The `signum` function in C++ is used to determine the sign of a number, returning -1 for negative values, 1 for positive values, and 0 for zero. Here’s how you can implement it:
#include <iostream>
int signum(int x) {
return (x > 0) - (x < 0); // Returns 1 for positive, -1 for negative, and 0 for zero
}
int main() {
int num = -10;
std::cout << "The sign of " << num << " is " << signum(num) << std::endl;
return 0;
}
Understanding the Basics of the Sign Function
The sign function, often referred to as the signum function, is a fundamental mathematical function used widely in programming, especially in C++. Mathematically, the function can be represented as:
sgn(x) =
- 1 if x > 0
- 0 if x = 0
- -1 if x < 0
This simple yet powerful definition enables developers to determine the sign of a number easily. When programming, scenarios such as determining the direction of a force, validating input values, or simplifying complex algorithms may necessitate the use of the sign function. Understanding how to implement and apply this function can greatly enhance your code's efficiency and clarity.
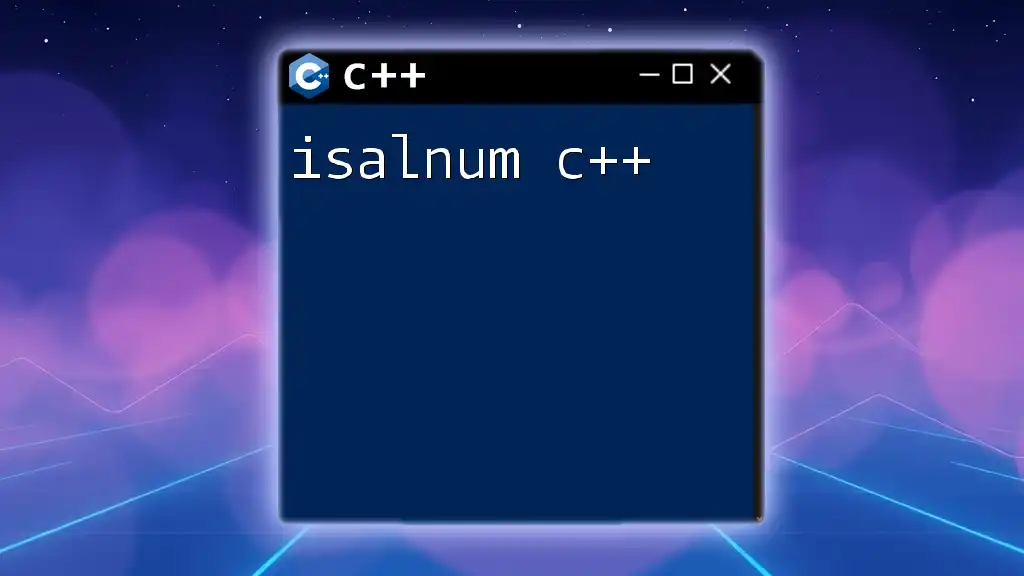
Implementing the Sgn Function in C++
Creating a Simple Sgn Function
Creating a basic implementation of the sgn function in C++ is straightforward. A simple version may look like this:
int sgn(int x) {
return (x > 0) - (x < 0);
}
In this example, we leverage the comparison operations to evaluate the integer `x`:
- If `x` is greater than 0, we return 1.
- If `x` is less than 0, we return -1.
- If `x` equals 0, the expression evaluates to 0.
This implementation is compact and efficient, allowing for quick determination of the sign of an integer.
Expanding the Functionality
The initial implementation caters only to integers. To accommodate various data types such as floating-point numbers, we can modify the function using C++ templates, like this:
template <typename T>
int sgn(T x) {
return (x > T(0)) - (x < T(0));
}
This template-based `sgn` function is versatile, accepting any type that supports comparison operators. The advantages of using templates here include:
- Type Safety: The function automatically adjusts to work with any data type, enhancing maintainability.
- Code Reusability: One function can serve multiple contexts without code duplication.
