An age calculator in C++ is a simple program that takes the user's birth year as input and calculates their age based on the current year.
Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
int birthYear, currentYear, age;
cout << "Enter your birth year: ";
cin >> birthYear;
cout << "Enter the current year: ";
cin >> currentYear;
age = currentYear - birthYear;
cout << "Your age is: " << age << " years" << endl;
return 0;
}
Understanding Age Calculation
Calculating age is a fundamental programming task that demonstrates your ability to work with date and time. The concept revolves around determining a person’s age based on their date of birth compared to the current date. In this section, we will explore the underlying mechanics of age calculation and why it's essential in programming.
A simplistic approach to age calculation is to subtract the birth year from the current year. However, this can lead to inaccuracies due to the varying number of days in months, leap years, and the actual day of the month. It’s crucial to account for these details to ensure accuracy in your final result.
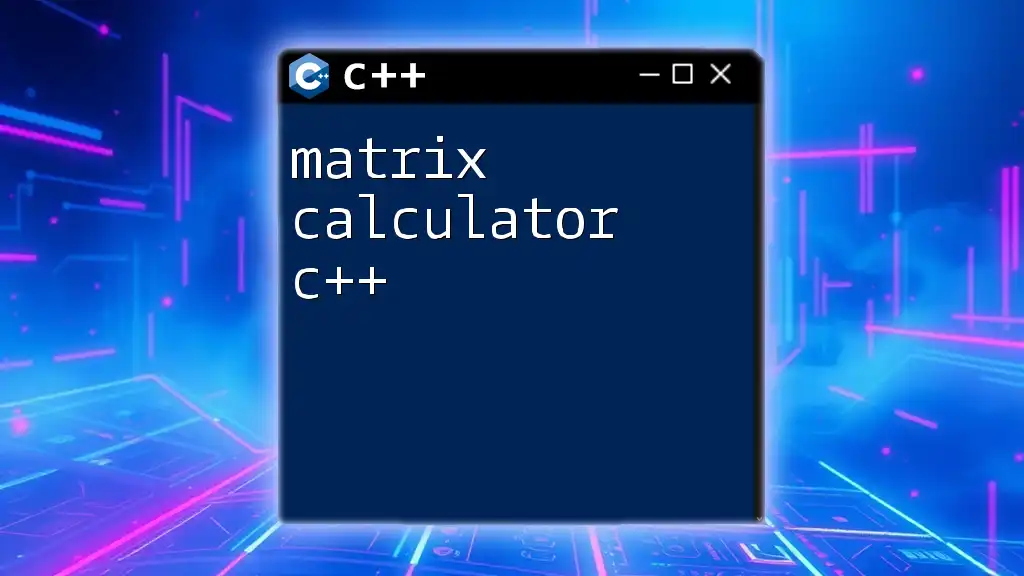
Key Concepts in C++
To effectively write an age calculator in C++, you need to be familiar with several key programming concepts:
Basic Data Types
C++ offers a rich set of data types. For an age calculator, the following types will be essential:
- `int`: Used to store the year, month, and day as these will always be whole numbers.
- `string` and `char`: Useful for handling text, such as displaying messages or storing input temporarily.
Date and Time Handling
Manipulating dates and times can be complex, but C++ offers built-in libraries that can simplify the process. The `<ctime>` library provides functionality to access the system's current time, which is vital for our age calculator.
