A C++ GPA calculator allows users to input their grades and credits for courses to compute their Grade Point Average efficiently.
Here's a simple example of a C++ GPA calculator:
#include <iostream>
using namespace std;
int main() {
int numCourses;
double totalPoints = 0, totalCredits = 0;
cout << "Enter number of courses: ";
cin >> numCourses;
for (int i = 0; i < numCourses; i++) {
double grade, credits;
cout << "Enter grade (0.0 - 4.0) for course " << (i + 1) << ": ";
cin >> grade;
cout << "Enter credits for course " << (i + 1) << ": ";
cin >> credits;
totalPoints += grade * credits;
totalCredits += credits;
}
double gpa = totalCredits > 0 ? totalPoints / totalCredits : 0;
cout << "Your GPA is: " << gpa << endl;
return 0;
}
Understanding GPA Calculation
What is GPA?
The Grade Point Average (GPA) is a widely recognized metric used to quantify a student's academic performance. It is usually measured on a scale of 4.0 in various educational institutions. Understanding how GPA is calculated is crucial, as it often plays a significant role in academic opportunities and career prospects.
Different institutions might use varied GPA scales, like a 5.0 scale or even weighted systems that account for the difficulty of courses. Knowing the specific scale your institution uses will ensure accurate calculations.
Key Components of GPA Calculation
Calculating GPA involves two primary components: grade points assigned for each letter grade and credit hours corresponding to each course. For instance, in a standard 4.0 scale:
- A: 4.0
- B: 3.0
- C: 2.0
- D: 1.0
- F: 0.0
If a student has completed three courses with the following grades and credit hours—A (4 credits), B (3 credits), and C (2 credits)—the GPA calculation would be as follows:
-
Calculate the total grade points:
- Course 1: 4.0 * 4 = 16.0
- Course 2: 3.0 * 3 = 9.0
- Course 3: 2.0 * 2 = 4.0
-
Sum the total grade points:
- 16.0 + 9.0 + 4.0 = 29.0
-
Sum the total credit hours:
- 4 + 3 + 2 = 9
-
Divide total grade points by total credit hours:
- GPA = 29.0 / 9 = 3.22
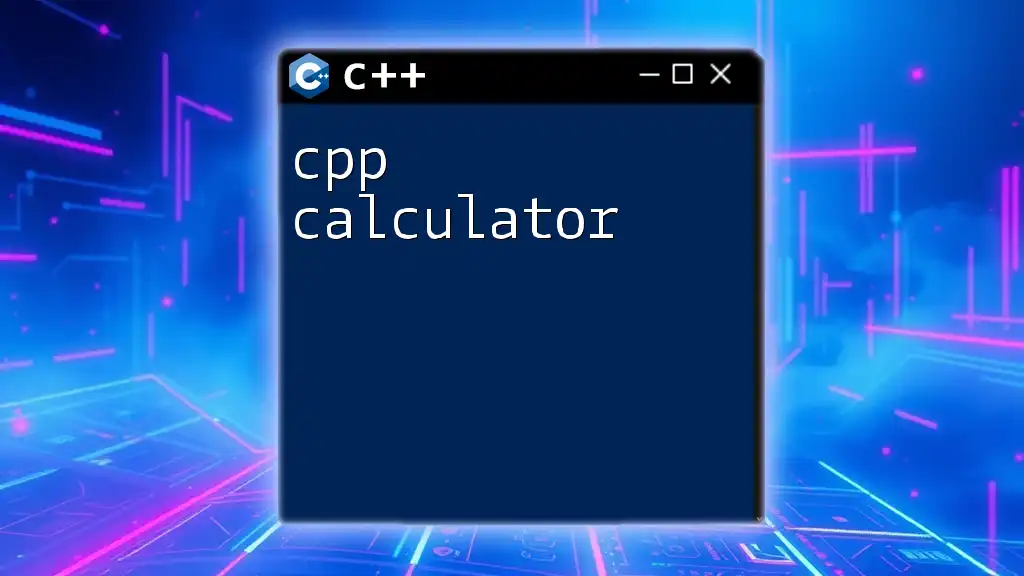
Introduction to C++
Why Use C++ for Your GPA Calculator?
