In C++, the logical OR operator `||` is used to combine two boolean expressions, returning true if at least one of the expressions evaluates to true.
Here's a code snippet demonstrating its usage:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a || b) {
cout << "At least one of the conditions is true." << endl;
} else {
cout << "Both conditions are false." << endl;
}
return 0;
}
What is the "or" Operator in C++?
The "or" operator in C++ is an alternative keyword for the logical OR operator, represented by `||`. It allows developers to combine multiple boolean expressions, evaluating them to determine whether at least one condition is true. The "or" operator is particularly useful in scenarios where you want to check multiple conditions and execute a block of code if any of them are met.
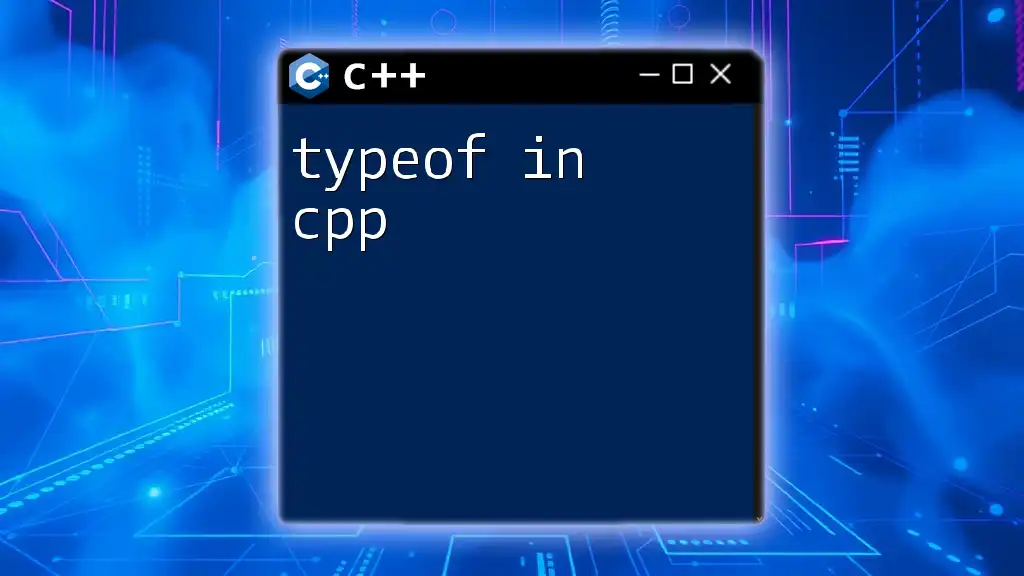
Syntax of the "or" Operator
The syntax for employing the "or" operator is straightforward. You simply use the word `or` in the logical expression:
condition1 or condition2
This evaluates to true if either condition1 or condition2 (or both) are true. It's important to note that using `or` makes your code more readable, especially for those who might be less familiar with the traditional symbols.