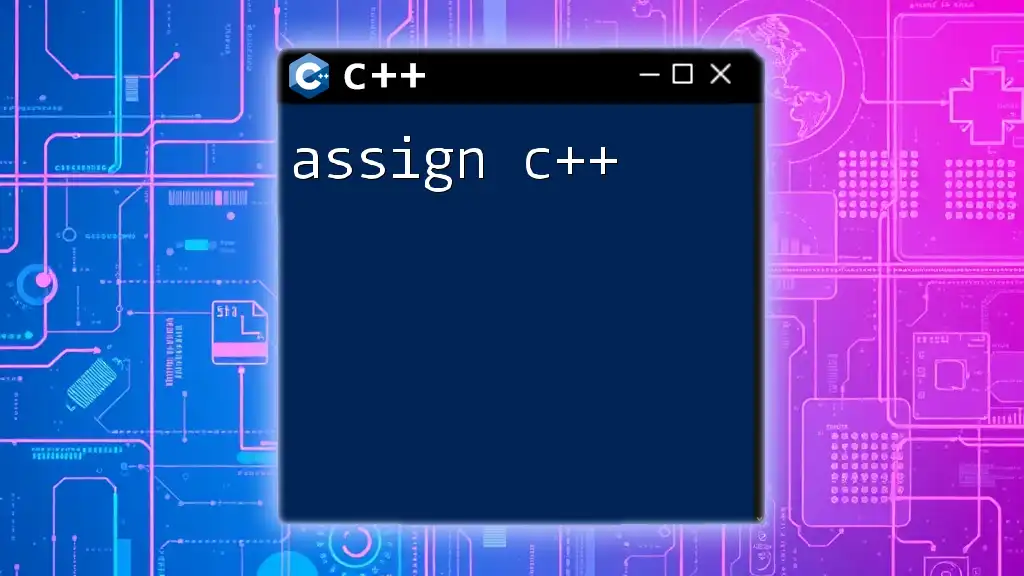
Real-World Applications of the Sign Function
The signum function finds practical applications across various programming domains. Here’s a look at some scenarios:
Machine Learning
In machine learning, the sign function might be used in gradient calculations to determine the direction of descent during optimization.
Physics Simulations
In simulations involving physics, the sign function can efficiently calculate acceleration, direction, and other dynamics based on forces acting upon objects.
Game Development
When controlling character movements within a game, the sign function helps determine whether to move left or right based on user input.
Example: Using Sign Function in a Physics Context
Consider a function that applies a force to an object based on its mass:
void applyForce(float mass, float force) {
float acceleration = sgn(force) * mass;
// Further calculations...
}
In this example, the sign function helps ascertain the direction of acceleration by factoring in the force applied. This principle can extend to more complex physics-based simulations, underpinning the importance of the sign function in real-time calculations.
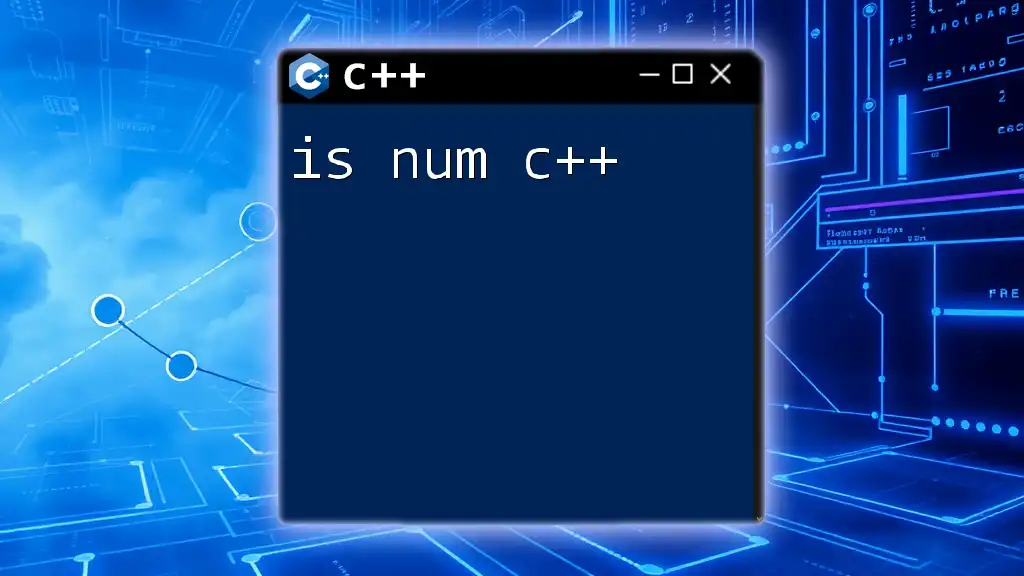
Optimizing Your C++ Code with the Signum Function
Best Practices for Using Sgn Function
While using the signum function, emphasis should be placed on readability and maintainability. Ensuring that your code is easily understood by others (or yourself in the future) is crucial for good software engineering practices. Keep the code as simple as possible, leveraging the efficiency of the `sgn` function when needed.