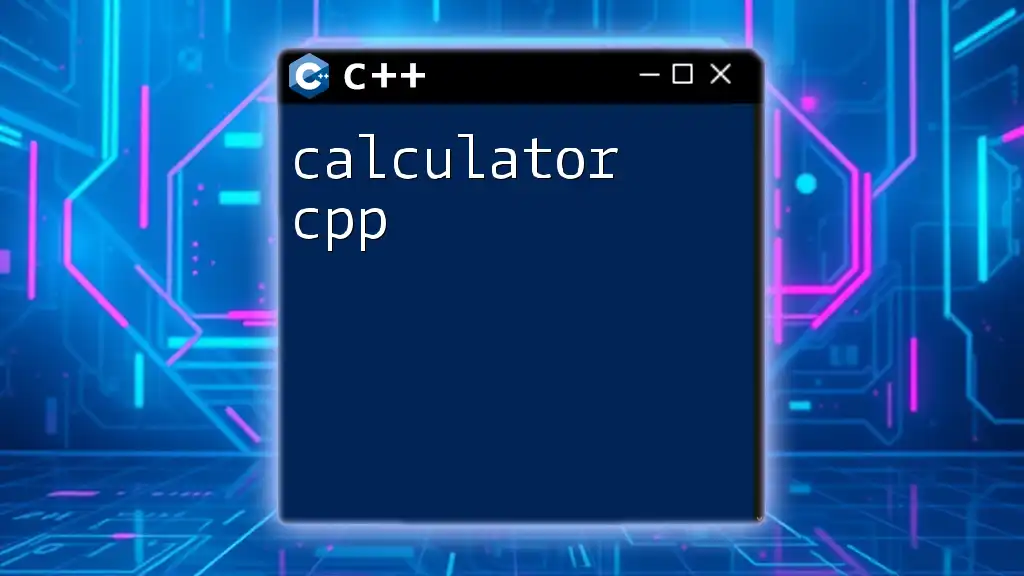
Setting Up the Environment
Before you start coding, ensure that you have a suitable development environment. Choose an Integrated Development Environment (IDE) or a simple code editor for your C++ programming. Popular choices include:
- Visual Studio
- Code::Blocks
- Dev-C++
Follow the installation instructions specific to your chosen tool, and you'll be ready to code your age calculator in no time.
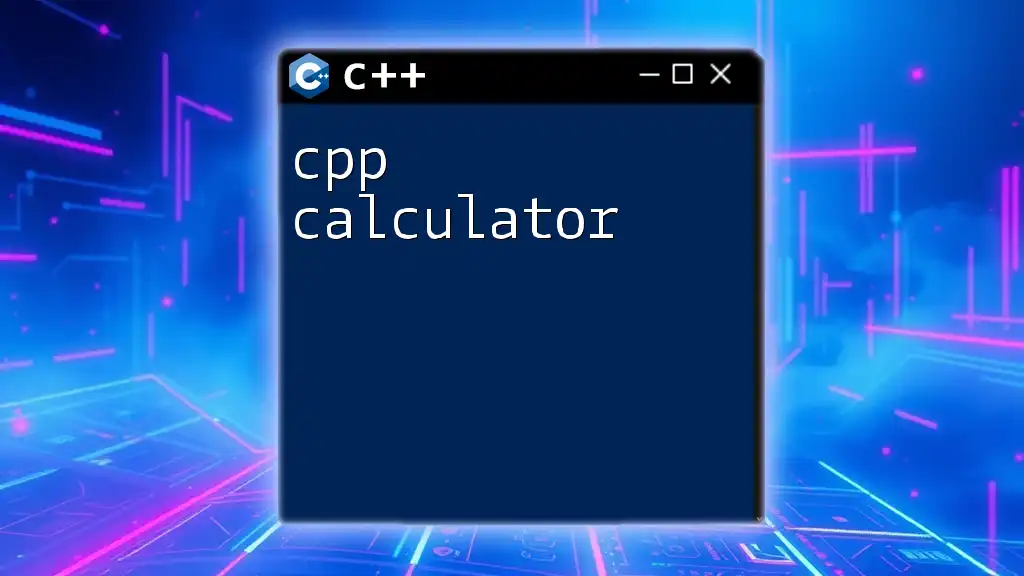
Writing the Age Calculator Program
Let’s break down the process of creating an age calculator in C++.
