The `new` operator in C++ is used to dynamically allocate memory for an object or array during runtime, allowing for flexible memory management.
// Example of using new to allocate memory for an integer
int* myNumber = new int(42);
// Don't forget to free the allocated memory when done
delete myNumber;
Dynamic Memory Allocation
Dynamic Memory Allocation is a powerful feature used to allocate memory at runtime. This contrasts with static memory allocation, where the size of the memory must be known at compile time. Dynamic memory allows for more flexible and efficient use of memory, especially in scenarios where the amount of required memory cannot be predetermined.
The ability to allocate and free memory dynamically is crucial for many types of applications, particularly those that need to manage resources effectively, like graphics applications, real-time simulations, and data-heavy applications.
Why Use the `new` Operator in C++?
The `new` operator in C++ is fundamental for creating dynamic memory. It provides several advantages:
- Flexibility: You can allocate memory based on user input or runtime conditions rather than beforehand.
- Efficiency: It can help minimize wasted memory by freeing up space when it's no longer needed.
- Complex Data Structures: It allows for the creation of complex data structures, such as linked lists, trees, and graphs, which might require dynamic memory allocation for their nodes.
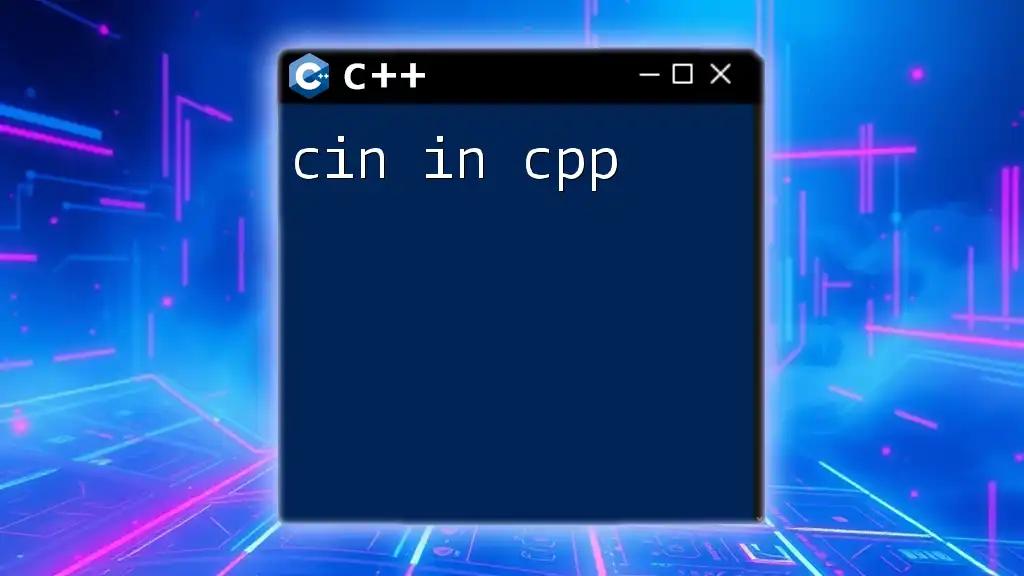
The `new` Operator in C++
Understanding the Syntax of the `new` Operator
The basic syntax of the `new` operator is straightforward:
Type* ptr = new Type;
In this example, you are declaring a pointer `ptr` of type `Type` and allocating memory for it using `new`. This memory will remain allocated until it is explicitly released with `delete`.
Example: Allocating Basic Data Types
To illustrate the `new` operator, consider the following code snippet that allocates memory for an integer:
int* ptr = new int;
*ptr = 42;
In this case, memory for an integer is allocated, and the value `42` is assigned to that memory address. This is a simple demonstration of dynamic memory allocation in action.
Common Use Cases for the `new` Operator
Creating Objects with `new`
The `new` operator can also be used for creating instances of classes in C++. Here’s a simple example:
class Dog {
public:
Dog() { /* constructor code */ }
};
Dog* myDog = new Dog();
Here, a new `Dog` object is being created dynamically. The constructor of the `Dog` class is called, and the object remains allocated until it's explicitly deleted.
Arrays and the `new[]` Operator
When you want to allocate an array dynamically, you can use the `new[]` syntax:
int* arr = new int[10];
This line allocates memory for an array of 10 integers. You can initialize this array as follows:
for (int i = 0; i < 10; ++i) arr[i] = i;
This will populate the `arr` array with integers from 0 to 9.
