C++ calculations often involve performing arithmetic operations on variables, as demonstrated in the following code snippet that calculates the sum of two integers.
#include <iostream>
int main() {
int a = 5;
int b = 10;
int sum = a + b;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Understanding CPP Commands for Calculation
Basic Concepts
C++ (CPP) is a powerful programming language that allows for efficient computational tasks. In CPP calculations, we utilize various commands that support mathematical operations. It’s essential to understand the types of data types available in CPP, such as:
- int: For whole numbers.
- float: For single-precision floating-point numbers.
- double: For double-precision floating-point numbers, allowing for more precision.
Operators in CPP
Understanding operators is fundamental for performing calculations in CPP. There are several categories of operators:
Arithmetic Operators
Operating mathematically in CPP is done using arithmetic operators:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (%): Useful for finding the remainder in division.
Comparison Operators
Using comparison operators helps in determining the relation between variables:
- Greater than (`>`)
- Less than (`<`)
- Equal to (`==`)
- Not equal to (`!=`)
Logical Operators
Logical operations allow for more complex decision-making:
- AND (`&&`)
- OR (`||`)
- NOT (`!`)
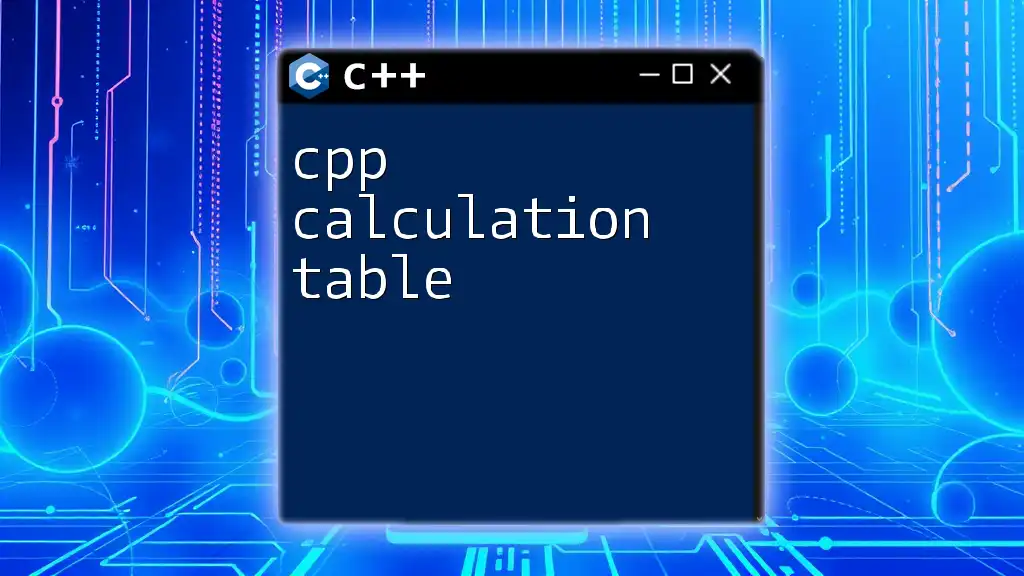
How to Figure Out CPP Calculations
Introduction to CPP Calculation Logic
To perform calculations effectively, one must grasp the logical flow of computation in CPP. A good understanding of variable declaration, initialization, and data types will set the stage for writing programs that handle calculations.
Steps to Perform a Calculation
Setting Up Your CPP Environment
First, ensure you have a suitable development environment. Popular choices include:
- IDE: Code::Blocks, Dev-C++, or Visual Studio
- Online Compilers: Repl.it or OnlineGDB
Writing a Basic Program
Here's an example of a simple addition program:
#include <iostream>
using namespace std;
int main() {
int a = 5;
int b = 10;
int sum = a + b;
cout << "The sum is: " << sum << endl;
return 0;
}
This code initializes two integers, performs addition, and outputs the result. The `cout` statement is critical in providing output to the user.