Step 1: Including Libraries
To make use of the date and time functionalities, we first need to include the necessary libraries in our program. The following code snippet illustrates this:
#include <iostream>
#include <ctime>
Step 2: Collecting User Input
Next, we need to collect the user's date of birth. We’ll use the `std::cin` command to obtain this data. It’s essential to prompt users clearly so that they understand what is expected:
int birthYear, birthMonth, birthDay;
std::cout << "Enter your birth year: ";
std::cin >> birthYear;
std::cout << "Enter your birth month: ";
std::cin >> birthMonth;
std::cout << "Enter your birth day: ";
std::cin >> birthDay;
Step 3: Getting the Current Date
After we have the user’s birth date, we need to extract the current date. This is done using the `<ctime>` library. The following code retrieves the current date and adjusts for the year and month, which may require some consideration:
time_t t = time(0); // Get current time
tm* now = localtime(&t); // Convert to local time structure
int currentYear = now->tm_year + 1900; // Adjust for 1900
int currentMonth = now->tm_mon + 1; // Adjust for 0 indexing
int currentDay = now->tm_mday;
Step 4: Calculating Age
Age is calculated by a straightforward formula; however, we must ensure correctness based on the current month and day. Here’s how this logic is implemented:
int age = currentYear - birthYear;
if (currentMonth < birthMonth || (currentMonth == birthMonth && currentDay < birthDay)) {
age--;
}
This logic is essential since it considers whether the birthday has occurred yet in the current year, allowing for accurate age representation.
Step 5: Displaying the Result
Finally, we display the calculated age to the user, using `std::cout`:
