Eigenvalues in C++ can be computed using the Eigen library, which offers a straightforward approach to handle linear algebra problems efficiently. Here's a code snippet to demonstrate how to compute the eigenvalues of a matrix:
#include <iostream>
#include <Eigen/Dense>
int main() {
Eigen::Matrix2d mat;
mat << 1, 2, 2, 3;
Eigen::EigenSolver<Eigen::Matrix2d> solver(mat);
std::cout << "Eigenvalues:\n" << solver.eigenvalues() << std::endl;
return 0;
}
Understanding Eigenvalues
What are Eigenvalues?
An eigenvalue is a special scalar associated with a linear transformation represented by a square matrix. In mathematical terms, if A is a square matrix and v is a non-zero vector, then λ is an eigenvalue if it satisfies the equation:
\[ A \cdot v = \lambda \cdot v \]
Here, v is an eigenvector corresponding to the eigenvalue λ. The concept of eigenvalues is crucial in various fields, including physics (where they describe stability), economics (in equilibrium models), and computer science, particularly in algorithms involving data analysis and machine learning, such as Principal Component Analysis (PCA).
The Role of Eigenvalues in C++
Understanding and calculating eigenvalues in C++ is not only essential for theoretical disciplines but also practical applications such as numerical simulations and optimization problems. C++ provides robust libraries that simplify working with eigenvalues, making it easier to implement complex mathematical concepts efficiently.
Libraries in C++ for Eigenvalues
One of the most widely used libraries for linear algebra is Eigen. This powerful C++ template library provides a rich set of features, including efficient ways to compute eigenvalues and eigenvectors. Another alternative is Armadillo, which also offers a coherent API and is highly optimized for performance.

How to Compute Eigenvalues in C++
Setting Up Your Environment
To work with eigenvalues in C++, you'll need to install the Eigen library. Eigen is a header-only library, which means you can simply download it and include it in your project. Here’s how to do it:
- Download the Eigen library from its [official website](https://eigen.tuxfamily.org).
- Unzip the downloaded file and place it in a location accessible to your C++ project.
- Include the Eigen header in your code as shown below:
#include <Eigen/Dense>
using namespace Eigen;
Basic Eigenvalue Calculation
Creating a Matrix
To compute eigenvalues, you first need to create a matrix. Here’s a simple example of initializing a 2x2 matrix:
Matrix2d mat;
mat << 1, 2,
3, 4;
In this code, we create a matrix mat and initialize it with the specified values. It's important to understand the structure of your matrix as it directly impacts the calculations that follow.
Using Eigen Library for Eigenvalues
Once you have your matrix set up, you can proceed to compute its eigenvalues.
Here’s how you can apply the Eigen library to compute the eigenvalues:
Eigen::EigenSolver<Matrix2d> es(mat);
std::cout << "Eigenvalues:\n" << es.eigenvalues() << std::endl;
In this example, we create an `EigenSolver` for our matrix `mat` and then retrieve the eigenvalues using `es.eigenvalues()`. This simple yet powerful approach saves time by leveraging the library’s built-in functionality.
Exploring Real and Complex Eigenvalues
When dealing with various types of matrices, you may encounter complex eigenvalues. Identifying whether your eigenvalues are real or complex is crucial, especially in stability analysis and control theory.
Example Code Snippet: Complex Eigenvalues Calculation
If your matrix has complex entries, you can calculate complex eigenvalues as follows:
Matrix2cd mat_complex;
mat_complex << 1, 2,
3, 4;
Eigen::ComplexEigenSolver<Matrix2cd> ces(mat_complex);
std::cout << "Complex Eigenvalues:\n" << ces.eigenvalues() << std::endl;
This code snippet demonstrates creating a complex matrix and computing its eigenvalues. Using `ComplexEigenSolver`, you can easily obtain both real and imaginary components of the eigenvalues.
