The `typeof` operator in C++ is used to determine the type of an expression at compile time, allowing for type-safe programming.
#include <iostream>
#include <typeinfo>
int main() {
int n = 42;
std::cout << "The type of n is: " << typeid(n).name() << std::endl;
return 0;
}
Understanding `typeof` in C++
`typeof` is a keyword in C++ that provides developers with a way to identify the type of a variable or expression at compile time. While it is not part of the standard C++, many compilers support it due to its utility in modern programming contexts. It simplifies code and assists with type inference in a way that promotes better readability.
Although `typeof` is prevalent in various programming languages, it is essential to understand its role and functionality in C++. Here, we will delve into its syntax, practical applications, limitations, and explore alternatives.
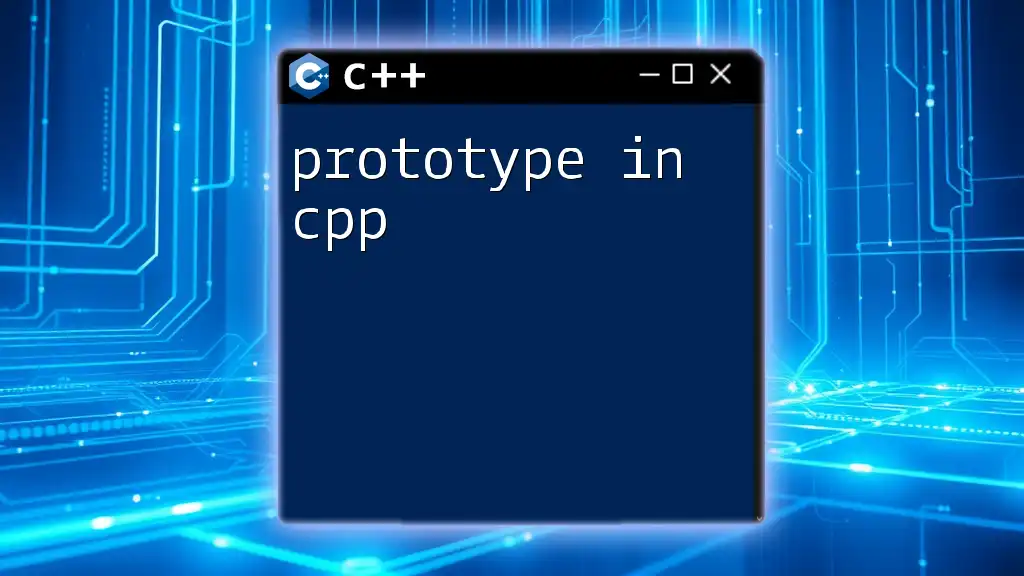
Syntax of `typeof` in C++
The basic syntax of `typeof` is straightforward. It can be used to create a new variable whose type is determined by the expression you pass to it. An example of basic usage is:
typeof(expression)
For instance, if you have a variable `x` defined as an integer, you can use `typeof` to declare another variable of the same type:
int x = 5;
typeof(x) y; // y is inferred to be of type int
In this case, `typeof(x)` evaluates to `int`, allowing `y` to be declared without explicitly specifying the type.