Example: Simple Addition Program
The program above shows the basic structure you’ll encounter frequently in CPP. Breaking it down:
- #include <iostream>: This includes the Input/Output stream library necessary for using `cout`.
- using namespace std;: This line allows you to use standard features without needing to prefix them with `std::`.
- The main function is the entry point of execution.
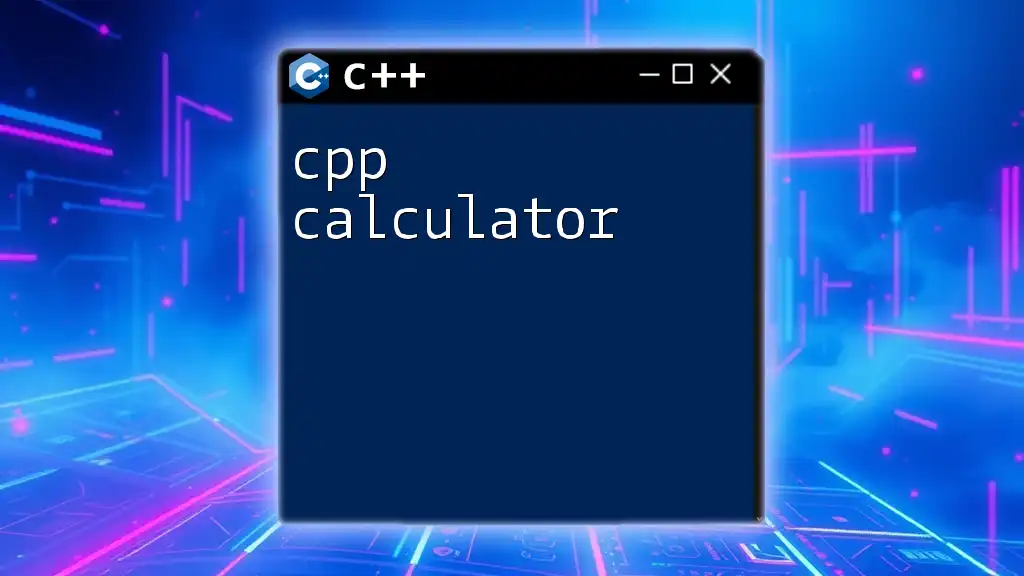
CPP Calculator Formula
Writing Functions for Calculations
Functions are a core component of CPP, allowing us to encapsulate a series of operations. Creating a dedicated function for calculations raises code reusability and clarity.
Creating a Generic Calculator Function
Consider the following generic calculator function:
#include <iostream>
using namespace std;
float calculate(float a, float b, char operation) {
switch(operation) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/':
if (b != 0) return a / b;
else {
cout << "Division by zero error!" << endl;
return 0;
}
default: return 0;
}
}
Here is how it works:
- The function takes two float parameters and a character indicating the operation.
- By utilizing a switch-case structure, it performs the respective arithmetic operation.
- It also includes error handling for division by zero.
