"Calculator cpp" refers to a simple C++ program that performs basic arithmetic operations, allowing users to input numbers and choose operations to calculate results efficiently.
Here's a basic example of a calculator in C++:
#include <iostream>
using namespace std;
int main() {
char operation;
float num1, num2;
cout << "Enter operator (+, -, *, /): ";
cin >> operation;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
switch(operation) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
if (num2 != 0)
cout << num1 / num2;
else
cout << "Error! Division by zero.";
break;
default:
cout << "Invalid operator.";
}
return 0;
}
What is a Calculator Program?
A calculator program is a fundamental application designed to perform mathematical operations efficiently. This could be a simple arithmetic calculator or a more sophisticated scientific calculator. The main purpose is to take user input, process calculations, and provide results promptly. Calculator programs are not only useful tools for everyday computations but play a significant role in educational contexts, computational demonstrations, and technical applications.
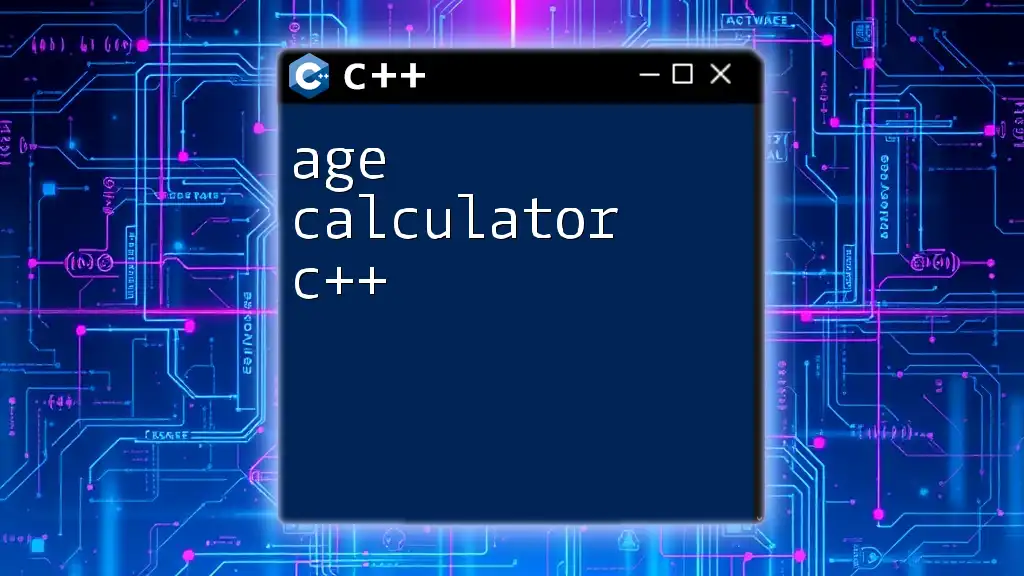
Why Use C++ for Building a Calculator?
C++ is a robust, high-performance programming language that offers a variety of features tailored for building efficient applications like calculators. Its key advantages include:
- Performance: C++ is compiled to machine code, leading to faster execution times, which is essential for real-time calculations.
- Support for Object-Oriented Programming (OOP): OOP principles allow for better code organization, making it easier to manage complex functionalities.
- Extensive Libraries: C++ has a rich set of libraries that can be leveraged to enhance calculator functionalities, such as graphical user interfaces or advanced mathematical computations.