C++ is an excellent choice for developing a GPA calculator due to its high performance and efficiency. C++ allows programmers to utilize object-oriented programming principles, leading to cleaner and more manageable code. This is especially beneficial when handling data and performing calculations, making it ideal for academic applications.
Setting Up Your C++ Environment
Before diving into coding, it's essential to set up a suitable development environment. Popular Integrated Development Environments (IDEs) such as Visual Studio, Code::Blocks, or even text editors with C++ support like Visual Studio Code can be used. Installing a C++ compiler (like GCC or Clang) is a necessity for compiling and executing your programs. Follow the specific instructions related to the IDE of your choice to ensure a smooth setup.
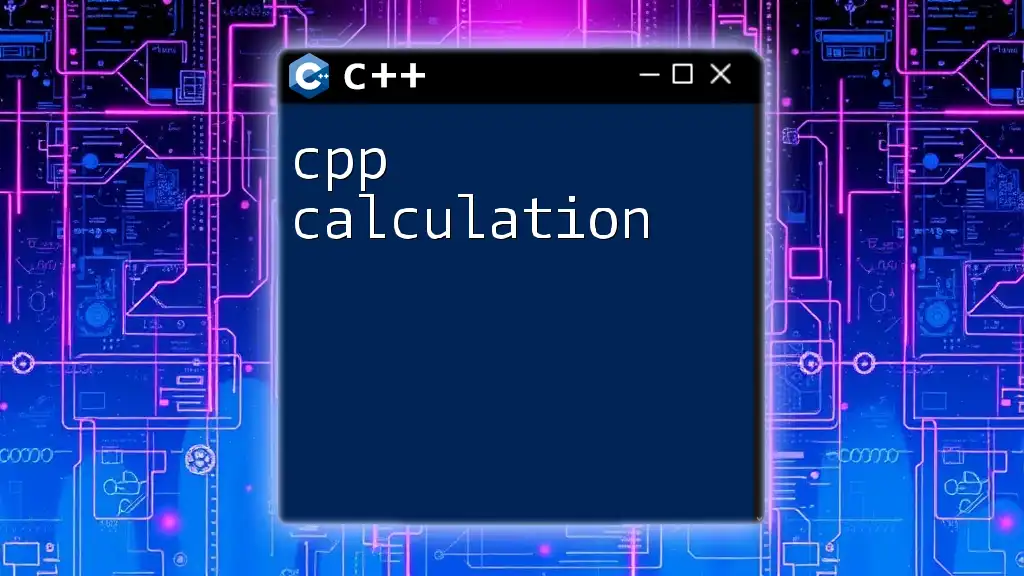
Building the GPA Calculator
Step 1: Defining the Program Structure
The foundation of a good program lies in its structure. For a C++ GPA Calculator, we will utilize functions to separate distinct functionalities, enhancing modularity and reusability. These might include functions for input gathering, GPA calculation, and output display.
Step 2: Implementing Input Functions
To calculate the GPA, we first need to gather grades and credit hours from the user. Here’s how we can do this:
#include <iostream>
#include <vector>
using namespace std;
void getInput(vector<float>& grades, vector<int>& credits) {
int numCourses;
cout << "Enter number of courses: ";
cin >> numCourses;
for(int i = 0; i < numCourses; i++) {
float grade;
int credit;
cout << "Enter grade for course " << i + 1 << ": ";
cin >> grade;
cout << "Enter credit hours for course " << i + 1 << ": ";
cin >> credit;
grades.push_back(grade);
credits.push_back(credit);
}
}
In the above code, we create a getInput function that prompts the user for the number of courses. The user is then asked to enter the grade and credit hours for each course. This information is collected in two vectors for ease of access during calculations.
Step 3: Implementing the GPA Calculation Logic
Next, we need to perform the actual GPA calculation based on the data gathered. Below is a simple function that accomplishes this:
float calculateGPA(const vector<float>& grades, const vector<int>& credits) {
float totalPoints = 0;
int totalCredits = 0;
for(size_t i = 0; i < grades.size(); i++) {
totalPoints += grades[i] * credits[i];
totalCredits += credits[i];
}
return totalCredits == 0 ? 0 : totalPoints / totalCredits;
}
This function, calculateGPA, computes the total grade points by multiplying each course’s grade by its credit hours. The total credit hours are also summed. Finally, the GPA is found by dividing the total points by total credit hours. The conditional statement ensures that we handle the case of zero credits gracefully.
