Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order, effectively "bubbling" the largest unsorted element to its correct position.
Here's a basic implementation in C++:
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
swap(arr[j], arr[j+1]);
}
}
}
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
cout << "Sorted array: \n";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
What is Bubble Sort?
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The process is repeated until the list is sorted. Although it is one of the most straightforward sorting algorithms to understand and implement, it is generally not efficient for large datasets due to its average and worst-case complexity of \(O(n^2)\).
Understanding Bubble Sort is crucial for anyone learning sorting algorithms and computer science fundamentals. This algorithm serves as a great introduction to sorting methods, allowing new programmers to grasp the concepts of iteration and swapping.

How Bubble Sort Works
The core concept behind Bubble Sort lies in its iterative approach. During each pass through the array, the algorithm compares each pair of adjacent items and swaps them if they are in the wrong order. The largest unsorted element "bubbles" to the end of the array after each complete iteration.
Key Concepts
Swap: This operation refers to exchanging the positions of two elements in the array.
Iteration: This is one complete pass through the array, which could involve multiple comparisons and swaps.
Pass: Each iteration can be thought of as a pass through the list, where the largest element is moved to its correct position.

Implementing Bubble Sort in C++
To start using the Bubble Sort algorithm in C++, you will need a basic development environment set up. Tools like Code::Blocks, Visual Studio, or any C++ compliant IDE can be used.
Basic Structure of the Bubble Sort Program
Here is a simple implementation of the Bubble Sort algorithm in C++:
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
}
}
}
}
In this code:
- The first loop iterates through each element, while the nested loop compares adjacent elements.
- The `swap()` function is used to exchange the positions of two elements if they are out of order.