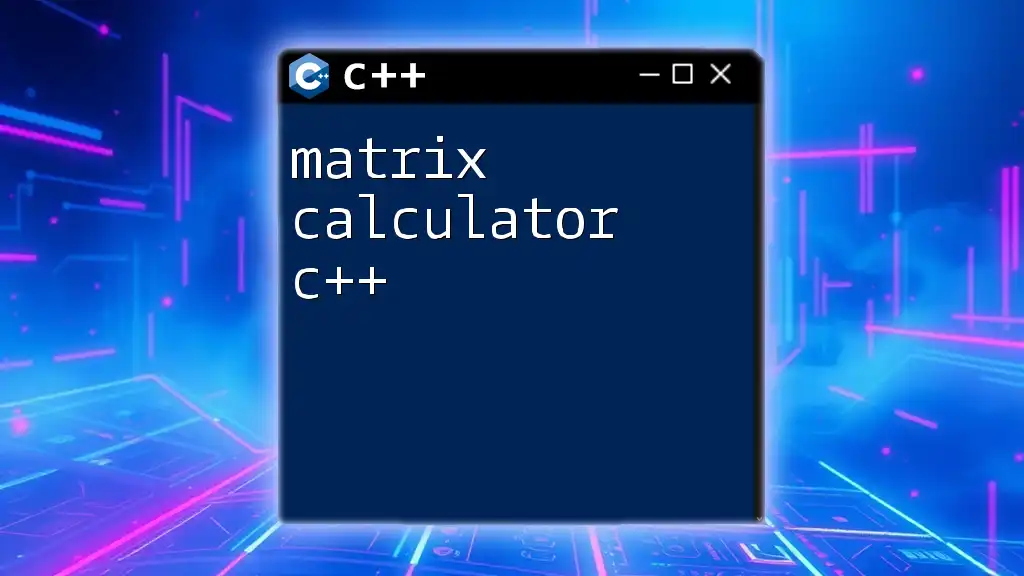
Setting Up Your Development Environment
Necessary Tools for C++ Development
Before diving into coding, it's crucial to set up your development environment properly. Start by choosing an Integrated Development Environment (IDE). Popular options include:
- Visual Studio: Known for its extensive feature set and user-friendly interface.
- Code::Blocks: Lightweight and open-source, ideal for beginners.
- Eclipse CDT: Offers advanced features for experienced developers.
Additionally, ensure that you have a C++ compiler installed. Options include GCC for Linux or MinGW for Windows.
Creating Your First C++ Project
Upon setting up your IDE, create a new project and initialize your workspace dedicated to the calculator program. This involves:
- Opening your chosen IDE.
- Selecting the option to create a new project.
- Configuring your project settings (name, output executable location, etc.).
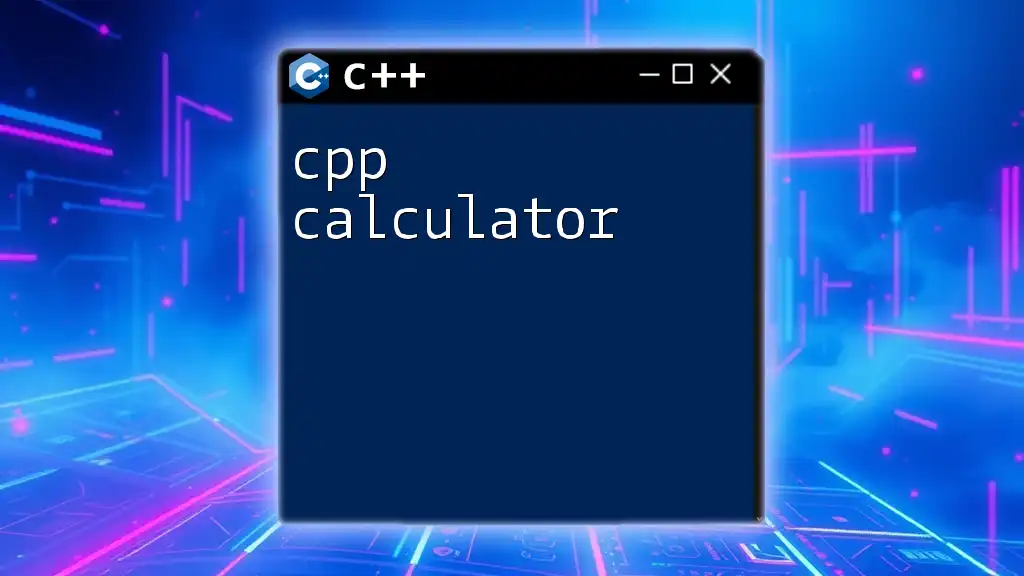
Basic Structure of a C++ Calculator
Understanding C++ Syntax
To successfully write a calculator in C++, it's essential to grasp the basic syntax. Understanding elements like data types, operators, and control structures is vital. Key components include:
- Data Types: Using `int`, `float`, or `double` for numbers.