std::cout << "Your age is: " << age << " years old." << std::endl;
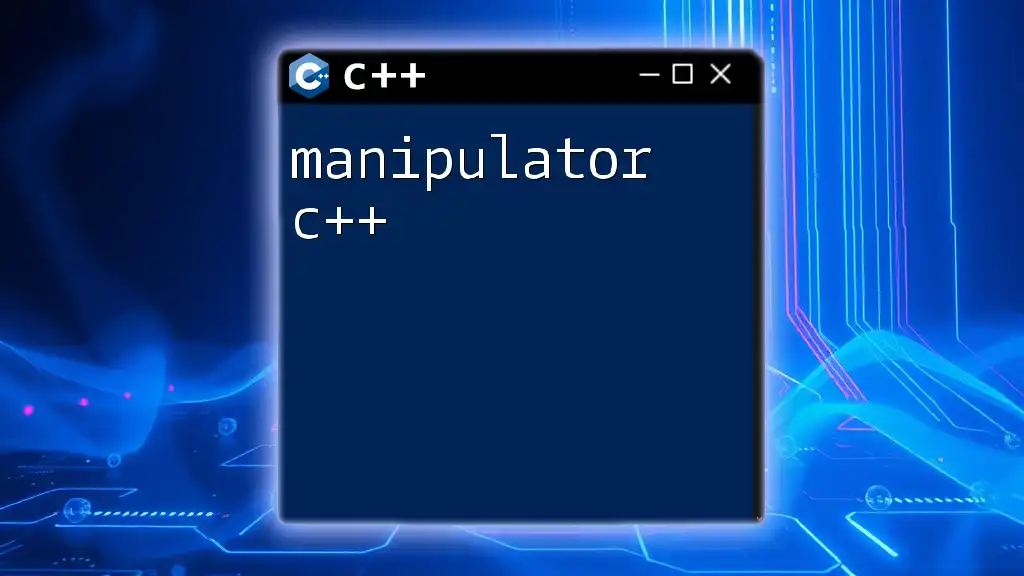
Full Example Code
Now that we've constructed each part of the program, here’s the complete version for your age calculator in C++:
#include <iostream>
#include <ctime>
int main() {
int birthYear, birthMonth, birthDay;
std::cout << "Enter your birth year: ";
std::cin >> birthYear;
std::cout << "Enter your birth month: ";
std::cin >> birthMonth;
std::cout << "Enter your birth day: ";
std::cin >> birthDay;
time_t t = time(0);
tm* now = localtime(&t);
int currentYear = now->tm_year + 1900;
int currentMonth = now->tm_mon + 1;
int currentDay = now->tm_mday;
int age = currentYear - birthYear;
if (currentMonth < birthMonth || (currentMonth == birthMonth && currentDay < birthDay)) {
age--;
}
std::cout << "Your age is: " << age << " years old." << std::endl;
return 0;
}
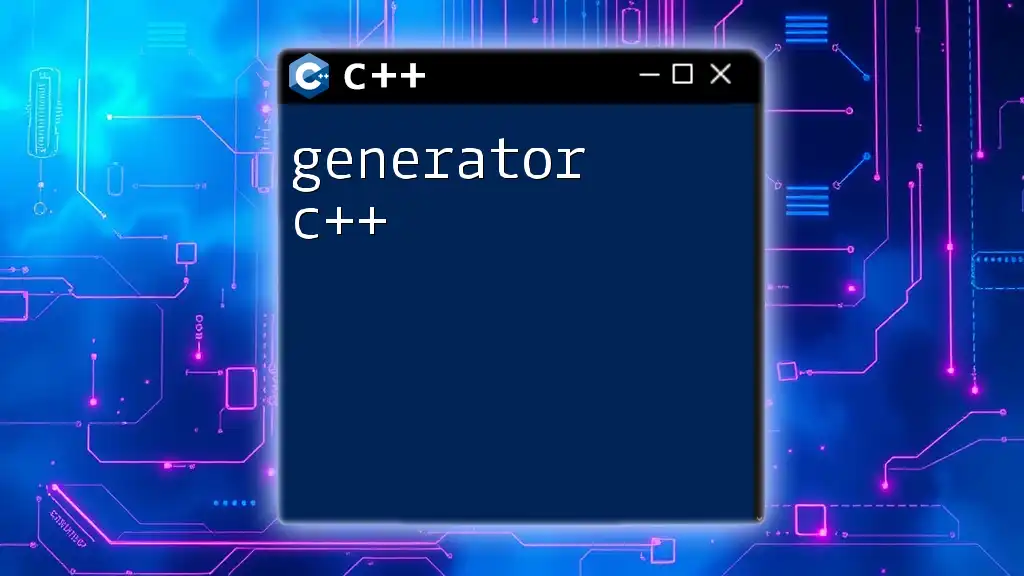
Common Errors and Troubleshooting
When developing your age calculator, you may encounter a few common pitfalls:
- Invalid Date Input: Ensure proper input validation is implemented to avoid crashes. For instance, check if the birth month is between 1 and 12 and if the days are appropriate for the specified month.
- Out-of-Bounds Errors: Always be cautious when performing operations on array-like data or user inputs. Make sure to sanitize your inputs accordingly.
Implementing user input validation will significantly improve your program and enhance user experience.
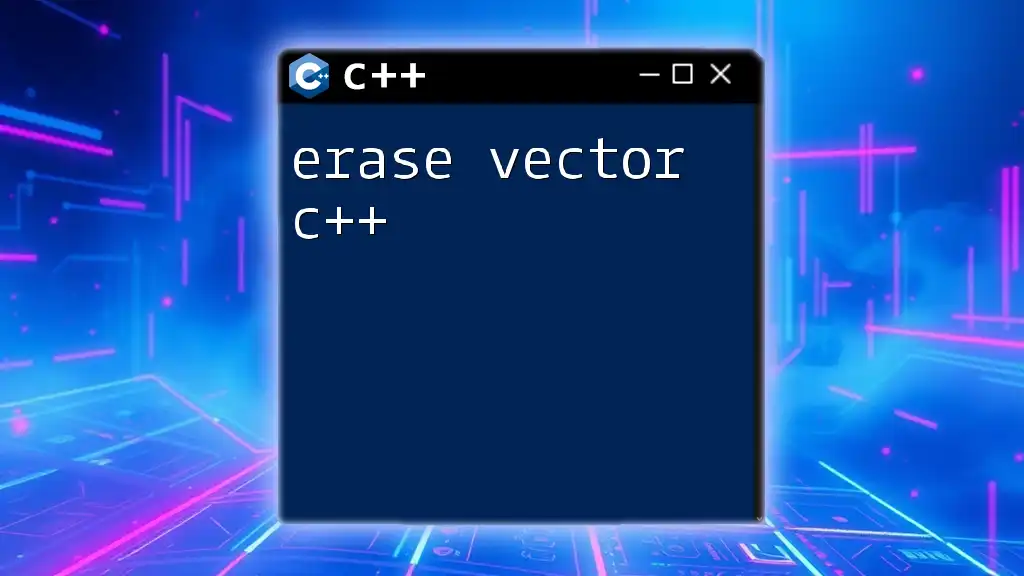
Enhancements and Future Improvements
Once you have your basic age calculator working, you may want to extend its functionality. Some ideas for enhancements include:
- Calculate exact age down to the hours and minutes.
