In C++, pointers can be categorized into several types including: regular pointers, null pointers, void pointers, smart pointers, and pointer to members, each serving different purposes for memory management and data manipulation.
Here’s a code snippet showcasing various types of pointers:
#include <iostream>
#include <memory>
int main() {
// Regular pointer
int a = 10;
int* regularPtr = &a;
// Null pointer
int* nullPtr = nullptr;
// Void pointer
void* voidPtr = &a;
// Smart pointer (unique_ptr)
std::unique_ptr<int> smartPtr = std::make_unique<int>(20);
std::cout << "Regular Pointer: " << *regularPtr << std::endl;
std::cout << "Null Pointer: " << (nullPtr ? "Not Null" : "Is Null") << std::endl;
std::cout << "Void Pointer (casted): " << *(static_cast<int*>(voidPtr)) << std::endl;
std::cout << "Smart Pointer: " << *smartPtr << std::endl;
return 0;
}
Understanding C++ Pointer Types
In C++, pointers are variables that store memory addresses. They are crucial for dynamic memory management, efficient array handling, and representing other complex data structures. Grasping the types of pointers in C++ allows developers to leverage their functionality effectively, improving both performance and code reliability.
Basic Pointer Type in C++
What is a Regular Pointer?
A regular pointer is the most fundamental type of pointer in C++. It points to a variable of a specific data type, allowing direct manipulation of that variable's value stored in memory.
To declare a regular pointer, you use the data type followed by an asterisk (*). Here’s an example:
int value = 10;
int* pointerToValue = &value; // Here, pointerToValue holds the address of value
This line of code initializes `value` and then creates a pointer called `pointerToValue` that holds the address of `value`. You can access the value stored at that address by using the dereferencing operator (*):
int dereferencedValue = *pointerToValue; // dereferencedValue now equals 10
Characteristics of Regular Pointers
Regular pointers have unique characteristics determined by the data type they point to. When you declare a pointer, the memory address of the variable it points to is stored. The `&` operator is used to obtain the address of the variable, while the `*` operator allows you to dereference it, giving you access to the actual value stored at that address.
Consider this snippet:
int a = 20;
int* ptr = &a; // ptr holds the address of 'a'
*ptr = 30; // value of 'a' now becomes 30
In this example, by dereferencing `ptr`, we modify the actual value of `a`.
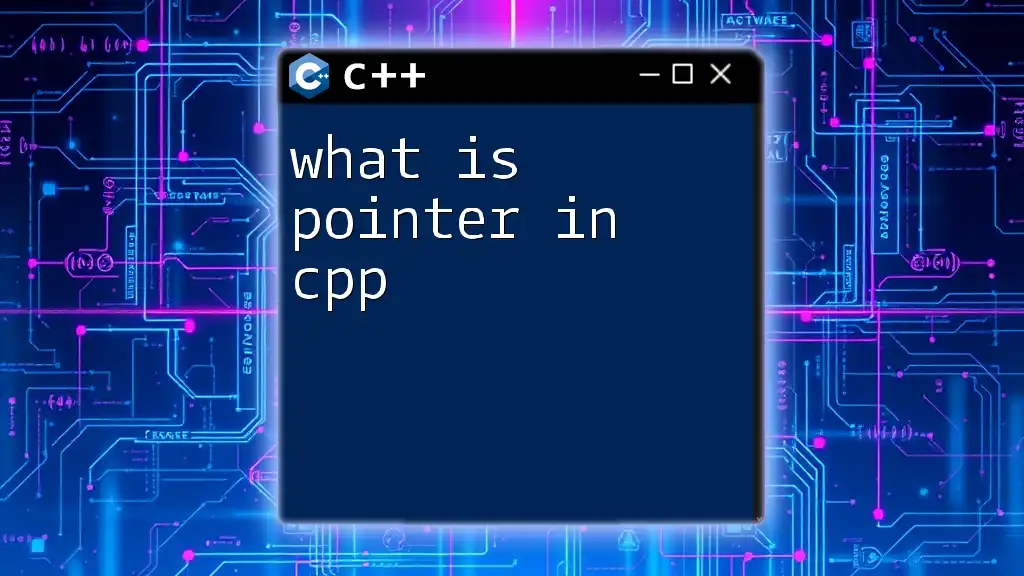
Specialized Pointer Types in C++
Void Pointers
A void pointer is a special type of pointer that doesn't have a specific data type associated with it. This makes void pointers versatile but requires careful usage since you can't directly dereference them.