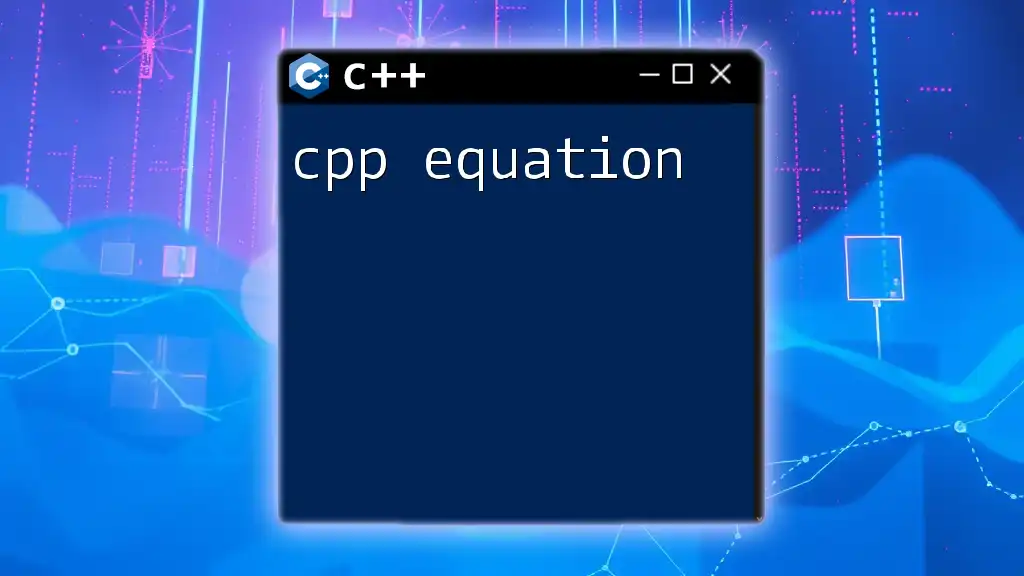
CPP Calculation Formula: Syntax and Usage
Understanding Syntax in CPP
Proper syntax is crucial for C++ programming. Key elements include the correct usage of semicolons to end lines, brackets for enclosing blocks of code, and ensuring data types match their variables.
Using the Calculation Formula Accurately
For accurate results, always double-check your operator usages and ensure your calculations align with expected mathematical principles.
Examples of Common Calculations
Common calculations might involve multiple operations. For instance, when evaluating expressions like:
float result = (a + b) * (c - d) / e;
Ensure that the order of operations (PEMDAS/BODMAS) is respected.
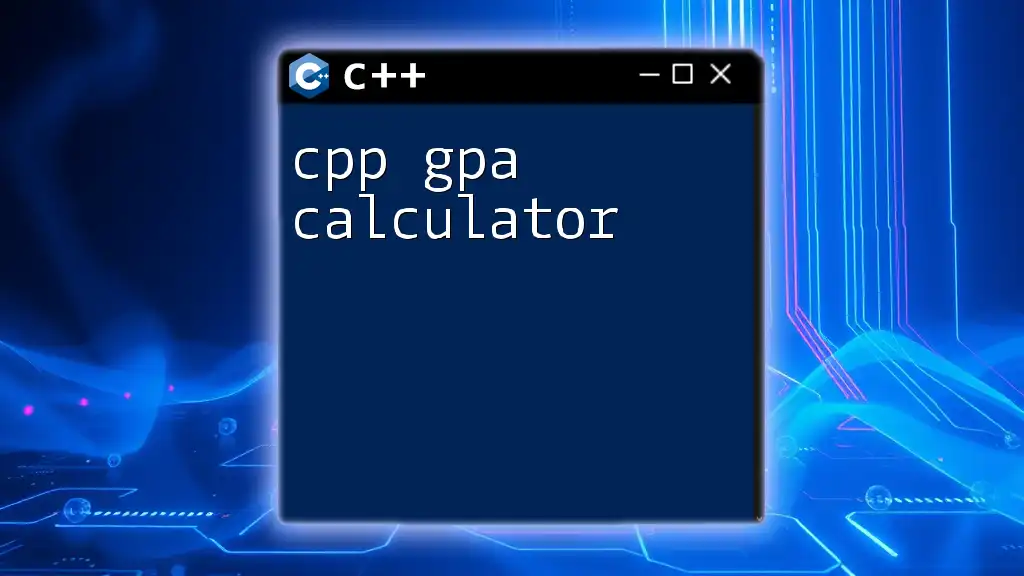
CPP Computation Examples
Basic Arithmetic Calculation Examples
Let’s cover a few simple arithmetic operations:
#include <iostream>
using namespace std;
int main() {
int x = 10, y = 2;
cout << "Addition: " << (x + y) << endl;
cout << "Subtraction: " << (x - y) << endl;
cout << "Multiplication: " << (x * y) << endl;
cout << "Division: " << (x / y) << endl;
cout << "Modulus: " << (x % y) << endl;
return 0;
}
This program demonstrates how to perform basic arithmetic operations and output the results.