- Operators: Including arithmetic operators such as `+`, `-`, `*`, and `/`.
- Control Structures: Utilizing `if`, `else`, and `switch` statements to process user input.
Key Components of a Calculator Program
A successful calculator program relies on three primary components:
- Input Handling: Accepting and validating user inputs is fundamental for any calculator.
- Operations: Implementing arithmetic calculations based on user requests.
- Output Display: Presenting results in a user-friendly manner.
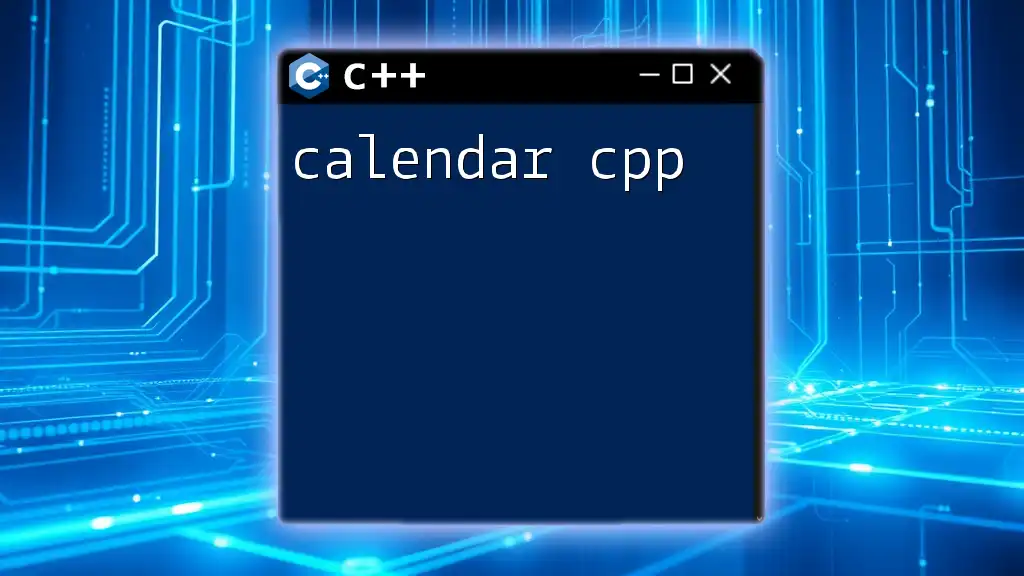
Building a Simple Console Calculator
Step-by-Step Implementation
Including Necessary Headers
Start your program by including the necessary headers. The following code snippet showcases this:
#include <iostream>
#include <cstdlib> // For exit function
Creating the Main Function
The next step is creating the main function, where the execution begins. Here's a basic setup:
int main() {
// Program code will go here
return 0;
}
Getting User Input
Utilize `std::cin` to gather input from the user. For example:
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter an operator (+, -, *, /): ";
std::cin >> operation;
std::cout << "Enter second number: ";
std::cin >> num2;
Performing Calculations
To process user requests, conditional statements can be employed. Here’s an example using a switch statement:
switch (operation) {
case '+':
std::cout << "Result: " << num1 + num2;
break;
case '-':
std::cout << "Result: " << num1 - num2;
break;
case '*':
std::cout << "Result: " << num1 * num2;
break;
case '/':
if (num2 != 0) {
std::cout << "Result: " << num1 / num2;
} else {
std::cout << "Error: Division by zero!";
}
break;
default:
std::cout << "Error: Invalid operator!";
}
Handling Errors and Edge Cases
Proper error handling is crucial. Ensure that your calculator gracefully manages situations such as dividing by zero or receiving invalid input. This can enhance user experience and prevent runtime errors.