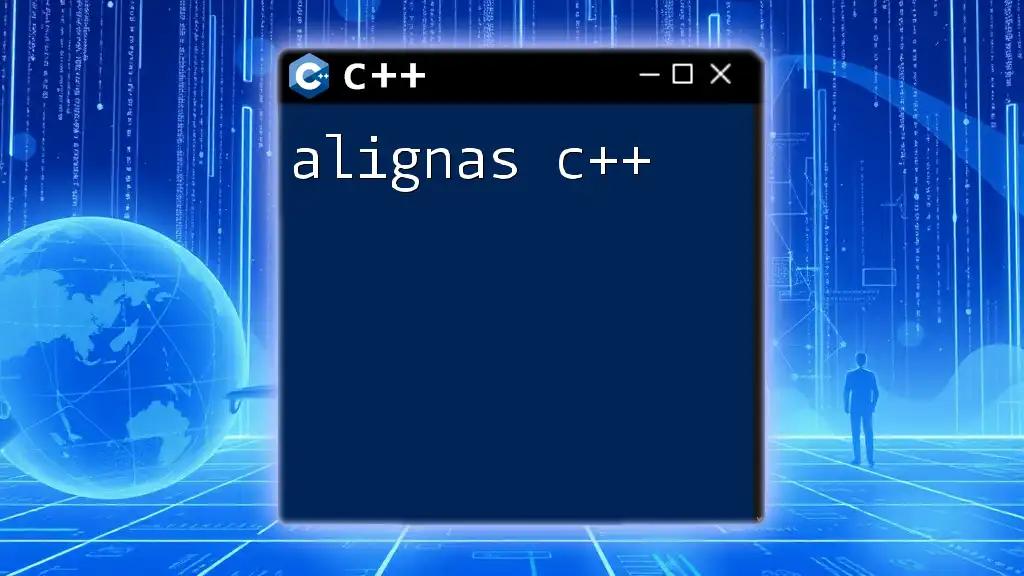
Advanced Eigenvalue Problems
Handling Large Matrices
Calculating eigenvalues for large matrices can be computationally intensive and inefficient if not handled correctly. The Eigen library implements several optimized algorithms for dealing with larger datasets. It allows for block decomposition and iterative solvers that significantly enhance performance.
Eigenvalue Decomposition
Eigenvalue decomposition is the process of decomposing a matrix into its eigenvalues and eigenvectors. This decomposition has various applications, including simplifying matrix operations and solving systems of differential equations.
Here's how to leverage the SelfAdjointEigenSolver in Eigen:
SelfAdjointEigenSolver<MatrixXd> solver;
solver.compute(mat);
std::cout << "Eigenvalues from SelfAdjointEigenSolver:\n" << solver.eigenvalues() << std::endl;
This code uses `SelfAdjointEigenSolver`, which is particularly efficient for symmetric matrices. This approach guarantees real eigenvalues and is usually the preferred method in applications involving real symmetric matrices.
Practical Applications of Eigenvalues in C++
Eigenvalues have significant applications in various domains. Here are some notable examples:
-
Principal Component Analysis (PCA): In machine learning, eigenvalues help reduce the dimensionality of data while preserving its essence, leading to faster algorithms and clearer insights.
-
Stability Analysis in Dynamical Systems: Analyzing eigenvalues of the system matrix provides insight into system behavior in control theory and engineering, determining system stability and response characteristics.
Code Snippet: A Simple PCA Implementation
Here’s a simplified version of a PCA implementation using eigenvalues:
// Load your data into a matrix 'data' (size m x n)
// 1. Standardize the dataset (mean normalization)
// 2. Compute the covariance matrix
MatrixXd covariance = (data.transpose() * data) / (data.rows() - 1);
// 3. Calculate eigenvectors and eigenvalues
SelfAdjointEigenSolver<MatrixXd> solver(covariance);
std::cout << "Eigenvalues for PCA:\n" << solver.eigenvalues() << std::endl;
In this example, we standardize the dataset, compute the covariance matrix, and then apply Eigen’s `SelfAdjointEigenSolver` to find the eigenvalues essential for PCA analysis.

Best Practices and Tips for Using Eigenvalues in C++
Debugging Common Issues
While working with eigenvalues, you may encounter several common issues, such as:
- Inconsistent eigenvalues due to matrix properties: Ensure that your matrix is square and well-conditioned.
- Performance concerns with large matrices: Always consider the efficiency of your chosen algorithms and data structures.
Performance Optimization
Optimizing your calculation for speed and efficiency is crucial, especially when dealing with large datasets. Here are some best practices:
- Use appropriate data structures: Efficient use of matrices, sparse matrices, or even specialized data types can significantly impact performance.
- Avoid unnecessary calculations: Reduce computations by leveraging existing results, especially when the eigenvalue calculations are part of iterative algorithms.