Detailed Breakdown of the Code
Line by Line Explanation
- `#include <iostream>`: This line includes the standard input-output stream library, allowing for console I/O.
- `using namespace std;`: This statement allows the program to use the standard library's names without prefixing them with `std::`.
- `void bubbleSort(int arr[], int n) {...}`: This function takes an array `arr` and its size `n` as parameters and sorts the array in place.
- The first `for` loop runs from 0 through \(n-1\), initiating each pass.
- The inner `for` loop runs from 0 through \(n-i-1\), ensuring only unsorted elements are compared.
Optimizing Bubble Sort
An enhanced version of Bubble Sort can improve efficiency. The minor tweak allows the algorithm to stop if no swaps are made during a pass, meaning the list is already sorted.
Here’s the optimized version of Bubble Sort:
void optimizedBubbleSort(int arr[], int n) {
bool swapped;
for (int i = 0; i < n - 1; i++) {
swapped = false;
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
swapped = true;
}
}
if (!swapped) break; // Stop the algorithm if no swaps occurred
}
}
In this version, the `swapped` boolean variable tracks whether any swaps were made in the current pass. If no swaps occur, the algorithm breaks out of the loop early, improving performance for nearly sorted arrays.
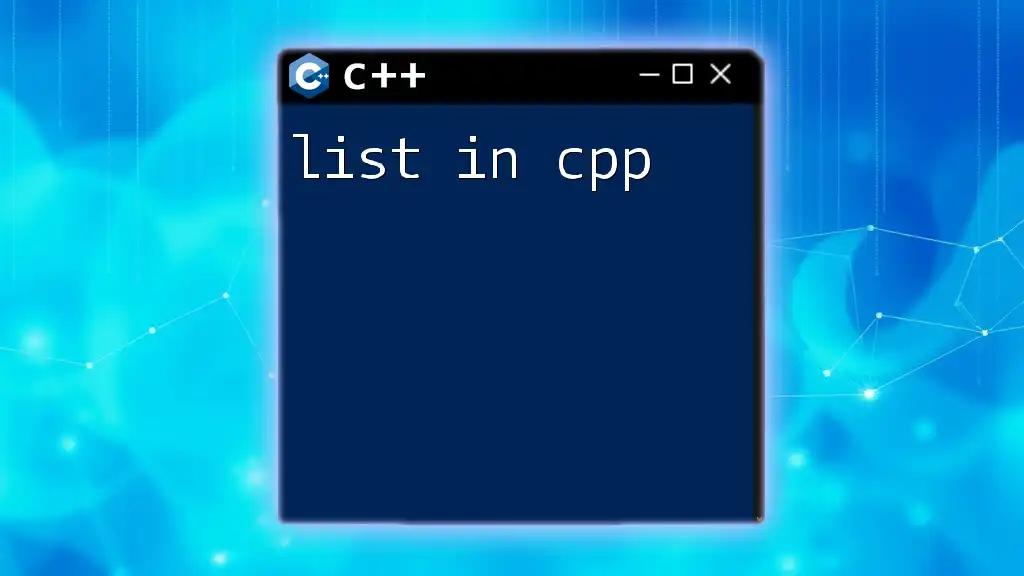
Time and Space Complexity
Analyzing Time Complexity
- Best Case: \(O(n)\) when the array is already sorted, allowing the algorithm to complete quickly due to early termination.
- Worst Case: \(O(n^2)\) when the array is sorted in reverse order, necessitating maximum comparisons and swaps.
- Average Case: Also \(O(n^2)\), considering elements in random order.
Understanding Space Complexity
The space complexity of Bubble Sort is \(O(1)\), indicating that it operates in constant space. It only uses a fixed amount of additional storage (for variables like `swapped`).
Comparative Analysis
While Bubble Sort offers a straightforward approach to sorting, it is generally outperformed by algorithms such as Quick Sort, Merge Sort, and even Insertion Sort for larger datasets. However, its simplicity often makes it a favored choice for educational purposes.
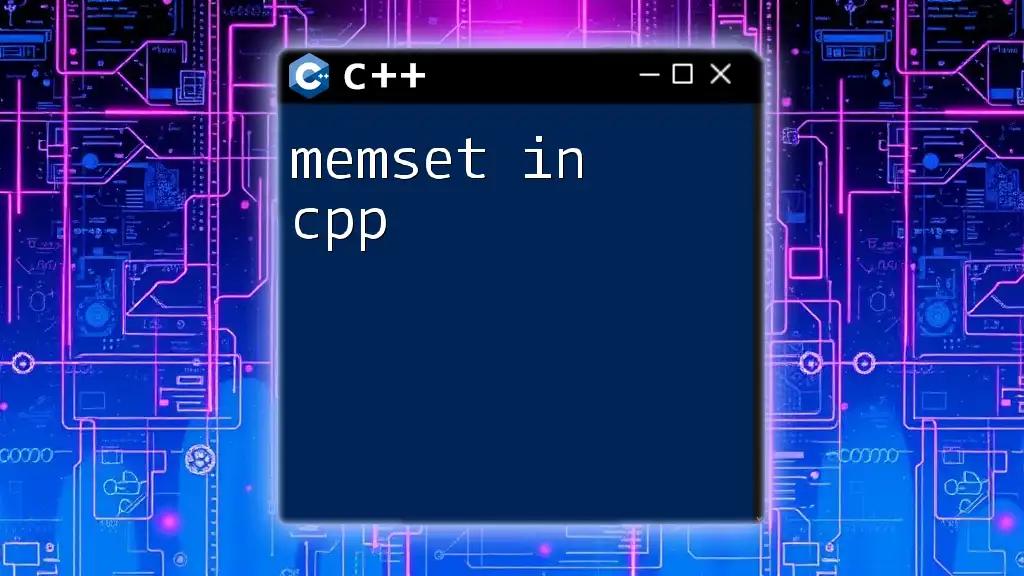
Real-World Applications of Bubble Sort
When to Use Bubble Sort
Bubble Sort has limited real-world applicability due to its inefficiency. However, it can be useful in scenarios where:
- The dataset is small.
- Educational demonstrations of sorting are required.
- The array is nearly sorted, capitalizing on the optimization technique.
Educational Purposes
Because of its easy-to-understand logic, Bubble Sort is often used in introductory programming courses. Learning this algorithm establishes a foundation for understanding more complex sorting algorithms and computer science principles.

Common Mistakes and Troubleshooting Tips
Debugging the Bubble Sort Implementation
New programmers often encounter issues such as:
- Forgetting to update the `n` value correctly.
- Bypassing the swap when conditions are met.
- Incorrectly initializing loops resulting in out-of-bound errors.
Frequently Asked Questions
- Why is Bubble Sort inefficient for large datasets? The algorithm's nested loops lead to exponential growth in time taken as data size increases, making it impractical for extensive or complex data handling.