Advanced Calculation Examples
Additionally, CPP offers various built-in functions for advanced calculations. For example, to calculate powers and square roots:
#include <cmath>
#include <iostream>
using namespace std;
int main() {
double base = 3.0;
double exponent = 2.0;
double result = pow(base, exponent);
cout << "3^2 = " << result << endl;
return 0;
}
In this case, we import the cmath library, enabling mathematical functions like `pow()`.
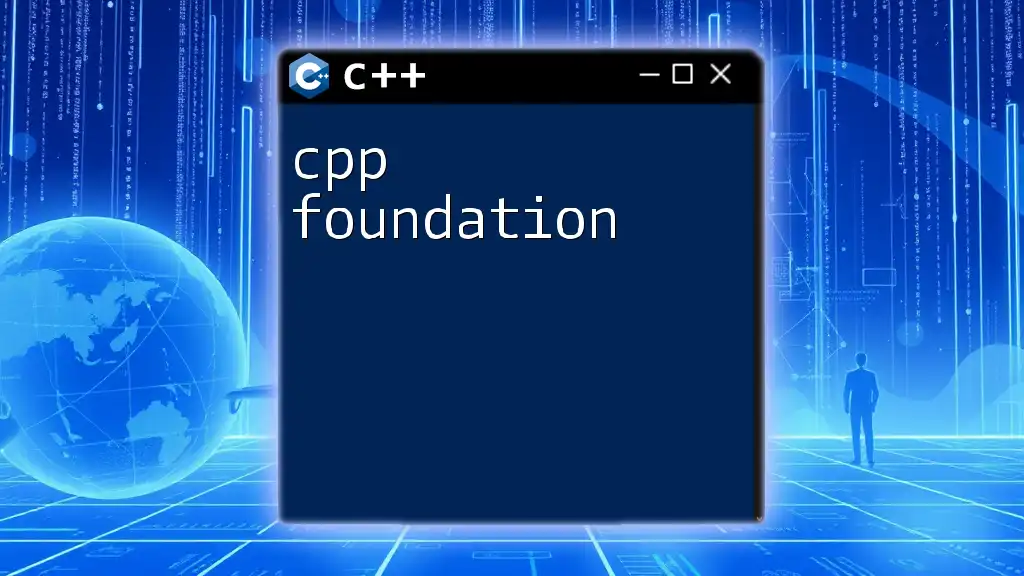
Error Handling in CPP Calculations
Common Errors During Calculation
When performing calculations, common issues include division by zero or using incompatible data types, which can lead to runtime errors.
Strategies for Error Handling
Implementing strategies such as if-statements can help ensure your code operates smoothly. Here’s an example:
#include <iostream>
using namespace std;
int main() {
float num;
cout << "Enter a number: ";
cin >> num;
if (cin.fail()) {
cout << "Invalid input! Please enter a valid number." << endl;
} else {
cout << "You entered: " << num << endl;
}
return 0;
}
This program checks for invalid input and provides user feedback, thereby enhancing the robustness of your code.