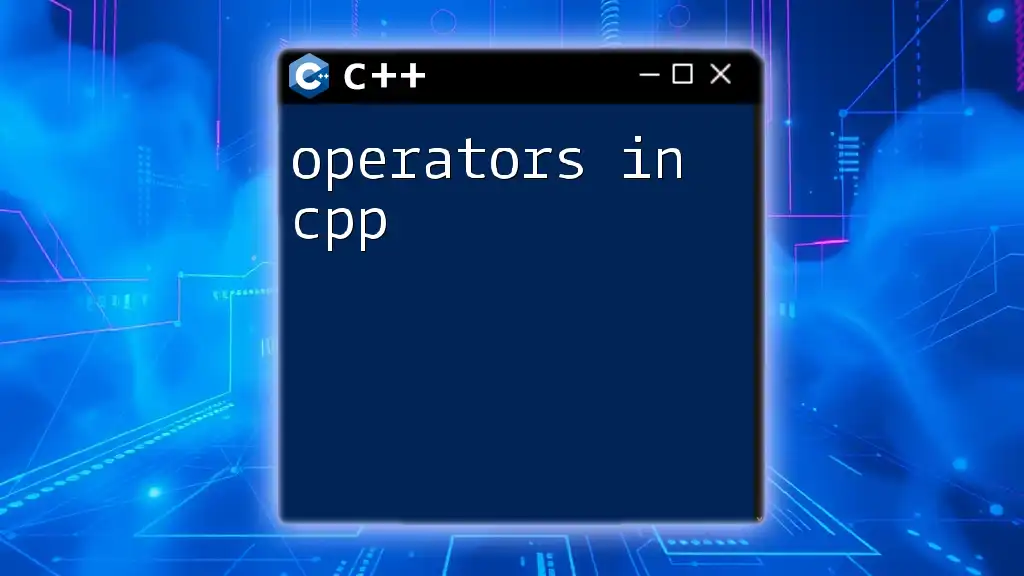
Using "or" in Conditional Statements
The Role of "or" in If Statements
Utilizing the "or" operator within if statements allows for more flexible conditional logic. For example:
if (age < 18 or status == "student") {
cout << "You qualify for a discount." << endl;
}
In this case, the code will execute if either the age is less than 18 or the status is equal to "student." This approach can significantly simplify your code when checking multiple conditions.
Combining Multiple Conditions with "or"
You can combine several conditions in a single statement by chaining the "or" operator. This is particularly useful for validating user input or permissions. For example:
if (userInput == "yes" or userInput == "no" or userInput == "maybe") {
cout << "Your input is recorded." << endl;
}
In this situation, the code executes if any of the specified string conditions are satisfied.
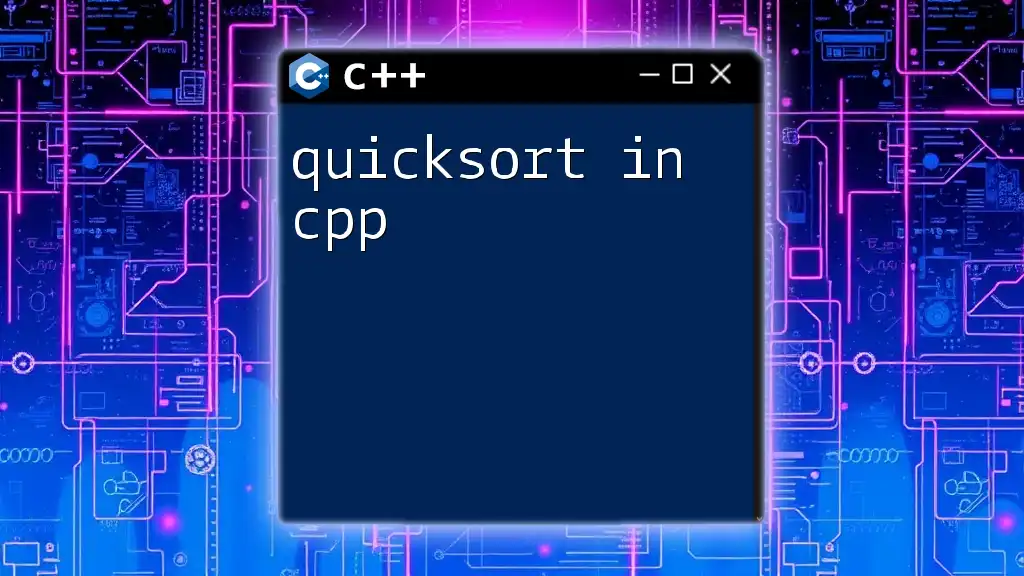
Practical Examples of "or" in C++
Example: Simple User Input Validation
An everyday use case for the "or" operator is in validating user input. For instance, when you want to ensure input belongs to a specific set of acceptable values, you can write:
string userInput;
cout << "Enter 'yes' or 'no': ";
cin >> userInput;
if (userInput == "yes" or userInput == "no") {
cout << "Valid input received!" << endl;
} else {
cout << "Invalid input. Please enter 'yes' or 'no'." << endl;
}
In this example, the program checks whether the user's input is either "yes" or "no."