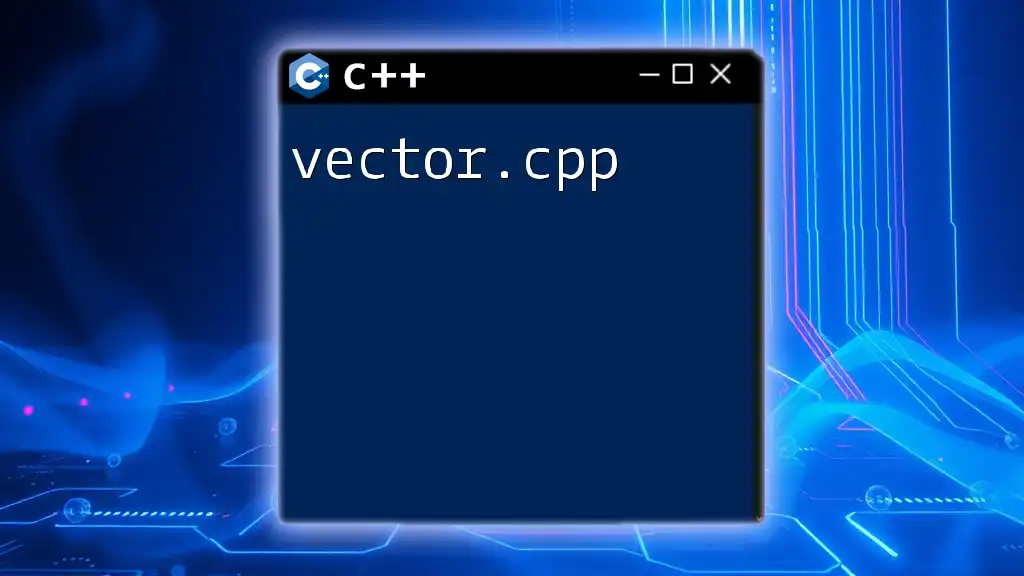
Enhancing Functionality with Features
Adding More Mathematical Operations
To make your calculator more versatile, incorporate additional operations like exponentiation and square roots. This can be done by using the `<cmath>` library:
#include <cmath>
std::cout << "Enter a number to find its square root: ";
std::cin >> num1;
std::cout << "Result: " << sqrt(num1);
Implementing a Loop for Continuous Calculation
To allow users to perform multiple calculations without restarting the program, implement a loop. The basic structure looks like this:
while (true) {
// Entire calculation process
}
Adding Support for Basic Memory Functions
Consider adding memory functions, such as storing a result temporarily. Implement operations like memory recall and memory clear for improved functionality.
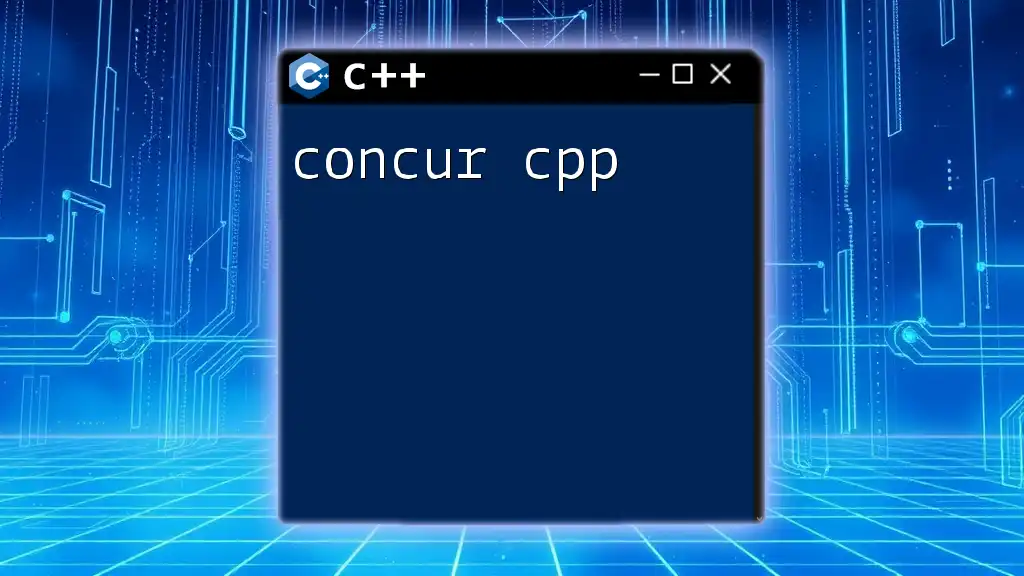
Creating a GUI-Based Calculator
Introduction to GUI Programming in C++
While console applications are excellent for beginners, graphical user interfaces (GUIs) provide a more modern user experience. Libraries such as Qt or wxWidgets are ideal for creating GUI applications.