Here's how to declare a void pointer:
void* voidPtr;
int data = 5;
voidPtr = &data; // voidPtr now holds the address of an integer
To use a void pointer, you typically need to cast it to the appropriate type before dereferencing:
int value = *(static_cast<int*>(voidPtr)); // We cast to int* before dereferencing
Null Pointers
A null pointer is a pointer that doesn't point to any valid memory location. It's essential to check for null pointers to avoid dereferencing an invalid address, leading to undefined behavior.
Declaration is simple:
int* nullPointer = nullptr; // Using nullptr is the preferred method in C++
You can check if a pointer is null like this:
if (nullPointer == nullptr) {
// Safe to use
}
Smart Pointers
Introduction to Smart Pointers
Smart pointers are template classes that manage the lifetime of dynamically allocated objects, preventing memory leaks and dangling pointers. They are preferable to raw pointers for resource management in modern C++.
Unique Pointer
A `std::unique_ptr` is a smart pointer that owns and manages another object through a pointer. A unique pointer cannot be copied, which ensures that only one unique pointer can own a particular resource.
To declare a unique pointer, use:
#include <memory>
std::unique_ptr<int> uniquePtr(new int(10)); // uniquePtr manages the memory of an int initialized to 10
When `uniquePtr` goes out of scope, the memory it manages is automatically released.
Shared Pointer
A `std::shared_ptr` allows multiple pointers to share ownership of a single resource. The memory is automatically freed when the last `shared_ptr` owning that resource is destroyed.
Whats refers to the declaration of a shared pointer:
#include <memory>
std::shared_ptr<int> sharedPtr = std::make_shared<int>(20);
This creates a shared pointer pointing to a dynamically allocated integer initialized to 20. The resource will be cleaned up automatically when there are no more references to it.
Weak Pointer
A `std::weak_ptr` is used in conjunction with `std::shared_ptr`. It holds a non-owning reference that allows you to check if the shared resource still exists without affecting reference counts.
Here's how to declare a weak pointer:
std::weak_ptr<int> weakPtr = sharedPtr; // weakPtr observes sharedPtr without owning it
You can convert a weak pointer to a shared pointer if needed:
if (auto shared = weakPtr.lock()) {
// Use shared safely, as it's still valid
}
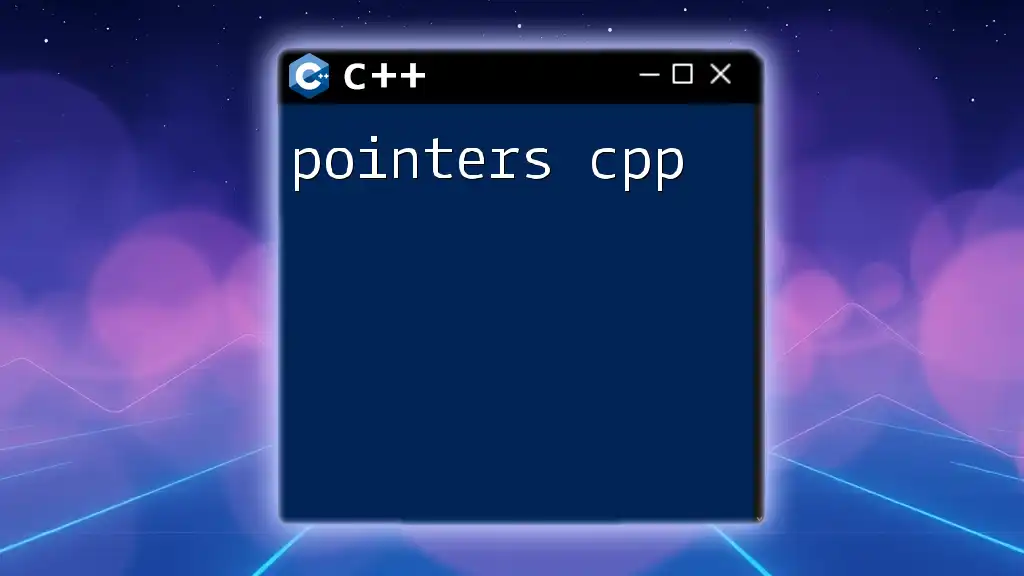
Other Pointer Types in C++
Function Pointers
Function pointers allow you to point to a function's address, enabling dynamic function calls and providing a way to pass functions as arguments. The syntax for declaring a function pointer looks like this:
void (*functionPtr)(int); // Pointer to a function taking an int and returning void
Here's an example illustrating how to use function pointers:
void printValue(int value) {
std::cout << "The value is: " << value << std::endl;
}
int main() {
void (*functionPtr)(int) = printValue; // point to printValue function
functionPtr(10); // Calls printValue(10)
}
Pointer to an Array
A pointer can also point to the first element of an array, allowing for efficient array manipulation. Pointer arithmetic can be used to navigate through the elements of the array.