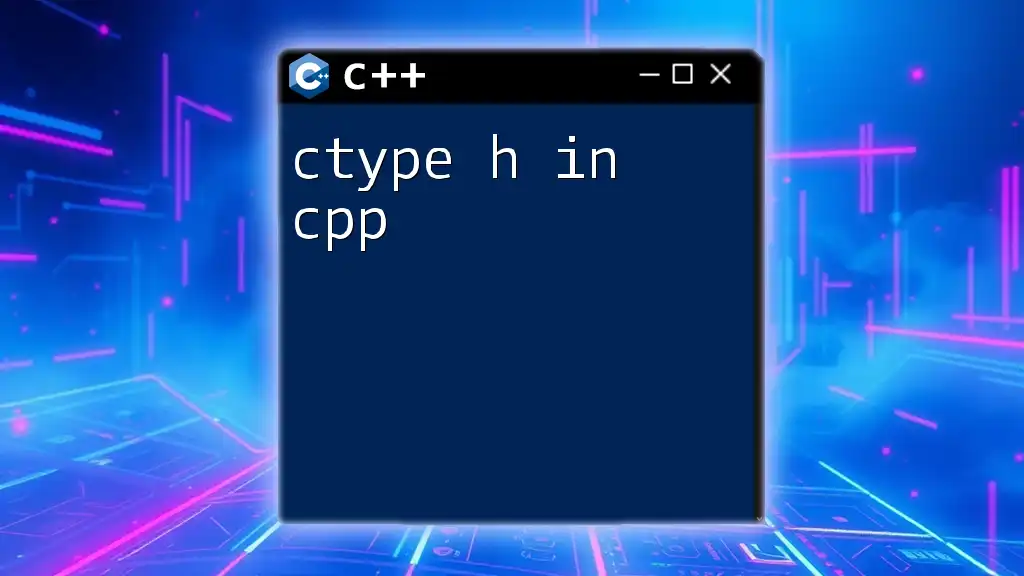
Differences Between `typeof` and Standard Type Declarations
The primary advantage of using `typeof` lies in its ability to simplify declarations. Unlike traditional type definitions, which require knowledge of the type of a variable before it is defined, `typeof` allows you to define types based on existing variables or expressions.
When to use `typeof` versus direct type declarations should depend on clarity and conciseness. If you are working with complex data types or template meta-programming, `typeof` can make your code much cleaner and easier to maintain. For example:
std::map<std::string, int> myMap;
typeof(myMap)::iterator it; // 'it' is an iterator type inferred from 'myMap'
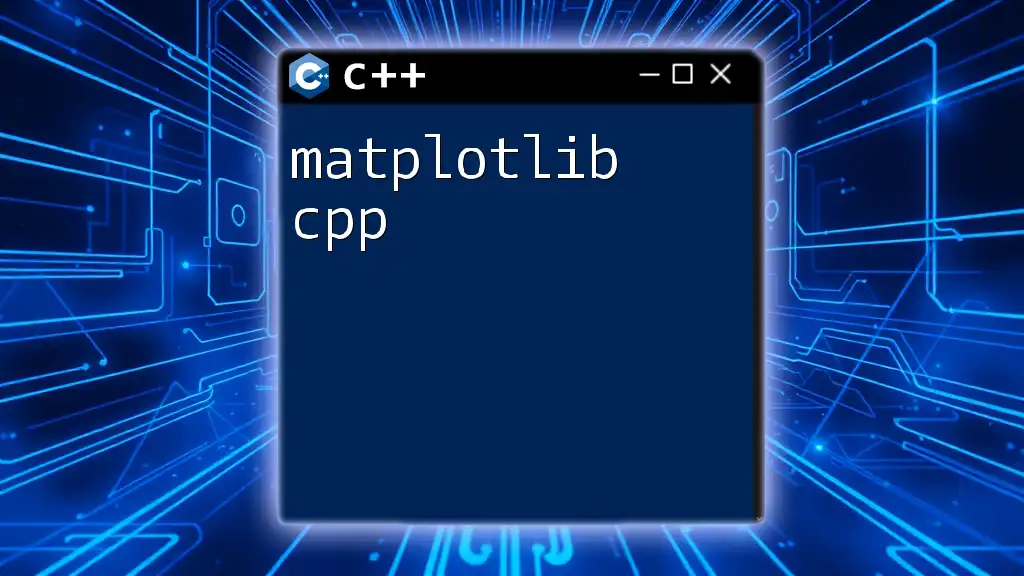
Practical Use Cases of `typeof` in C++
Dynamic Typing Scenarios
`typeof` is especially useful in scenarios where type determination is done dynamically. This is often seen in template programming or when working with callbacks and lambda functions, where types are not always obvious.
Enhancing Code Readability and Maintenance
Using `typeof` can significantly improve the readability of your code, especially in contexts where type definitions can get unwieldy. For instance:
std::vector<std::pair<int, float>> vec;
typeof(vec)::iterator it; // Clear declaration without complicating the syntax
This use case shows how `typeof` clarifies the type of `it`, making it easier for other developers (or even yourself in the future) to understand the code quickly.
Using `typeof` in Function Return Types
`typeof` can also be beneficial when defining function return types, particularly when using templates:
template<typename T>
typeof(T()) create() {
return T();
}
Here, the function `create` returns a new instance of type `T`, succinctly defined by `typeof`.