Step 4: Displaying the Results
The final step is to present the GPA to the user clearly. Here's a simple function for displaying results:
void displayGPA(float gpa) {
cout << "Your GPA is: " << gpa << endl;
}
A clean output is essential for user experience. Here, we format the output message to present the calculated GPA effectively.
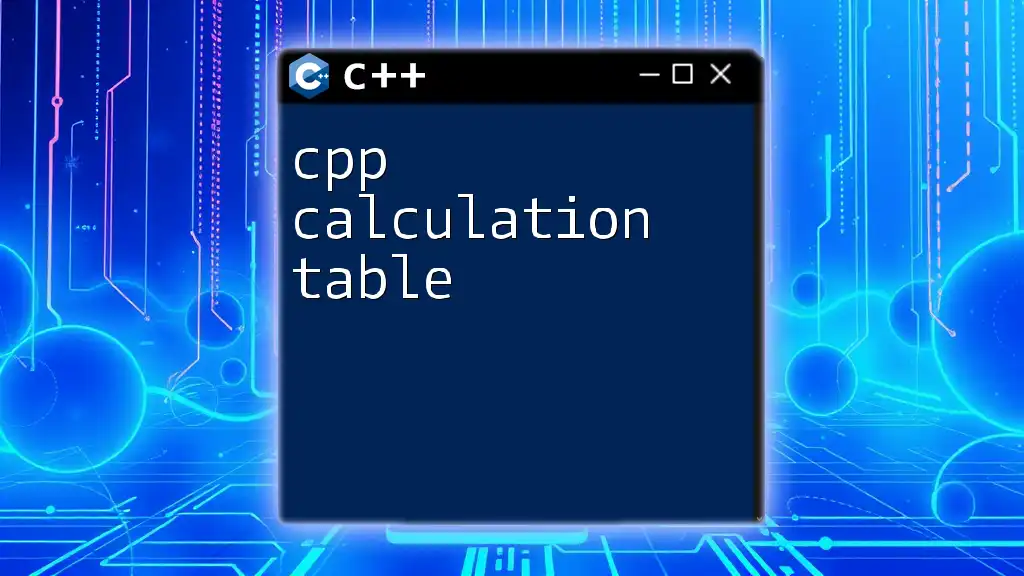
Complete Program Example
Now that we have all the individual components, we can integrate them into a full program:
#include <iostream>
#include <vector>
using namespace std;
void getInput(vector<float>& grades, vector<int>& credits);
float calculateGPA(const vector<float>& grades, const vector<int>& credits);
void displayGPA(float gpa);
int main() {
vector<float> grades;
vector<int> credits;
getInput(grades, credits);
float gpa = calculateGPA(grades, credits);
displayGPA(gpa);
return 0;
}
This complete C++ GPA Calculator incorporates input gathering, GPA calculation, and result display. Each component interacts seamlessly, demonstrating the power of modular programming.