Here's the syntax to declare a pointer to an array:
int arr[] = {1, 2, 3, 4, 5};
int* ptrToArr = arr; // ptrToArr now points to the first element of arr
This allows you to access elements of the array using pointer syntax:
int firstElement = *ptrToArr; // 1
int secondElement = *(ptrToArr + 1); // 2
Pointers to Pointers
A pointer to a pointer (also known as a double pointer) is a pointer that points to another pointer. This can be particularly useful in functions that need to modify the address a pointer stores.
Here's how you declare a pointer to a pointer:
int value = 100;
int* ptr = &value; // pointer to int
int** ptrToPtr = &ptr; // pointer to pointer to int
You can access the original value using:
int originalValue = **ptrToPtr; // originalValue equals 100
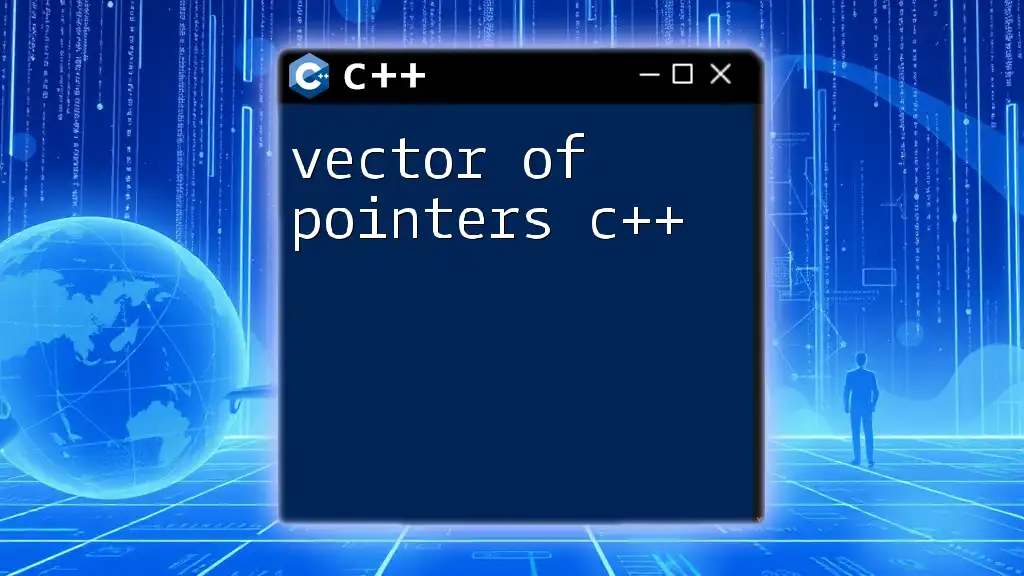
Conclusion: Navigating Through Pointer Types in C++
Understanding the types of pointers in C++ is paramount for effective programming. Each pointer type serves unique purposes, from simple memory address manipulation to sophisticated memory management via smart pointers.
As you work with various pointer types, practice is essential. Familiarity with pointers can greatly enhance your competency in C++, paving the way for more advanced programming techniques.