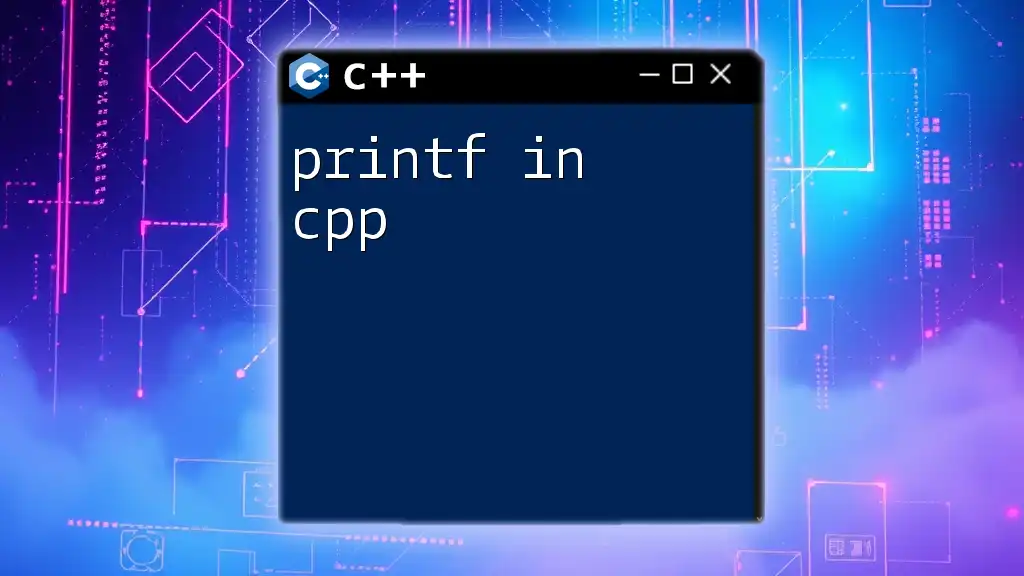
Real-World Examples
Example 1: Using `typeof` with Collections
Consider a scenario where you have a `std::vector` and you want to iterate over its elements. Using `typeof` makes the declaration of the iterator straightforward:
std::vector<int> numbers = {1, 2, 3, 4, 5};
typeof(numbers)::iterator it;
for (it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
Example 2: Nested Data Structures
When dealing with nested data structures, `typeof` can simplify the process of determining types that may be complex:
typedef std::map<int, std::vector<float>> MyMap;
MyMap myMap;
typeof(myMap)::iterator it = myMap.begin(); // It can infer the type of the iterator
Example 3: Template Programming and `typeof`
In template-heavy code, `typeof` can help keep types in check without cluttering your codebase:
template<typename T>
typeof(T()) initialize() {
return T(); // A new instance of T
}
By doing so, you ensure consistency without having to explicitly define each type, thereby reducing the risk of errors.
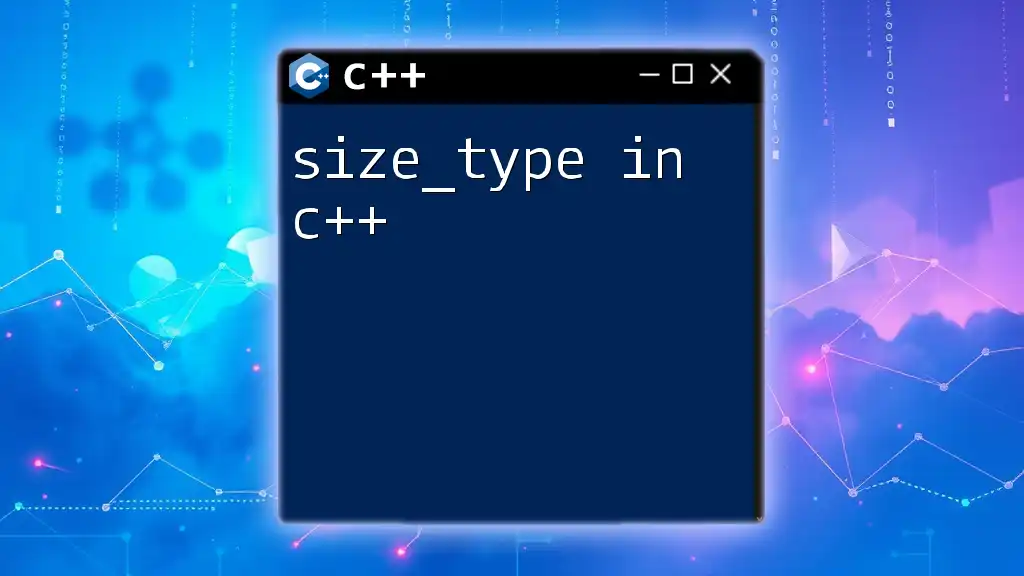
Limitations of `typeof` in C++
Despite its advantages, `typeof` is not without its limitations. It can introduce ambiguity in cases where type inference is not straightforward, especially when dealing with overloaded functions or templates. Moreover, `typeof` is not universally supported across all C++ compilers, making it necessary to ensure compatibility based on the chosen development environment.