Common Pitfalls to Avoid
When implementing the sign function, developers should be mindful of several common pitfalls:
- Ignoring Edge Cases: Neglecting to account for special cases like NaN (Not a Number) can lead to unpredictable behavior.
- Excessive Function Calls: In performance-critical sections of code, excessive calls to the `sgn` function can degrade performance. Consider computing the sign in one place and reusing the result where necessary.
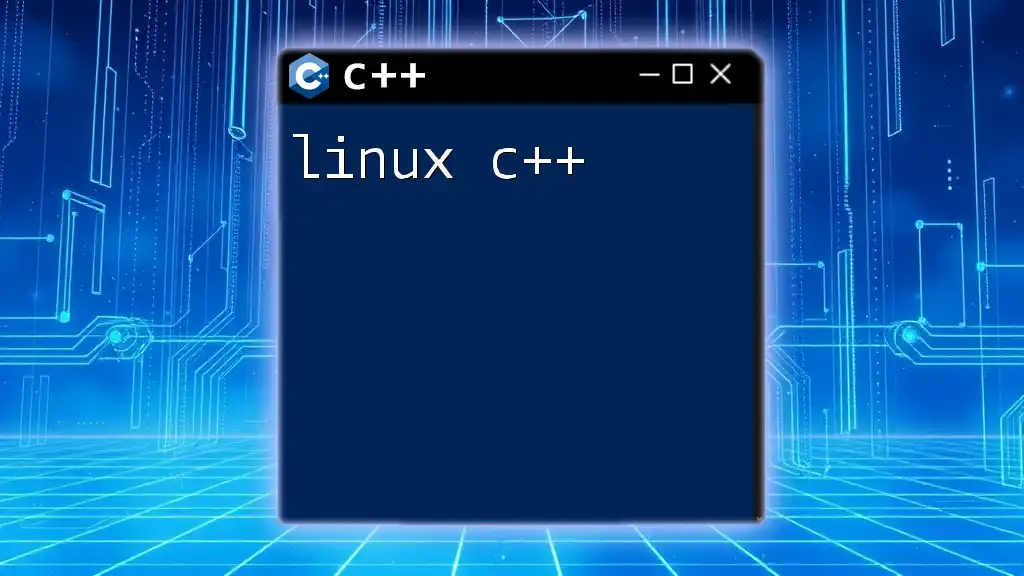
Customizing the Sign Function
Overloading the Sign Function
To provide maximum versatility, you can overload the `sgn` function for different data types:
int sgn(int x);
float sgn(float x);
double sgn(double x);
Overloading ensures that your function can handle different numeric types while keeping your code organized.
Handling Edge Cases
It’s essential to manage edge cases appropriately. For instance, differentiating between `0` and NaN in your implementations can prevent bugs and logical errors in your programs:
int sgn(float x) {
if (std::isnan(x)) return 0; // Define your policy for NaN
return (x > 0) - (x < 0);
}
This addition helps make your function more robust, enabling it to process a wider variety of inputs effectively.