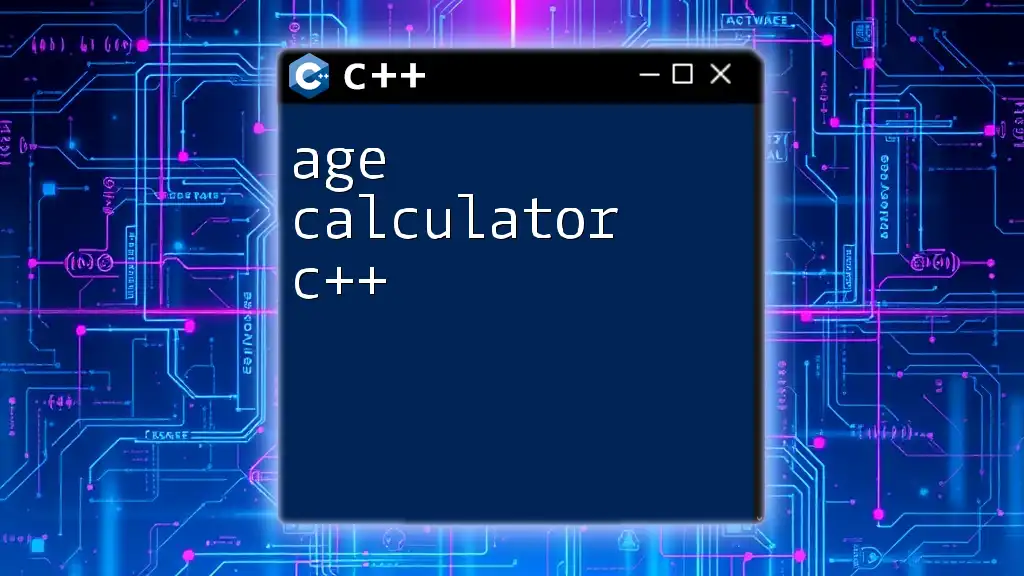
Testing Your GPA Calculator
Debugging and Validating Inputs
In any software application, especially those that involve user input, error handling is crucial. Common input errors can include invalid grades (e.g., entering a grade greater than 4.0) or negative credit hours. Implementing simple checks right after input can prevent these issues:
if (grade < 0 || grade > 4.0) {
cout << "Invalid grade entered. Please enter a valid grade." << endl;
// Handle error appropriately
}
if (credit <= 0) {
cout << "Credit hours must be greater than zero." << endl;
// Handle error appropriately
}
Testing Scenarios
Testing is vital to ensure your GPA calculator works as intended. Run through various scenarios:
- Input where all grades are the same.
- Input with varying credit hours.
- Edge cases like no courses or invalid grades.
Confirming that the expected outputs match the actual calculations will boost reliability and user trust.
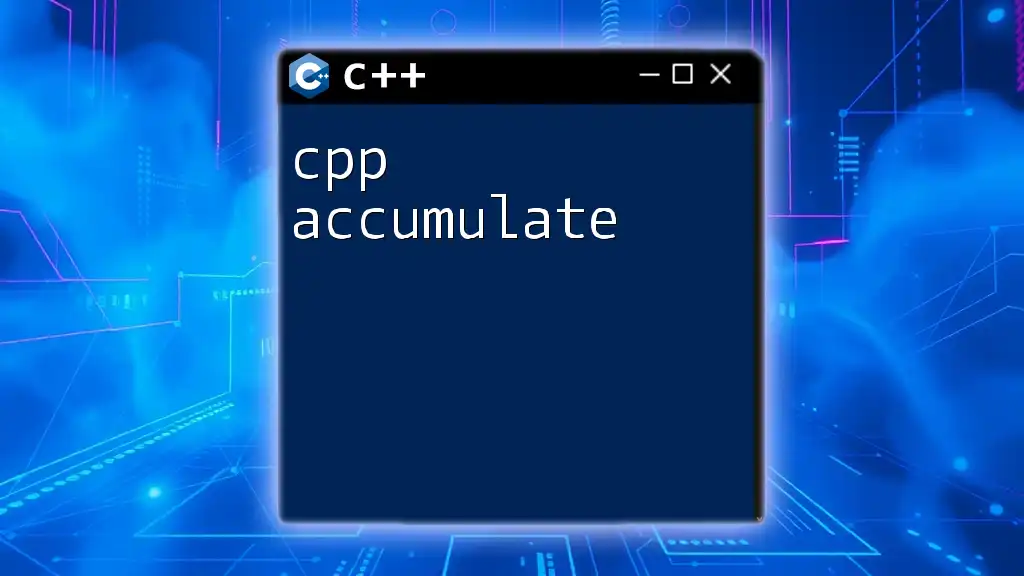
Conclusion
In this guide, we've thoroughly examined the process of creating a C++ GPA calculator. From understanding GPA calculations to implementing a functional program, each step has reinforced the importance of structure, data integrity, and user experience.
I encourage you to expand this calculator further. Consider adding features like weighted GPA calculations, historical GPA tracking, or even a graphical user interface (GUI) for enhanced usability.