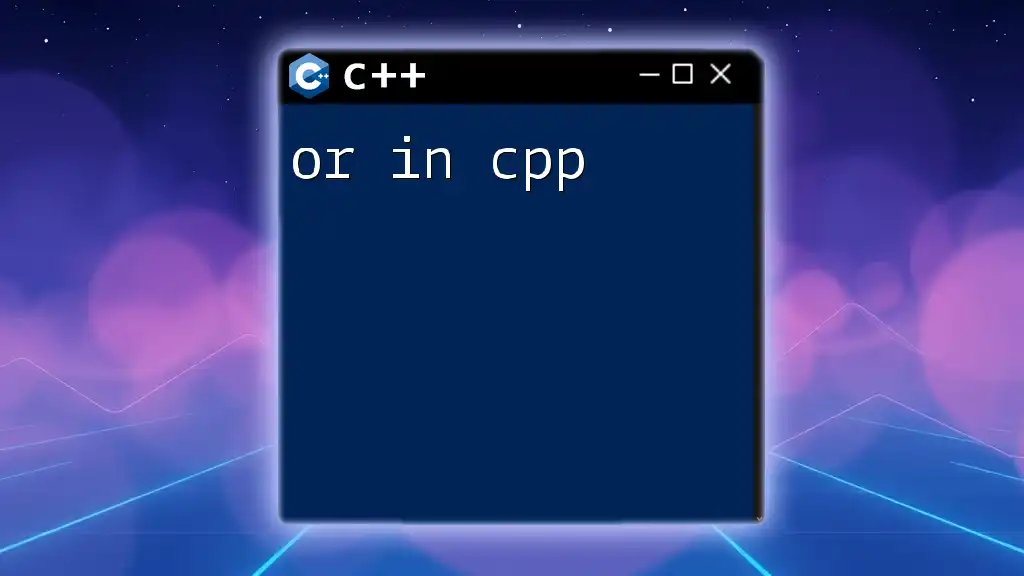
Alternatives to `typeof` in C++
If you're looking for alternatives to `typeof`, consider the following keywords that are part of the C++ standard:
`decltype`
`decltype` is designated for determining the type of an expression without evaluating it. It offers similar functionality and is part of the C++11 standard.