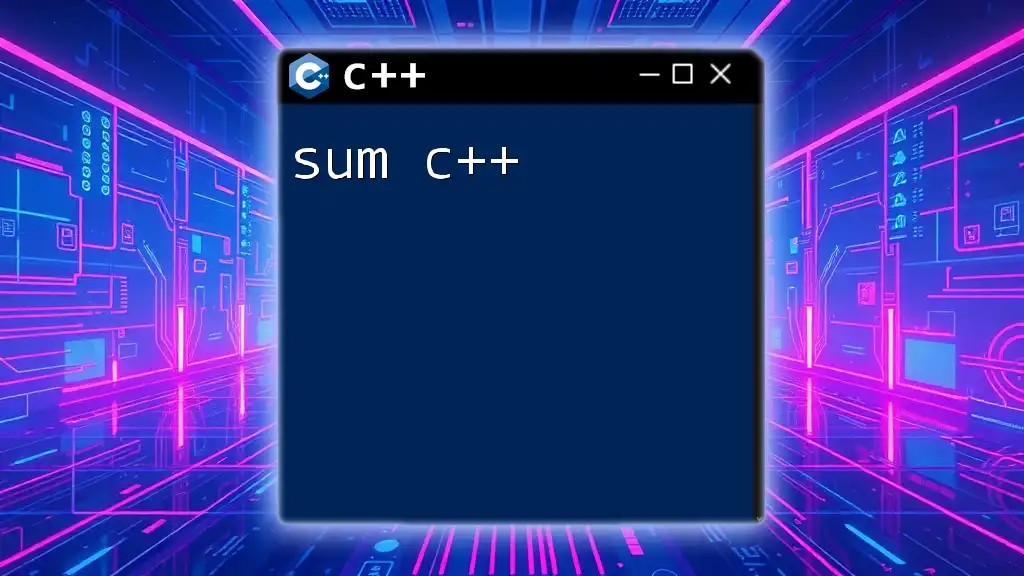
Performance Considerations
When considering performance, it’s essential to weigh the pros and cons of employing a custom sign function against using built-in alternatives. In most cases, the gains from tailored logic in the sign function can outweigh potential performance drawbacks, especially when leveraging C++ templates for flexibility.
In performance-critical applications, always benchmark different approaches to identify the most efficient method for your specific use case.
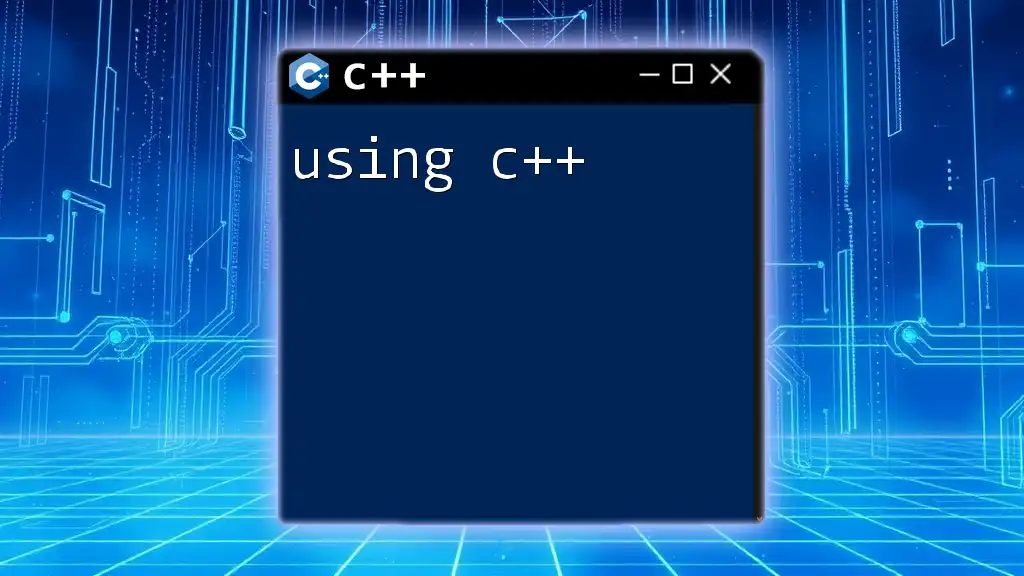
Conclusion
The signum function in C++ is an invaluable tool for any developer looking to simplify their programming logic. Whether applied to machine learning, physics simulations, or character movements in games, its versatility can significantly enhance your code's readability and efficiency. By understanding how to implement and customize the sgn function, you can dramatically improve your C++ projects and drive better outcomes.