Building a Simple GUI Calculator
Setting up a GUI calculator involves additional complexity but can significantly enhance usability. Ensure you follow the setup instructions for your chosen library, then create components like buttons, input fields, and display sections.
Handling User Input in GUI
In GUI applications, user interaction is event-driven. Understanding event handling is essential for creating responsive applications. You'll need to manage button clicks and input changes effectively to perform calculations dynamically.
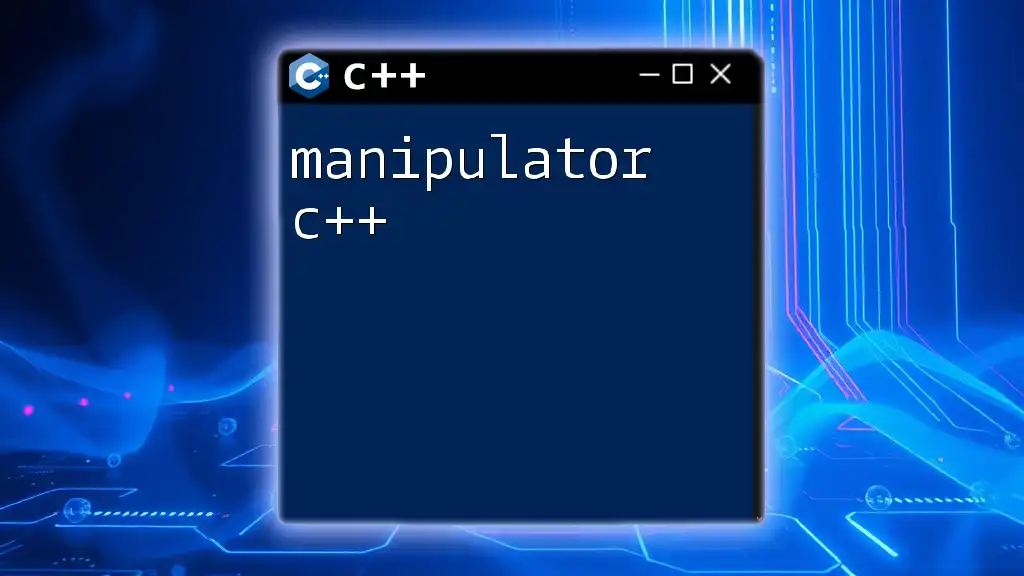
Conclusion
Building a calculator in C++ is an excellent project for both beginners and experienced developers alike. By implementing basic arithmetic operations and enhancing features progressively, you can create a robust tool that covers simple calculations to advanced functionalities.
Encouragement for further learning for C++ developers includes exploring Object-Oriented Programming, deeper mathematical operations, and even diving into graphical interfaces. Embrace the challenge of expanding your calculator's capabilities and delve into more advanced programming concepts as you continue your journey in C++.