Example: Checking User Permissions
The "or" operator also shines when checking user permissions for access control systems. Here’s an example:
bool isAdmin = false;
bool isModerator = true;
if (isAdmin or isModerator) {
cout << "Access granted!" << endl;
} else {
cout << "Access denied!" << endl;
}
In this scenario, the code executes the "Access granted!" message if the user is either an admin or a moderator.
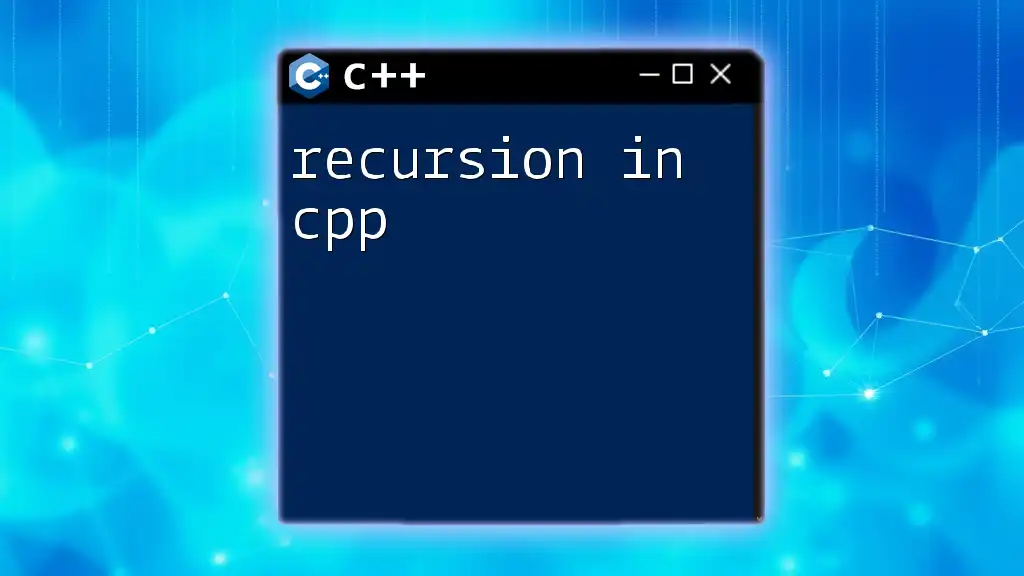
Best Practices When Using "or" in C++
Readability and Maintainability
When employing the "or" operator, prioritize the readability of your code. Clarity is paramount, especially for complex conditions. Using "or" instead of `||` can make your intentions clearer, making it easier for others (or yourself) to follow along later.
Combining with Other Logical Operators
The "or" operator can be seamlessly integrated with other logical operators like `and` or `not`. For example:
if ((a > b and a < c) or (x == y)) {
// This covers a range of conditions
}
Using parentheses helps avoid confusion, ensuring the logical expressions are evaluated in the proper order.