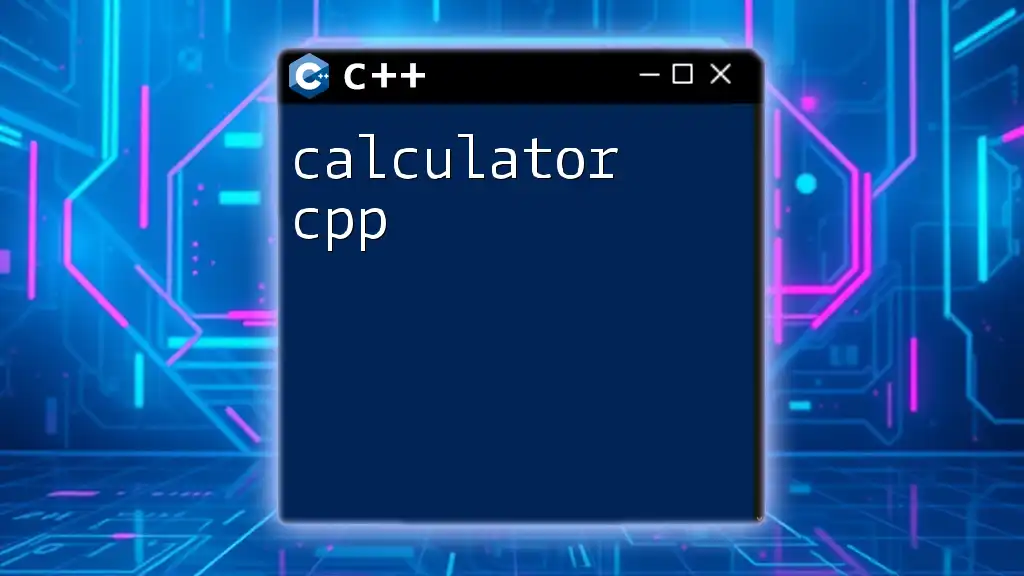
Additional Resources
To deepen your understanding of C++ and further hone your programming skills, explore official C++ documentation and online tutorials. Books from reputable authors in programming can also widen your insights and elevate your coding proficiency.
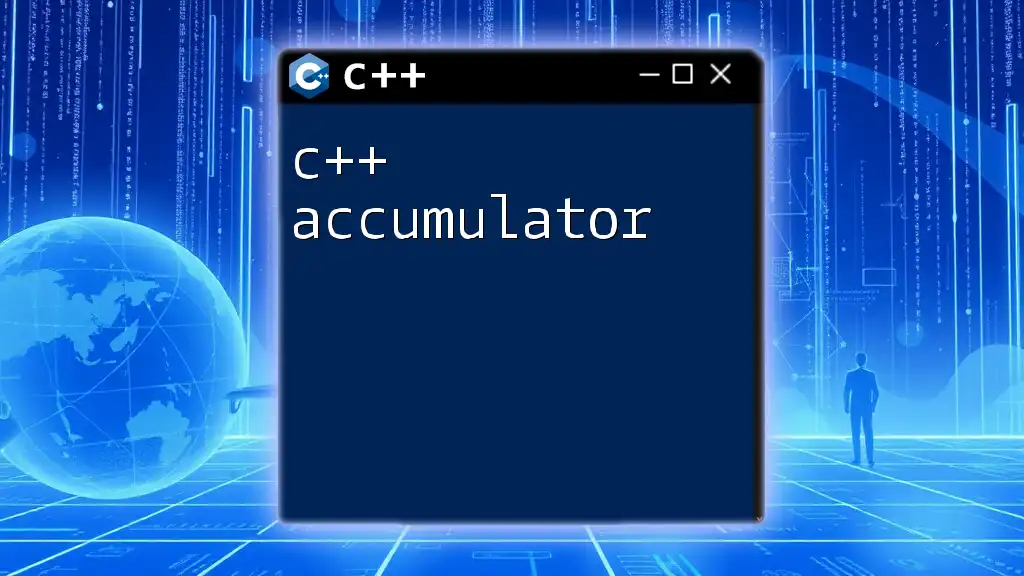
FAQs about the C++ GPA Calculator
-
What if I want to implement weighted grades?
- You can easily adjust your grade input to include weights based on course difficulty.
-
Is it possible to have a graphical user interface?
- Yes, various libraries like Qt or SFML can help you create graphical interfaces for your applications.
-
Can C++ handle larger datasets for GPA?
- Absolutely! C++ is designed to efficiently handle large datasets with minimal overhead, making it robust for educational applications.
By leveraging the insights provided in this article, you can not only calculate GPA but also strive to develop greater insights and applications that utilize C++.