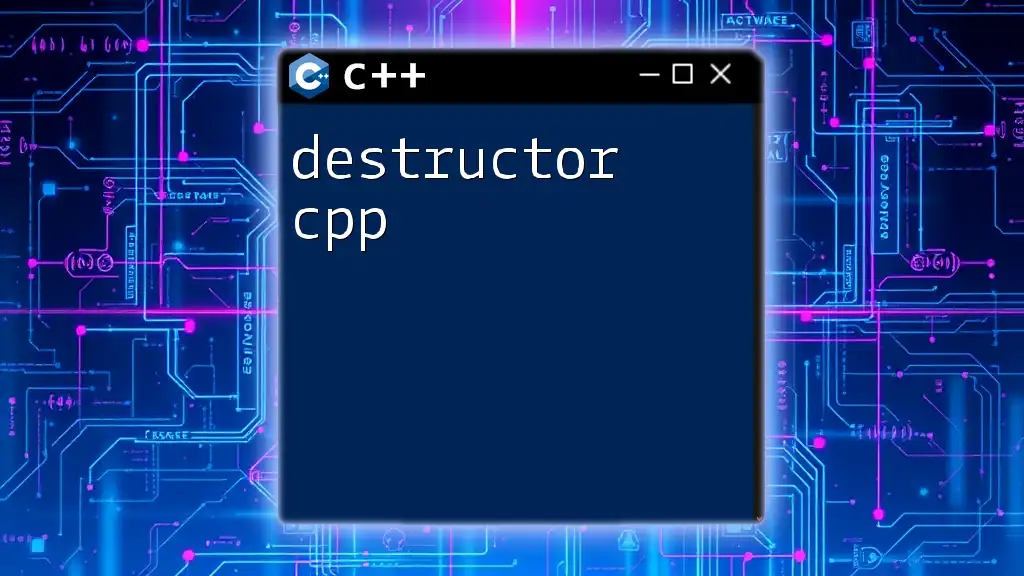
FAQs about C++ Calculator Programs
Common Questions and Answers
How to handle floating-point arithmetic? Use `double` or `float` for calculations involving decimals, and consider implementing functions to manage precision issues.
Why is my calculator not recognizing the operator? Ensure that the operator variable is being correctly read using `std::cin` and check that you have covered all operator cases in your switch statement.
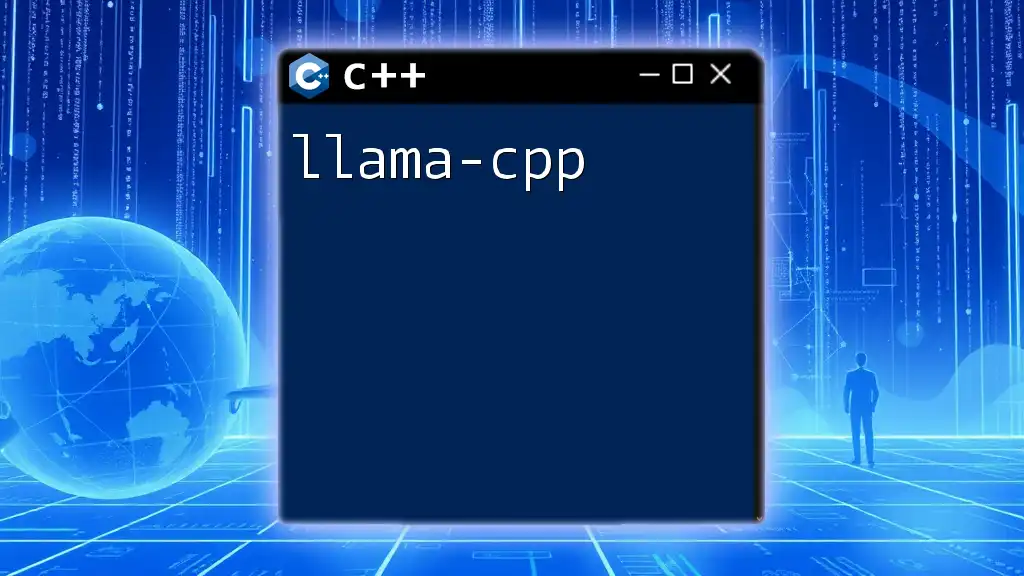
Call to Action
Join our community for more tutorials and tips! Sign up for our newsletter to receive updates and links to social media and forums for C++ enthusiasts. Whether you're a novice or an advanced programmer, there’s always more to learn and explore in the realm of C++.