Conclusion
In summary, understanding bubble sort in cpp allows budding programmers to learn fundamental concepts of sorting algorithms, iteration, and array manipulation. Though not the most efficient sorting method, mastering Bubble Sort sets the stage for tackling more advanced algorithms. Practicing the implementation and its variations will reinforce your coding skills and enhance algorithmic thinking.
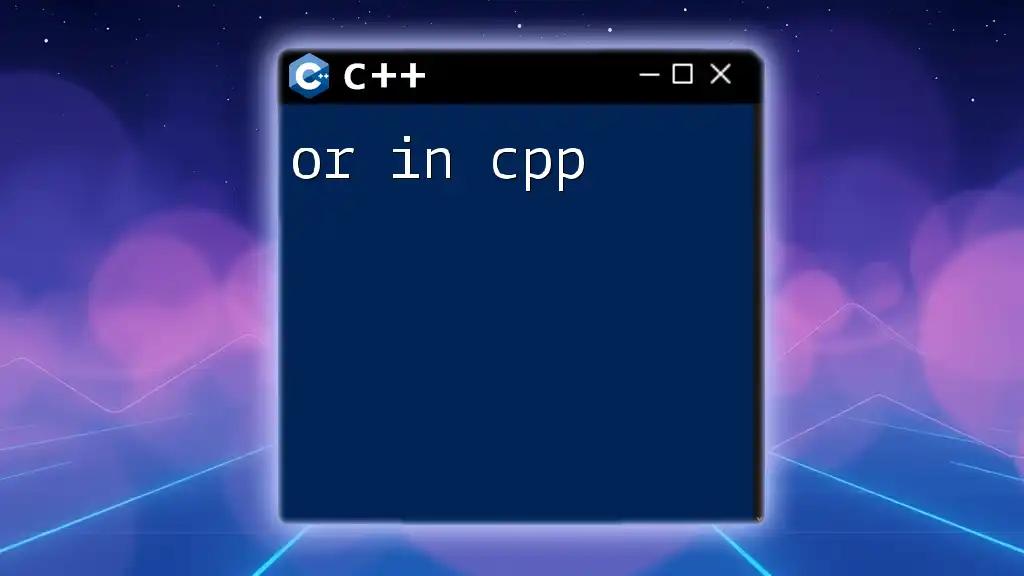
Call to Action
We encourage you to implement Bubble Sort on your own, experimenting with varying datasets and optimizing the code. Discover the nuances of this classic algorithm in your C++ projects, and enjoy the process of learning!
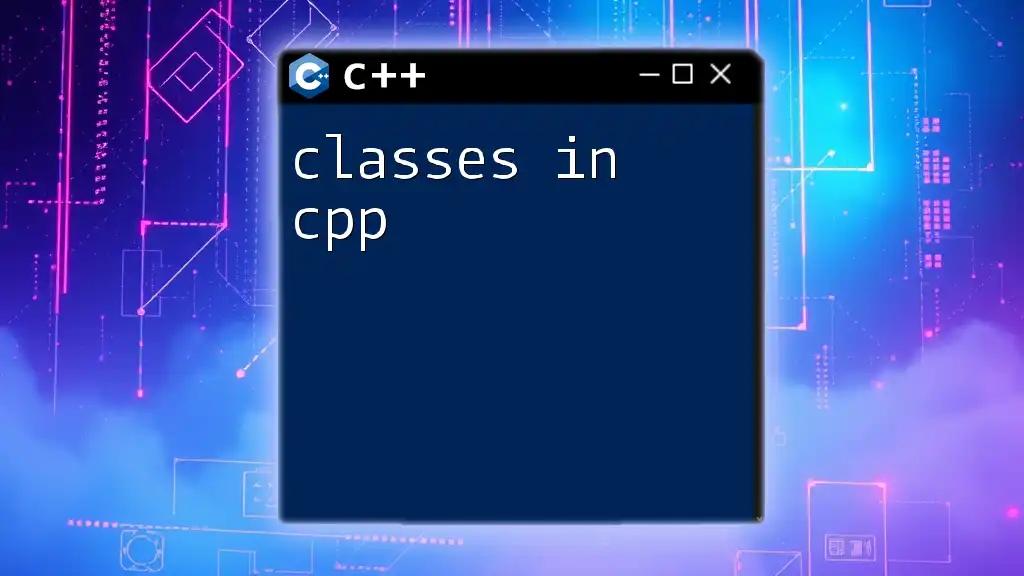
Appendix
Complete Code Example
Here's a complete implementation incorporating both basic and optimized Bubble Sort versions:
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
}
}
}
}
void optimizedBubbleSort(int arr[], int n) {
bool swapped;
for (int i = 0; i < n - 1; i++) {
swapped = false;
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
swapped = true;
}
}
if (!swapped) break; // Stop if no swaps occurred
}
}
Additional Resources
Seek out books, online courses, and reputable websites for further reading on sorting algorithms and C++. Exploring a variety of resources will deepen your knowledge and skill in the domain.