocated
Using smart pointers is generally recommended over raw pointers because they offer better safety and easier memory management.
Pointer Arithmetic
Pointer arithmetic allows you to perform operations on pointers. For instance, incrementing a pointer advances it to the next memory address of its type:
int array[5] = {1, 2, 3, 4, 5};
int* ptr = array;
ptr++; // Now ptr points to the second element (2)
Understanding pointer arithmetic is crucial for iterating over arrays and manipulating data at a low level.
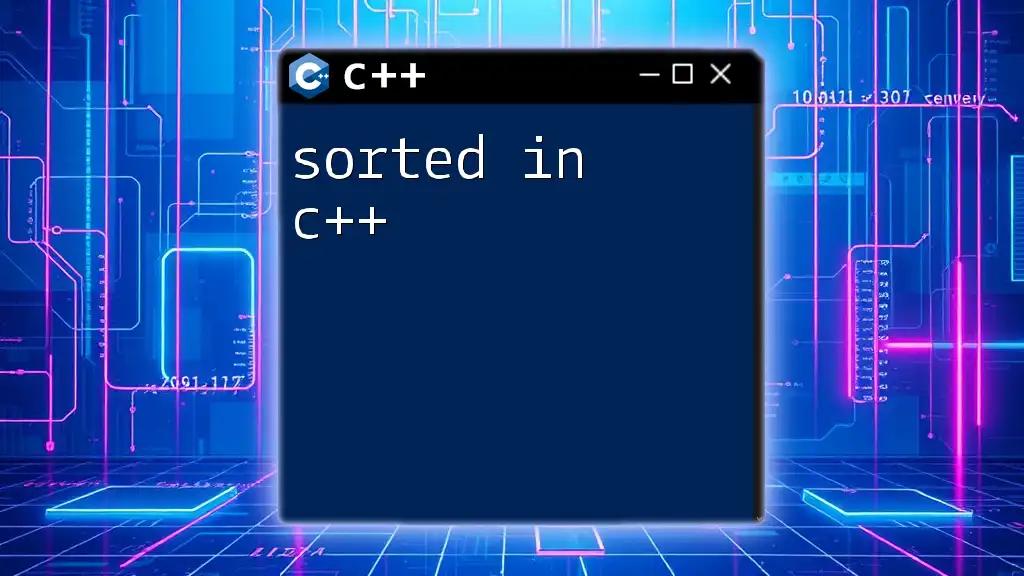
Best Practices When Using Pointers
Avoiding Common Mistakes
Some best practices can help mitigate common issues associated with pointers:
-
Always initialize your pointers before use. Consider setting them to `nullptr` to avoid undefined behavior.
-
Always check for null before dereferencing a pointer. This can prevent crashes and undefined behavior.
When to Use Pointers
Use pointers when you need dynamic memory allocation or when passing large structures to functions where you want to avoid making copies. They are also essential in implementing complex data structures and algorithms.
