In C++, passing by pointer allows a function to modify the original variable by using its memory address instead of passing a copy of the variable itself.
#include <iostream>
void increment(int* ptr) {
(*ptr)++;
}
int main() {
int value = 5;
increment(&value);
std::cout << value; // Output: 6
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer is a variable that stores the memory address of another variable. Pointers allow programs to interact with memory directly, which opens up many possibilities. Unlike regular variables that hold values (like integers or characters), pointers hold the address of where a value is stored in memory.
For example, if you declare a pointer variable like this:
int *ptr;
Here, `ptr` is a pointer that can point to an integer value.
Why Use Pointers?
Using pointers provides several advantages:
- Dynamic Memory Allocation: Pointers are essential for dynamically allocating memory during runtime, allowing you to create and manage data structures like linked lists, trees, and other complex data types.
- Efficient Data Manipulation: Instead of passing large structures by value, you can pass pointers, which can significantly enhance performance by avoiding the overhead of copying data.
- Scope Modification: Pointers allow functions to modify variables that are outside their local scope, facilitating alterations of variable values that persist after the function returns.
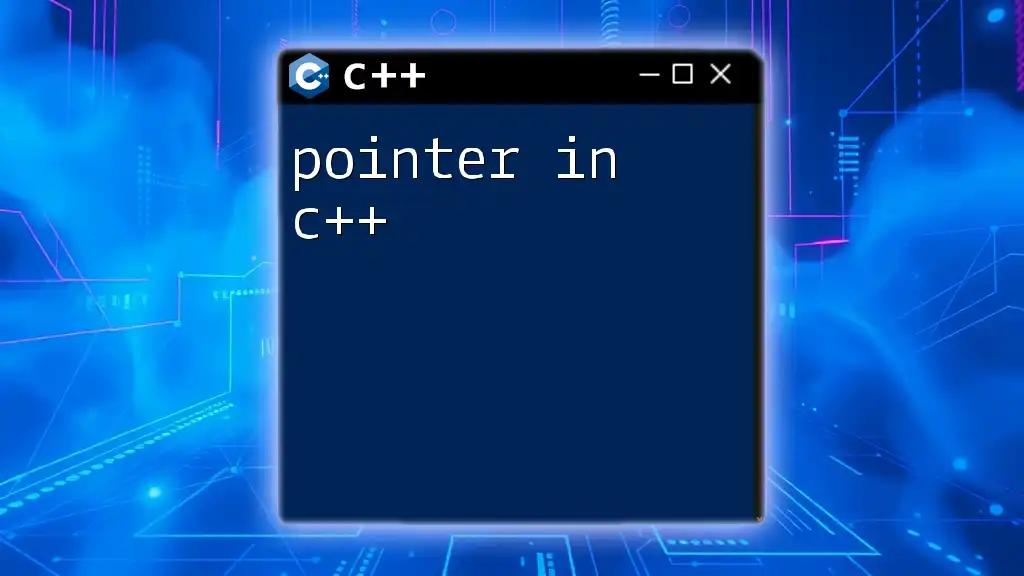
Pass by Pointer Explained
What Does "Pass by Pointer" Mean?
Pass by pointer refers to the practice of using pointers as function arguments. When you pass an argument by pointer, you're passing the memory address of the variable rather than a copy of its value. This means any changes made to the parameter inside the function will affect the original variable.
For example, consider the following function signatures:
void modifyValue(int value) { value = 10; } // Pass by value
void modifyPointer(int *ptr) { *ptr = 10; } // Pass by pointer
In the `modifyValue` function, the original value remains unchanged after the function call because only a copy is modified. In contrast, the `modifyPointer` function allows the original variable to be modified directly.
Syntax for Passing by Pointer
To pass a pointer to a function, the function parameter must be defined as a pointer type. Here's a simple example:
void changeValue(int *ptr) { *ptr = 20; }
In this snippet, `changeValue` updates the value at the address pointed to by `ptr`.