int x = 10;
decltype(x) y; // y is inferred to be of type int
`auto`
The `auto` keyword allows for automatic type inference, which can often serve as a more flexible alternative to `typeof`:
auto z = 15; // z is inferred to be of type int
Comparison of `typeof`, `decltype`, and `auto`
While `typeof` provides type inference based on existing expressions, `decltype` checks types without evaluation and `auto` simplifies type declarations. A practical example comparing the three could be:
int x = 5;
typeof(x) a; // a is of type int
decltype(x) b; // b is also of type int
auto c = x; // c is inferred as int
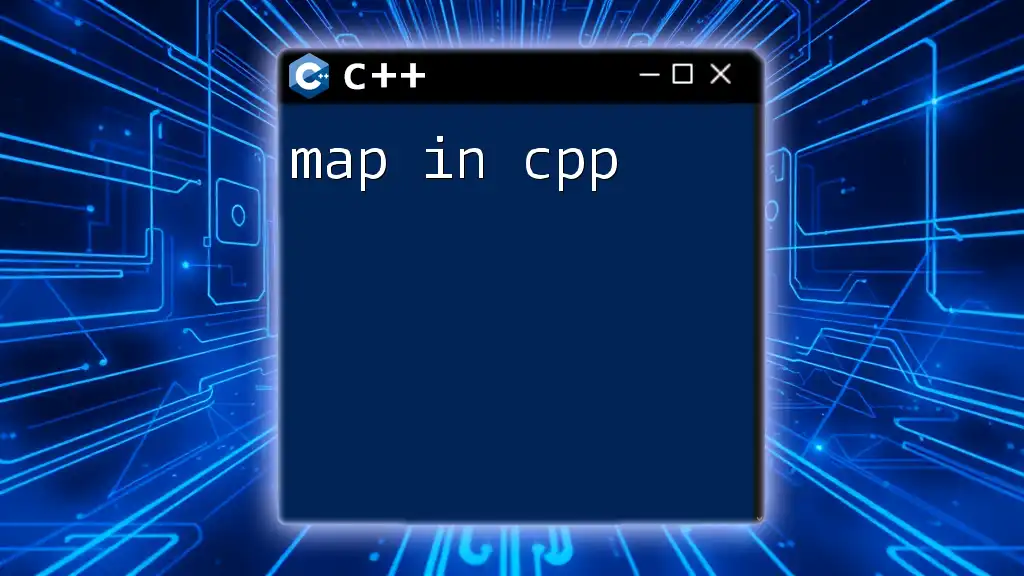
Future of `typeof` in C++
The future of `typeof` in C++ remains relevant as the language continues evolving. While it may be less common in modern codebases given the introduction of `decltype` and `auto`, understanding `typeof` can still enhance a developer's ability to write clean, concise, and maintainable code.
As C++ standards progress, the role of type inference will undoubtedly become even more essential, ensuring that developers can keep pace with complex programming needs while maintaining clarity in their code.
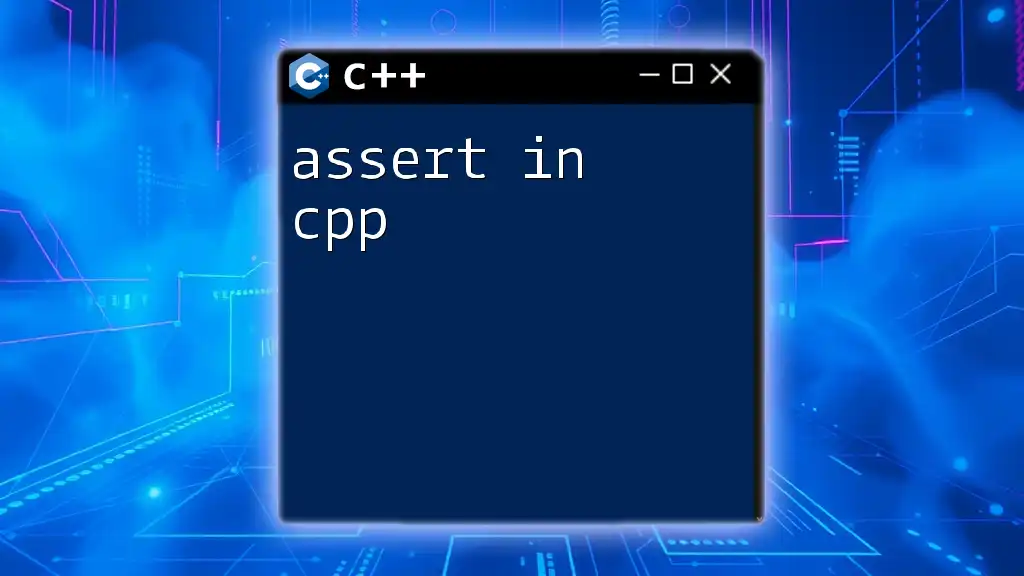
Conclusion
In summary, `typeof` in C++ is a powerful tool for type inference that can enhance code readability and simplify complex declarations. By understanding its syntax, practical applications, and limitations, developers can leverage this feature to write cleaner and more efficient code. So, consider incorporating `typeof` into your programming practices, and explore its beneficial uses in your projects for a smoother coding experience.