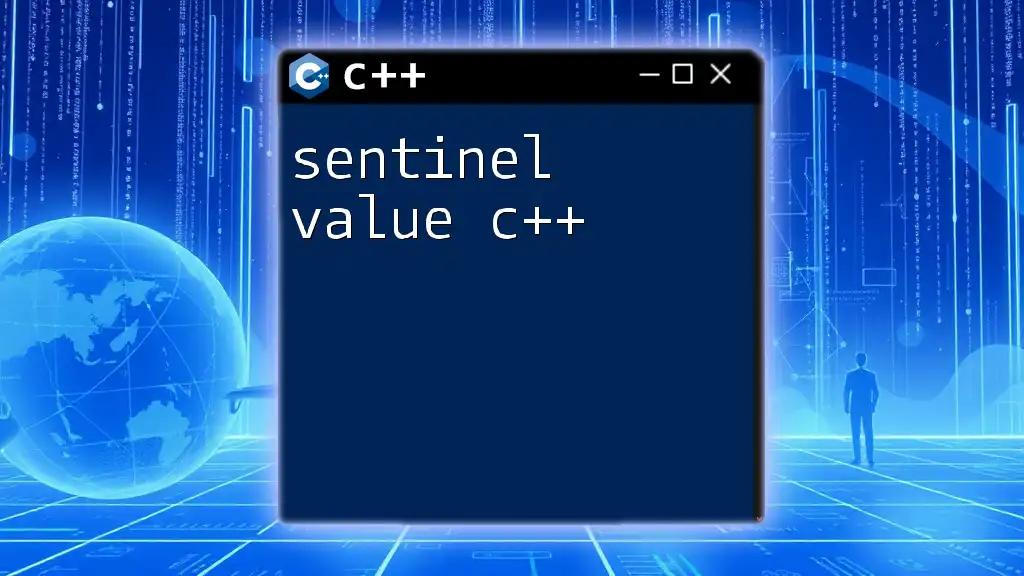
Conclusion
In summary, understanding and calculating eigenvalues in C++ is invaluable for programming in domains requiring linear algebra, data analysis, and machine learning. Utilizing libraries like Eigen allows for efficient and effective calculations, making complex mathematical concepts more accessible. By mastering the intricacies of eigenvalues and their computation, you open doors to powerful applications that span across various fields.
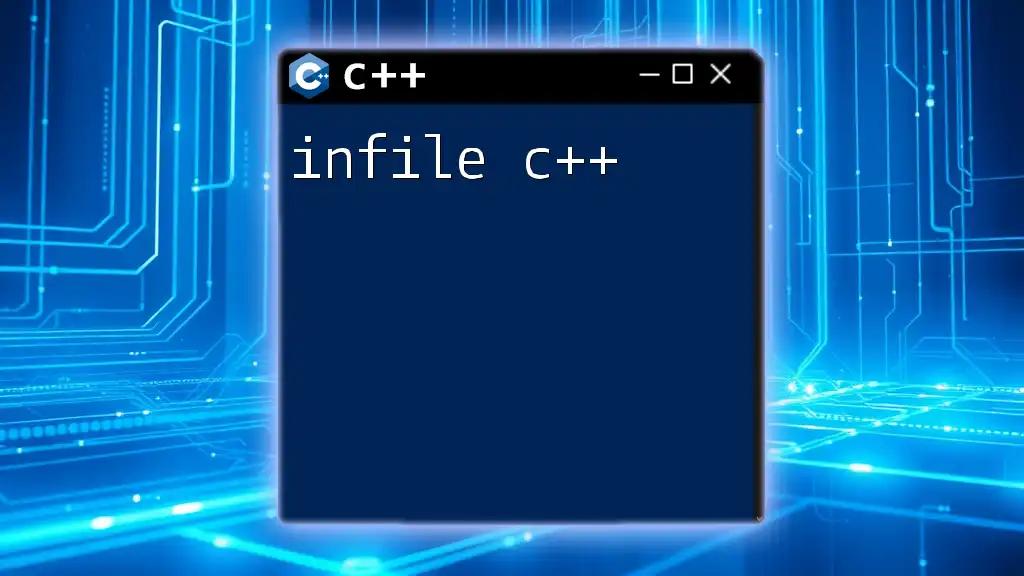
Additional Resources
For further exploration of eigenvalues in C++, consider checking the following resources:
- Official Eigen library documentation
- Recommended textbooks focusing on linear algebra and computational mathematics
- Online courses that guide you through practical applications of eigenvalues and C++ programming
FAQs about Eigenvalues in C++
-
What are common use cases for eigenvalues?
- Common use cases for eigenvalues include stability analysis, PCA in data science, and solving differential equations in physics.
-
Can I compute eigenvalues for non-square matrices?
- No, eigenvalues are only defined for square matrices. Non-square matrices do not have eigenvalues in the traditional sense.