- Include error handling to manage invalid user inputs gracefully.
- Utilize advanced libraries like `<chrono>` to handle dates more robustly, making your program more efficient and reliable.
These improvements will not only increase the complexity of your project but also significantly enhance your understanding of C++.
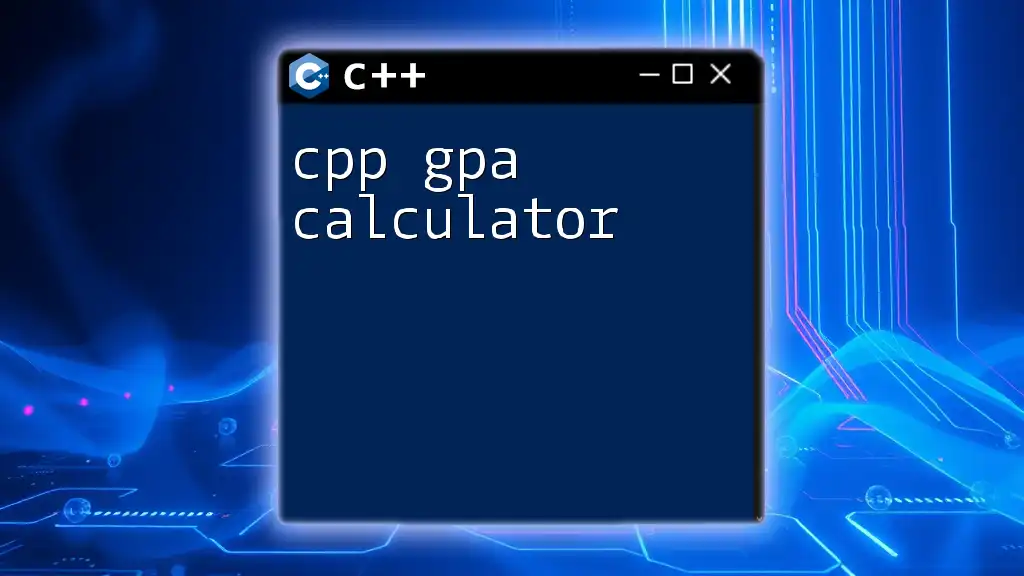
Conclusion
In this article, we covered the essential components of creating an age calculator in C++. We delved into how to retrieve user input, manipulate dates, and display results. Creating functional C++ applications enhances your skills and prepares you to tackle more complex programming challenges.