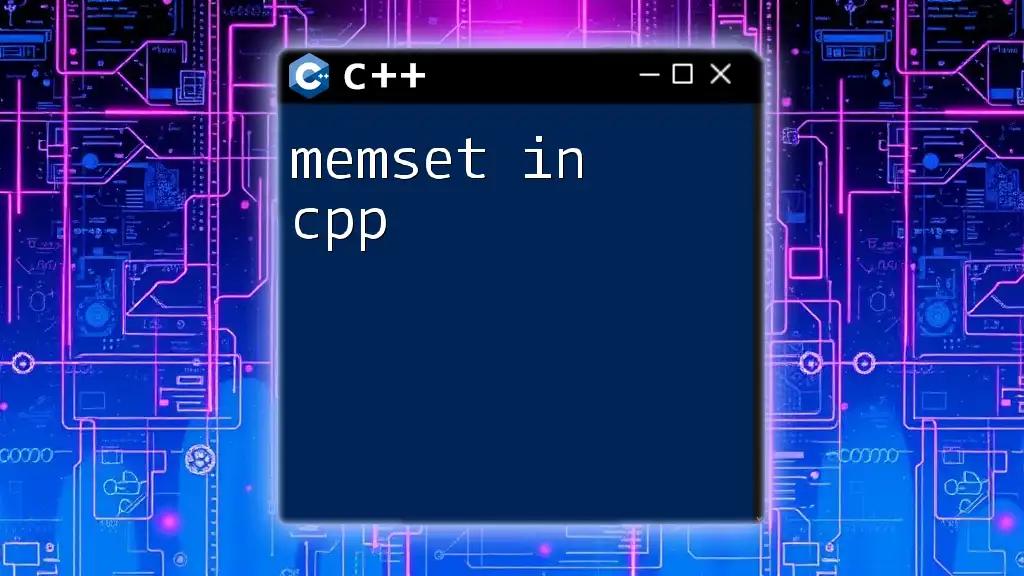
Memory Management with `new` and `delete`
Understanding Memory Leaks
A memory leak occurs when a program allocates memory on the heap but fails to free it. As a result, that memory remains allocated even when it is no longer needed, leading to increased memory usage and potentially exhausting the available memory, which in extreme cases can crash the program.
Using the `delete` Operator
To effectively manage memory and avoid leaks, it’s essential to use the `delete` operator to free allocated memory:
delete ptr; // For single object
delete[] arr; // For array
The first command releases the memory allocated for the integer pointed to by `ptr`. The second command releases the memory allocated for the array `arr`.
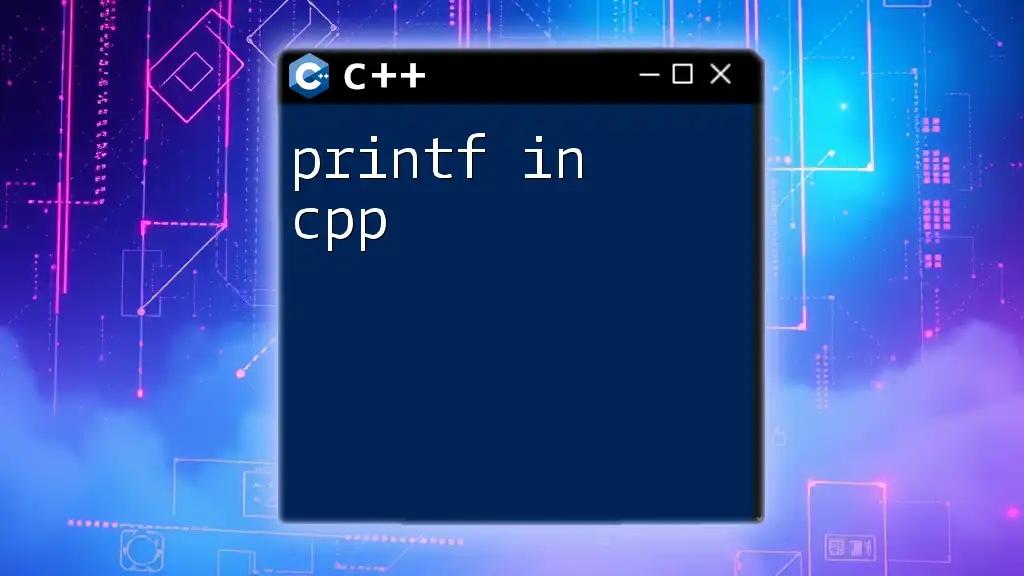
Best Practices for Using the `new` Operator
When to Use the `new` Operator?
While dynamic memory allocation is powerful, it should be used judiciously. The `new` operator is ideal when:
- The lifetime of the data must exceed the scope in which it was created.
- You need to allocate large amounts of memory that may not fit on the stack.
Avoiding Memory Leaks
To avoid leaks, always ensure that every `new` operation has a corresponding `delete` operation. Tools like Valgrind and built-in IDE memory profiling tools can help identify potential leaks in your C++ applications.
Using Smart Pointers
Modern C++ provides smart pointers like `std::unique_ptr` and `std::shared_ptr` that automate memory management. By using smart pointers, your code becomes safer and easier to manage, as these pointers automatically deallocate memory when they go out of scope:
#include <memory>
std::unique_ptr<Dog> mySmartDog = std::make_unique<Dog>();
This approach minimizes the risk of memory leaks since `mySmartDog` automatically releases its memory when it is no longer in use.