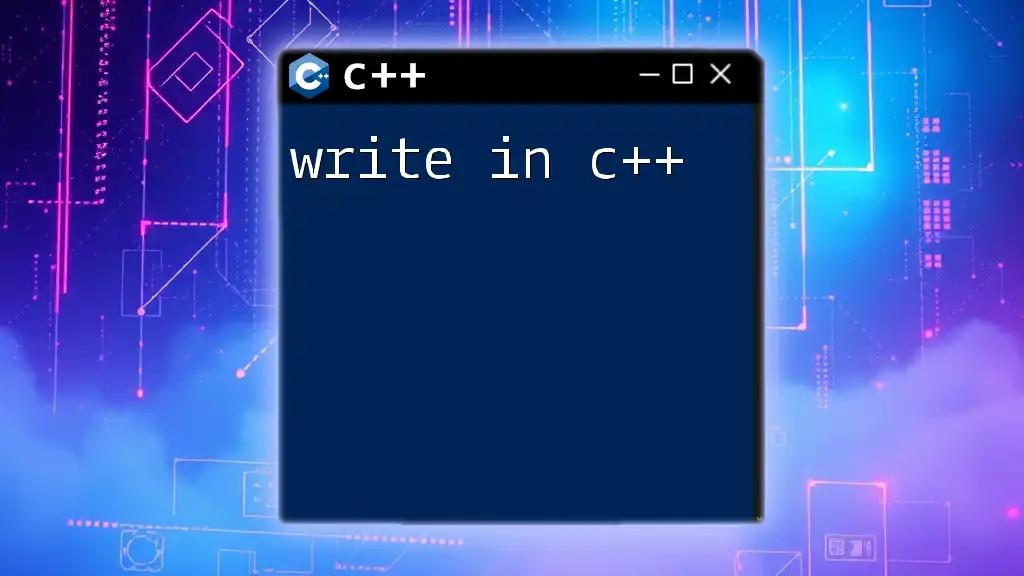
Recap of Pointers in C++
In this comprehensive guide on pointers in C++, we explored the definition of pointers, their types, memory management, dynamic memory allocation, smart pointers, and best practices. Pointers are a fundamental concept in C++ programming that enables efficient data management. By embracing the power of pointers, you'll be able to write more effective and memory-efficient applications.

Additional Resources
To delve deeper into the world of pointers and memory management, consider exploring the following resources:
- Official C++ documentation
- Recommended books such as "The C++ Programming Language" by Bjarne Stroustrup
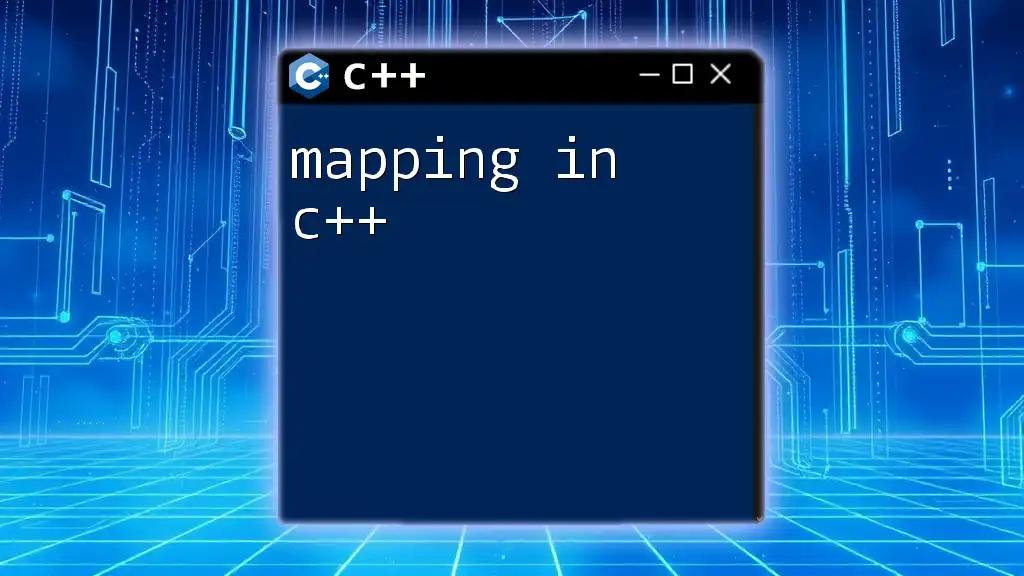
Common Questions Answered
What is the difference between a pointer and a reference?
While both pointers and references are used to refer to other variables, a pointer can be reassigned to point to different variables, while a reference cannot be changed once initialized.
How do I avoid memory leaks when using pointers?
The primary way to avoid memory leaks is to ensure that you always deallocate memory that you allocate dynamically. Using smart pointers is also highly recommended as they manage memory automatically.