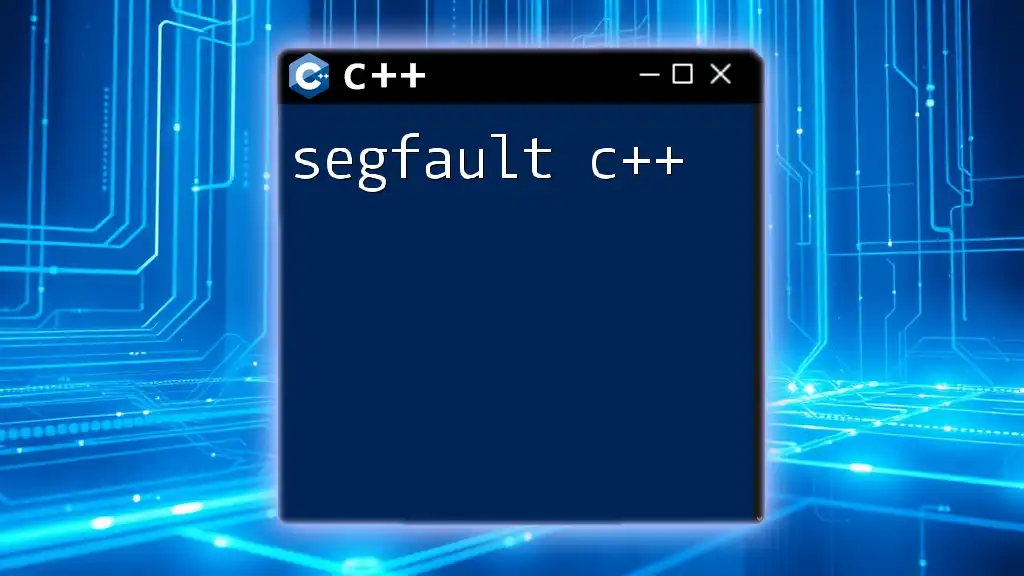
Call to Action
Now that you have the basic framework of an age calculator, I encourage you to modify and expand upon this project. Try adding new features or functionalities that make it more robust and user-friendly. Also, share your creations and subscribe for more C++ tutorials and tips to enhance your programming journey.
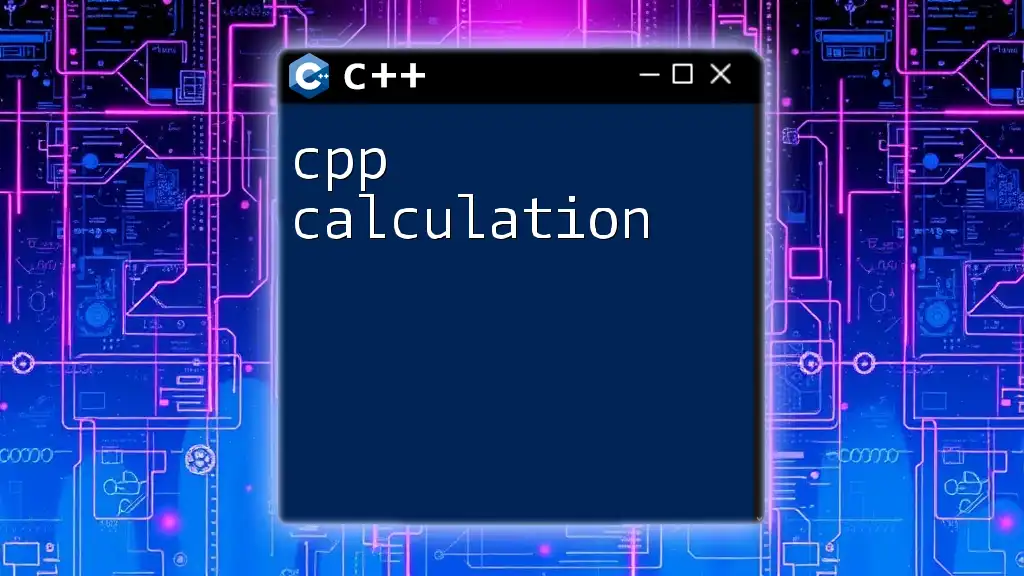
Additional Resources
For further learning, consider exploring the following resources:
- C++ documentation [cplusplus.com](http://www.cplusplus.com/)
- Online programming courses through platforms such as Udacity or Codecademy to deepen your knowledge in C++.