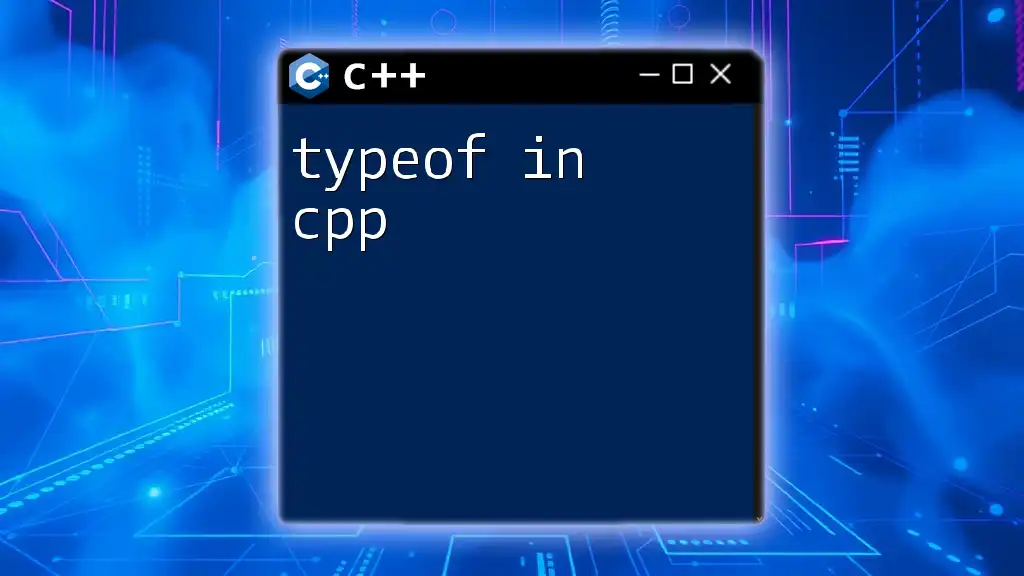
Additional Resources for Learning about Pointer Types in C++
- Books: Look for titles such as "Effective C++" by Scott Meyers or "C++ Primer" for in-depth explorations of pointers.
- Online Courses: Platforms like Coursera and Udemy offer targeted courses on C++ and memory management.
- Documentation: Official C++ documentation is an excellent resource for understanding pointer types and best practices.
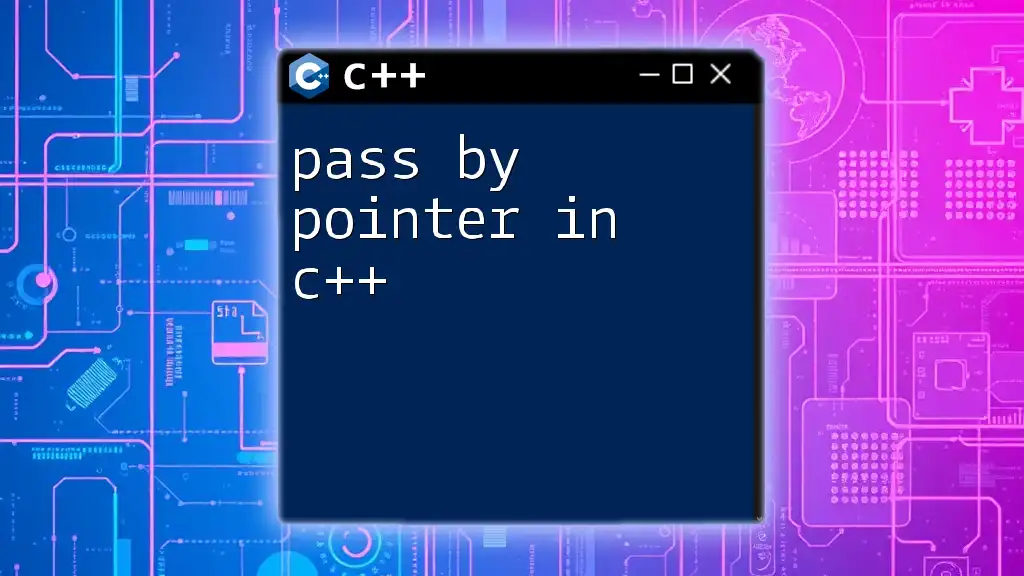
Call to Action
Stay tuned for more insightful articles on C++ programming. Join our mailing list for exclusive learning materials and resources that will elevate your coding prowess!