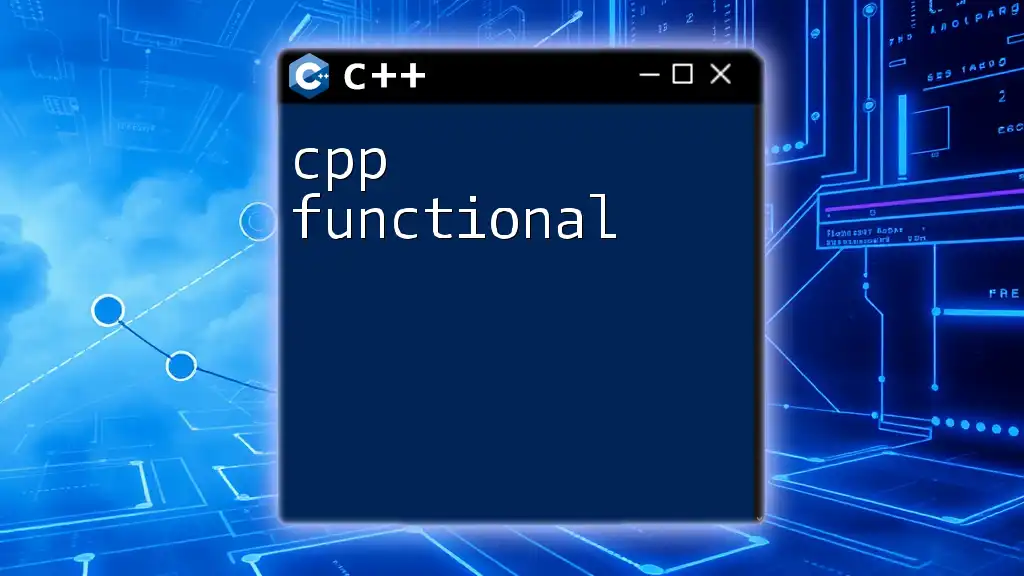
Conclusion
In this comprehensive guide on CPP calculation, we explored essential commands, functions, and examples for performing calculations efficiently. We covered fundamental concepts of data types, operators, and control flow to help ensure proper syntax and error handling.
The importance of practice cannot be overstated; engaging with these concepts will enable you to become proficient in performing calculations in C++.
Hopefully, this strong foundation will encourage you to dive deeper into CPP programming and develop your calculator programs, enhancing your computational capabilities in various applications.
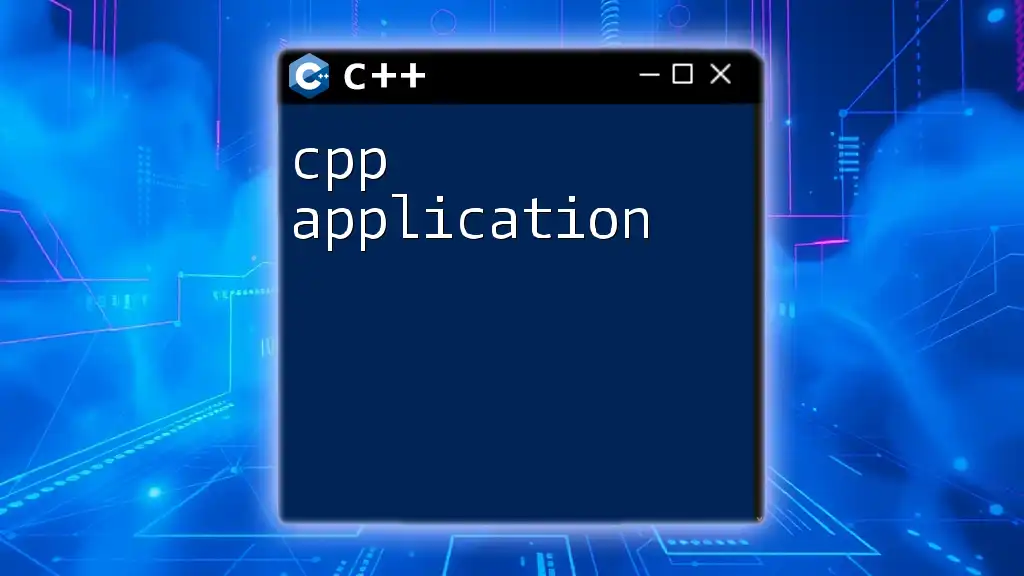
Additional Resources
For further growth in your CPP journey, do explore the official C++ documentation and recommended resources such as books and online courses. These materials will provide you with a broader understanding and mastery over C++ and computation.