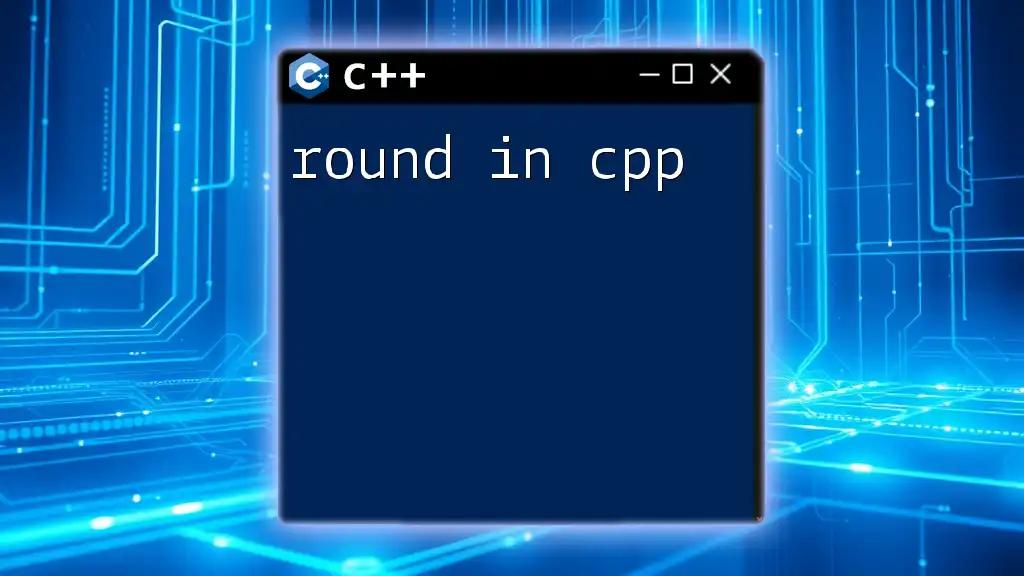
Advanced Topics
Placement New in C++
Placement new allows construction of an object in a pre-allocated buffer. This can be useful for optimizing performance in certain applications. The syntax looks like this:
void* buffer = operator new(sizeof(MyClass));
MyClass* obj = new (buffer) MyClass();
This method constructs `MyClass` in the memory location pointed to by `buffer`. It is essential to ensure that this memory is properly managed afterward, as it does not automatically deallocate.
Using `new` with Custom Allocators
Creating a custom allocator can optimize memory management in applications with specific needs or constrained environments. It introduces a level of control over memory allocation and deallocation patterns.
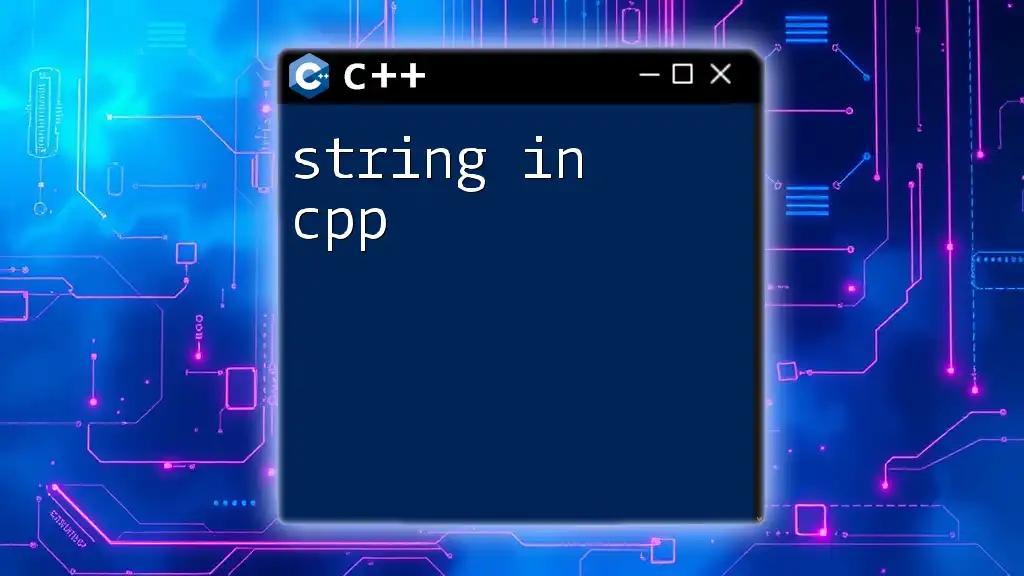
Conclusion
In summary, understanding new in C++ is crucial for effective memory management in your applications. By mastering the `new` operator, its syntax, memory allocation practices, and utilizing smart pointers, you will enhance the reliability and performance of your C++ projects.
For further learning, consider exploring comprehensive resources or advanced topics in C++, as this knowledge will prove invaluable in building robust applications.