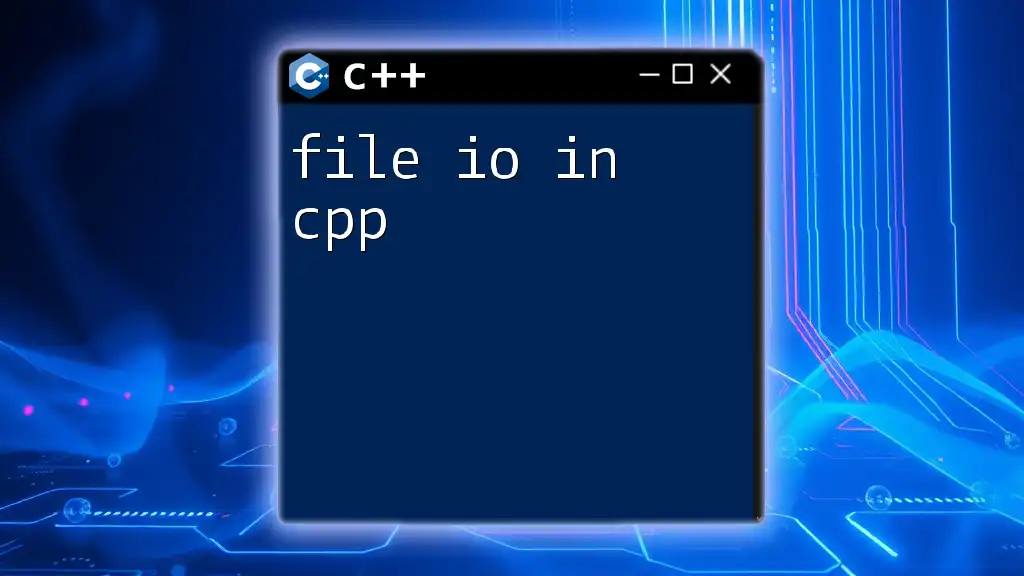
Common Mistakes to Avoid
Misusing "or" with Boolean Values
A common pitfall is misusing the "or" operator when checking boolean values. Remember that you should be comparing boolean expressions rather than assigning them as conditions. Consider this incorrect usage:
if (true or false) {
// Works as expected
}
if (a = 5 or b = 10) { // Incorrect assignment
// Will not work as expected
}
Using a single equals sign `=` is an assignment operator, not a comparison, which can lead to logic errors in your code.
Forgetting Parentheses in Complex Conditions
When combining multiple logical operators, it's easy to forget parentheses, which can lead to unintended evaluation order. For example:
if (a > 10 or b < 5 and c == 0) { // Logical error
// Corrections required
}
To ensure the correct order of operations, you should employ parentheses:
if ((a > 10) or (b < 5 and c == 0)) {
// Logic works as intended
}
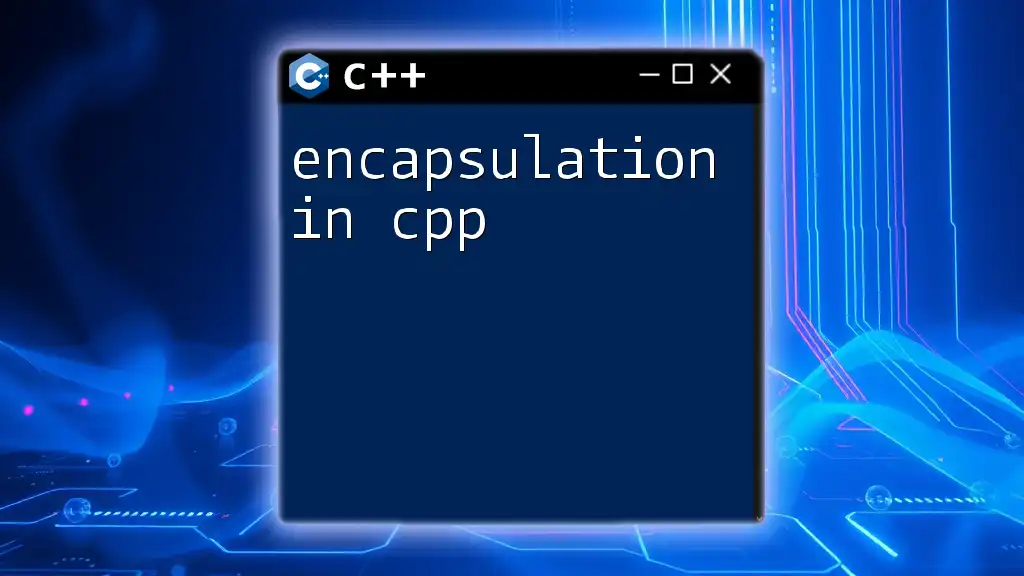
Conclusion
Understanding and effectively utilizing the "or" operator in C++ is crucial for writing clear and efficient conditional statements. By practicing the examples and avoiding common mistakes like improper assignments and oversight in parenthesis usage, you can enhance your programming skills. Experiment with various conditions and incorporate the "or" operator into your code to deepen your grasp of logical operations in C++.