Practical Examples
Basic Example: Modifying a Variable's Value
Let’s look at a straightforward example of how a function can modify a variable through a pointer:
#include <iostream>
void updateValue(int *p) {
*p = 30;
}
int main() {
int num = 10;
updateValue(&num);
std::cout << "Updated Value: " << num << std::endl;
return 0;
}
In this example, we declare an integer variable `num` and pass its address to the `updateValue` function using the `&` operator. Inside the function, we dereference the pointer `p` to change the value of `num` to `30`. When we print `num` in `main`, it reflects the modification because it holds the memory address of the original variable.
Advanced Example: Swapping Values Using Pointers
Pointers can also be used to perform operations like swapping two integers. Here’s how you can do that:
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 5, y = 10;
swap(&x, &y);
std::cout << "Swapped values: x = " << x << ", y = " << y << std::endl;
return 0;
}
In this code, the `swap` function takes two pointers as parameters. By dereferencing these pointers, we can swap their values. After executing `swap`, `x` and `y` reflect the swapped values, demonstrating how pass by pointer efficiently manipulates data outside the function's scope.
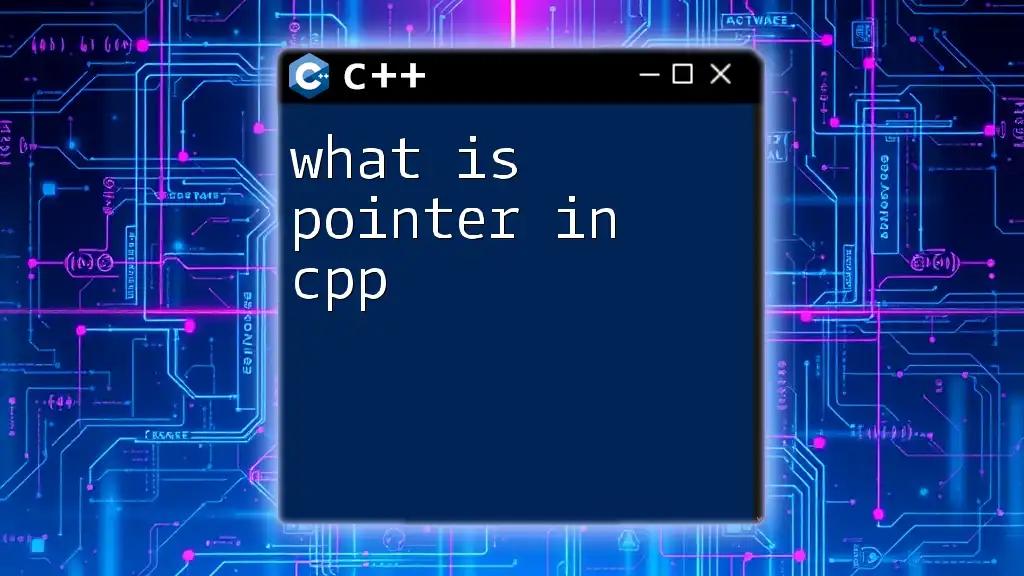
Advantages of Using Pass by Pointer in Functions
Memory Efficiency
Passing arguments by pointer saves memory because you avoid copying large data structures. For example, passing a pointer to a large array will only require passing the address rather than duplicating the entire array. This is particularly advantageous when dealing with data structures like graphs or trees.
Flexibility in Function Design
Using pointers empowers functions to modify multiple values. Instead of returning a single result, you can pass multiple pointers as function parameters. For instance:
void getMinMax(int *arr, int size, int *min, int *max) {
*min = *max = arr[0];
for (int i = 1; i < size; ++i) {
if (arr[i] < *min) *min = arr[i];
if (arr[i] > *max) *max = arr[i];
}
}
In this example, the function calculates the minimum and maximum values in an array, returning both through pointer parameters instead of using a structure or reference.
Modifying Global Variables
Functions that use pointers can directly change global variables, enabling you to create versatile functions that affect the overall program state. Here's a simple illustration:
int globalVar = 0;
void updateGlobal(int *ptr) {
*ptr = 100;
}
With this design, calling `updateGlobal(&globalVar);` alters `globalVar` directly.

Common Pitfalls with Pass by Pointer
Dereferencing Issues
One common mistake in C++ is improperly dereferencing a null or uninitialized pointer. This can lead to runtime errors or crashes. For instance:
int *nullPointer = nullptr;
// std::cout << *nullPointer; // Will lead to a runtime error
Prior to dereferencing pointers, always ensure they point to a valid memory location.
Memory Leaks
Improper use of pointers, particularly with dynamic memory management, can result in memory leaks. For instance, if memory allocated with `new` is not freed properly using `delete`, that memory remains inaccessible. Always ensure you manage memory efficiently to prevent leaks.

Conclusion
In summary, pass by pointer in C++ is an essential technique that enhances memory efficiency, flexibility, and the capacity for functions to interact with variables at a deeper level. By understanding pointers and their application in function arguments, you can write more efficient and robust C++ code. Practice using pointers and explore their versatile capabilities to solidify your understanding and skills in C++. Remember, the journey of mastering C++ is ongoing, and each concept you learn is a stepping stone towards becoming a proficient programmer.

Additional Resources
For those eager to delve deeper into pointers and memory management, consider exploring books on C++, online tutorials, or coding platforms that offer interactive exercises to practice pointer concepts and enhance your programming expertise.