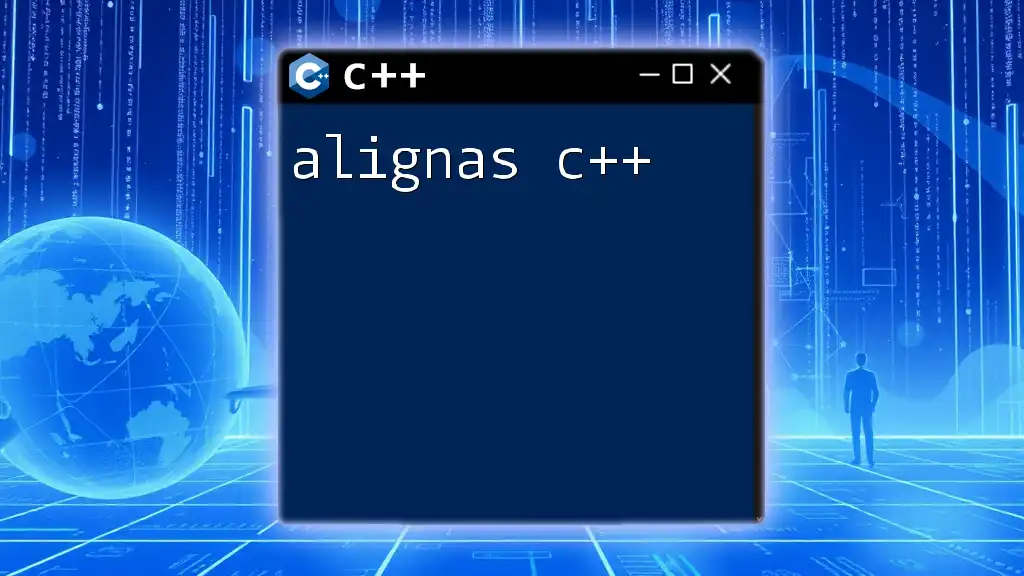
Further Reading and Resources
To deepen your understanding of the sign function and its myriad applications, consider exploring programming textbooks, online tutorials, and documentation on mathematical functions in C++. Staying abreast of evolving best practices will continue to refine your skills and expertise in C++.
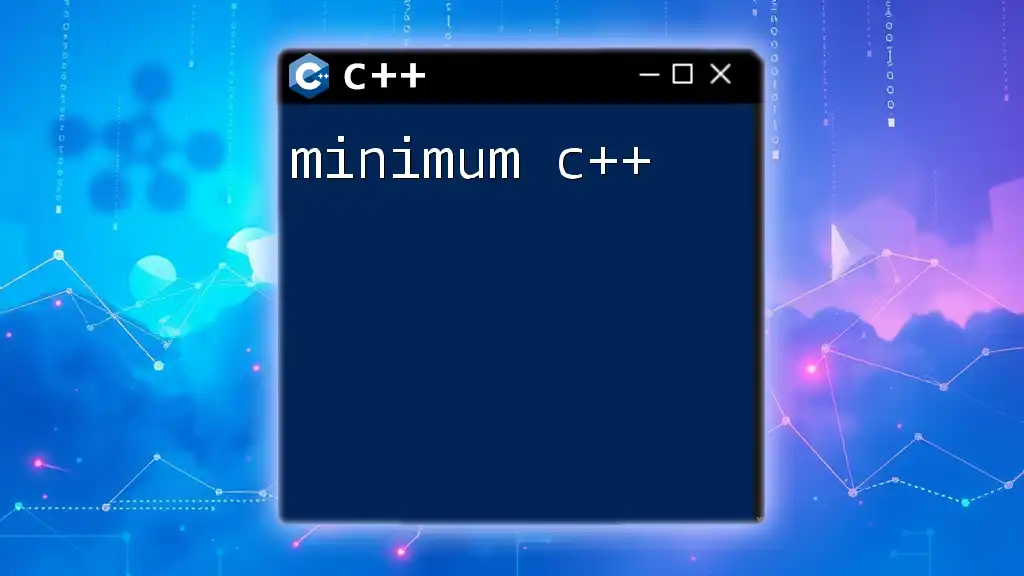
FAQs About the Signum Function in C++
-
What is the difference between the sign function and the absolute value? The sign function indicates the sign of a number, while the absolute value returns the magnitude without regard to sign.
-
Can the sign function be integrated into a class? Yes, encapsulating the sign function within a class can be done using static methods or member functions, allowing for even more structured code design.